With the datetime class in Python, you get powerful tools for manipulating and viewing time and date. Whether you need the current time, want to calculate time intervals, or take different time zones into account – the datetime module provides a clear and effective solution.
Here you will learn how to use this module to easily manipulate and display time data. Follow the steps below to understand and apply the various properties and functions of datetime in Python.
Key Takeaways
- The datetime module allows for the representation and manipulation of time and date.
- There are various classes within the module such as datetime, time, and date.
- Time and date values can be easily created, displayed, and compared.
- Time differences can be determined to, for example, calculate age or remaining time.
Step-by-Step Guide
1. Import the Module
To utilize the functions of datetime, you first need to import the module. Use the following line in your code:
You can do this in your Python environment. Once you have imported the module, you are ready to work with time and date.
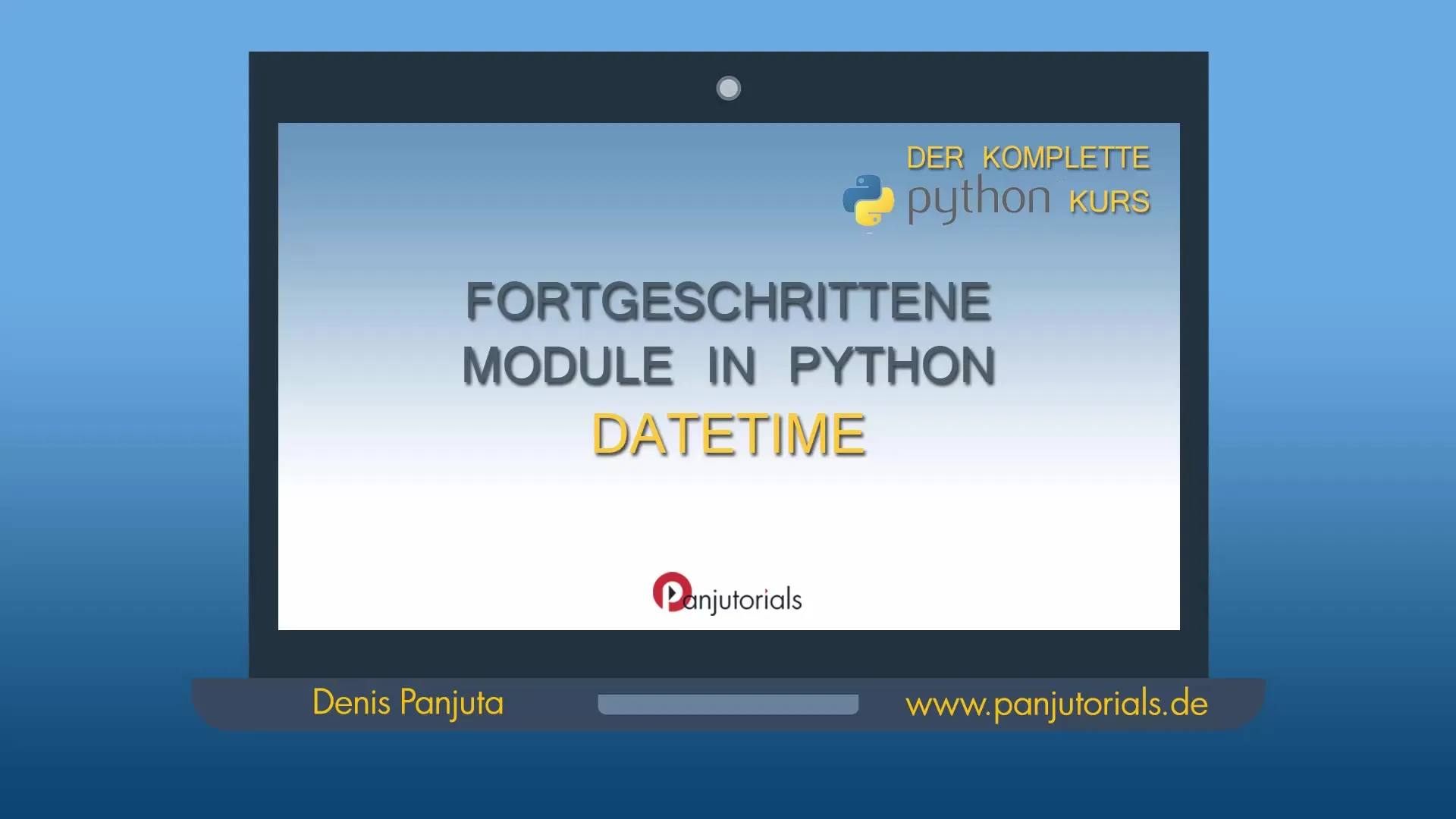
2. Create a Time Instance
To specify a specific time, you can create a time object.
This call creates a time instance that you can use in your program.
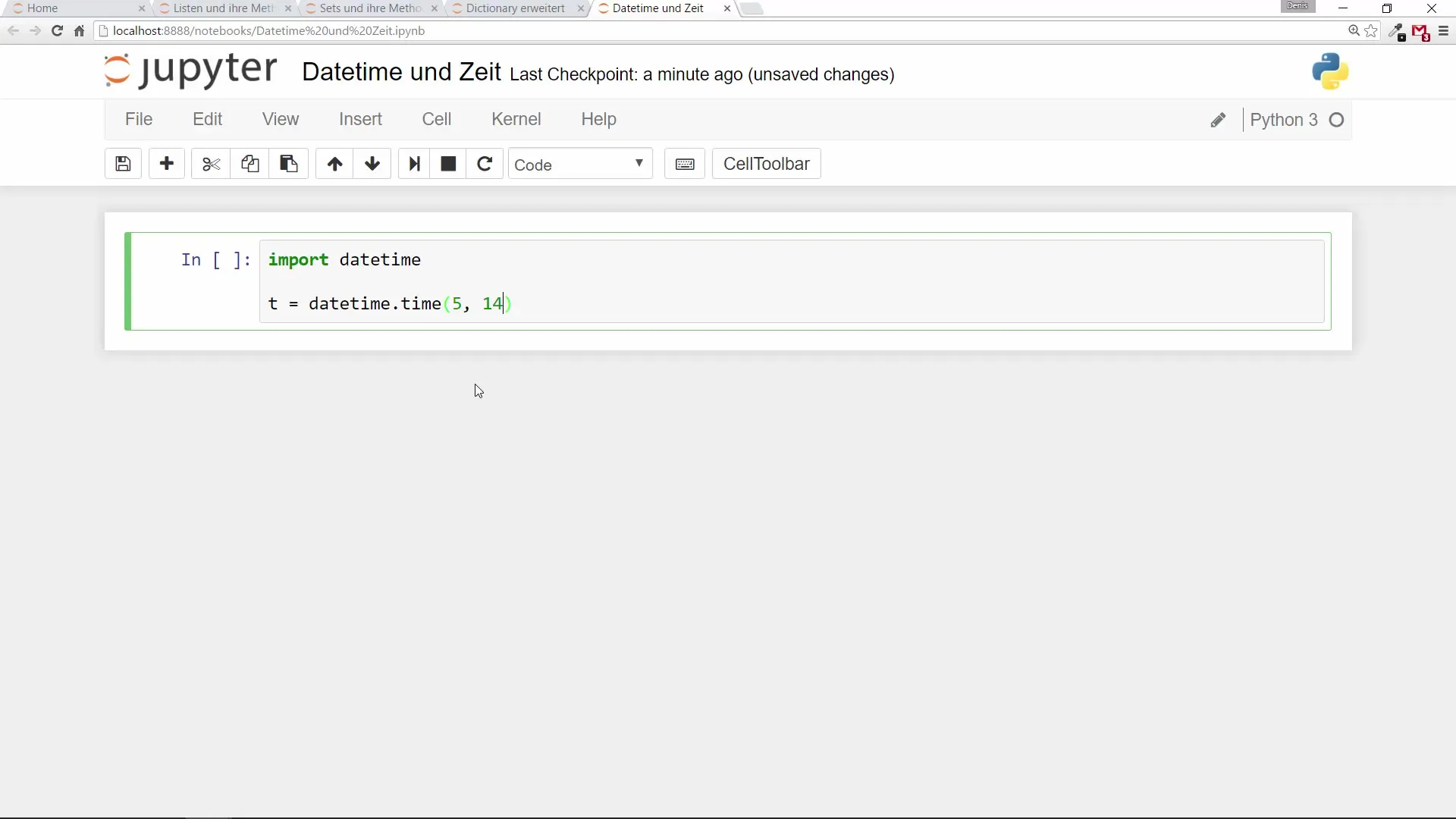
3. Access Individual Time Components
To retrieve specific elements of time, you can use.hour,.minute,.second, and.microsecond.
Each of these commands returns the corresponding time values, which are now easily accessible.
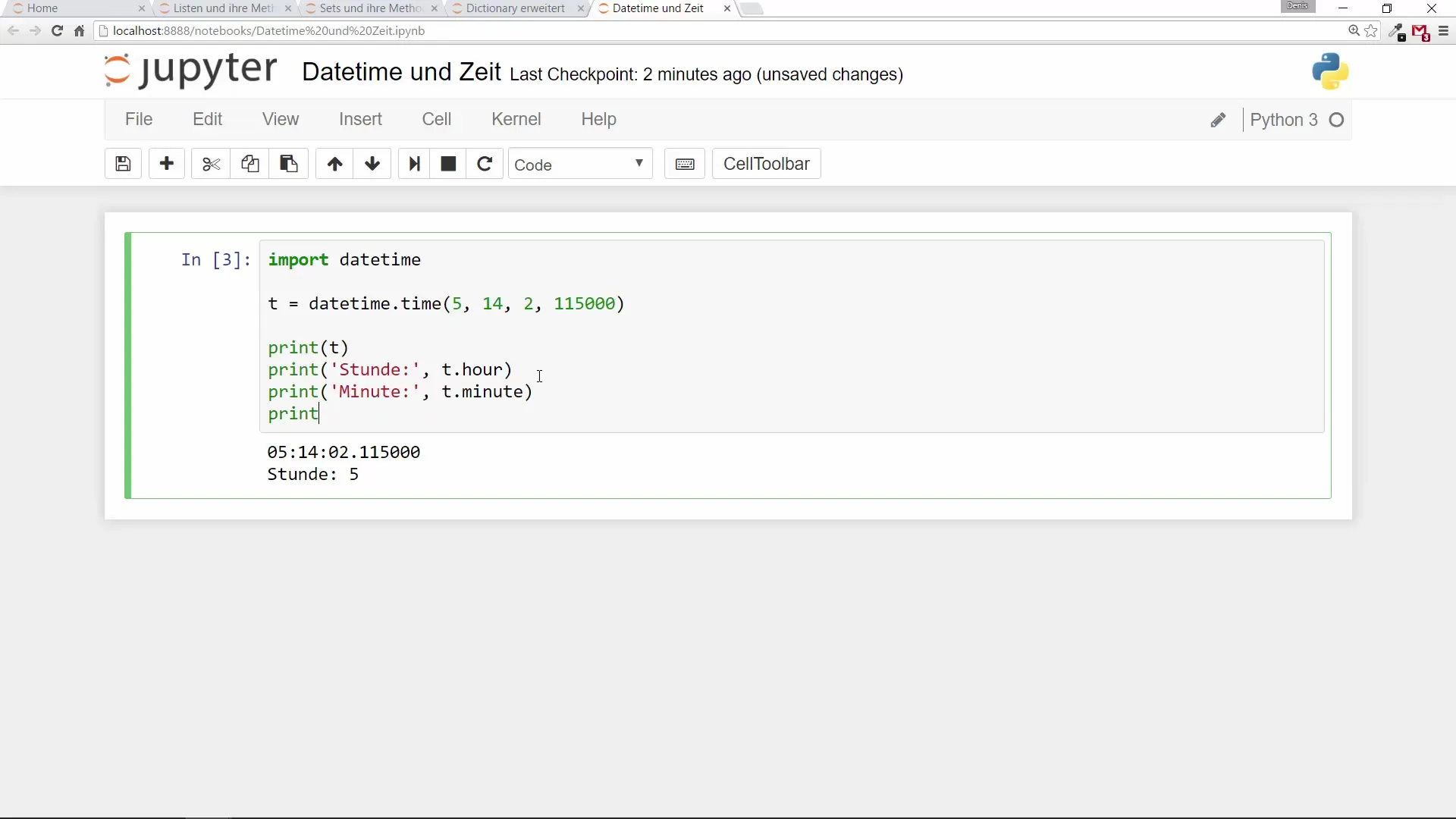
4. Create a Date with Time
If you also want to use the date, you need to utilize the datetime class.
This command captures both the date and time, which may be important in your programs. Here you can also query the individual components of the date.
5. Query the Current Date
To retrieve today's date, you can use the today() method.
This will give you the current date, such as 2023-10-25, depending on the actual date when the code is executed.
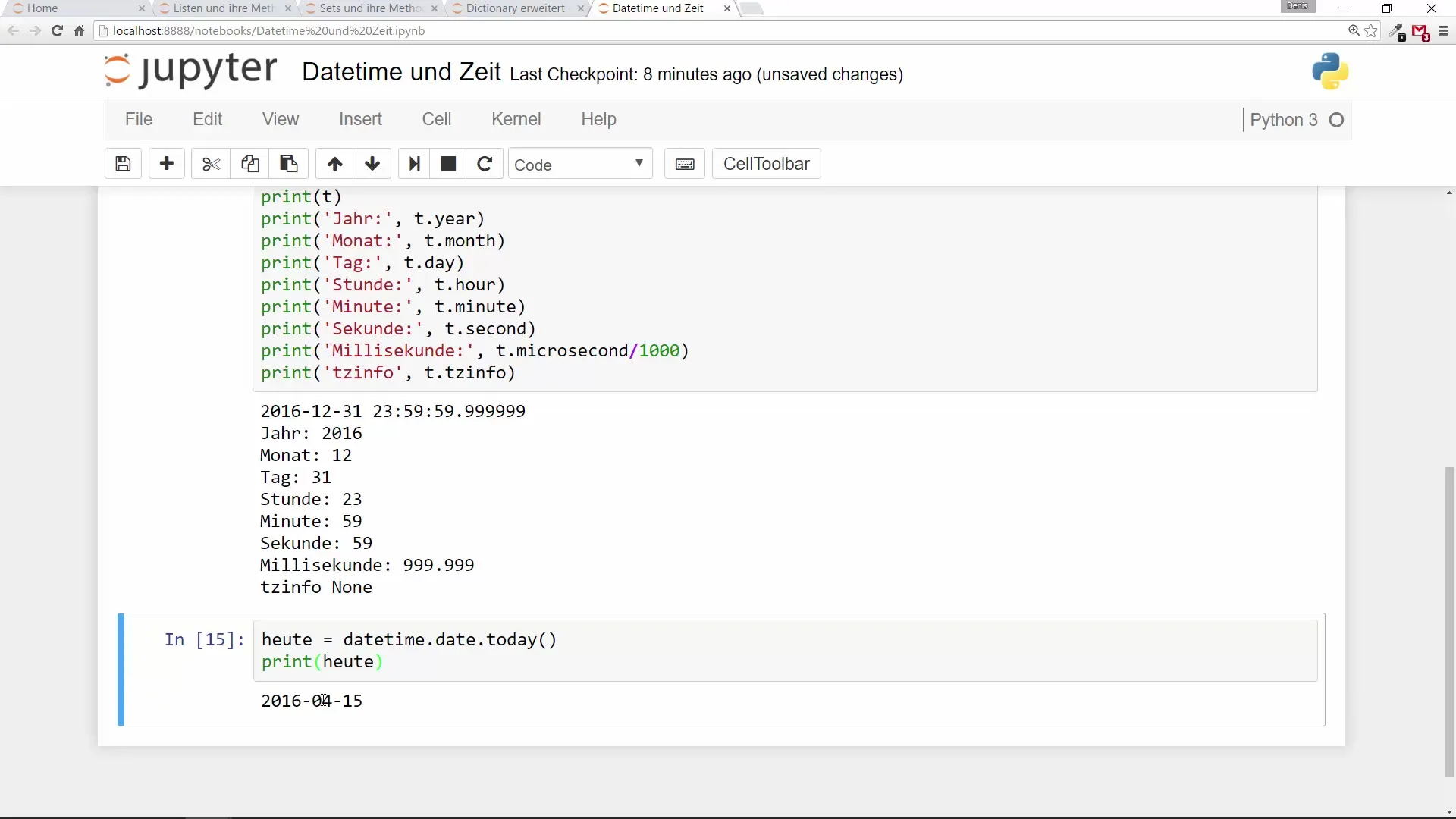
6. Calculate Time Differences
The datetime module provides a simple way to calculate the difference between two points in time.
The result shows you how many days are between the two dates, which can be useful in many applications.
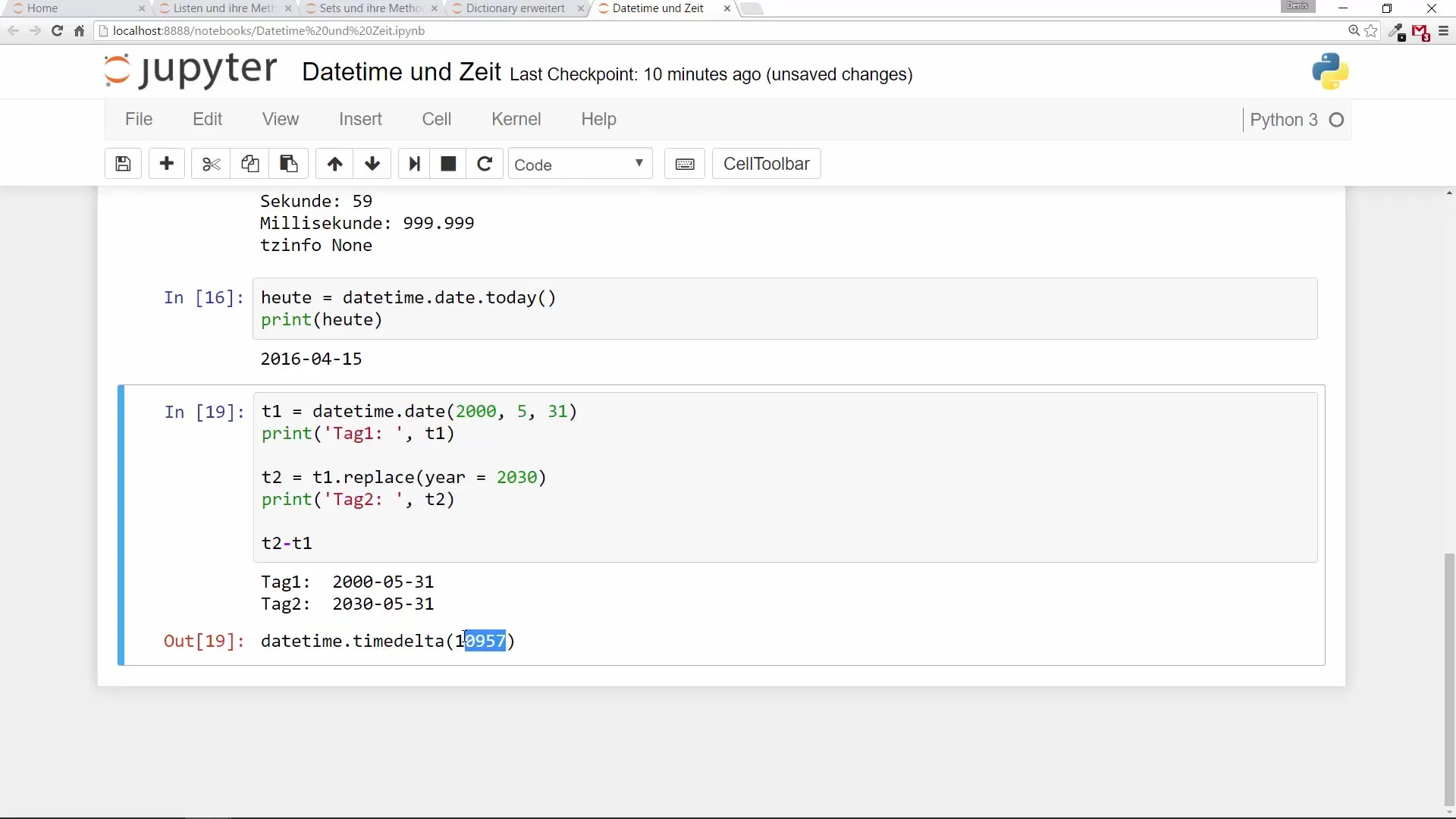
7. Using Time Zones
To use time zones, you can integrate the pytz module.
local_timezone = pytz.timezone('Europe/Berlin') local_time = datetime.datetime.now(local_timezone) print(local_time)
The above snippet will give you the current time in the defined time zone.
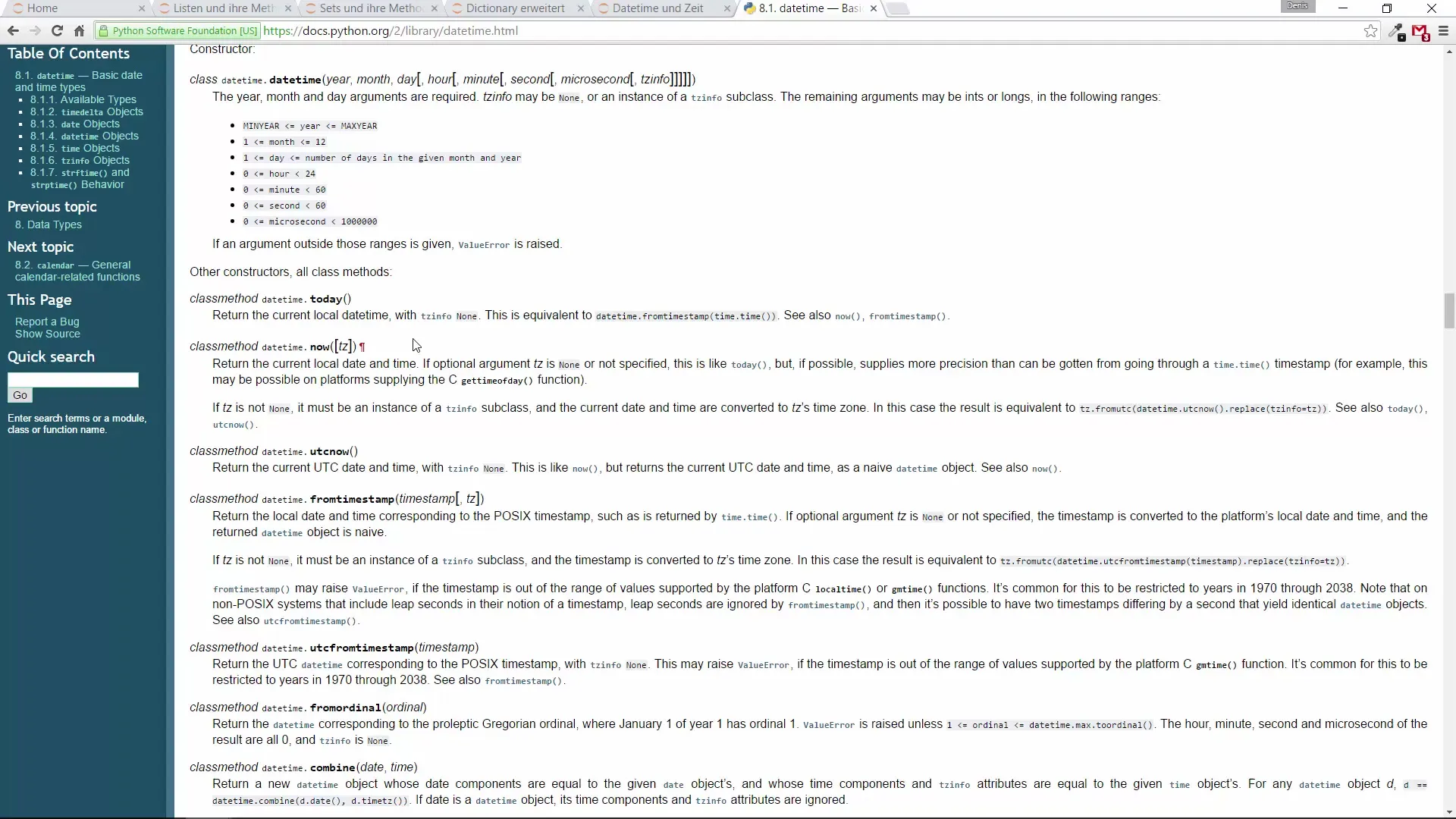
Summary - Python Programming for Beginners – 52 Datetime
In this guide, you have explored the fundamentals of the datetime module in Python. You have learned how to create times and dates, query the corresponding values, and calculate time differences. Additionally, it has been demonstrated how time zones can be considered, enabling you to make your applications globally relevant.
Frequently Asked Questions
What is the datetime module in Python?The datetime module provides classes for manipulating date and time.
How do I create a time instance?You can use datetime.time(hours, minutes, seconds, microseconds) to create a time instance.
How do I find the current date?Use the function datetime.date.today() to retrieve the current date.
How do I calculate the difference between two dates?Simply subtract one datetime object from another to get the difference.
How can I use time zones in Python?Use the pytz module to implement time zones and obtain localized time.