Inheritance is a central concept in object-oriented programming that allows you to learn from existing classes and create your own classes that provide additional functionalities. In this guide, you will learn how to effectively apply inheritance in Python and how to create classes that inherit from other classes.
Key Insights
- Classes can inherit from other classes, thereby adopting their properties and methods.
- Inheritance helps to structure and reuse code more efficiently.
- Subclasses can implement specific functions that inherit from the superclass as well as add their own specific functions.
Step-by-Step Guide
1. Creating a Base Class
Start by creating a very simple class. The class Car is used here as an example. This class has basic properties and methods that a car should have.
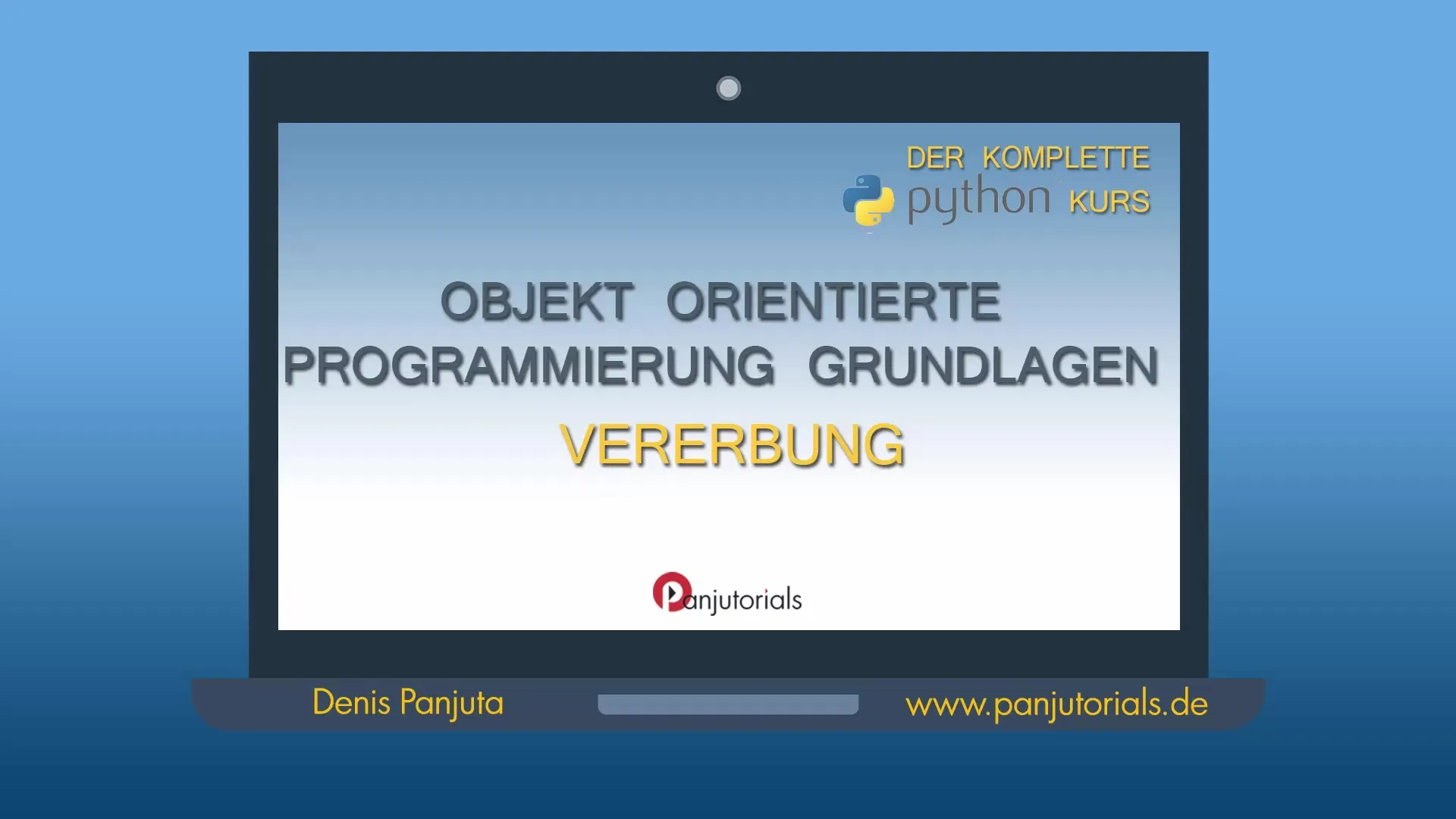
2. Instantiating the Base Class
After defining the class, you can create an instance of Car. This instance will inherit the methods and properties defined in the class.
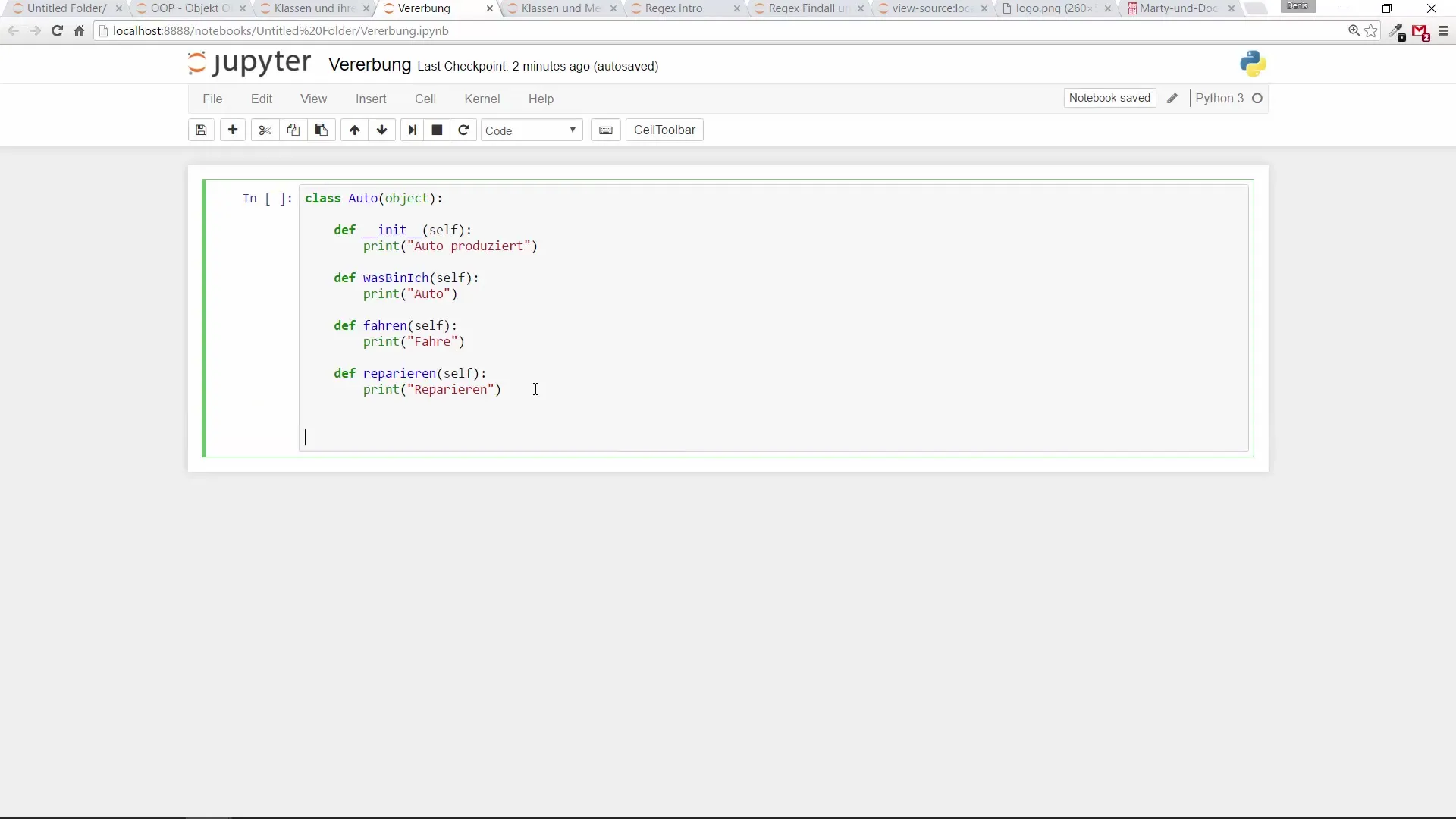
3. Creating a Subclass
Now create a subclass BMW that is supposed to inherit its functionalities from the Car class. The subclass will provide specific features and behaviors for a BMW car.
4. Instantiating the Subclass
Create an instance of the subclass BMW to see how the inherited methods work and what new functions the subclass additionally offers.
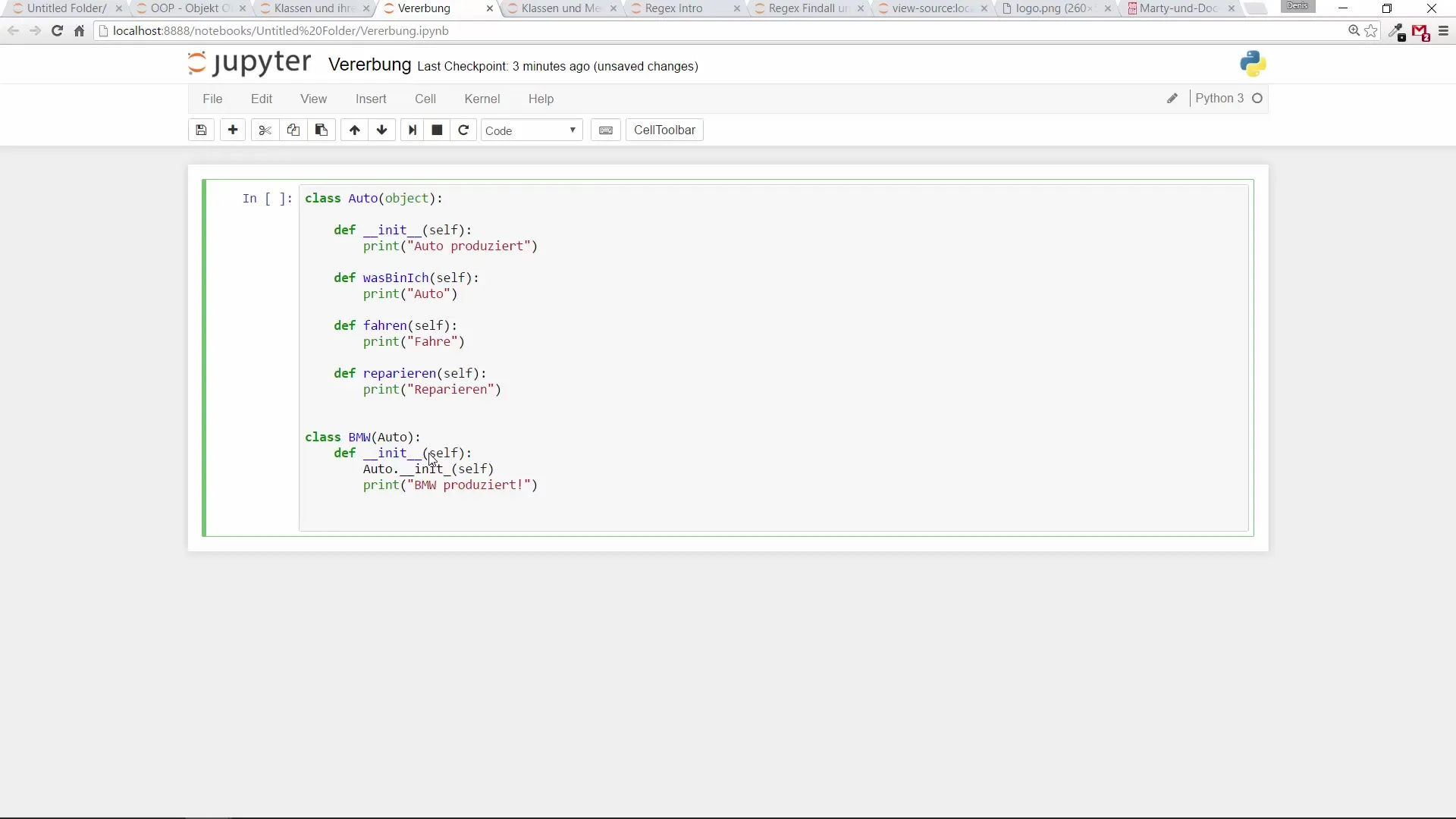
5. Overriding Methods
You can also override superclass methods in the subclass to provide specific implementations. Here, the what_am_I method is used in the BMW class.
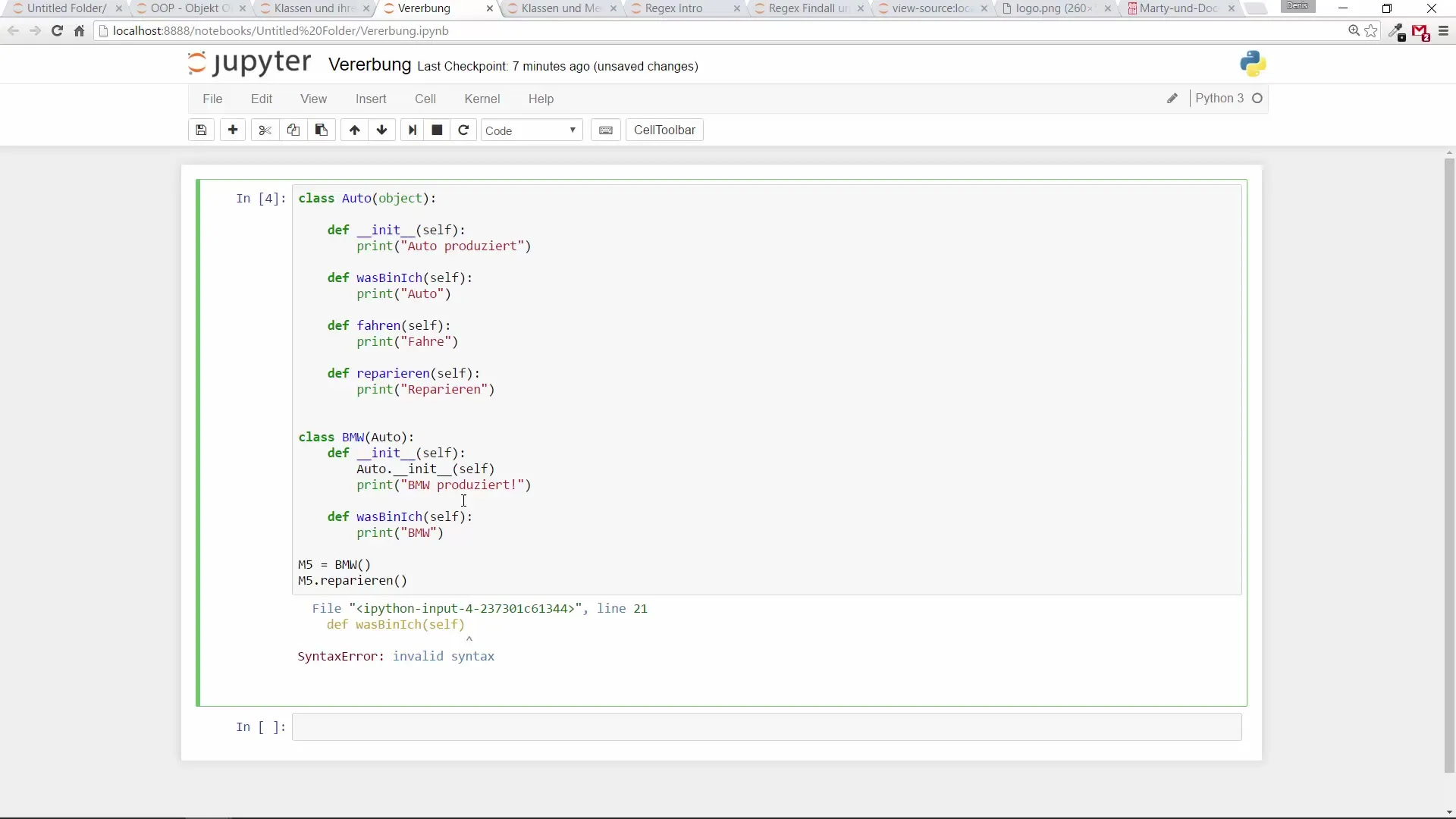
6. Creating Specific Functions
You can also add your own specific methods to the subclass that do not exist in the superclass. For instance, overtake is defined as a function for the BMW class.
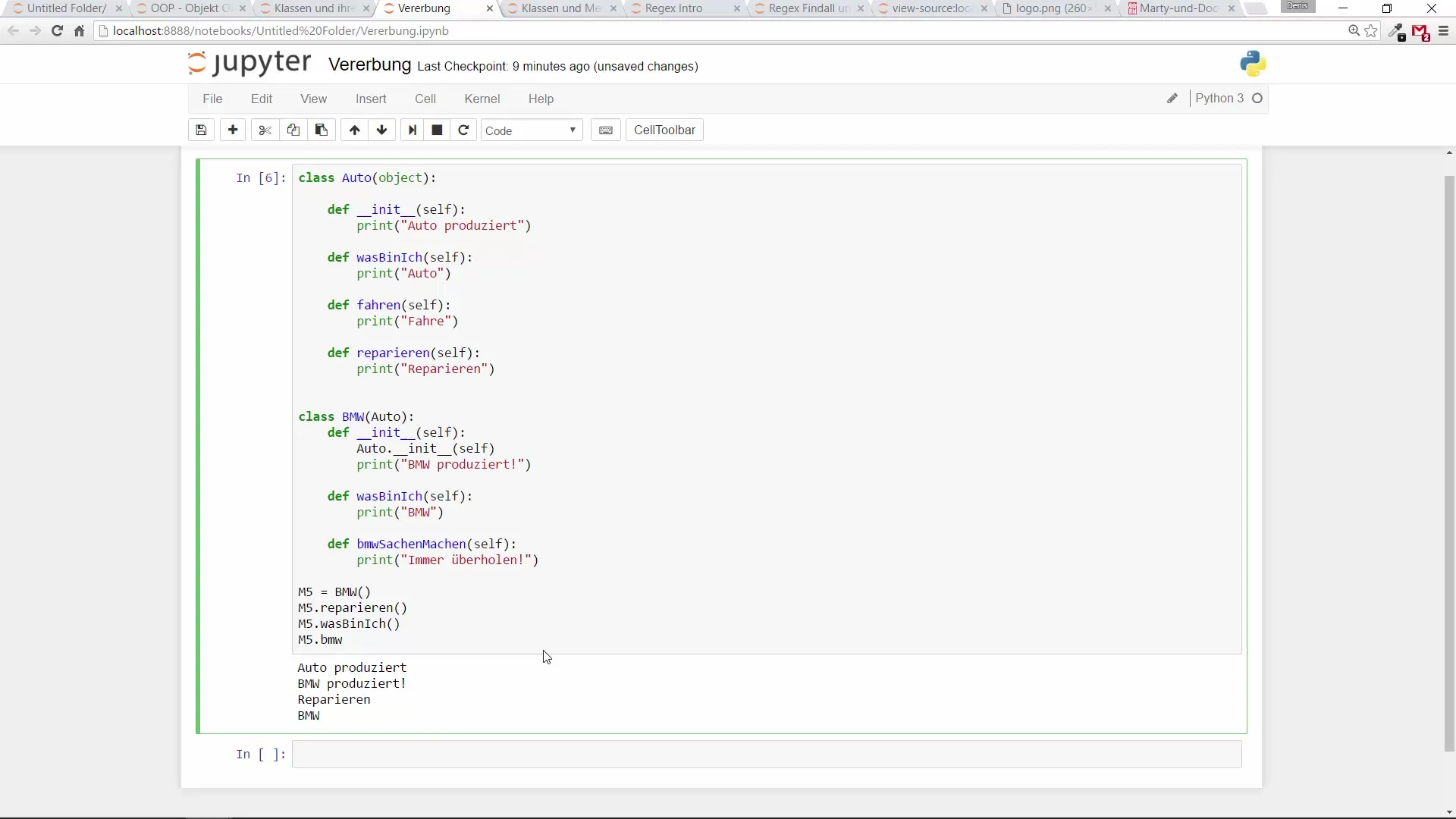
7. Error Handling on Mismatch
If you try to call a specific function of the subclass BMW on an instance of the superclass Car, an error will occur. This is important to understand in order to handle inheritance correctly.
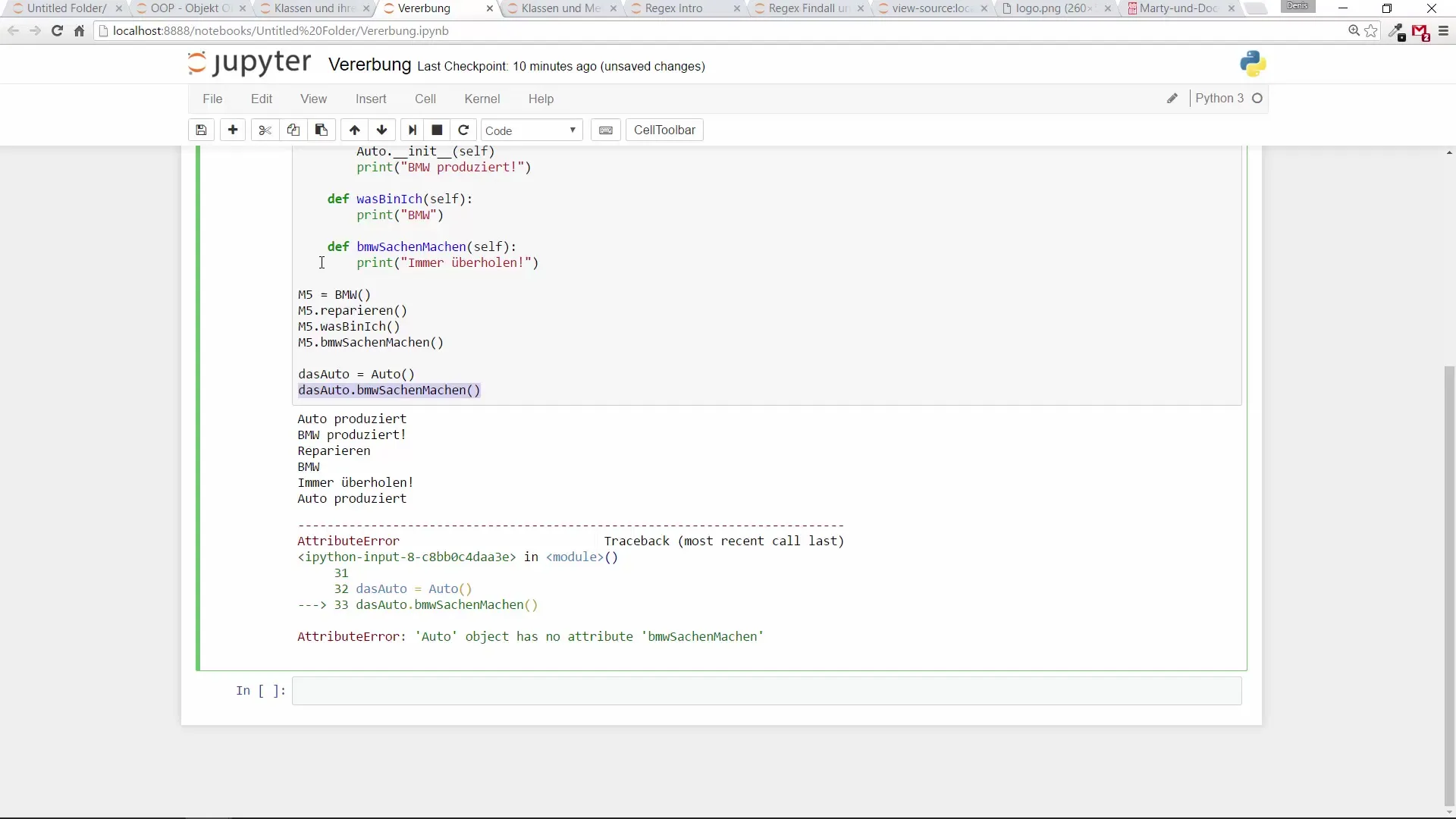
8. Creating Another Subclass
You can further refine your programming approach by creating another subclass of BMW, e.g., M3. This class inherits all functionalities from BMW and can implement its own methods.
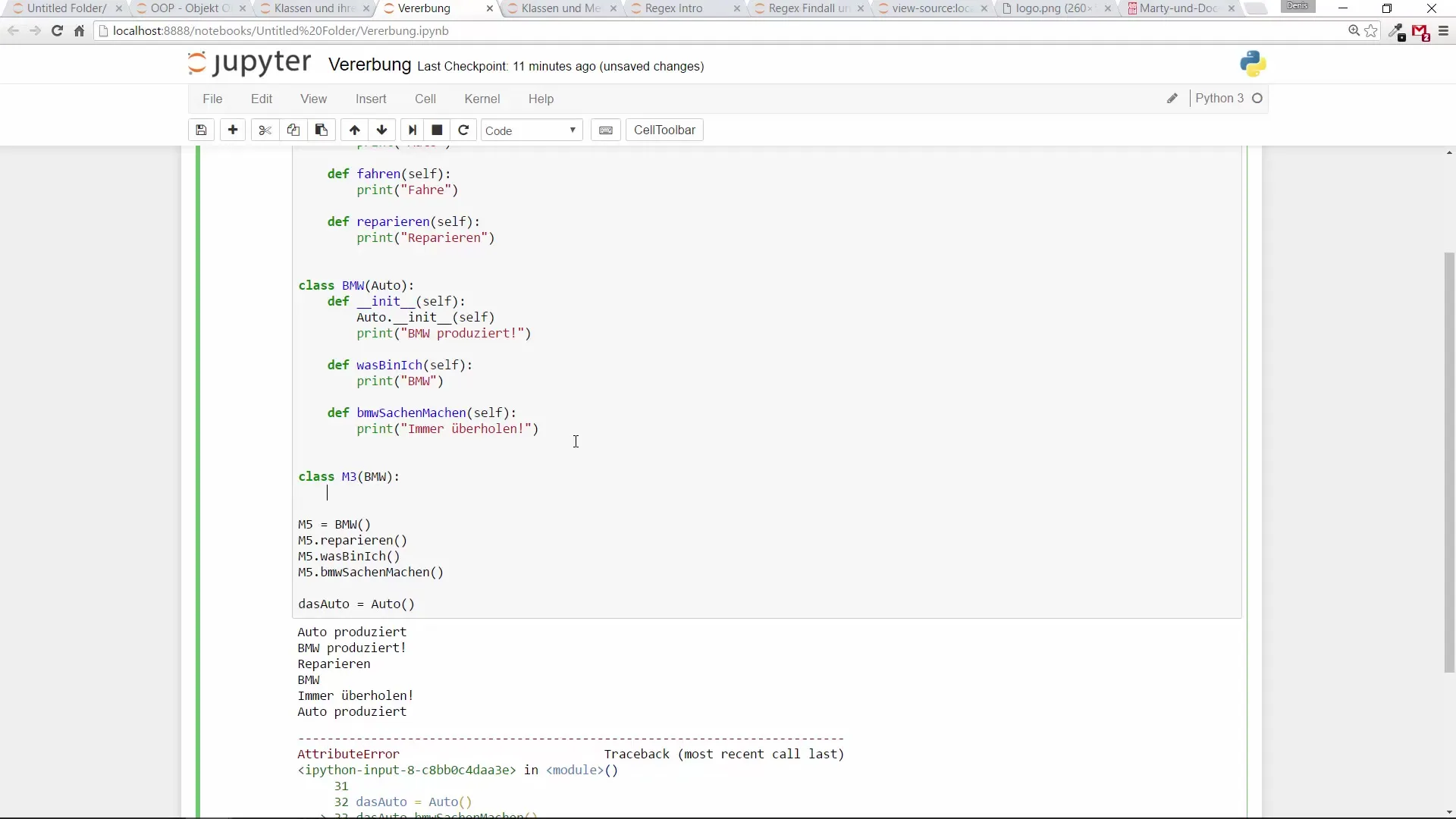
9. Merging Functions
Test the hierarchies and the integration of all the created classes. An instance of M3 will now have access to all functions of its ancestors.
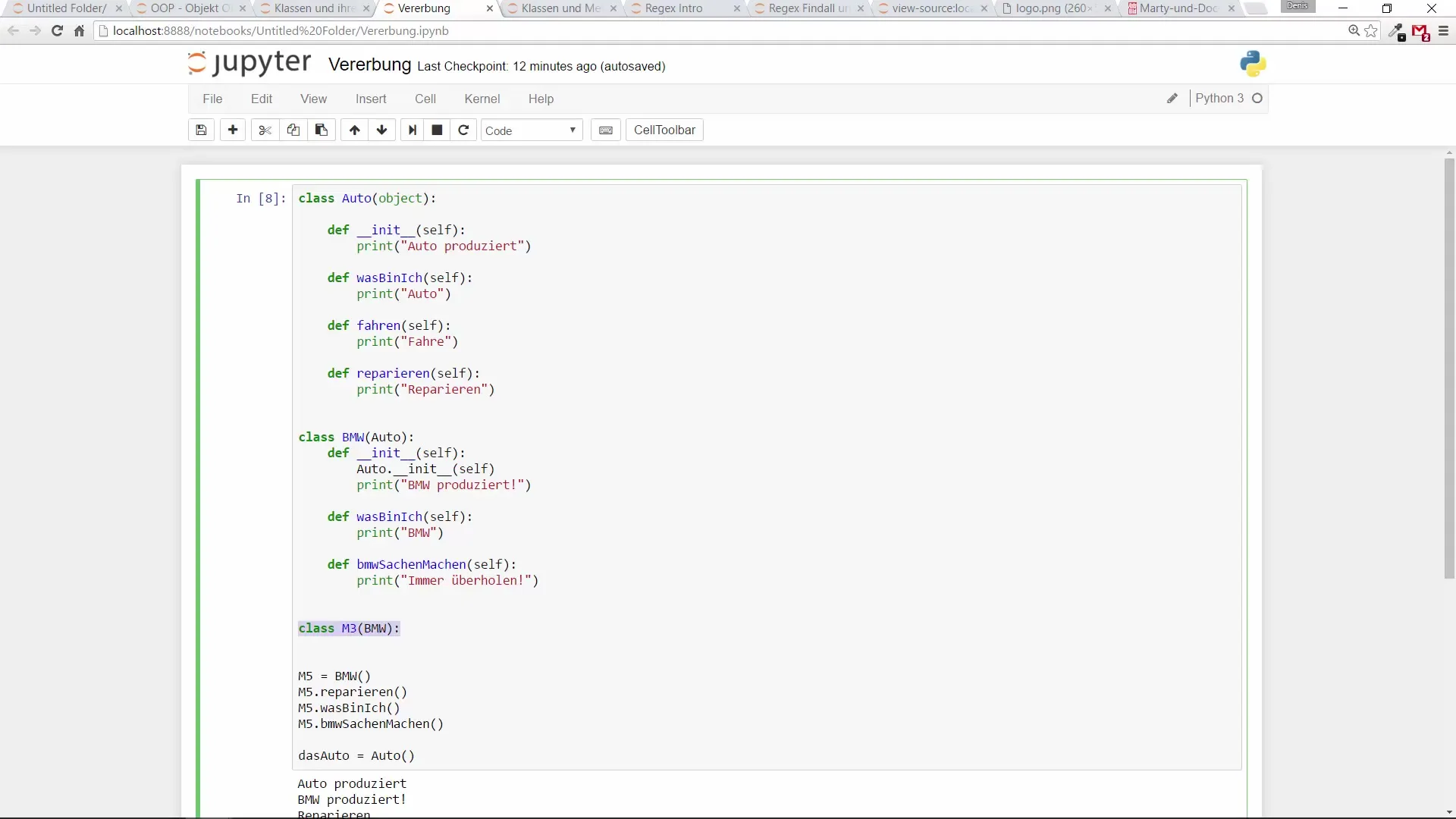
Summary – Understanding and Implementing Inheritance in Python
Inheritance in Python allows you to extend existing classes and implement specific functionalities in subclasses. Through simple implementations and inheriting methods and properties, you can create effective, structured code that is easy to maintain and extend.
Frequently Asked Questions
What is inheritance in Python?Inheritance is a concept where a class (subclass) inherits properties and methods from another class (superclass).
How do I create a subclass?A subclass is created by specifying the superclass in parentheses when defining the subclass.
Can I override superclass methods in the subclass?Yes, you can override superclass methods in the subclass with the same method signature.
What happens if I call specific functions of the subclass on the superclass?This will lead to an error since the superclass does not know the specific functions of the subclass.
How do I create multiple levels of subclasses?You can create another subclass from an existing subclass to create a deeper hierarchy.