The filter function in Python provides an elegant way to filter specific elements from a list. This can be particularly useful when working with large amounts of data, whether processing numbers, words, or even passwords. In this tutorial, you will learn how to effectively use the filter function to select data according to your specifications.
Key Insights
- With the filter function, you can filter elements from a list based on a condition.
- You can create your own functions to determine the filter criteria.
- Lambda functions provide a compact way to define filter conditions without writing separate functions.
Step-by-Step Guide
The following guide will systematically walk you through the process of applying the filter function in Python.
Introduction to the Filter Function
Let's start with a basic introduction to the filter function. The filter function allows you to iterate through a list and select specific elements based on a defined condition. Each element is passed to the specified function, and only the elements that pass the test are returned.
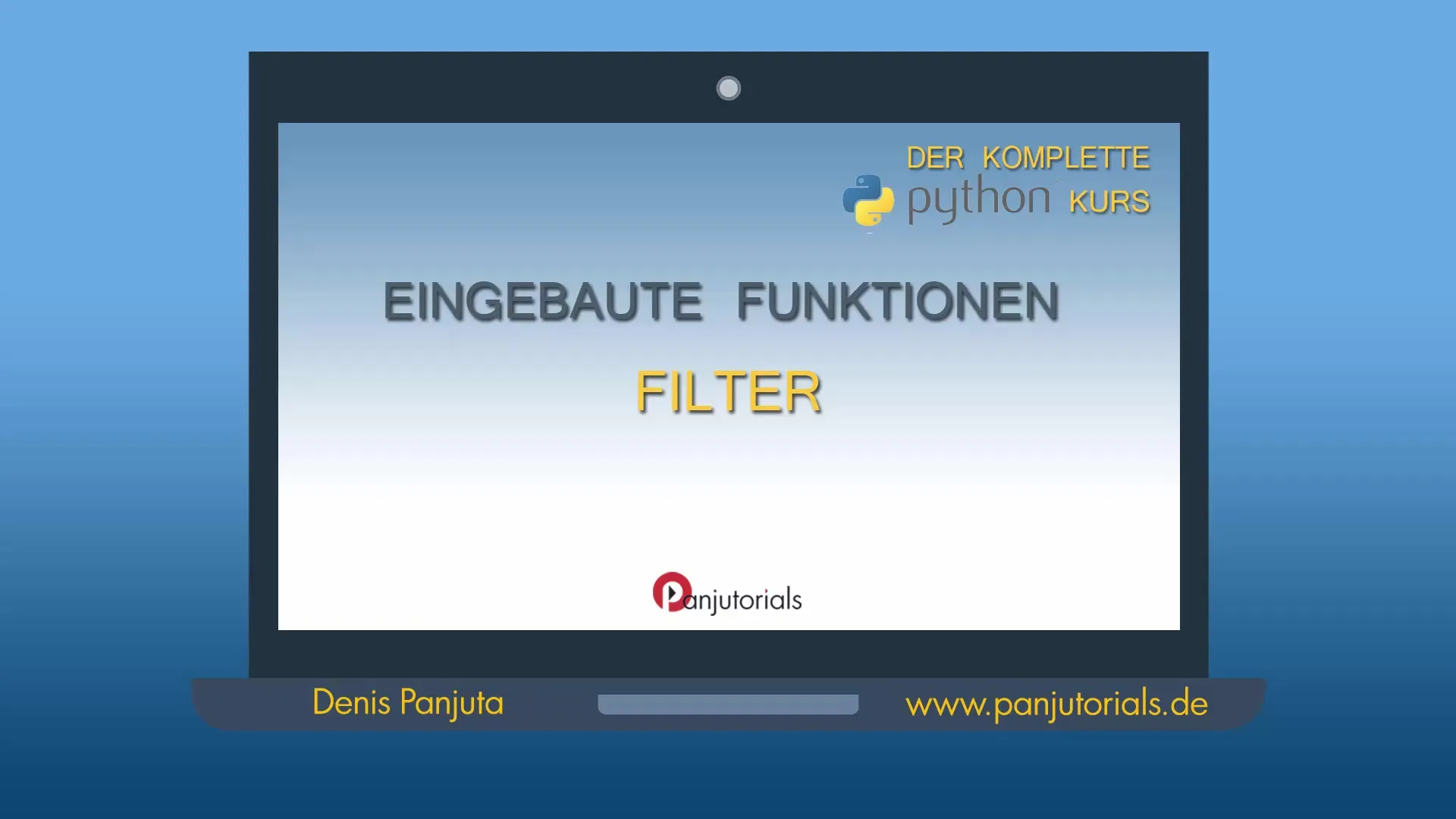
Creating the Test Function
First, we will create a function that checks if a number is odd. This is done using the modulo operator. If the remainder of the division of the number by 2 equals 1, it is an odd number, and the function returns True.
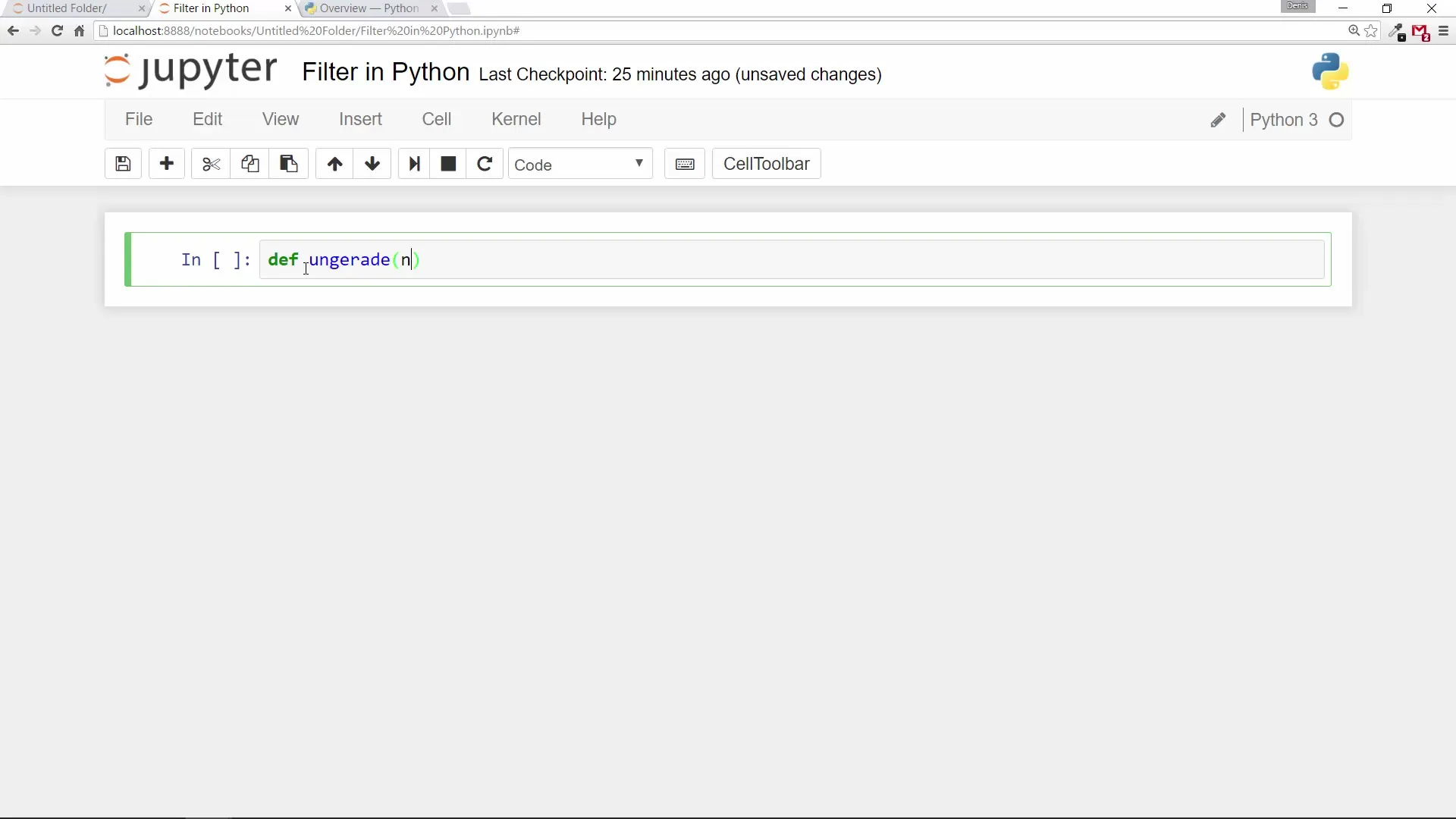
Applying the Filter Function
Now that you have the test function, it is time to create a list of numbers and apply the filter function. We will create a range from 0 to 19 and use the filter function to filter out the odd numbers.
The result of this operation will give you a list of the odd numbers from 1 to 19.
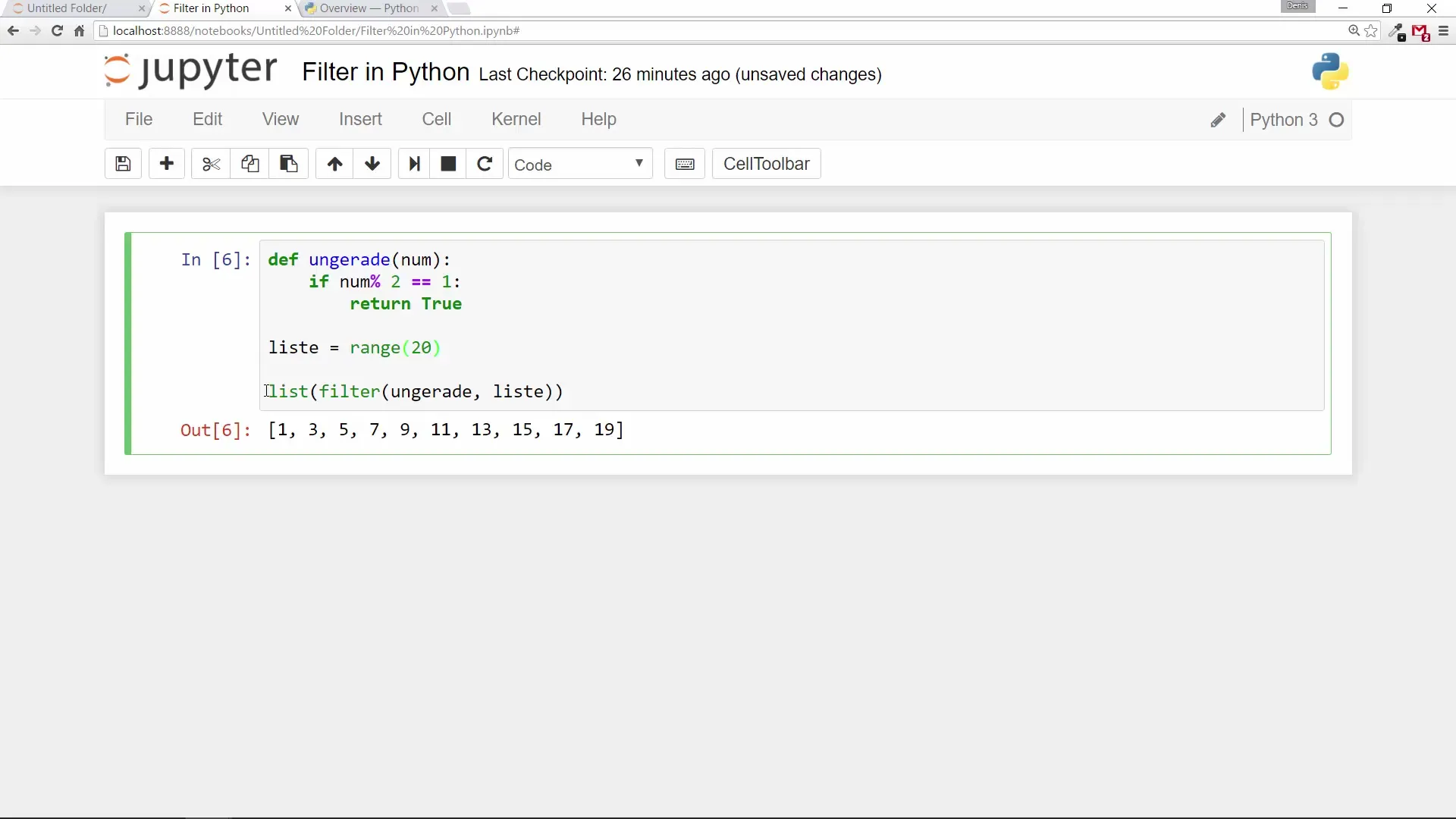
Example with Passwords
Imagine you have a list of passwords, and you want to find out which one is correct. You can create a function similar to the odd number check that checks if a password is correct.
Now create a list of passwords that you want to check.
Then you can apply the filter function.
If you output the list of correct passwords now, you will only get those that match your secure password.
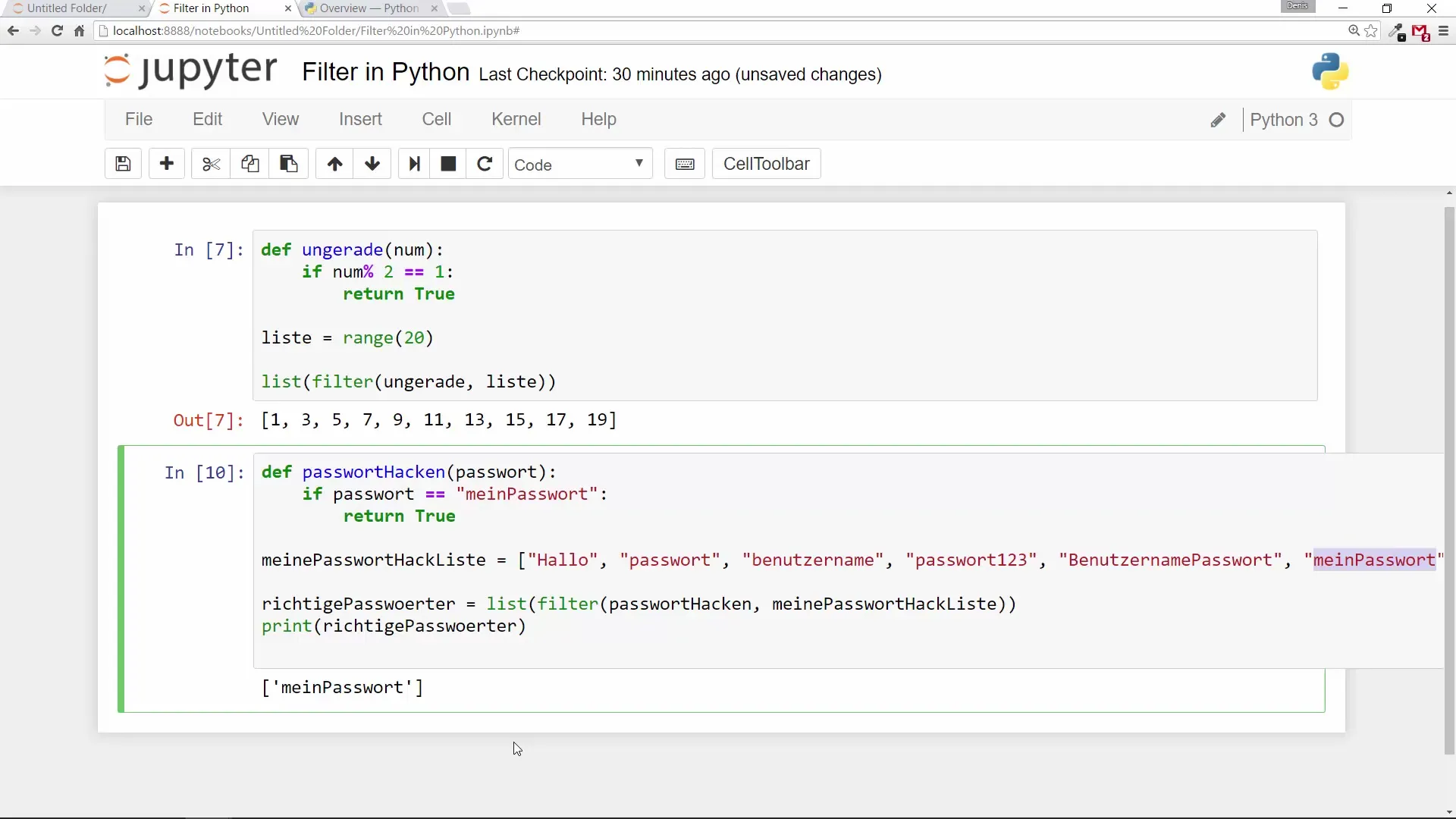
Using Lambda Functions
A sleek way to make the filter function even more compact is through the use of lambda functions. Lambda functions can be used to define simple test criteria directly within the filter function.
This example filters out odd numbers from a list and directly returns the results without you having to define a separate function.
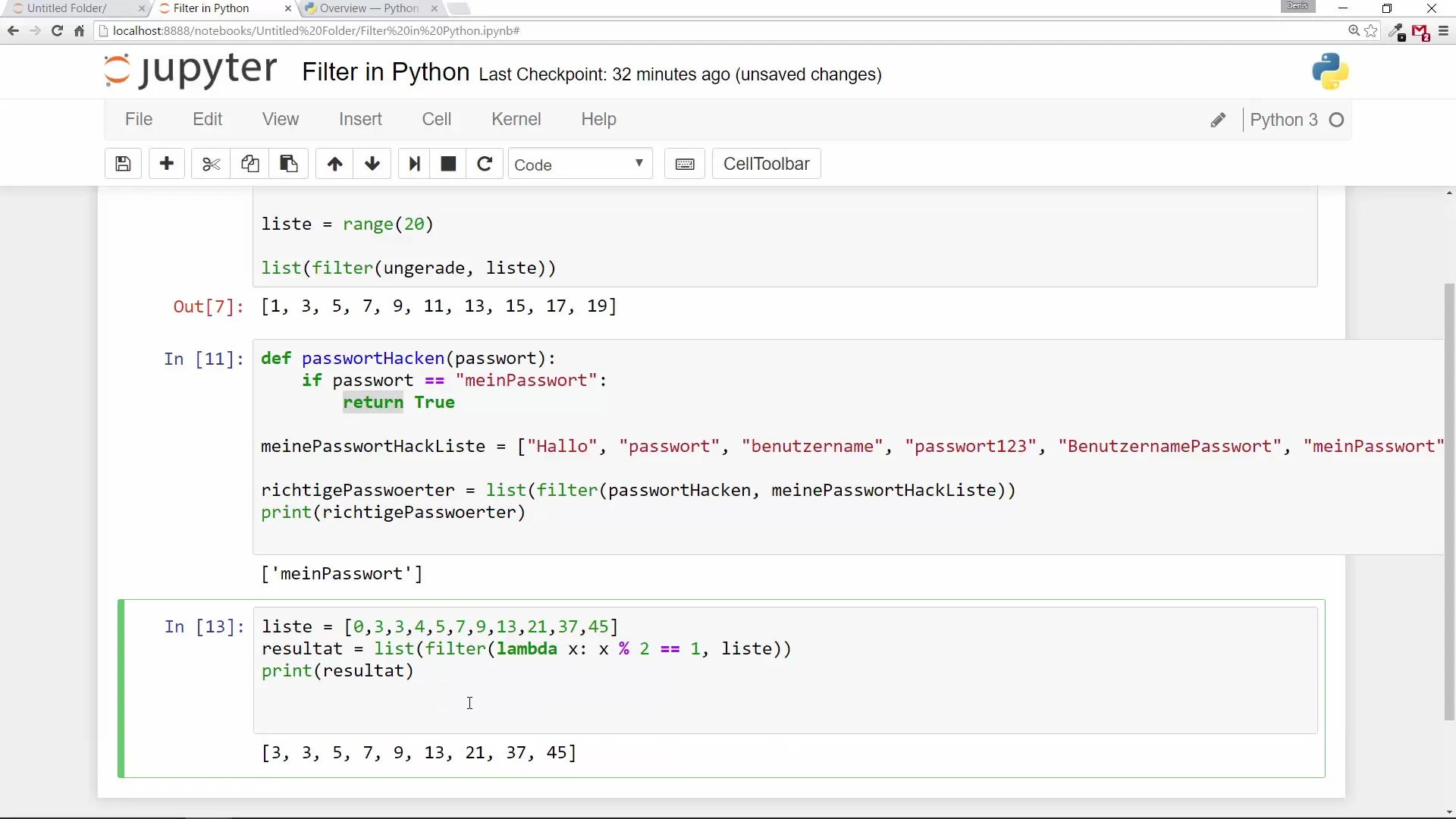
Diving into Advanced Filter Criteria
If you want to use filter functions for more complex data structures, you can also set any conditions. Consider a case where you search for even numbers or specific words in a list. The flexibility of filter and lambda in Python allows you to filter almost any conceivable data stream.
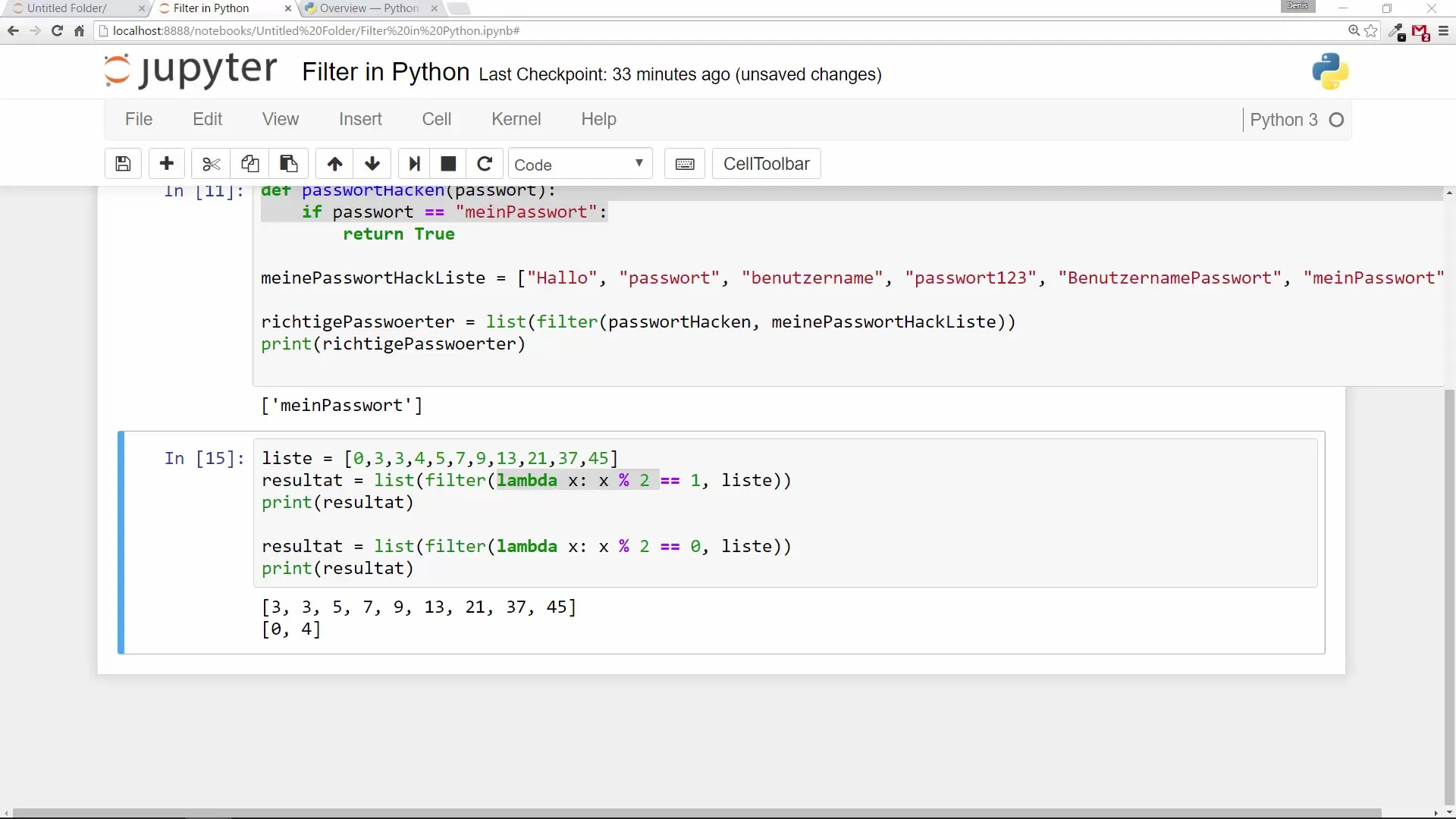
Summary – Create and Apply the Filter Function in Python
The filter function is a powerful tool in Python that allows you to selectively filter values from a list. Whether you are identifying odd numbers or checking passwords, the principles remain the same. With some practice, you will be able to successfully implement the filter function in your projects.
Frequently Asked Questions
How does the filter function work in Python?The filter function applies a test function to the elements of a list and returns only those elements that evaluate to True by the function.
Can I use my own functions for the filter?Yes, you can create any function that checks a condition.
Why should I use lambda functions?Lambda functions allow for a compact syntax when you want to use simple conditions directly in the filter function.
How can I apply multiple filter criteria?You can define more complex conditions in your test function or preferably in a lambda function to apply various filter criteria.
What is the advantage of the filter function?It offers an efficient and elegant way to work with lists and data streams without iterating through loops.