Functions are a central concept in Python programming. They allow you to create reusable code that significantly enhances the readability and maintainability of your program. Below, you will learn how to create, call, and effectively use functions in Python. You can expect practical examples and a step-by-step guide to help you develop a deeper understanding of working with functions.
Key insights
Functions are a practical means to structure and reuse code. Their basic structure consists of the keyword def, followed by a name and a parameter list. Once defined, a function can be called anytime and anywhere in the code. This saves time and effort when you need the same block of code multiple times.
Step-by-step guide
1. The structure of a function
First, take a look at the basic syntax of a function. Start with the keyword def, followed by a function name and a parameter list in parentheses. A colon concludes this, and immediately after it, you follow with the statements that the function should execute.
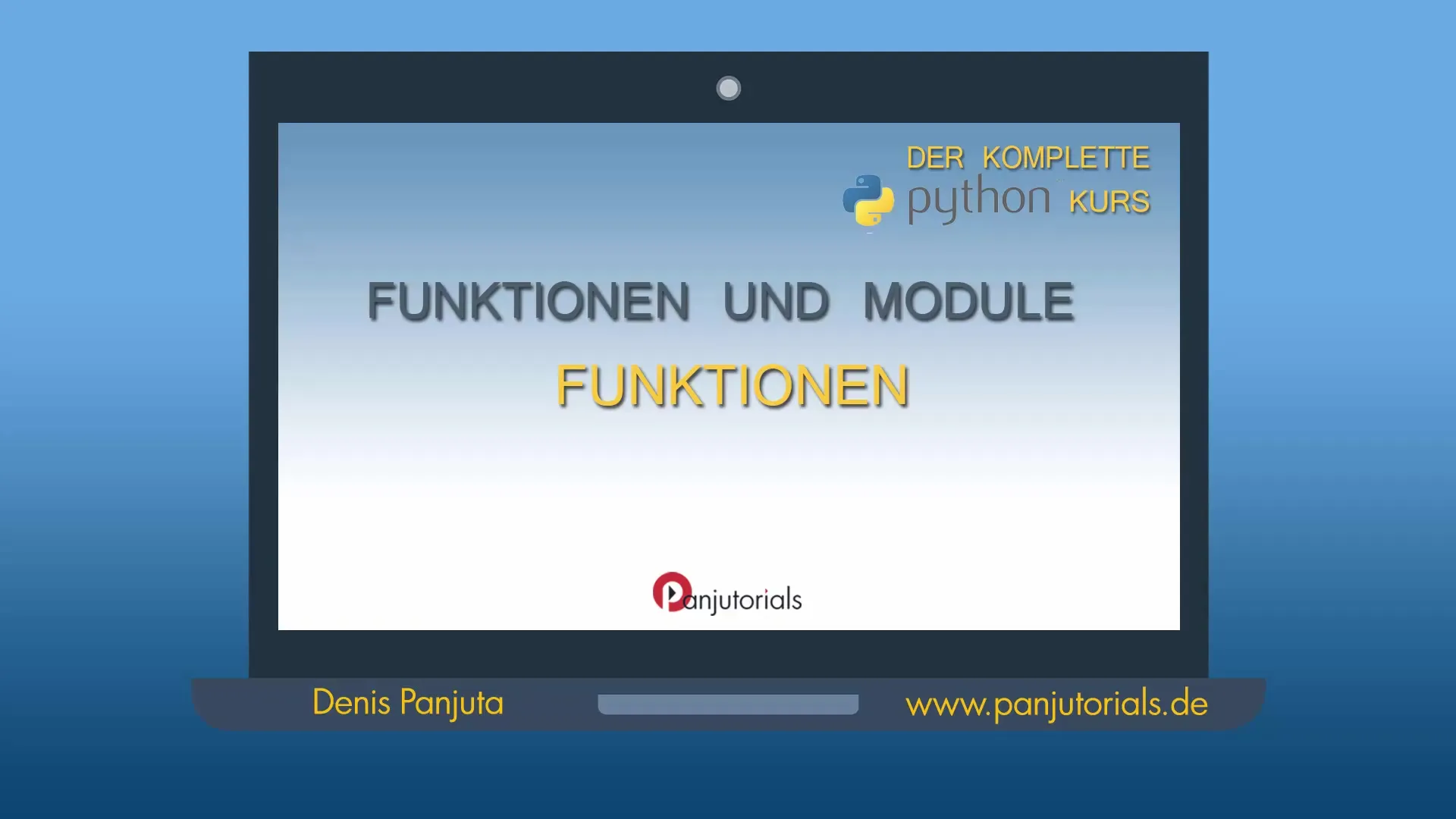
2. Creating a simple function
To demonstrate how a simple function works, you will now create a function that simply outputs "Hello". Name this function say_hello.
This function contains only one statement after the colon. It outputs the text "Hello" when you call it.
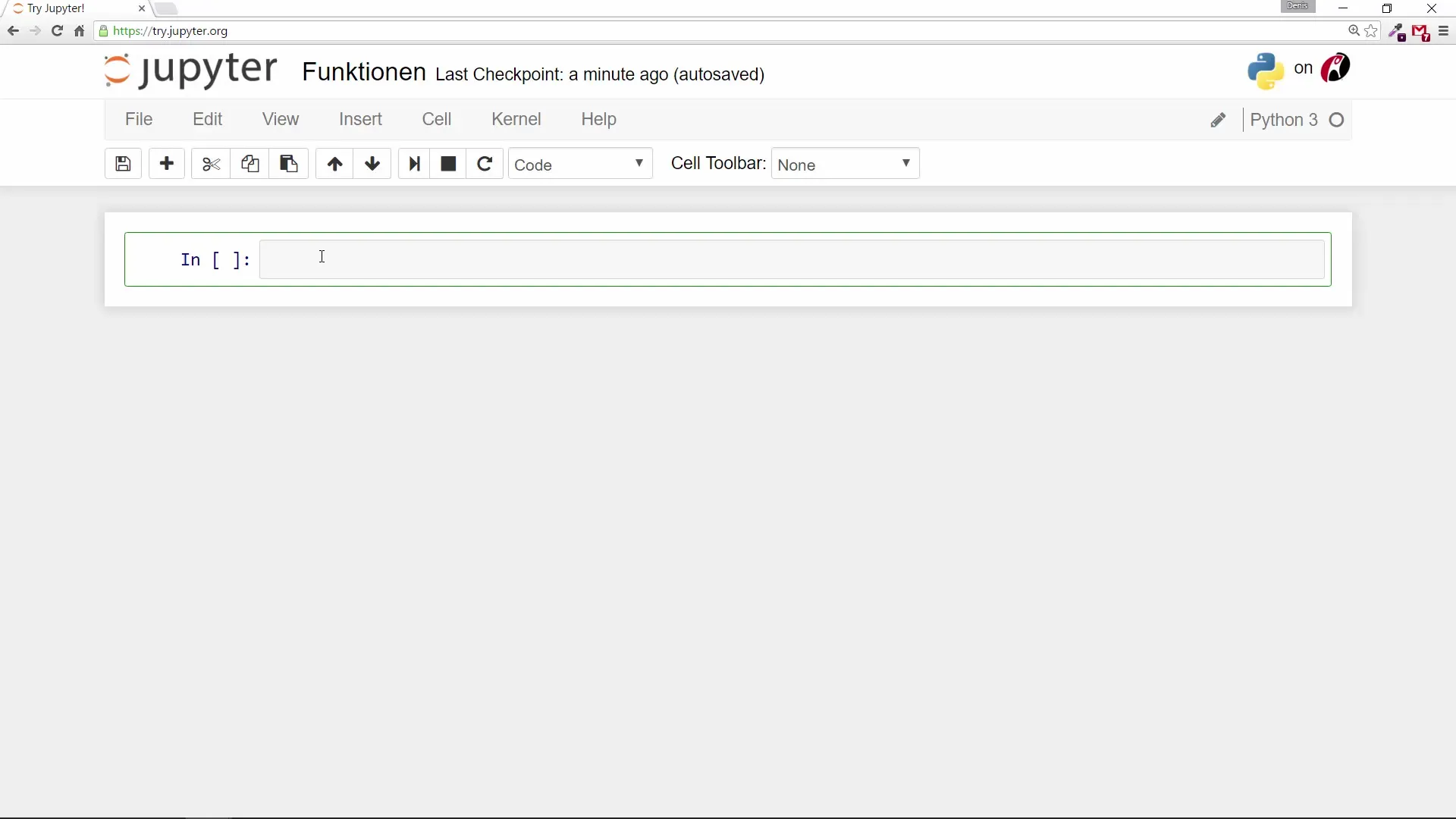
3. Calling a function
To execute this function, you simply call it in your program. This is done by using the function name followed by parentheses. For example:
The result should display "Hello" in your terminal. You can repeat this call multiple times to see that the function outputs the same text each time.
4. Defining a function with variables
Now let's expand the function. Suppose you want a function that outputs two values.
Here, two variables are defined and outputted when the function is called.
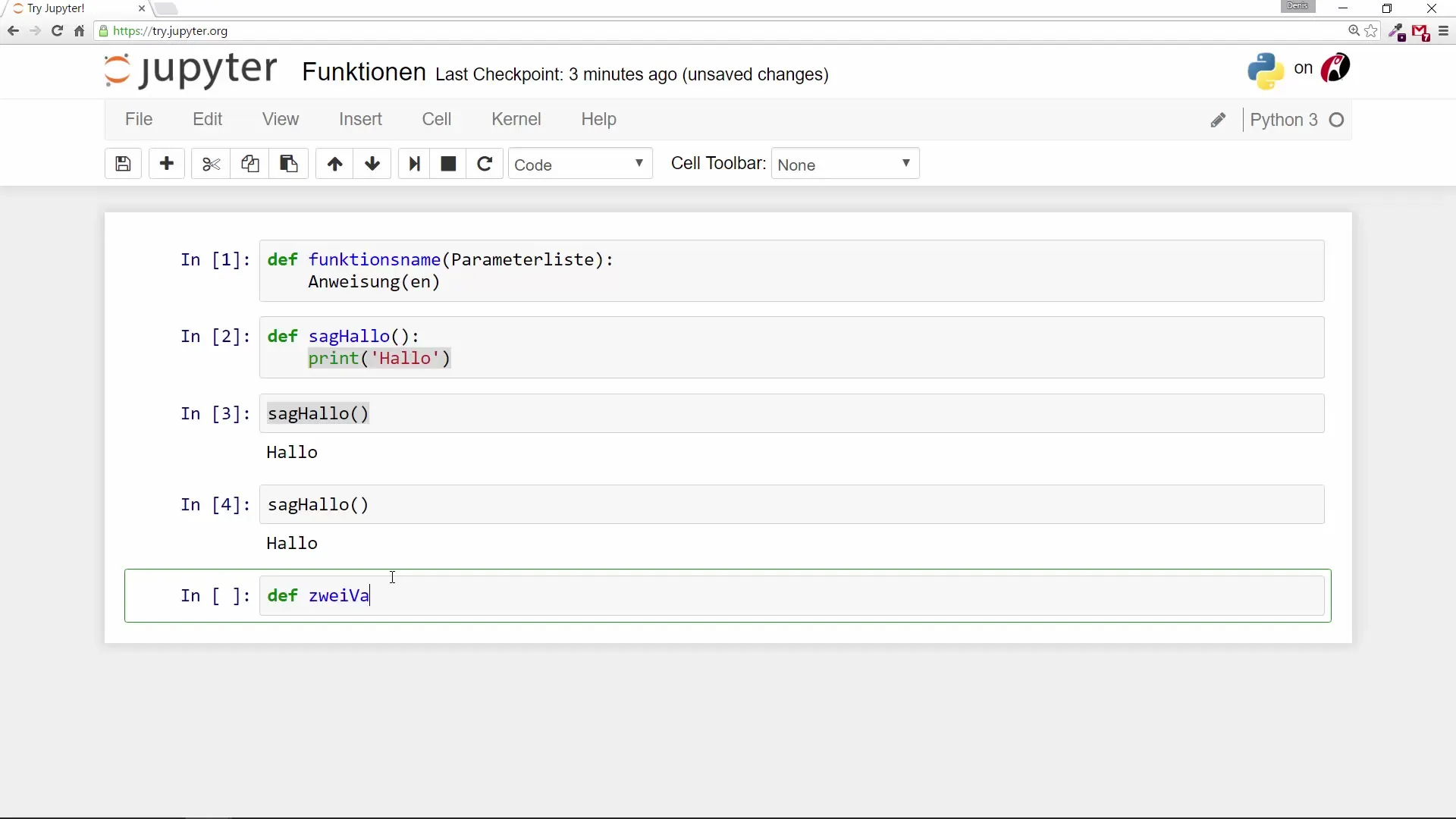
5. Calling the function
To execute the updated function, you again use the call show_numbers() in your code.
Once the function is defined, it does not matter where in the code the call is made – you can place it anywhere.
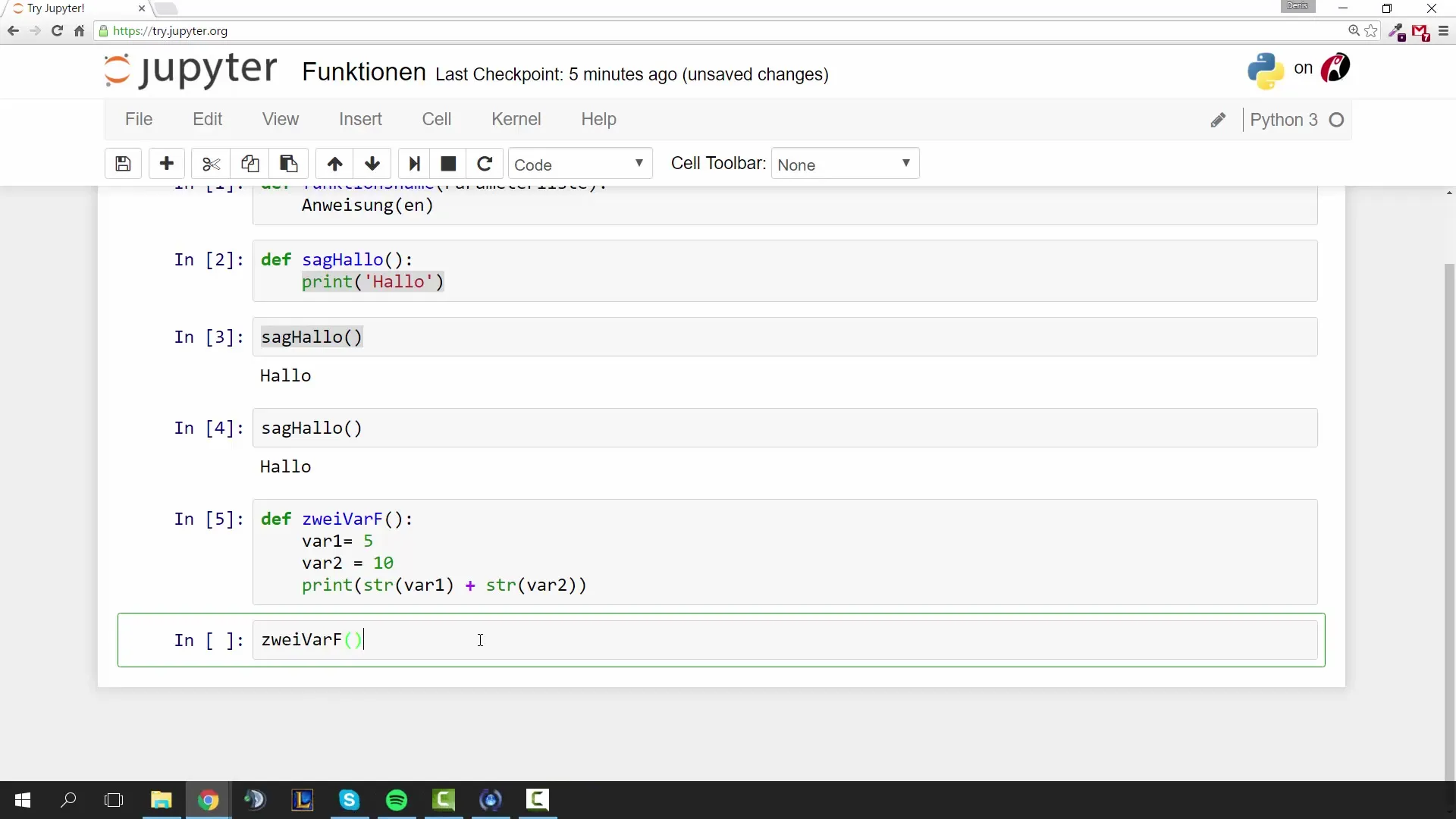
6. Functions with parameters
In the next step, it gets exciting: You will learn how to create functions with parameters.
Here, the function accepts two values that it adds together and outputs the result.
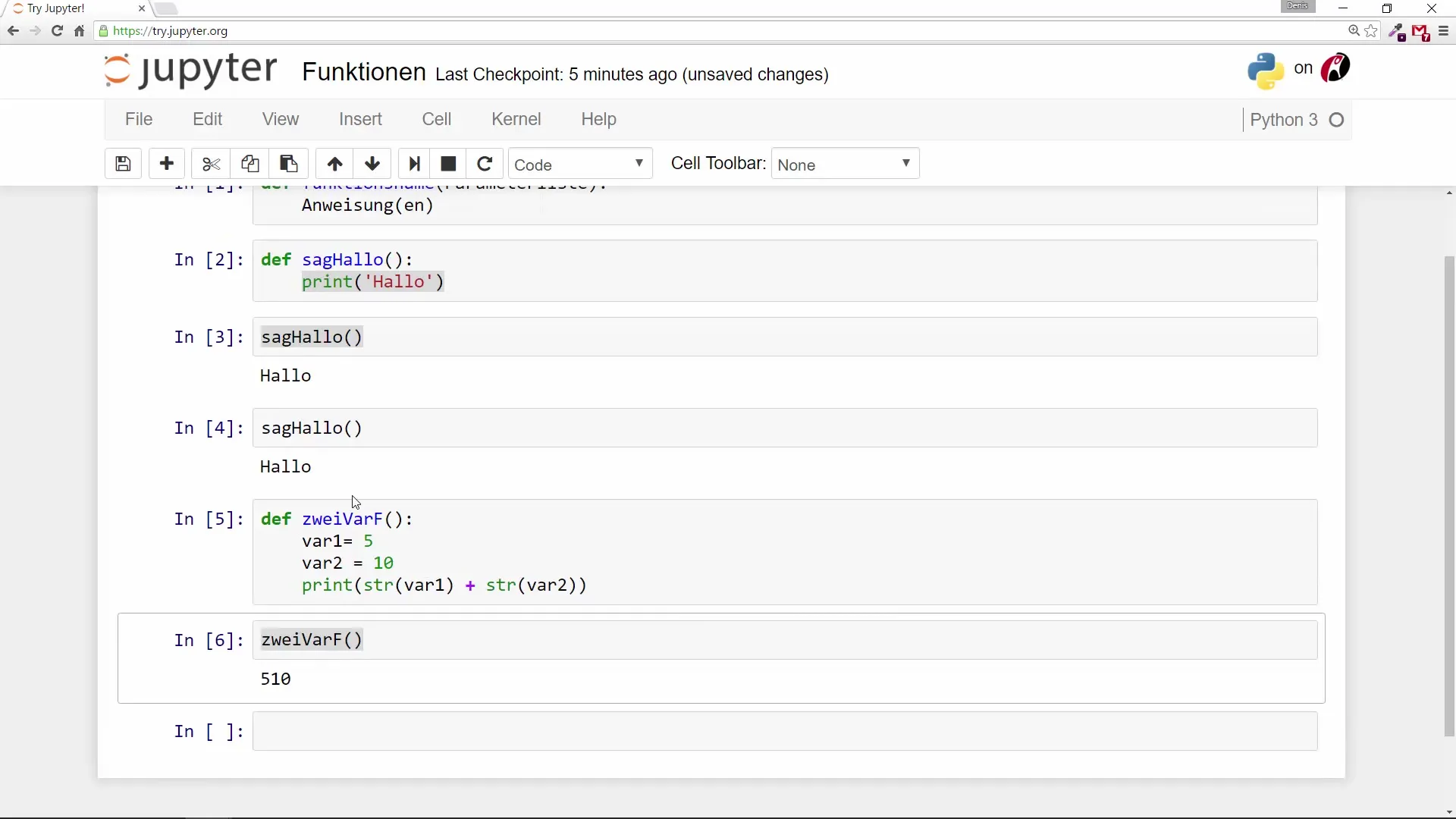
7. Calling a function with parameters
The result is the sum of the two values, which in this case is "15". You can pass different values to get different results.
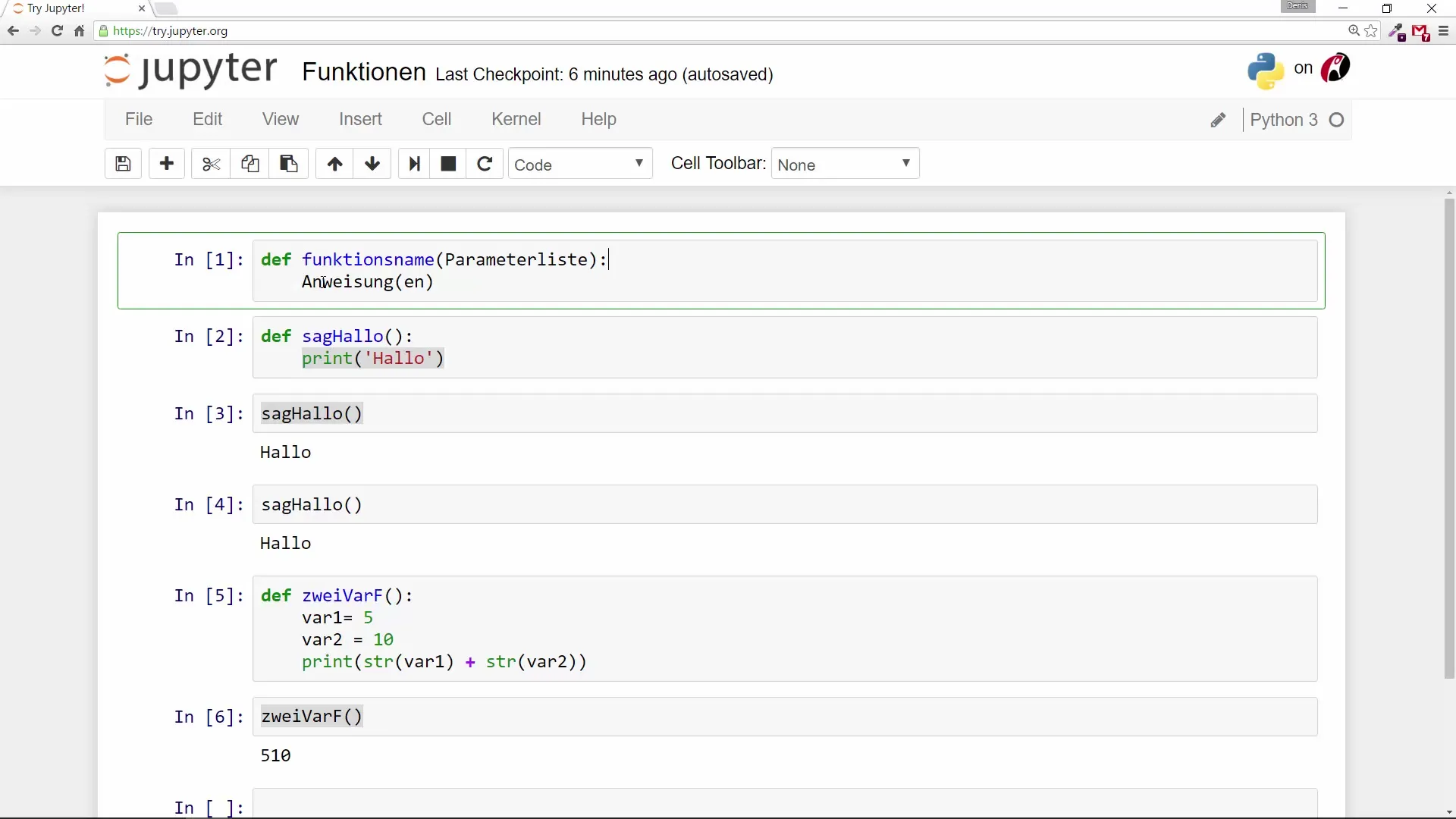
8. The next step with return values
So far, we have used only functions without return values. To gain more flexibility, you can also define a return value.
Here, the function returns the product of the two parameters.
9. Working with the return value
You process the return value, which gives you more options in programming.
10. Summary and outlook
Functions are a fundamental concept in Python that helps you organize and reuse your code. You have learned how to define and call functions, both simple outputs and those with parameters and return values. You are well-prepared to further explore functions, including more complex applications and working with parameters in the next video.
Summary – Python functions for beginners
In this tutorial, you have learned the basics of function definition in Python. You have created simple functions, called them, used variables, and experimented with return values. Functions are a valuable tool that will help you write more efficient and maintainable programs.
Frequently Asked Questions
How do I define a function in Python?A function is defined with the keyword def, followed by a function name and parentheses.
Can I call functions multiple times in the code?Yes, a once-defined function can be called as many times as needed.
How do I use parameters in functions?Parameters are specified in the function definition in parentheses and can be passed when calling the function.
What is the difference between print and return?print outputs values directly, while return sends a value back to the caller.
Can I use uppercase letters in function names?It is recommended to start function names with lowercase letters and avoid using special characters.