Regular expressions are a powerful tool for text analysis and manipulation. They allow you to find, test, replace, or even split specific patterns in a text. This guide will teach you the basics of using regular expressions in Python with the re.match and re.search modules.
Key Insights
- Regular expressions help to search for specific strings in texts.
- re.match checks if a pattern exists at the start of the text.
- re.search finds the pattern anywhere in the text.
- The re library in Python is necessary for working with regular expressions.
Step-by-Step Guide
To understand how regular expressions work, here are the steps for implementation in Python.
First, create a new file in Python 3. There you will define some terms that you want to search for later in a text. For example, we will use the terms "term 1" and "term 2":
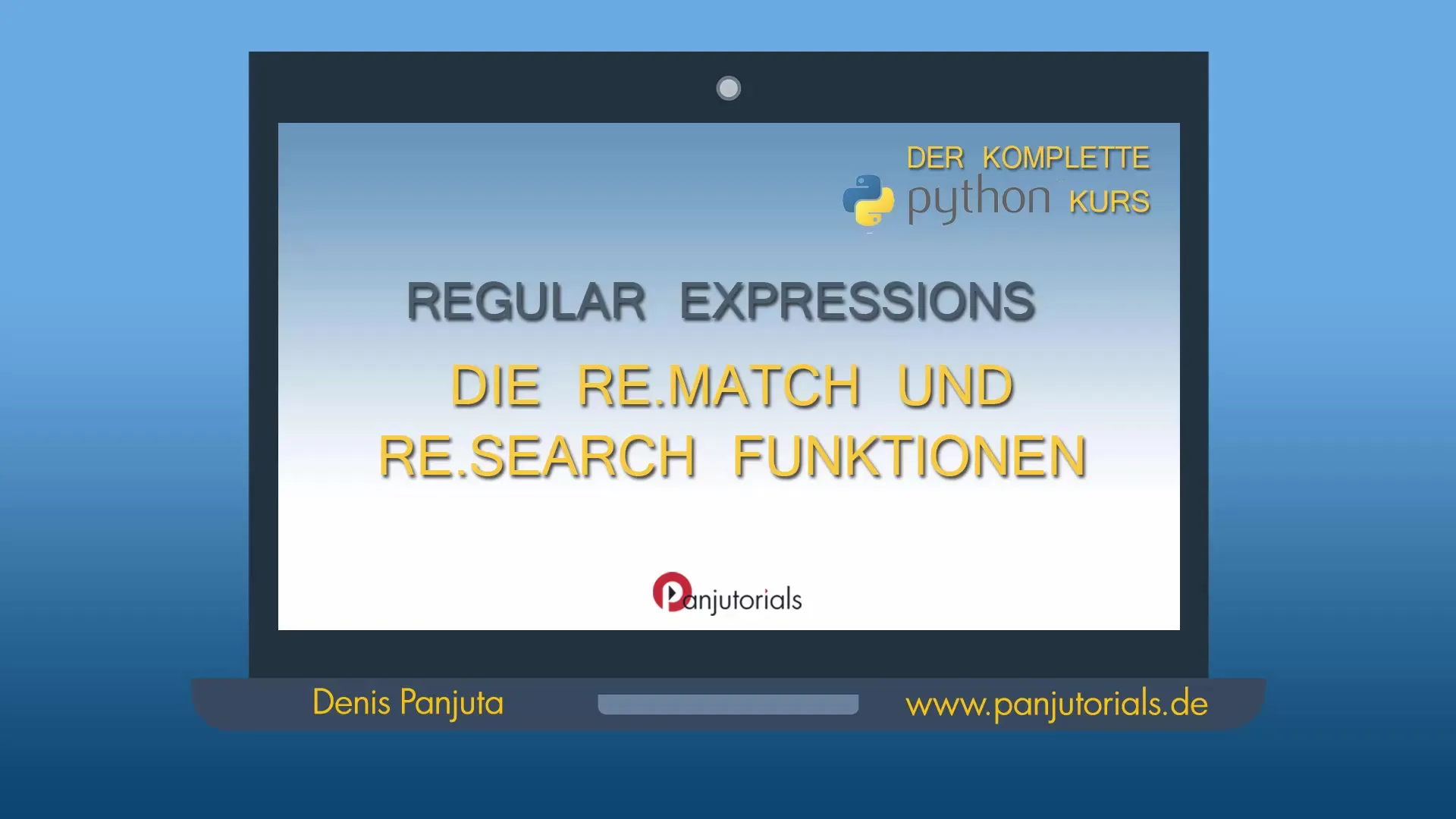
Next, you will write a text. This text will contain the terms you want to search for. For example: "This is a string with term 1 or back 1, but it has no other way."
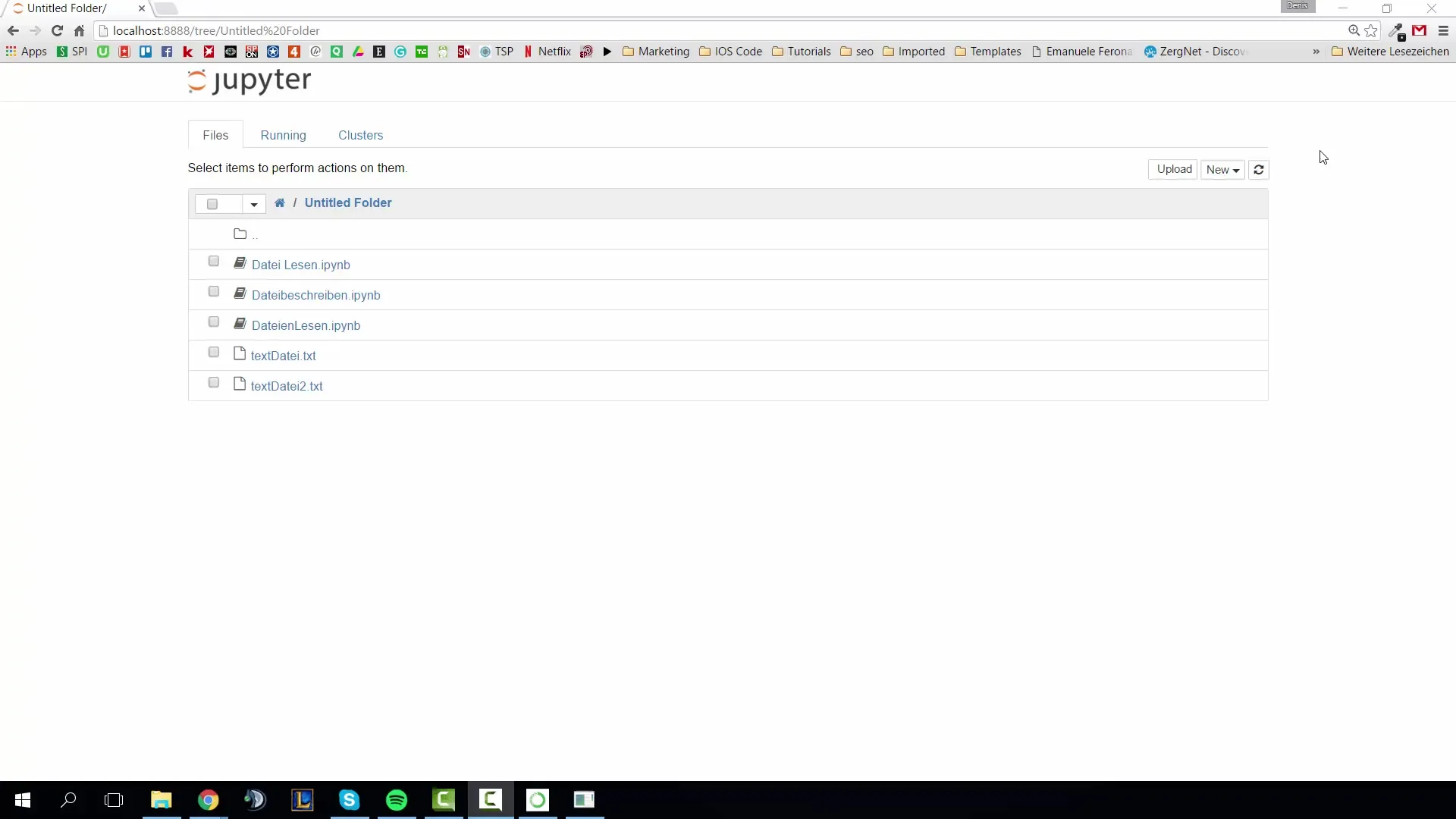
Now you create a loop that iterates through your defined terms. In this loop, you use the print function to display the search results. The print function will output the current search term in the console.
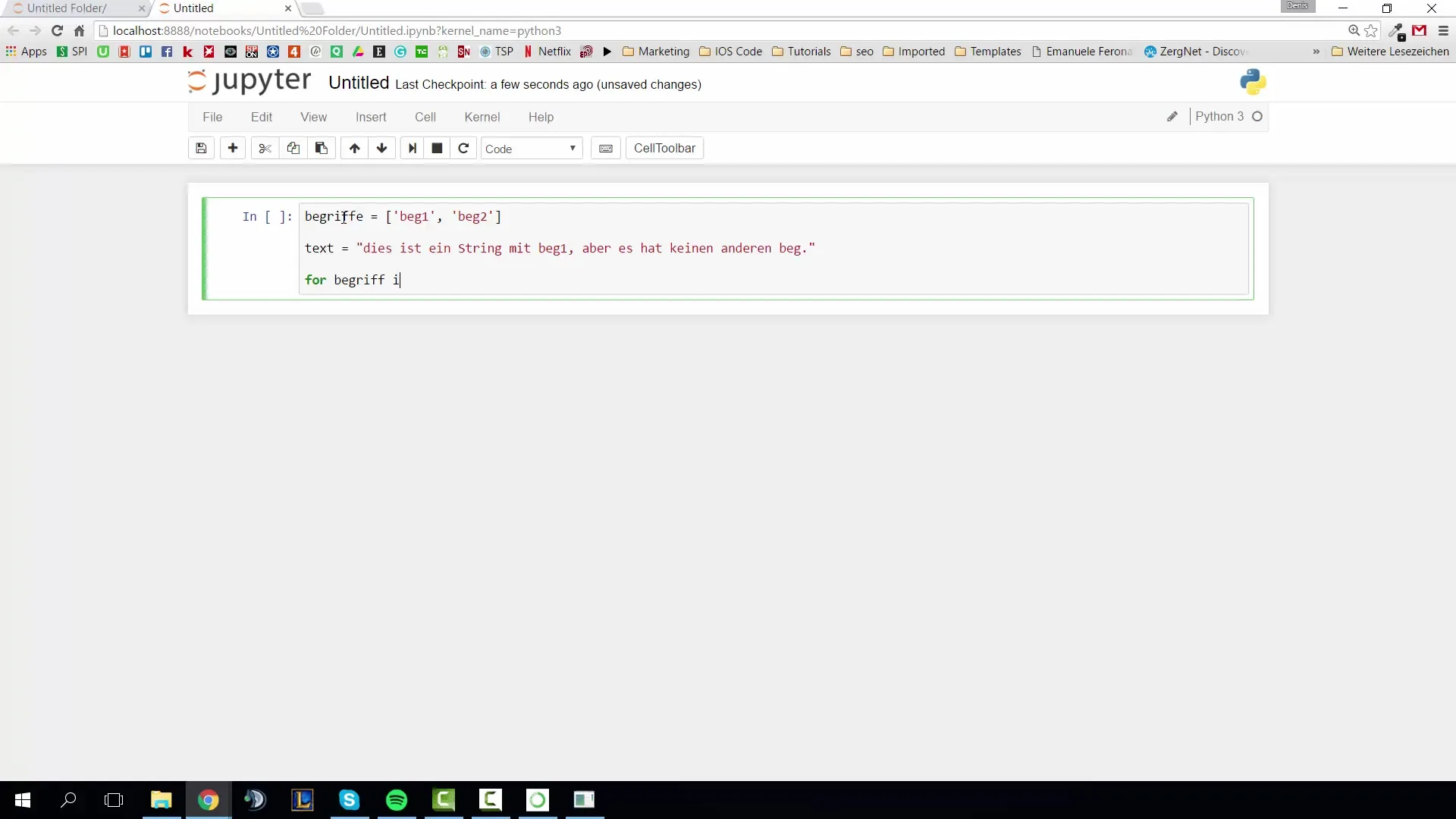
To make the search results more readable, you can format the string. Here, you use placeholders to dynamically display the current term as well as the text.
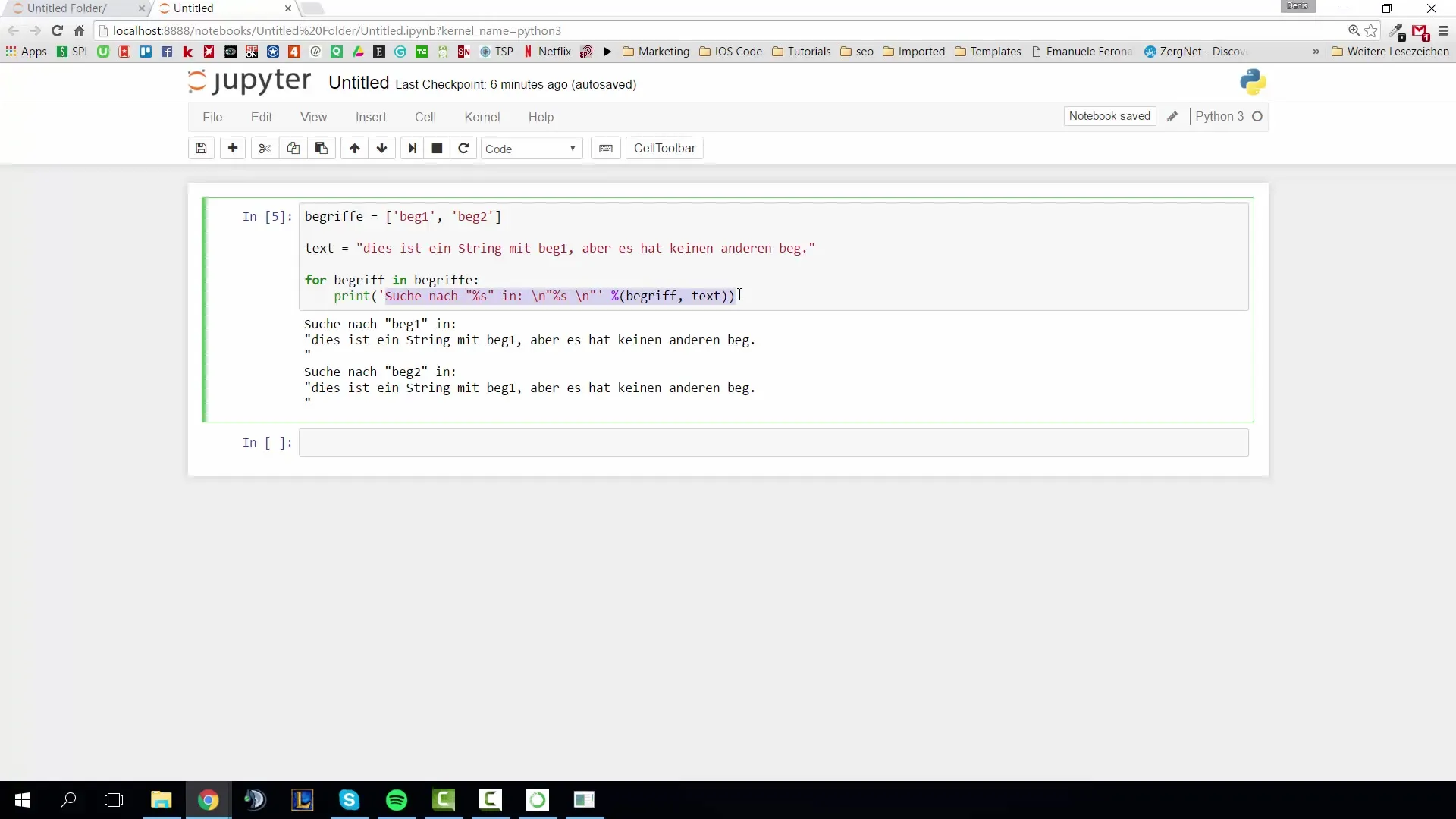
Next, you implement the logic to check if each term is present in the text. For this, we use re.search, which is a useful method to search for a pattern within a text.
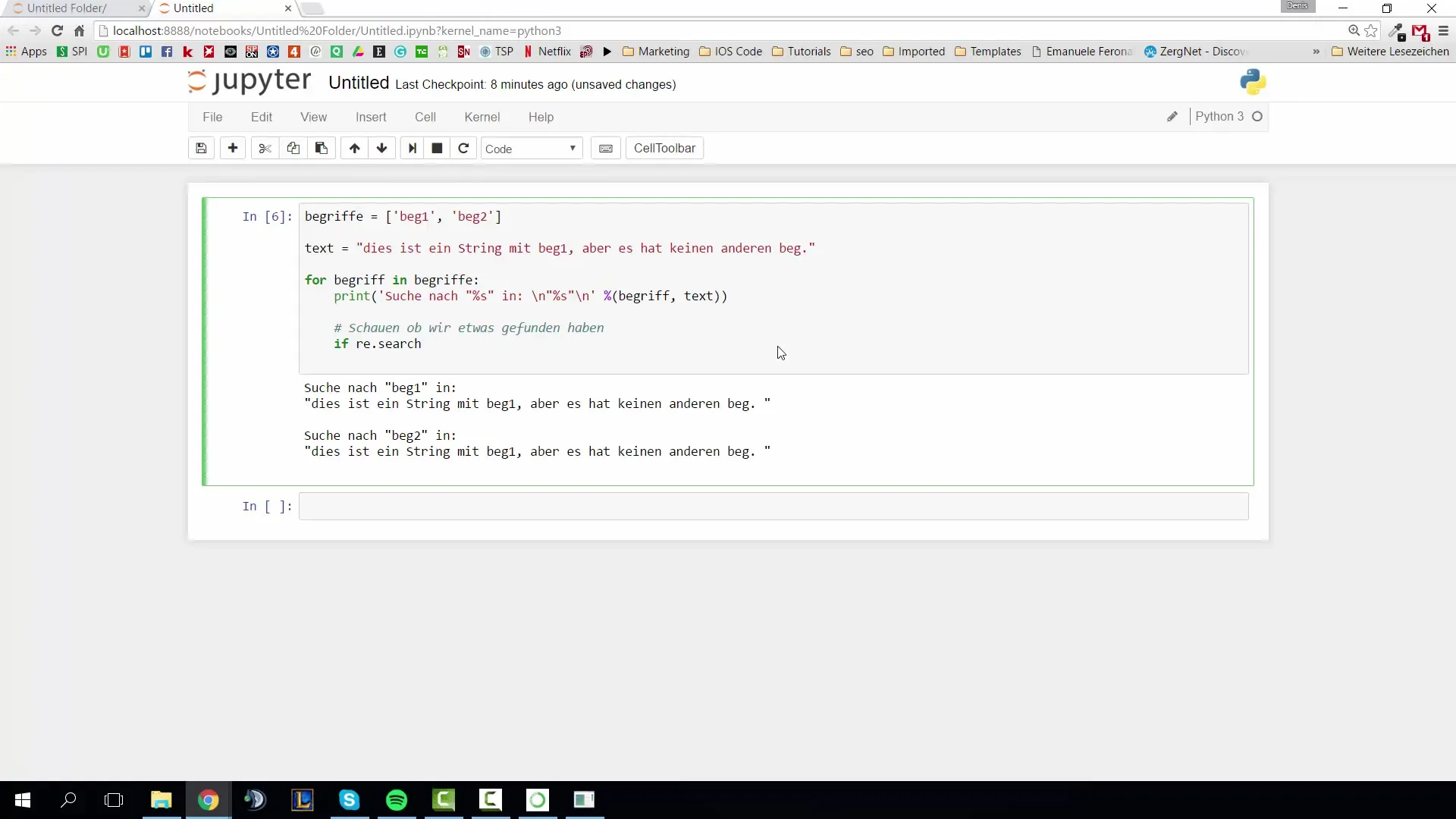
If re.search finds a match, you output that the term is present, and if not, the output informs that it is not present. The output is designed to be easily understandable, clarifying what the result is.
The result will show you which terms are found in the text and which are not. If you add additional terms, the loop will run for each of those terms.
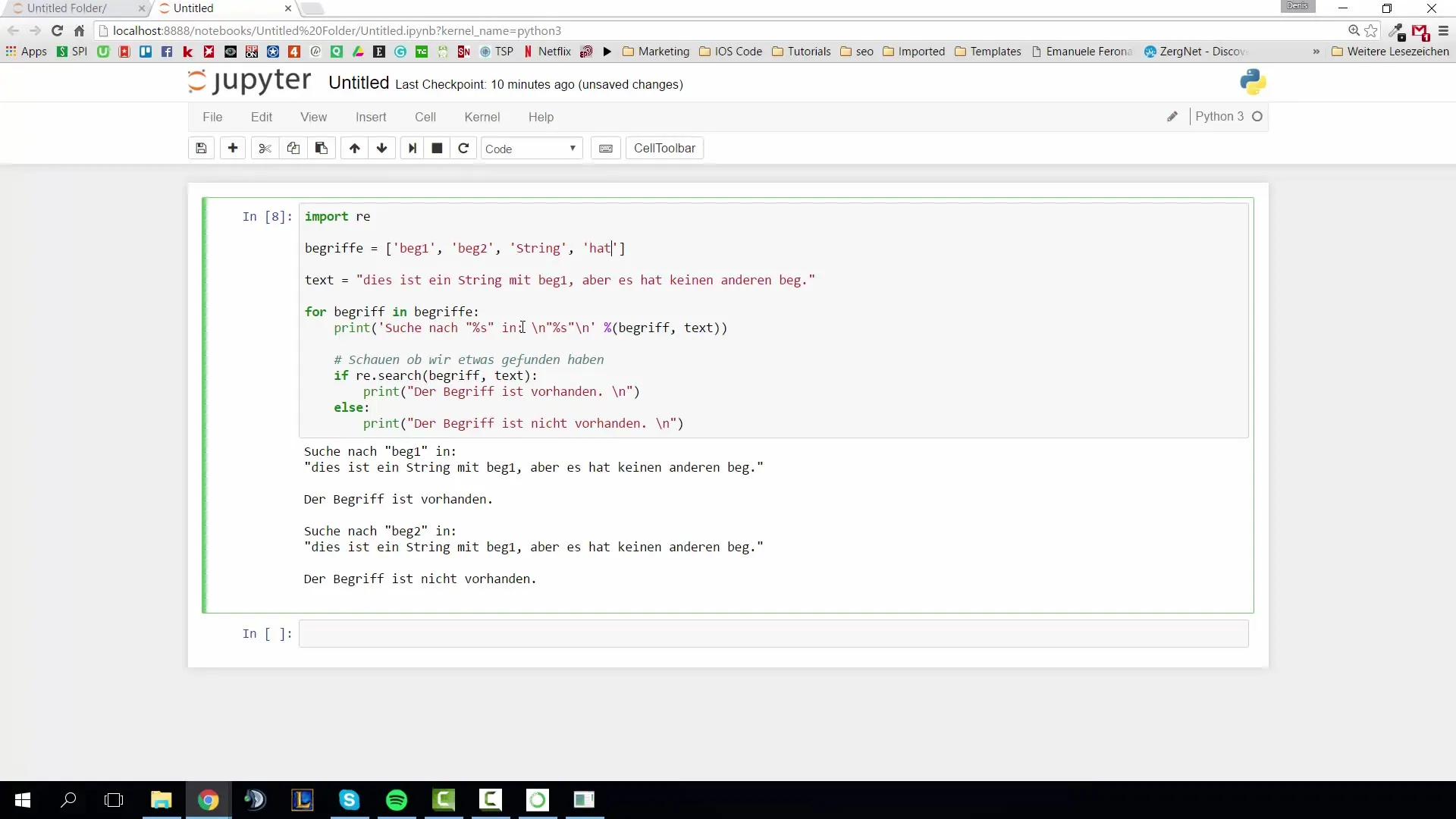
To find out the position of a term in the text, you can use the match.start() and match.end() functions. These provide the start and end positions for your search results.
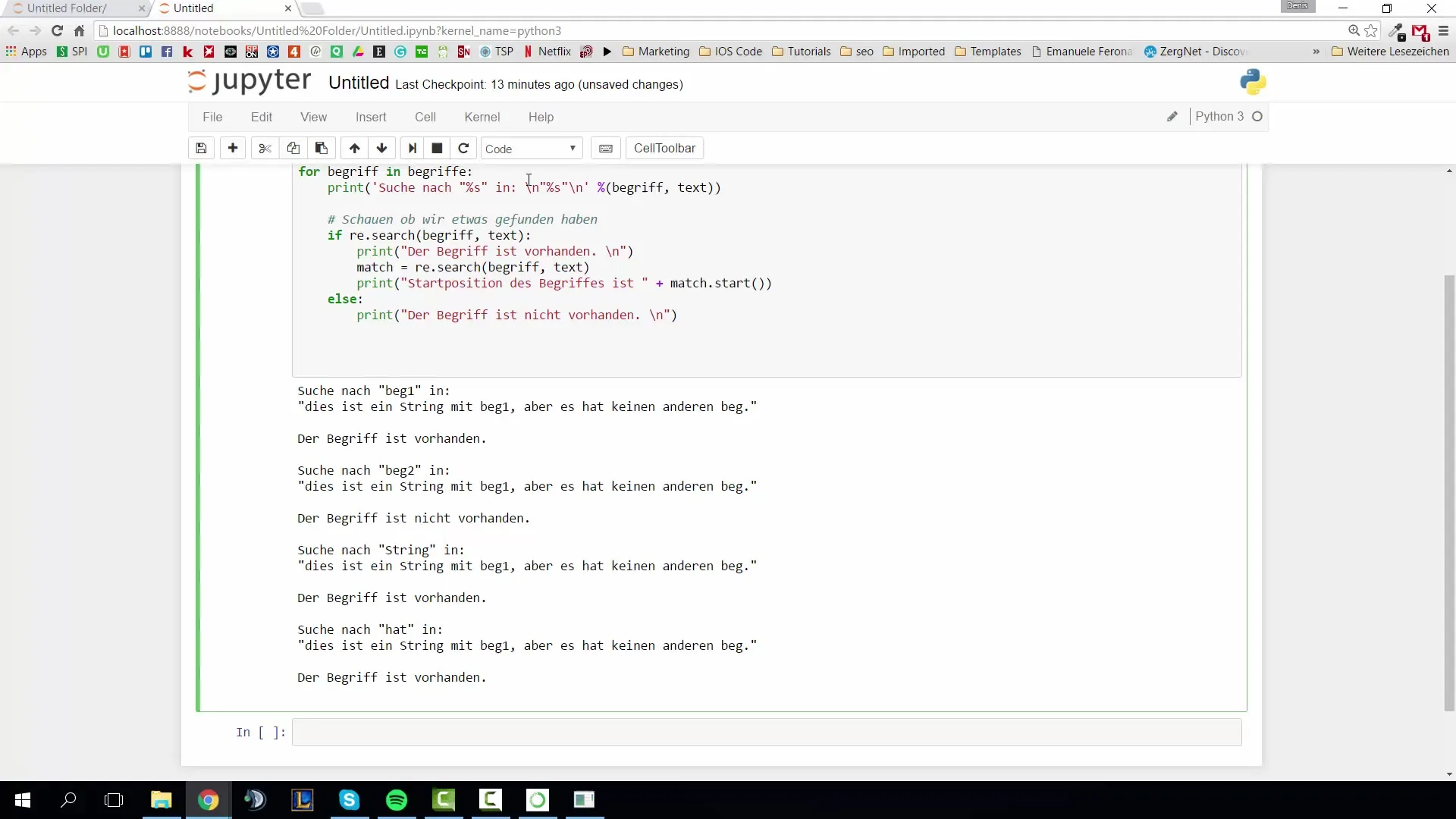
Using match.start() gives you the exact position where the term starts within the text. With match.end(), you get the end position of the found term.
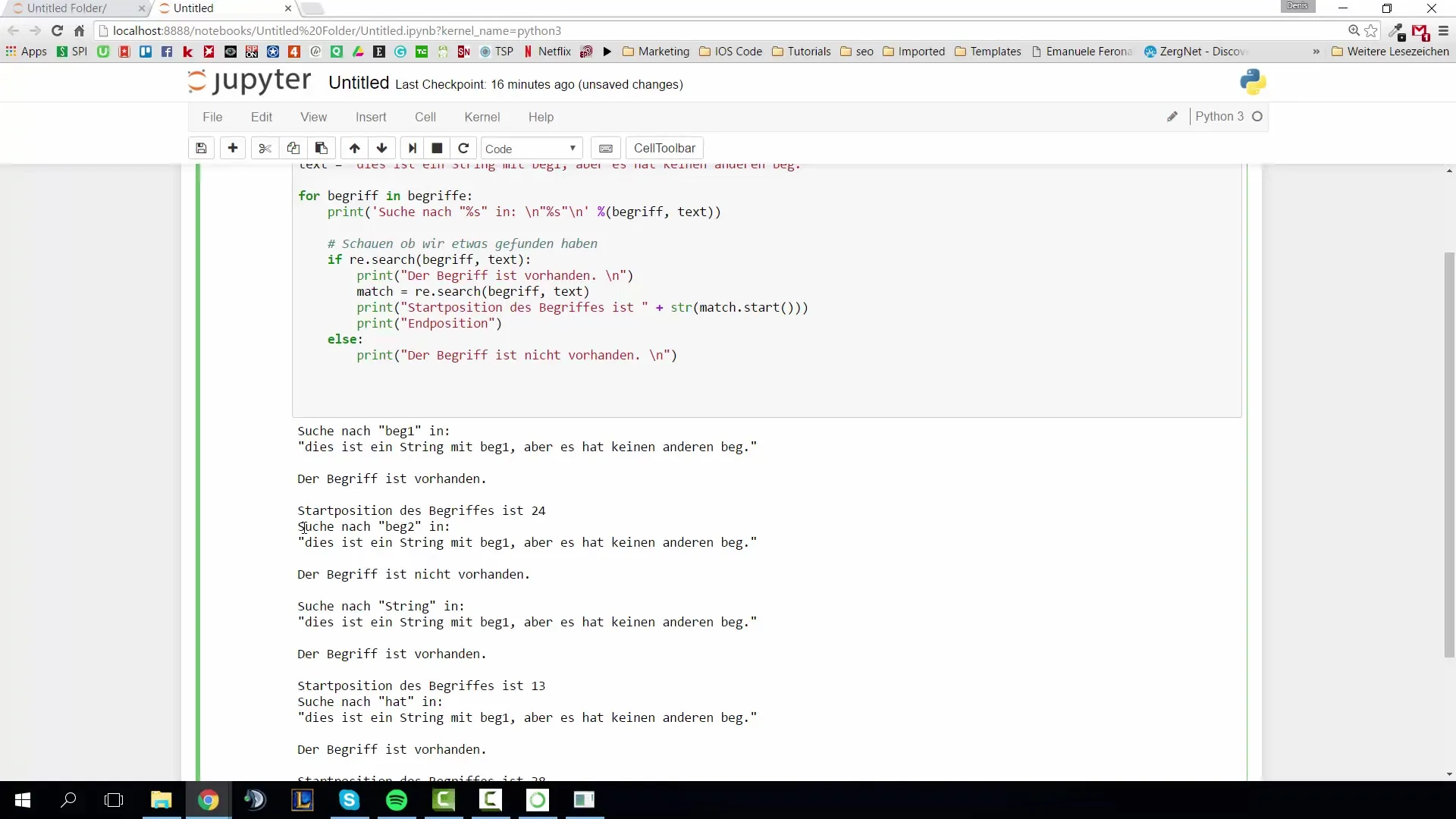
In this way, you can not only identify the found terms in the text but also determine their exact positions, which is helpful for targeted editing.
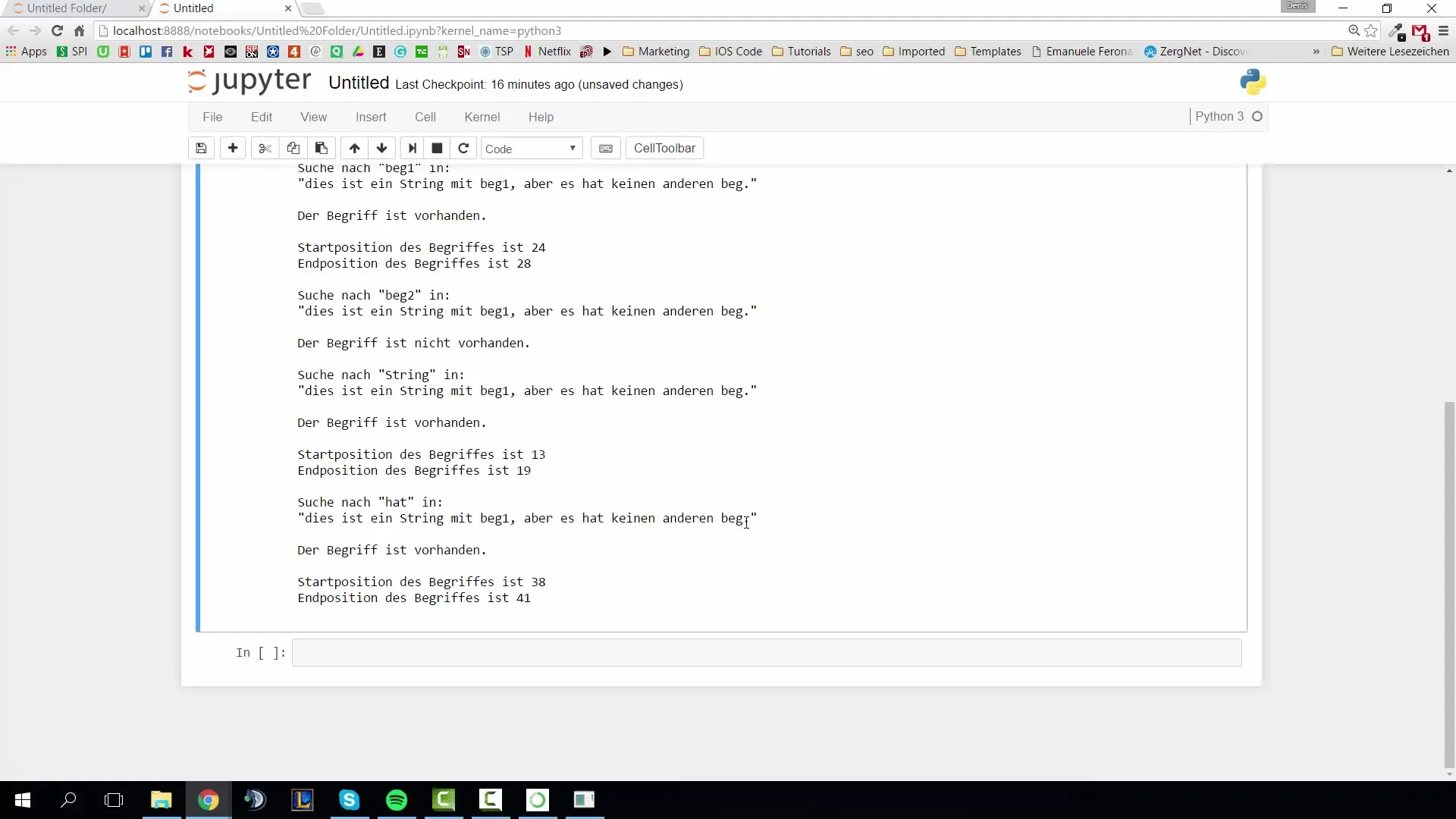
In the next step, it gets exciting because the following guide will show you how to not only search patterns with regular expressions but also isolate or replace specific parts of the text. This opens up a multitude of possibilities for performing textual manipulations.
Summary – Introduction to Regular Expressions with Python: re.match and re.search
Regular expressions are an essential tool in programming when it comes to analyzing and modifying text data. In this guide, you have learned how to use Python's re.match and re.search modules to find terms in a text. You have also learned how to determine the positions of the searched terms.
Frequently Asked Questions
How do I import the re module in Python?Import the module with "import re".
What is the difference between re.match and re.search?re.match only searches at the beginning of the string, while re.search searches anywhere in the string.
How do I find the position of a term in the text?Use the methods match.start() and match.end().
Can I use regular expressions to replace text?Yes, for that you can use the re.sub() function.
Where can I learn more about regular expressions?Check the official Python documentation or online resources on regular expressions.