Programming with Python is an exciting adventure that opens up many thrilling possibilities. A valuable part of this journey is understanding the various data types. In this tutorial, you will become familiar with a special data type – the tuple. Tuples are an effective way to store multiple values in a single data structure, without being able to change them. Let's dive deeper and explore the possibilities of tuples in Python.
Key Insights
- Tuples are immutable sequences of elements.
- They can contain different data types, including numbers and strings.
- Tuples have special functions like length, index, and count that make their use easier.
- There are limitations when it comes to overwriting elements in tuples.
Creating and Using Tuples
To create a tuple in Python, you use parentheses. This is the first important step.
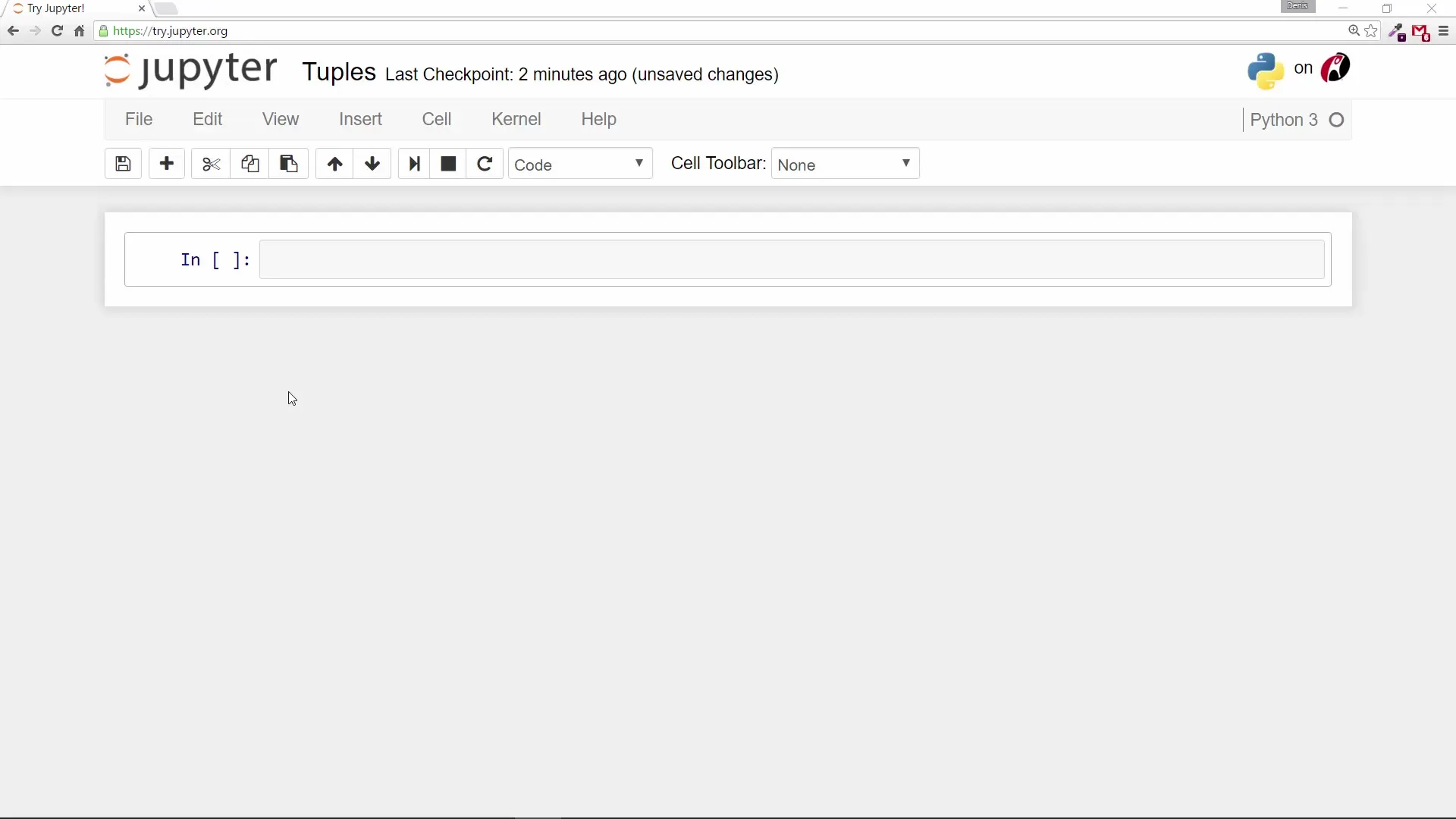
Here you have created a tuple with the values "a", "b", and "c". If you want to print this tuple now, just enter t.
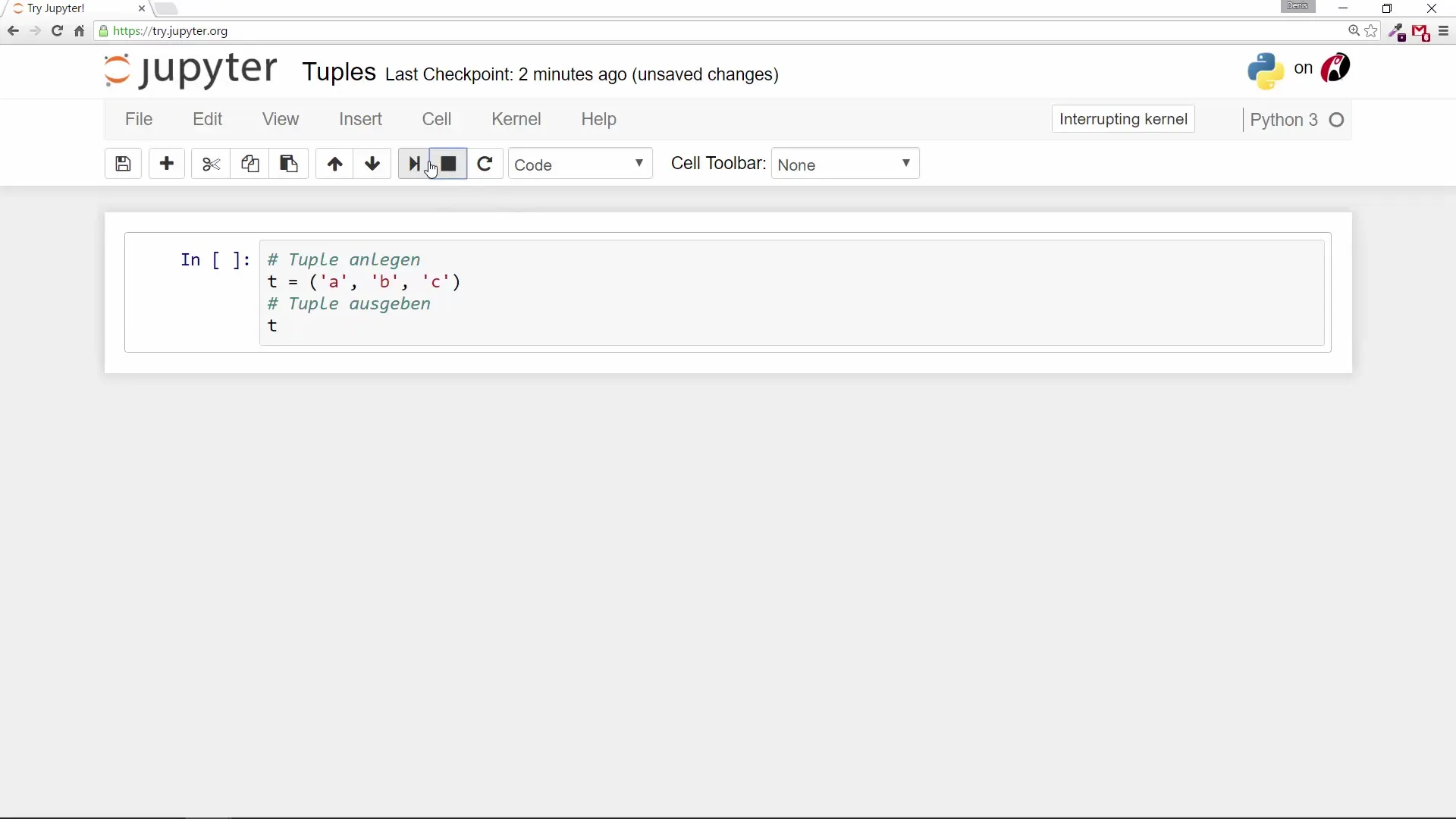
The result will be that the values ABC will be displayed. Note that the crucial difference between tuples and other data types like lists is the brackets used. While tuples use round brackets, lists use square brackets and sets use curly brackets.
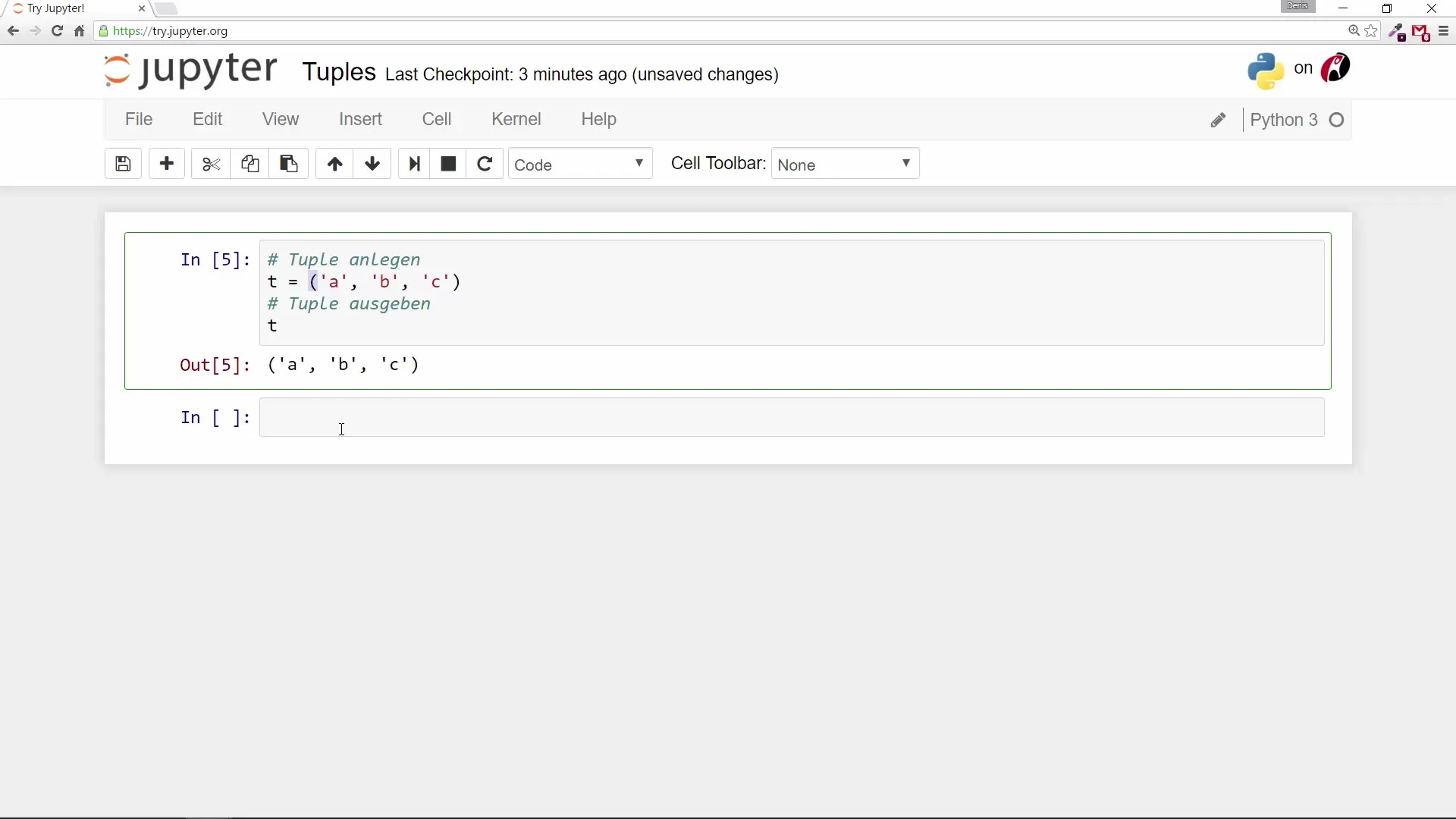
Finding the Length of a Tuple
To find the length of a tuple, you can use the len() function. This is especially useful when working with a large amount of data.
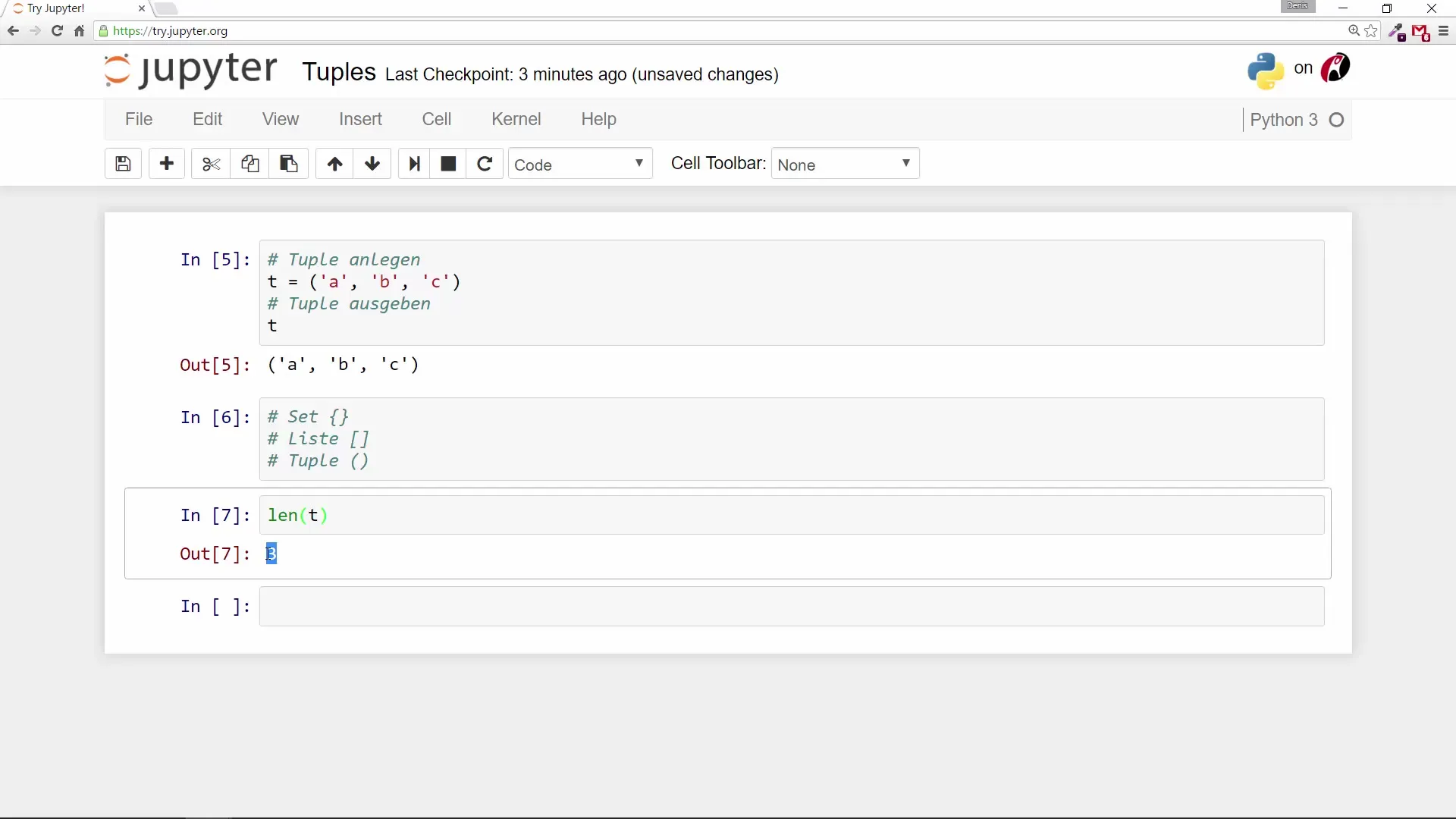
With len(t), you will get back the number of elements in the tuple.
Accessing Elements within a Tuple
One of the practical properties of tuples is that you can access their elements based on their index. In Python, indexing starts at 0.
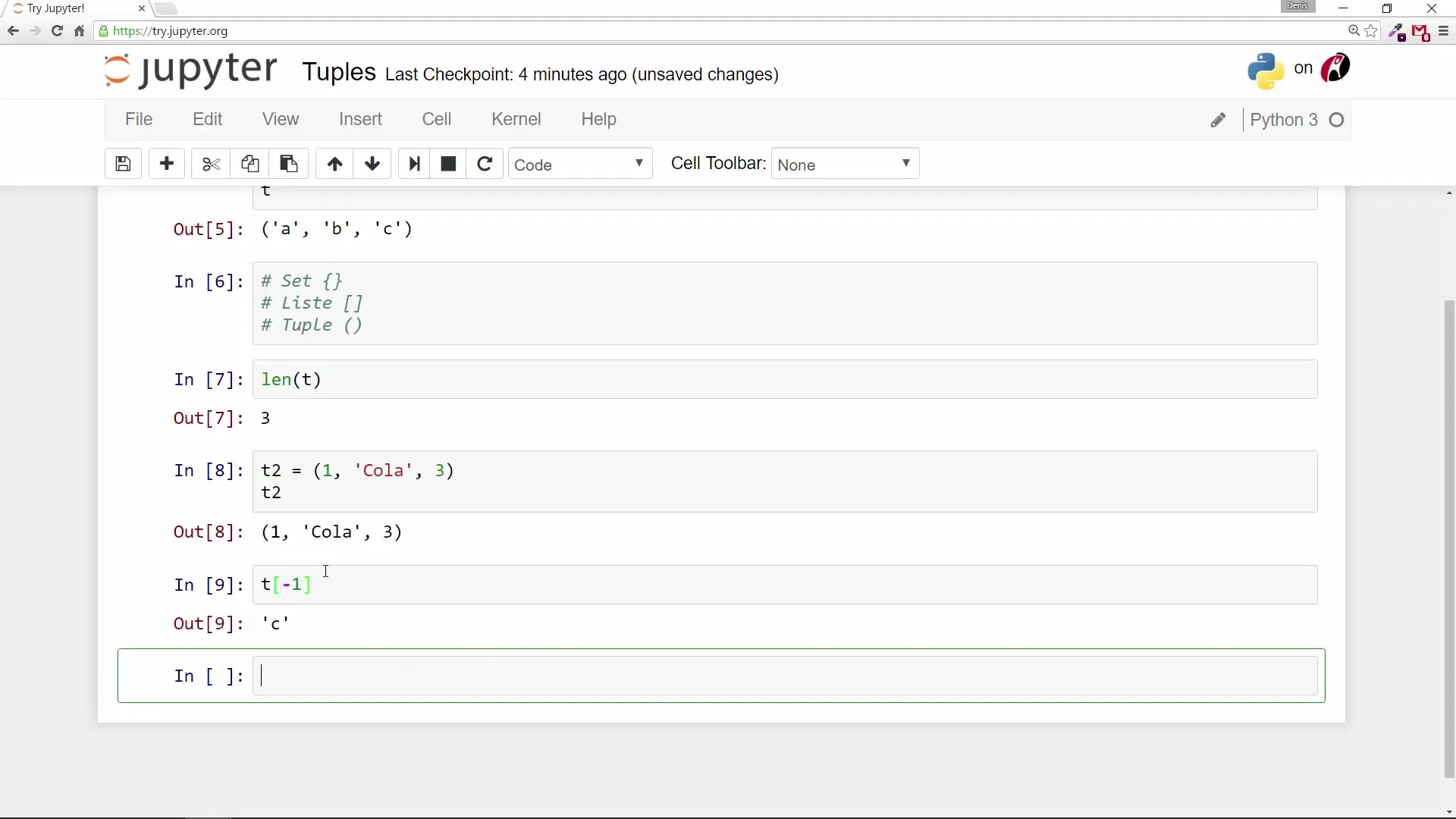
So, if you want to access the last element, you can use the negative index:
For a tuple like t = ("a", "b", "c"), this will yield "c".
Slicing Tuples
You can use the slicing method in Python to extract parts of a tuple. This means you can get a subset of the values from your tuple.
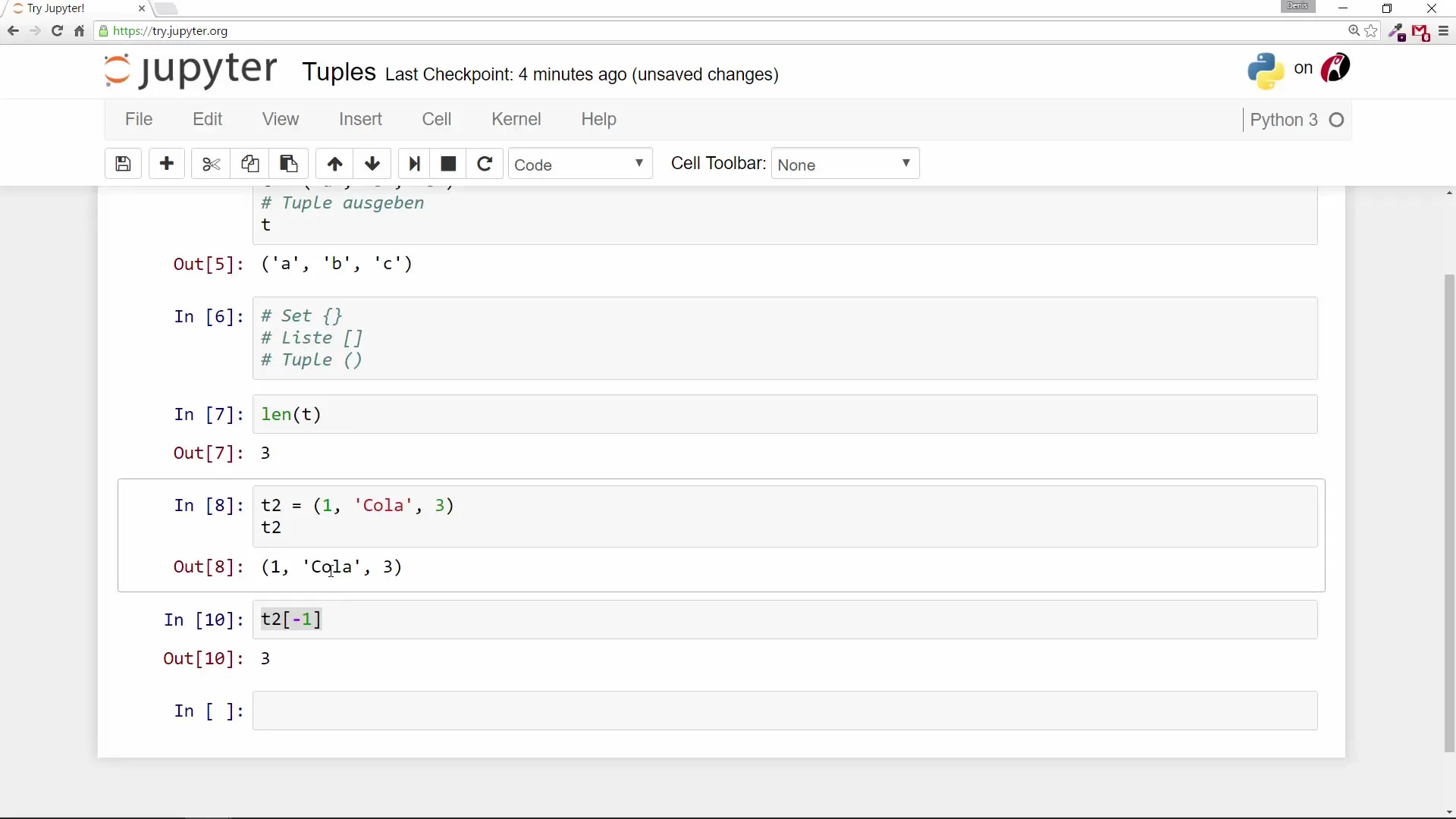
An example would be t[1:], where you get all elements starting from the second element to the end of the tuple.
Using Functions for Tuples
You can apply special functions to tuples to get additional information. These include:
Finding the Index
With the index() function, you can find out the position of a certain value in the tuple.
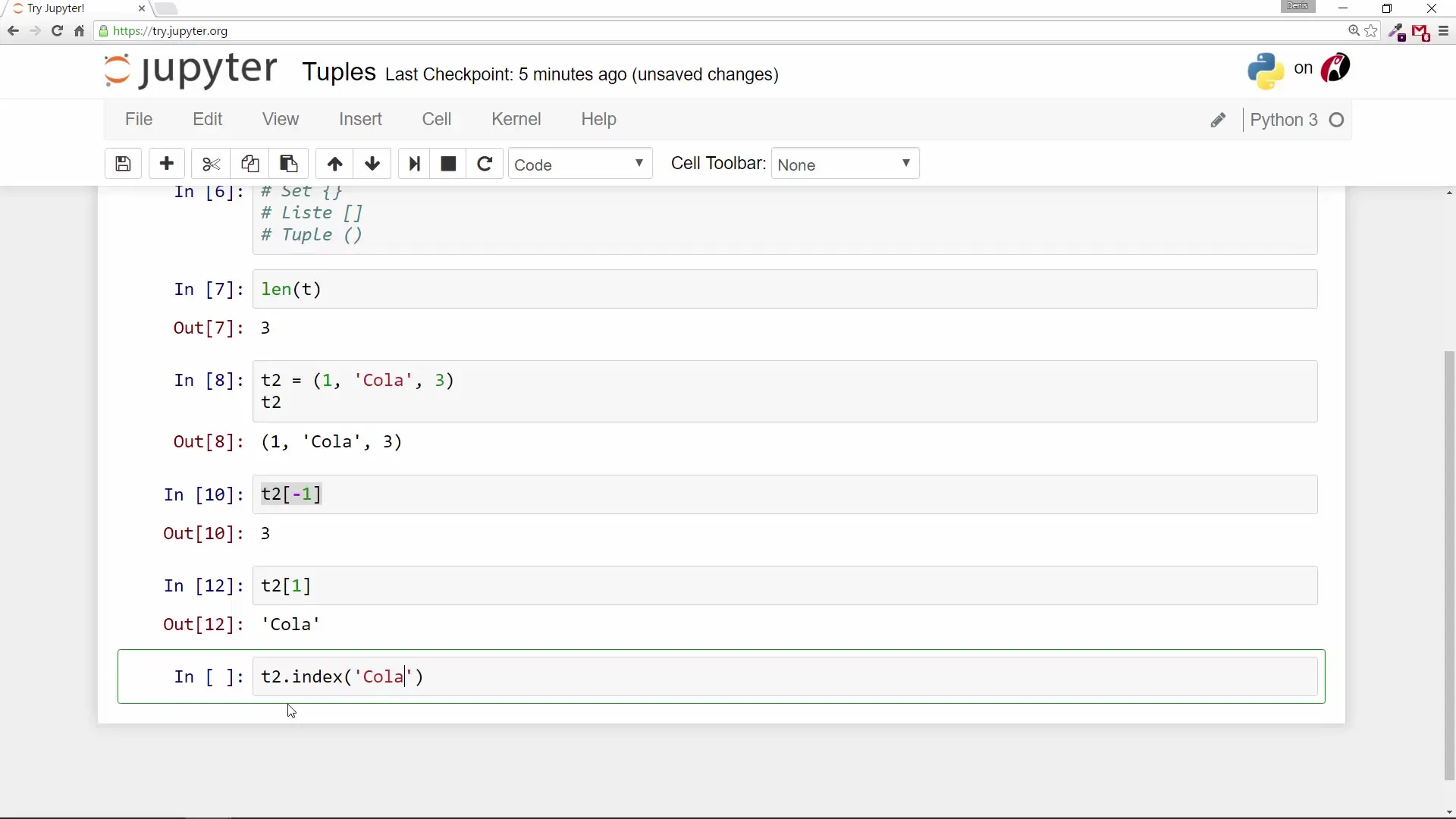
Counting Frequency
What to do if you want to know how often a certain value appears in the tuple? This is where the count() function comes into play:
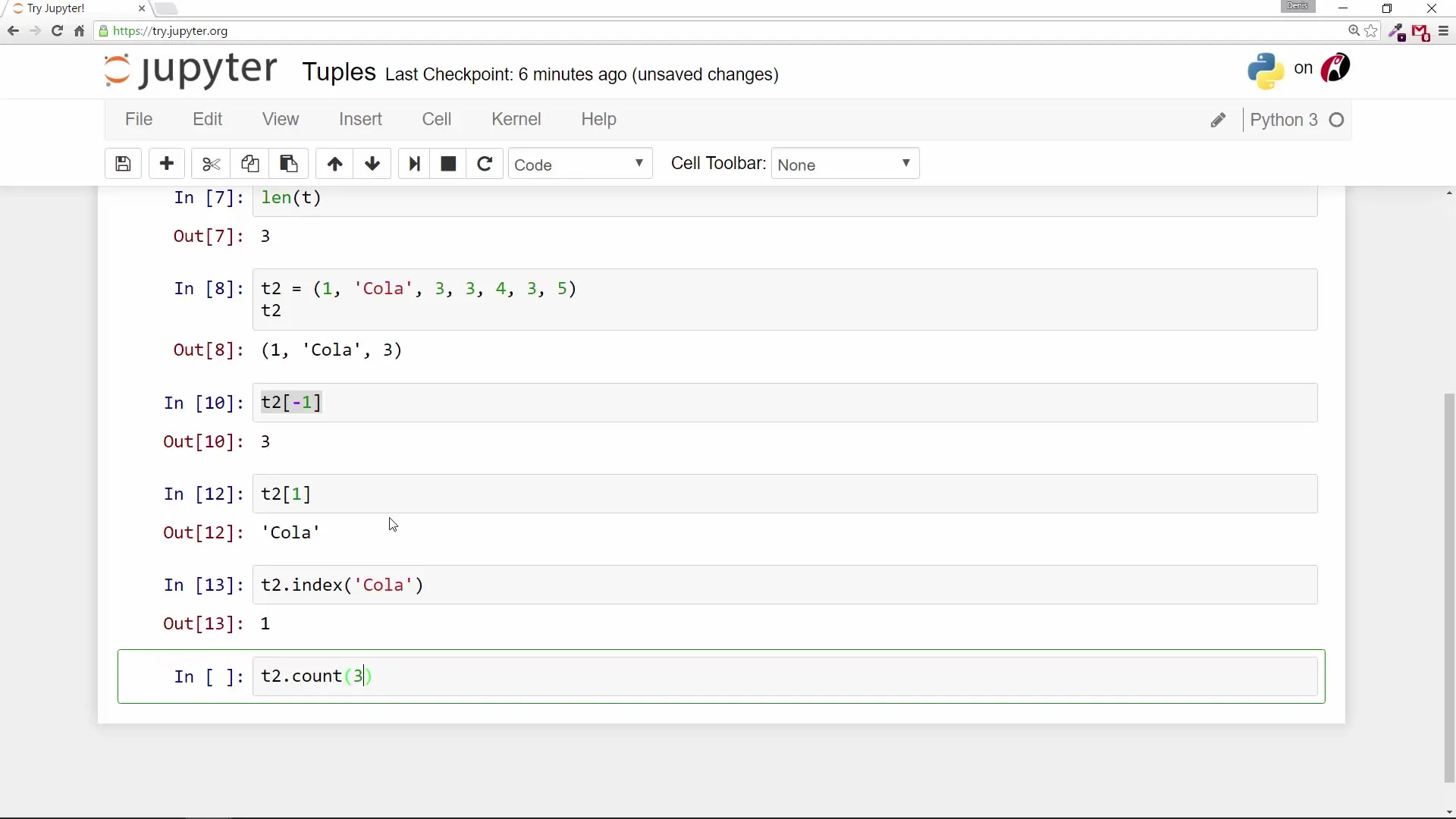
With t.count("c"), you will get the number of occurrences of "c" in the tuple.
Making Changes to Tuples
An important point to keep in mind: Tuples are immutable. You cannot directly change an element in a tuple.
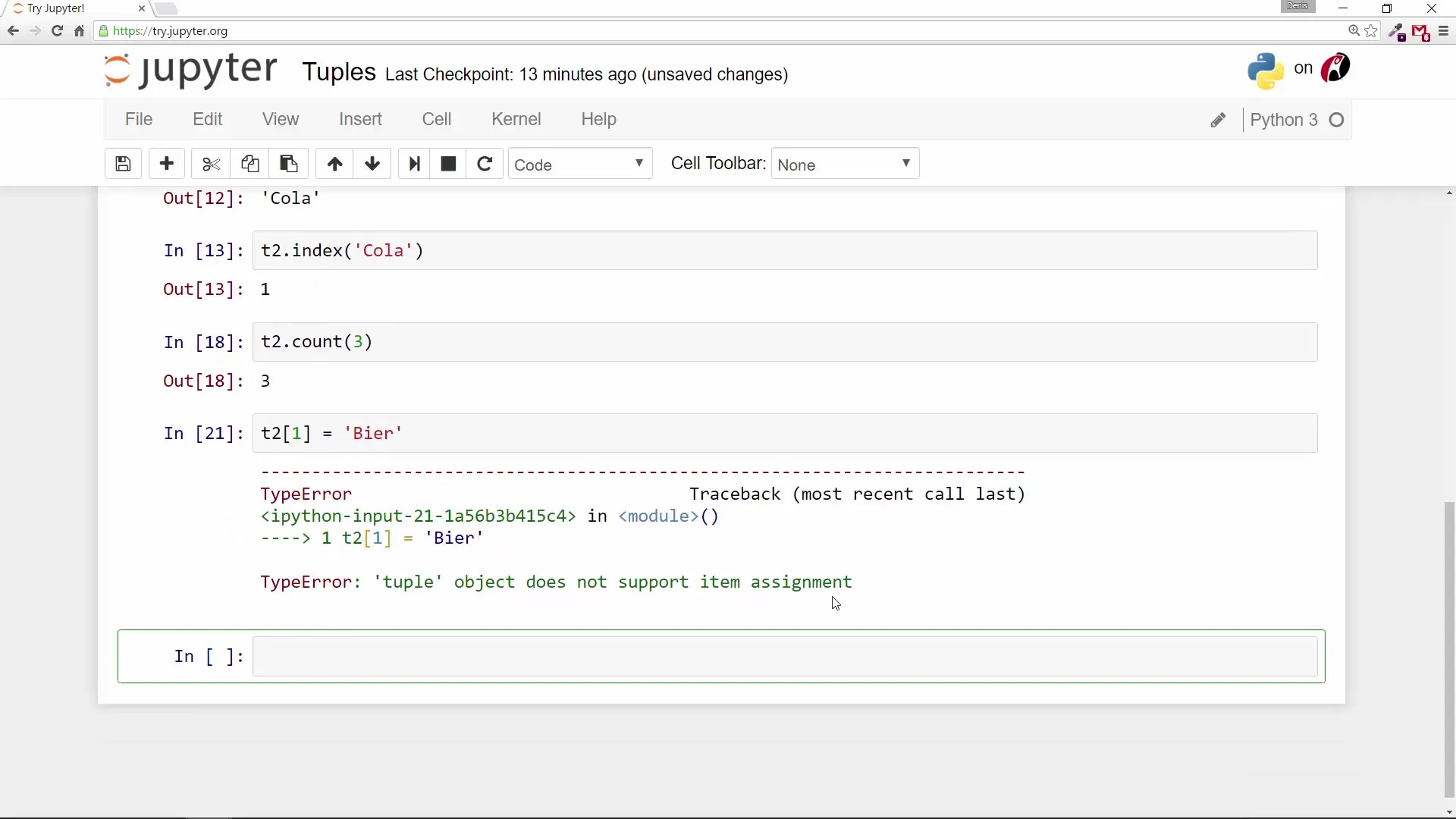
Creating a New Tuple
If you want to change the contents of a tuple, a new tuple needs to be created. A common strategy is to define a new tuple while using the old tuple in specific parts.
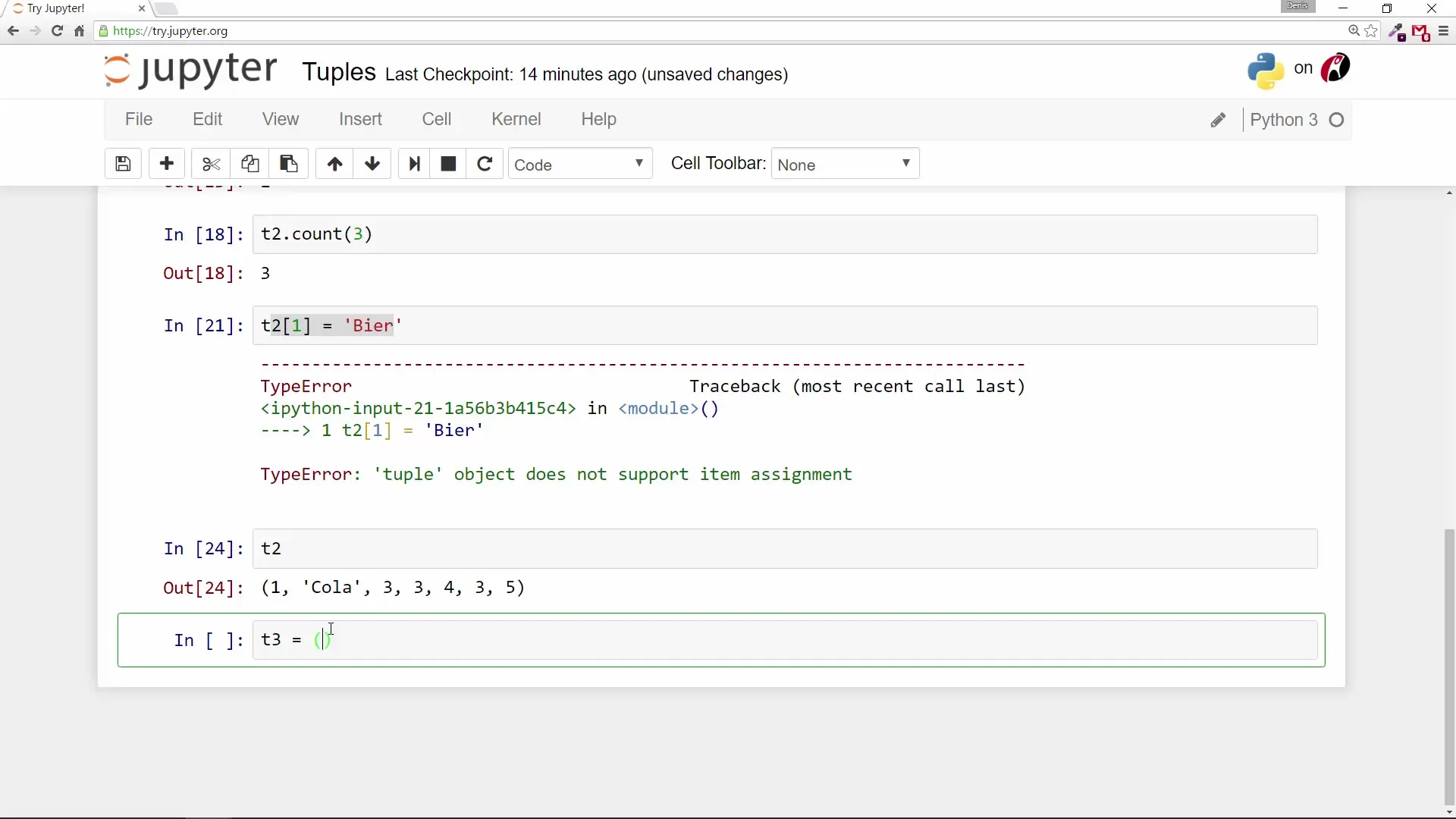
The result is a new tuple, where the first element is now "beer".
Conclusion
After this detailed introduction to tuples, you have learned the basics of working with this data type in Python. You now know how to create a tuple, access it, and perform some basic operations with it. The immutability of tuples offers a certain level of security and can help prevent unwanted changes to important data.
Summary – Python Programming for Beginners: Tuples in Detail
Frequently Asked Questions
What are tuples in Python?Tuples are immutable sequences of elements created using round brackets.
How do I create a tuple?A tuple is created with round brackets, e.g., t = ("a", "b", "c").
Can I change the values in a tuple?No, tuples are immutable and do not allow direct changes to the values.
How do I find the length of a tuple?Use the len() function, e.g., length = len(t).
What does the index() function do?The index() function returns the position of a specific value in the tuple.