Dictionaries are one of the most powerful features in Python, allowing you to store and retrieve data in a structured way. They are more than just a simple list of values; dictionaries allow you to associate values with a key, which significantly simplifies data navigation. In this guide, you will learn without hesitation how to effectively work with dictionaries.
Key Insights
- A dictionary allows the association of key-value pairs.
- You can store different data types in a dictionary.
- It is possible to create nested dictionaries to represent complex data structures.
- The order of elements in a dictionary is not important.
Step-by-Step Guide to Dictionaries in Python
First, it is important to clarify what dictionaries are and how they differ from lists. While lists have a defined order of elements, dictionaries operate on key-value assignments. Let's take a closer look at this.
1. Creating a Dictionary
To create a dictionary, you use curly braces.
2. Accessing Values
If you want to access a specific value in your dictionary, you do this with the respective key.
This will return the value "house". Make sure you have no spaces between the key and the access to avoid errors.
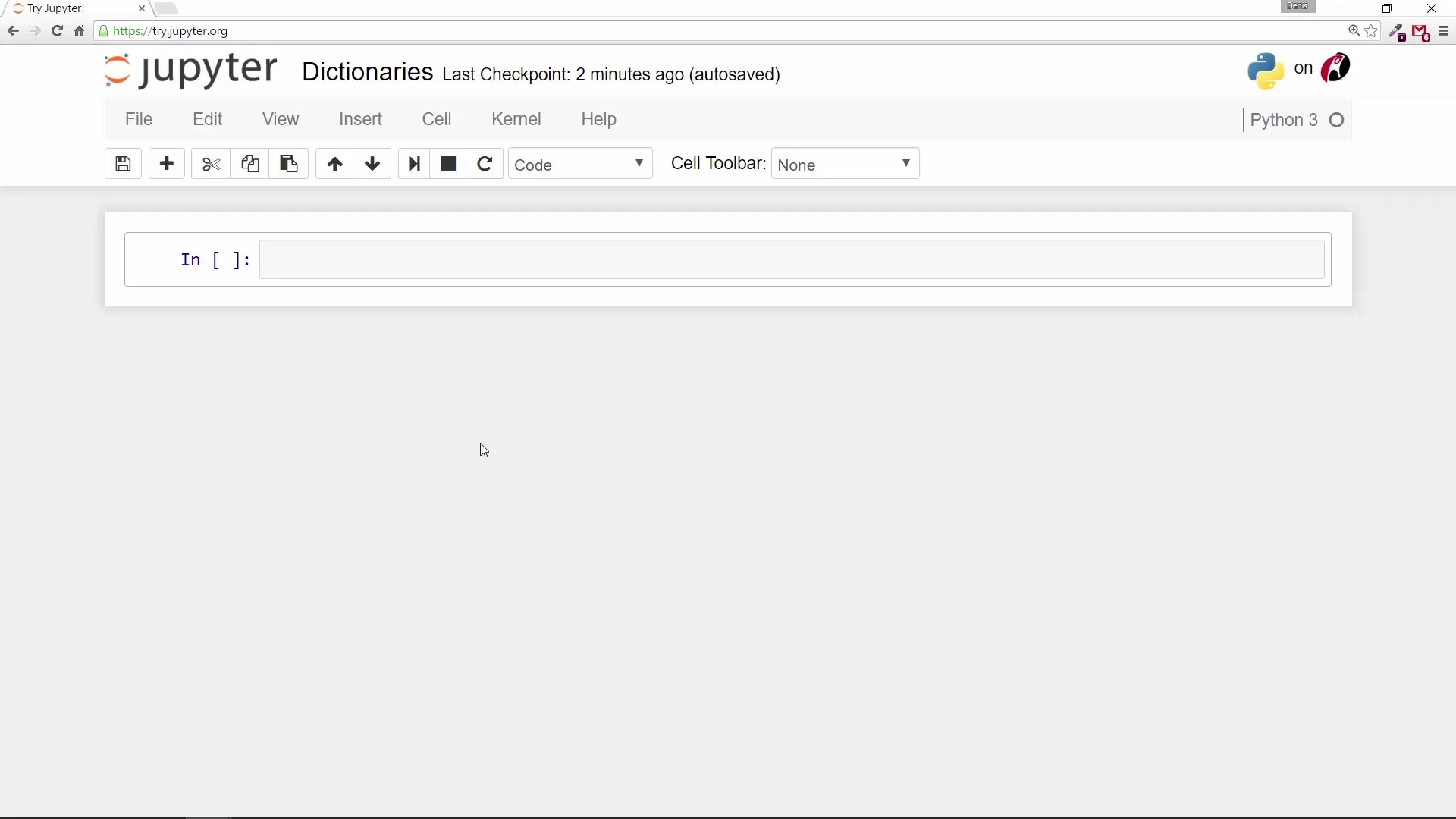
3. Different Data Types
Another significant advantage of dictionaries is the ability to store various data types.
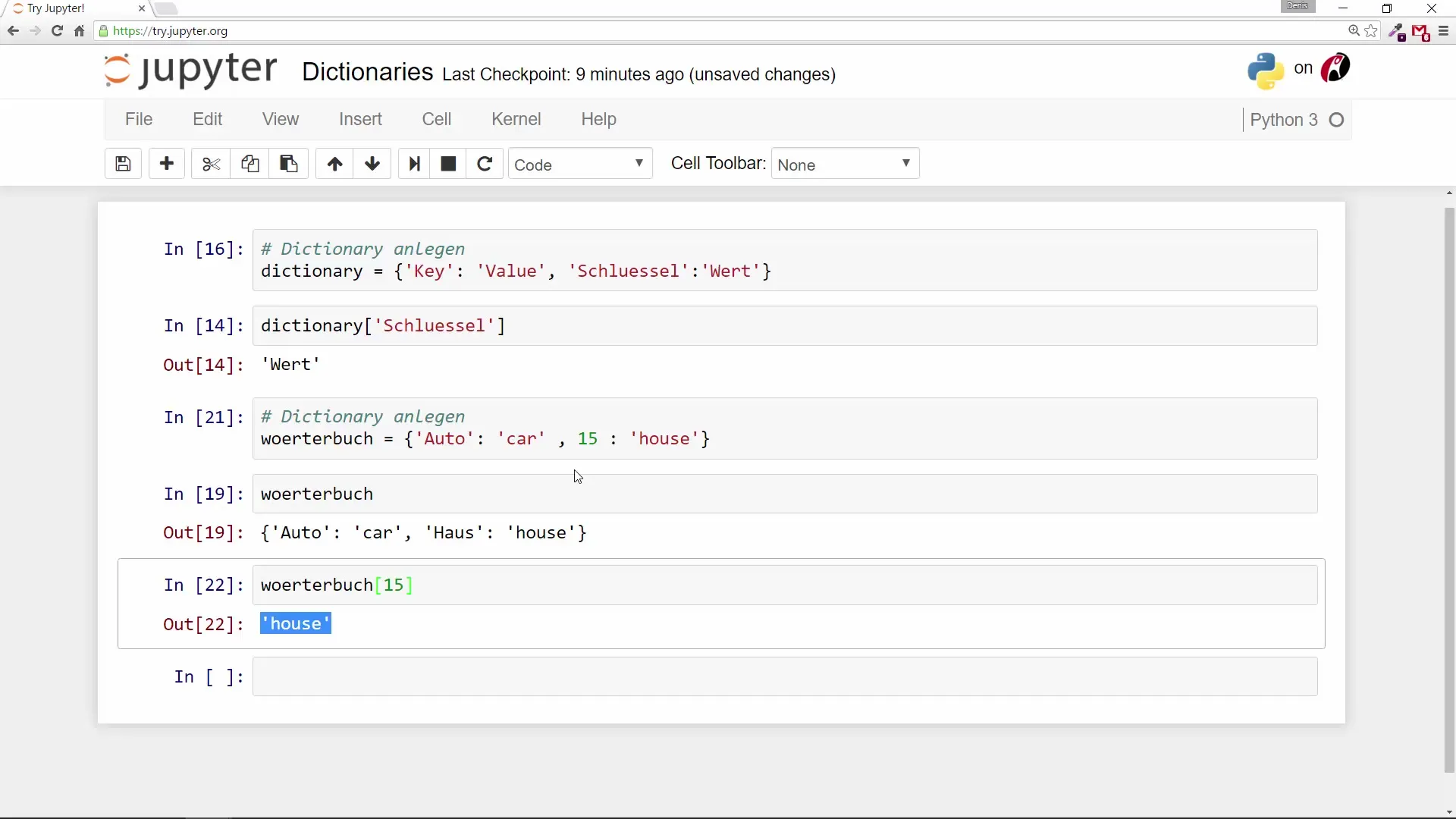
4. Using Lists in Dictionaries
You can access the second element of the list by specifying the key and then the index of the list.
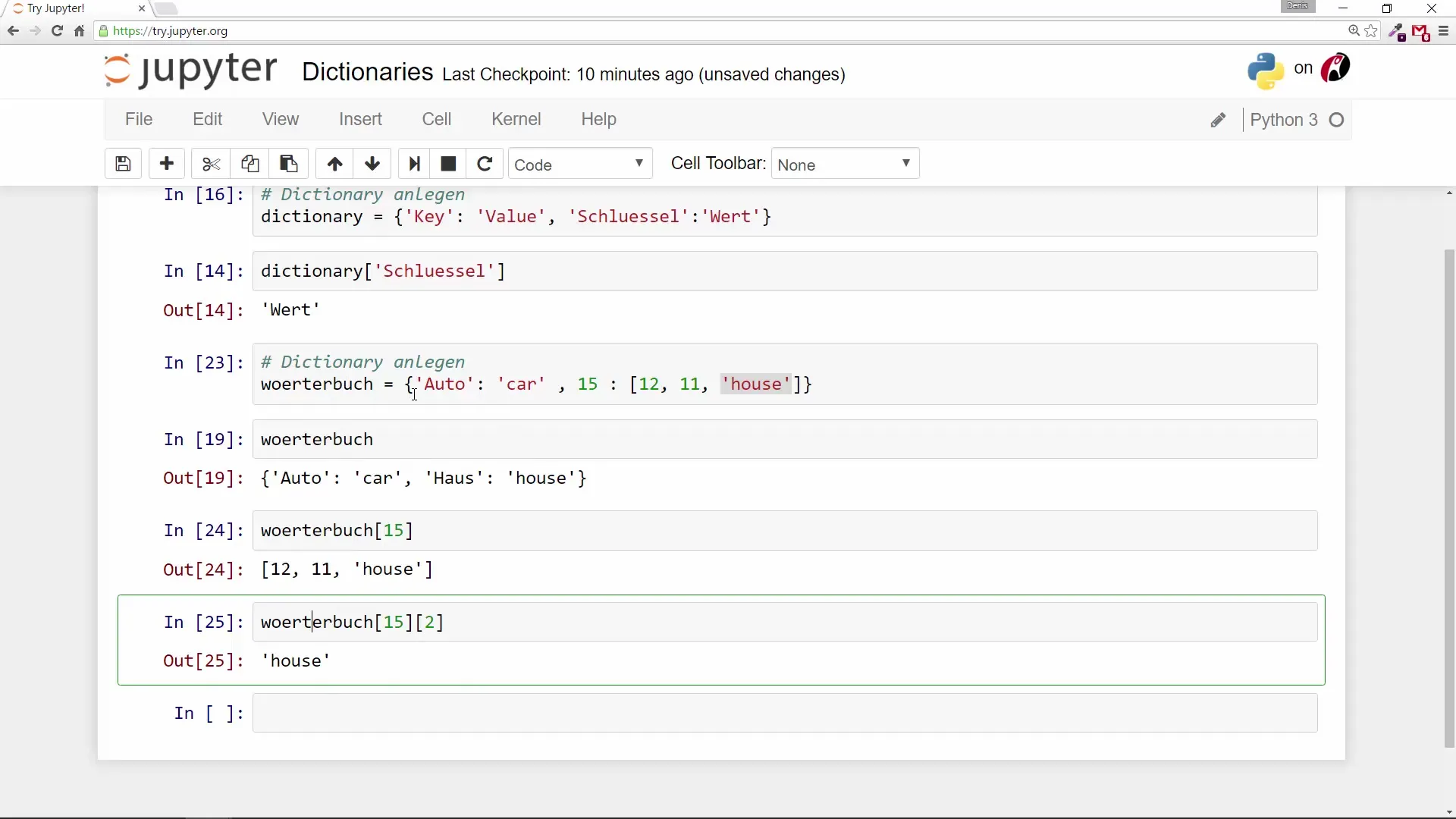
5. Empty Dictionary and Value Assignment
To create an empty dictionary, you can simply use the curly braces. Define the dictionary and then assign values.
Now you can gradually fill the contents of the empty dictionary.
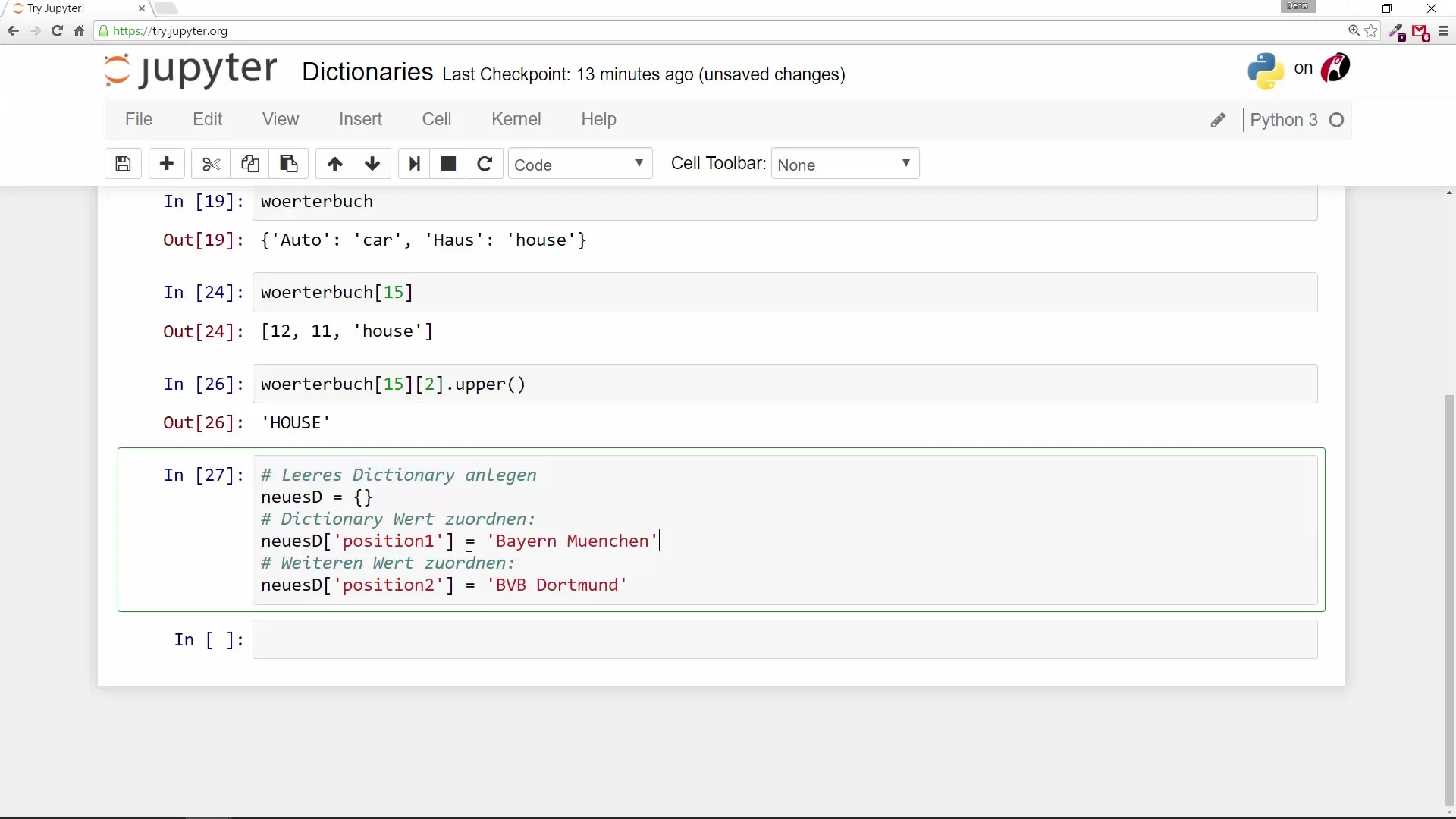
6. Working with Functions
With dictionaries, you can use functions like.keys() and.values() to gain information about the keys and values.
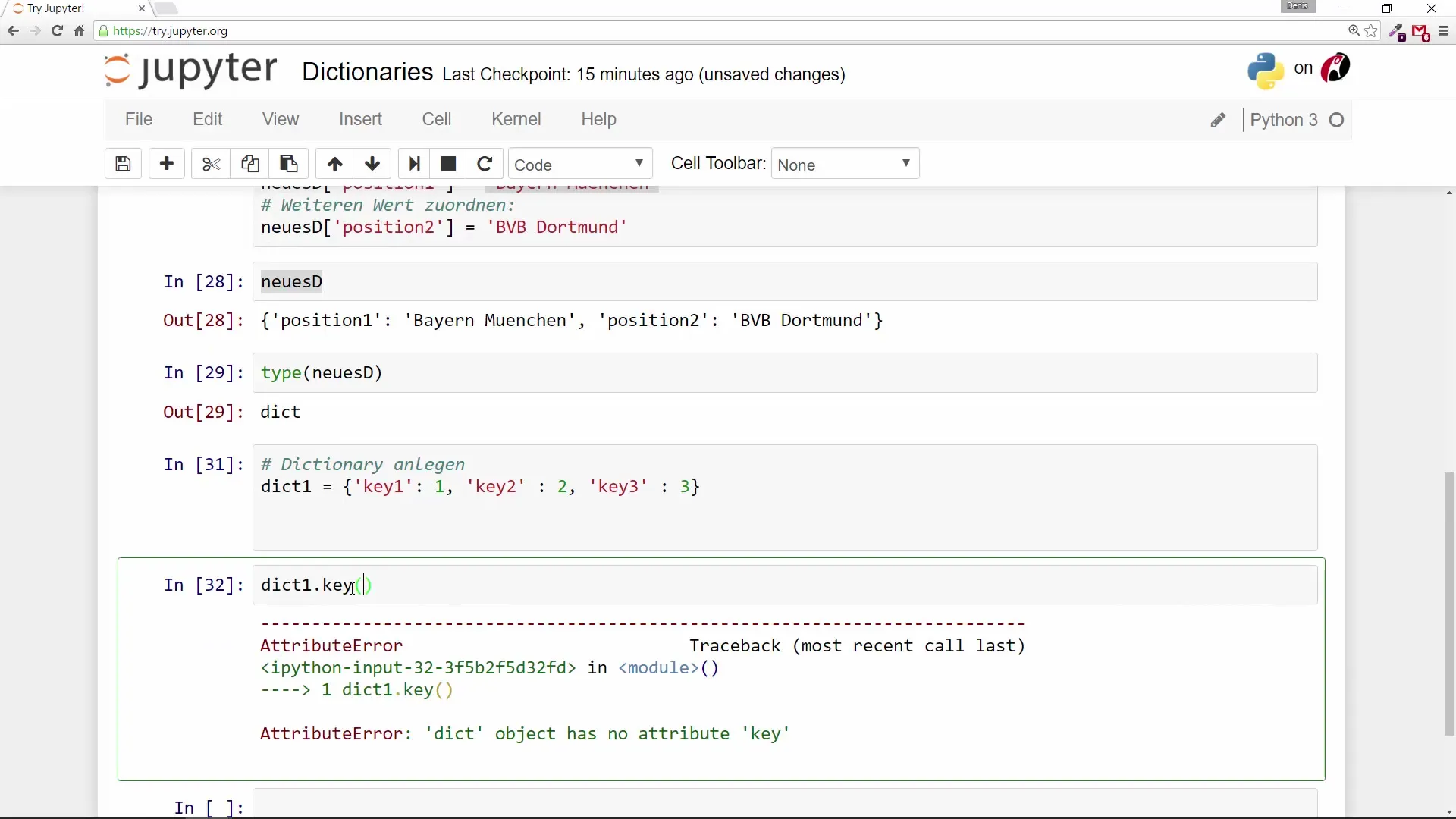
7. Nested Dictionaries
Dictionaries can also be nested. This means that a dictionary can be stored as a value in another dictionary.
To access a value in a nested dictionary, use the key syntax.
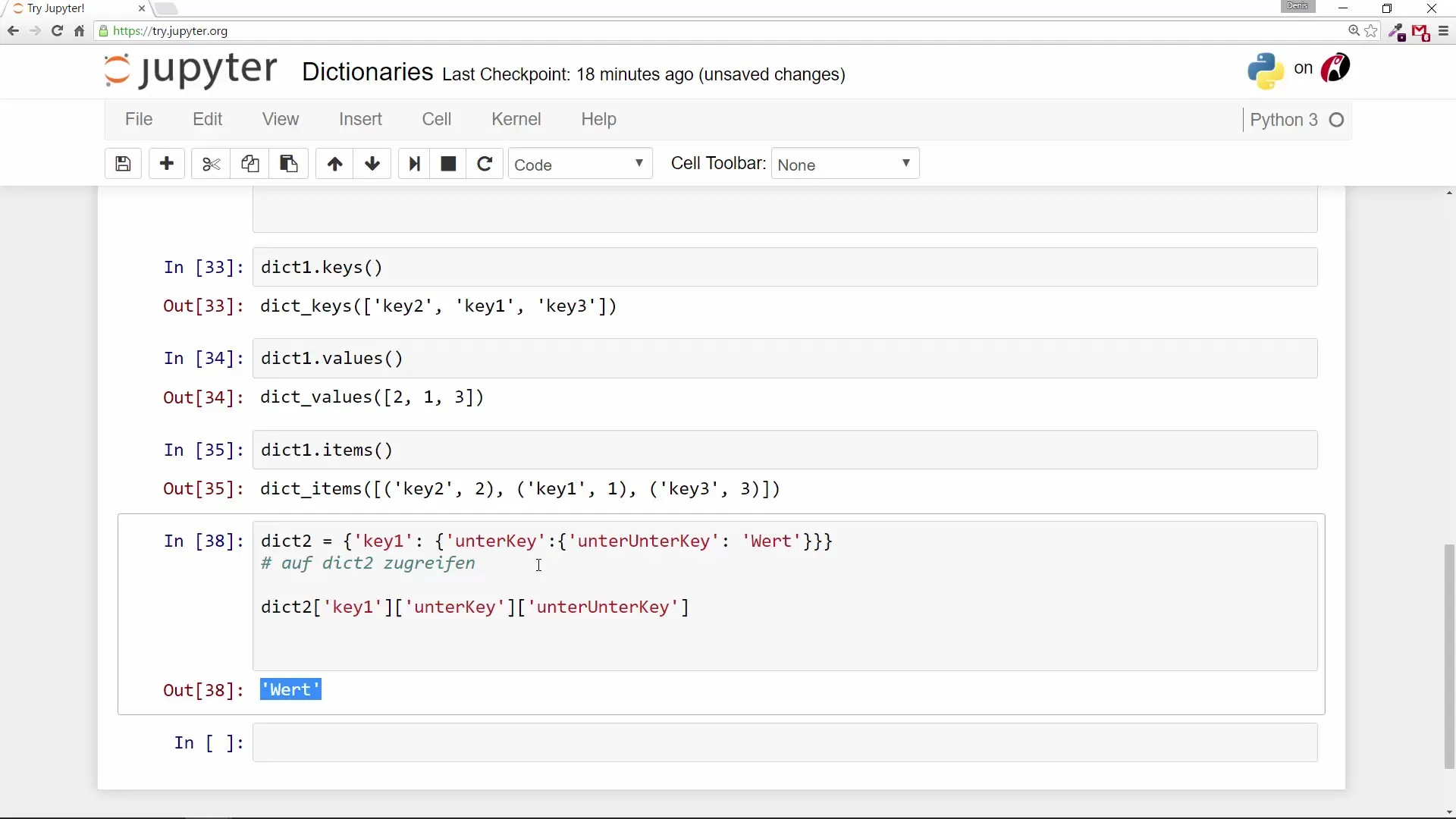
8. Summary of Functionality
We have seen how to create dictionaries, how to assign different data types, access values, and create more complex data structures with nested dictionaries and lists. Dictionaries are powerful tools for organizing and managing data in your programs.
Summary – Introduction to Working with Python Dictionaries
In this guide, you learned what dictionaries are, how to create them, and how they can help you organize data efficiently. There is a clear difference between dictionaries and lists, as well as the possibility to create more complex data structures.
Frequently Asked Questions
What is a dictionary in Python?A dictionary is a data type in Python that allows for the mapping of key-value pairs.
How do I create an empty dictionary?You create an empty dictionary by simply using two curly braces: meinDictionary = {}.
Can I store different data types in a dictionary?Yes, you can store different data types in a dictionary, including strings, numbers, and lists.
What happens if I access a non-existent key?If you try to access a non-existent key, Python will raise a KeyError.
How can I change a value in a dictionary?To change a value, you can use the key and assign the new value: meinDictionary["key"] = newValue.