When you start with Python, it is essential that you understand the concept of Booleans and logical operators. In this tutorial, I will introduce you to these basic yet crucial concepts so that you can effectively use them in your programs.
Key insights
- Booleans are variables that can only take the values true, false, or none.
- Logical operators help you make comparisons between values to determine whether a condition is true or false.
- Handling conditions in Python is essential for programming functional applications.
Introduction to Booleans
Let's start with what a Boolean actually is. A Boolean is a variable that can take exactly two values – true or false. Sometimes, the state of the variable can even be none, which means it has no value. You can easily create such a variable in a Python environment like Jupyter Notebook.
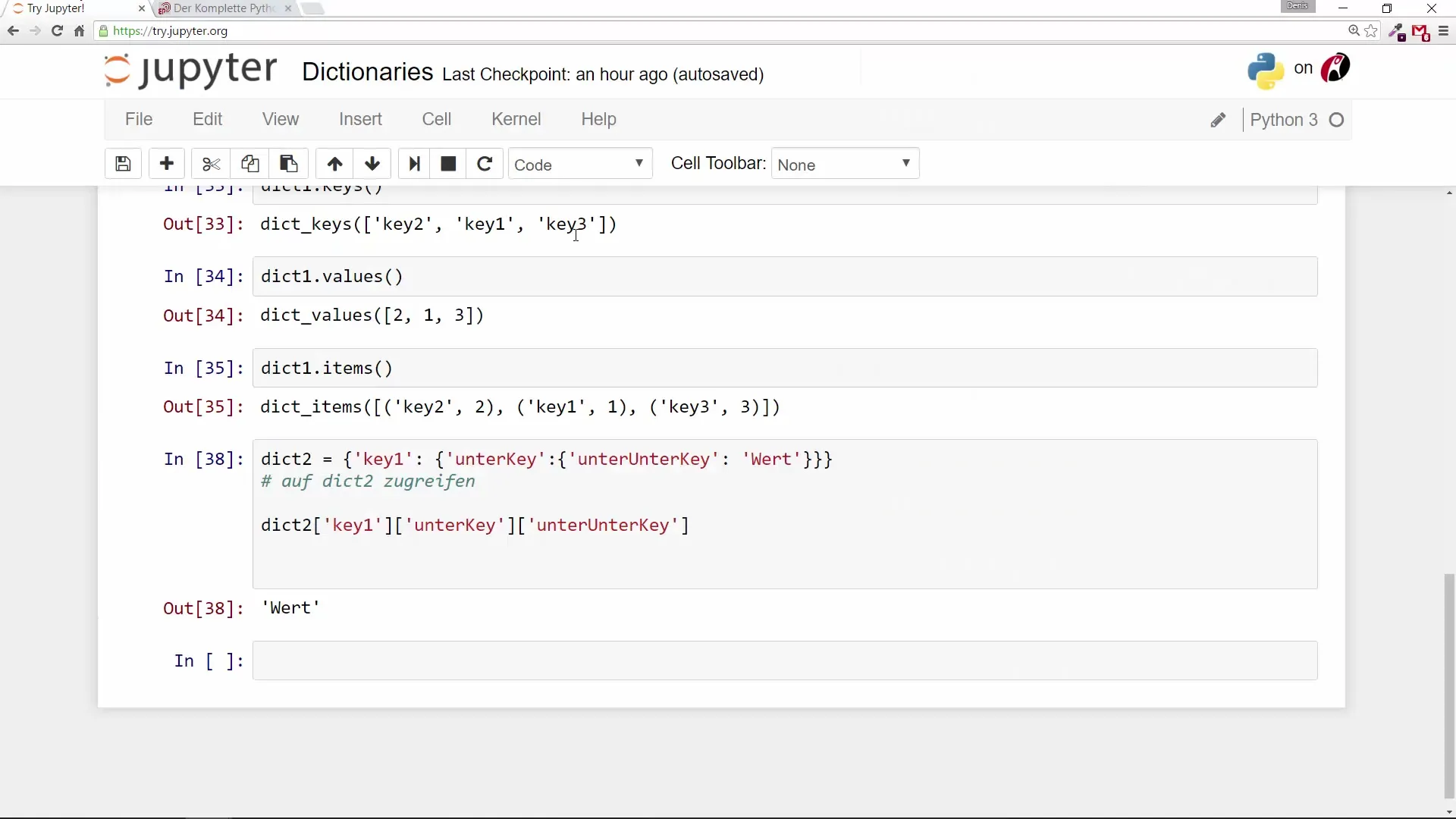
To illustrate, let's say you create a variable named B and set it to true. This means that this variable has the value true. You must pay attention that true is capitalized in Python, otherwise, it will generate an error.
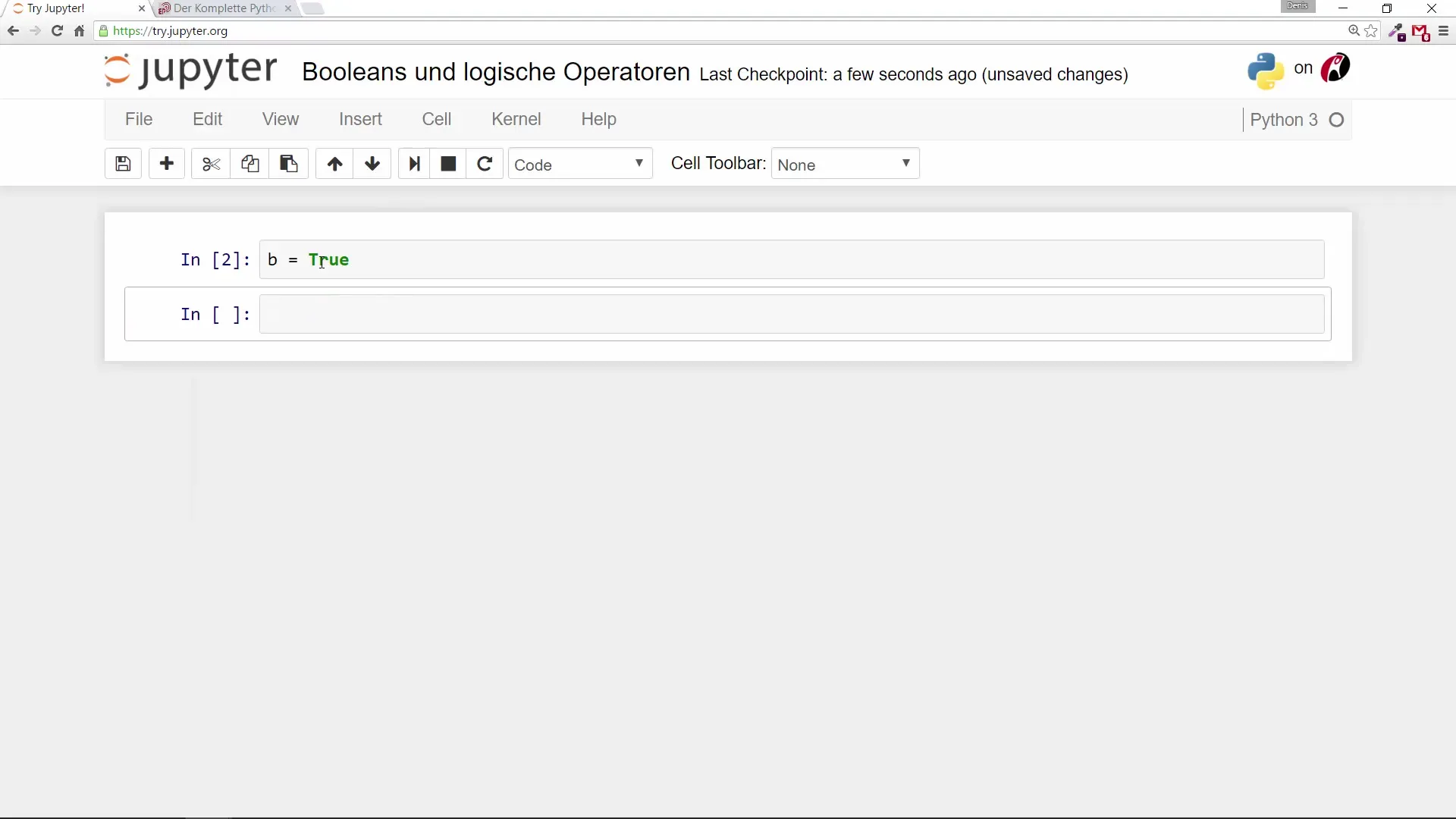
Imagine you are developing an application where you need to know whether a user is logged in or whether they have premium access. You can simply create a Boolean variable like is Premium Member and set it to true when the user logs in. This gives you access to certain areas of your program.
Conversely, the value could be set to false if the user is not logged in, thereby denying access to premium content.
Comparing Booleans
Booleans are not only useful as state indicators, but they can also be derived from other variables. For example, if you want to find out if 3 is greater than 5, you can simply phrase this statement: is 3 > 5? The result is false because that is not true.
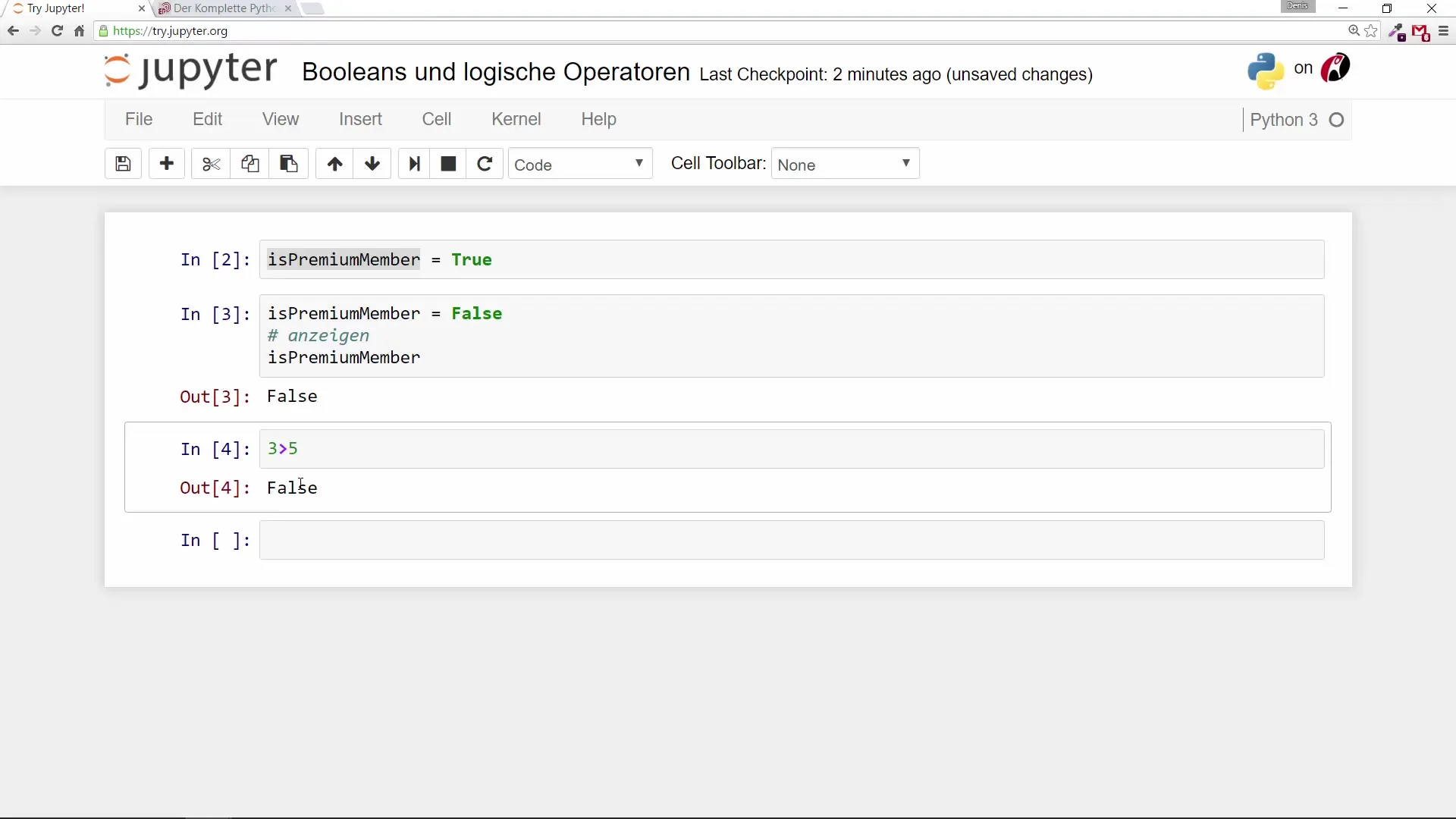
We can also work with other operators, like comparing states. If you create a variable C and assign it the null value (none), it means that C currently has no value and is neither true nor false.
Logical Operators
Now that you have a basic understanding of Booleans, let's take a closer look at logical operators. These operators allow you to check conditions that then return true or false. For example, there is the equality operator (==), which allows you to check if two values are equal.
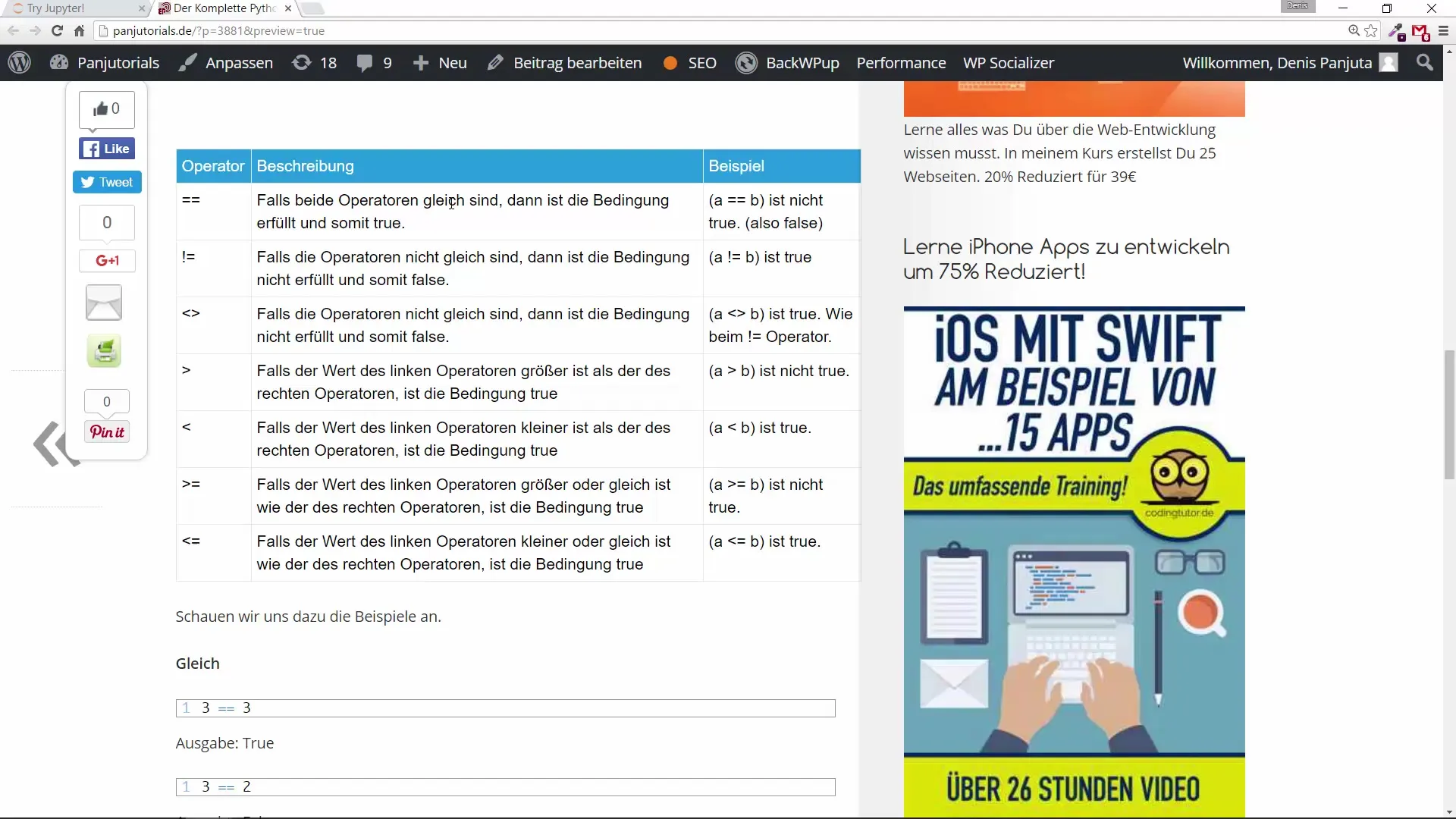
If you now have a variable A1 that equals 1, and another variable B that is also equal to 1, then the evaluation of A1 == B is of course true. However, if B has the value 2, the answer will be false.
There is also the inequality operator to determine whether two values have a different state. Here, querying 3!= 2 yields the result true because 3 and 2 are indeed unequal.
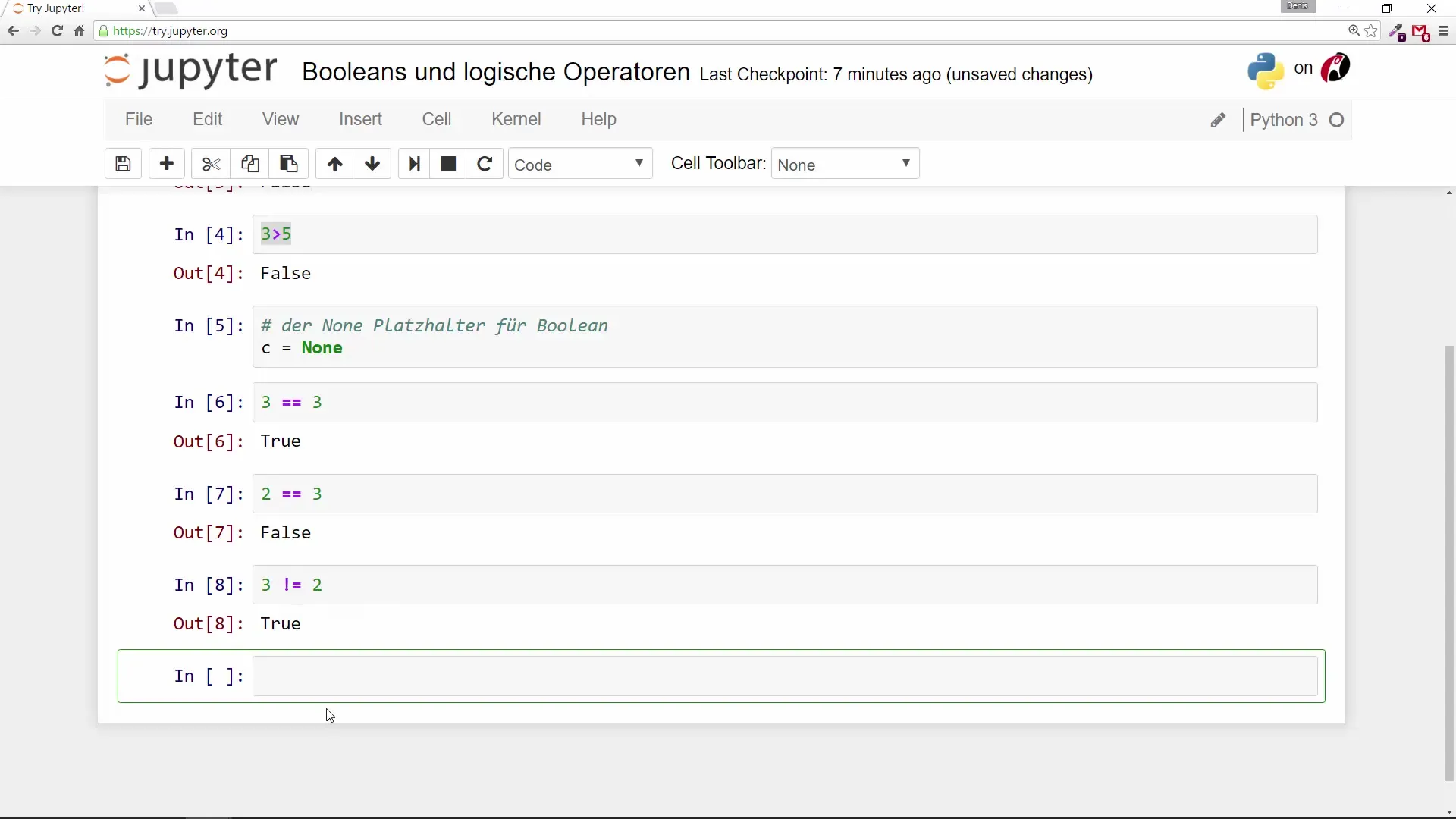
Operators like greater than or less than (> and <) also work in a straightforward manner; for example, querying 3 > 1 returns true, while 3 > 5 shows the opposite. Thus, these operators provide you with a variety of ways to classify values.
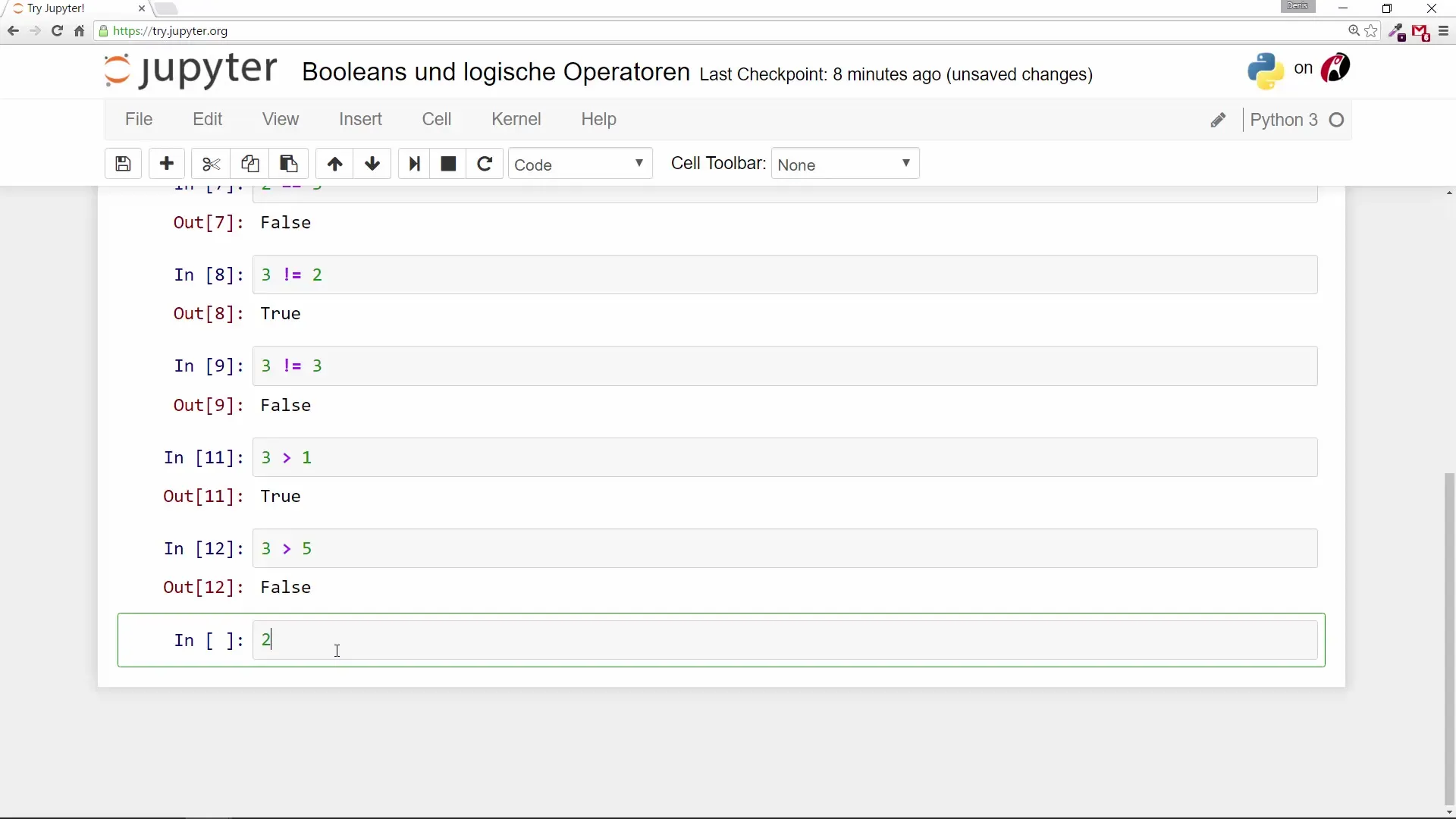
Comparison operators like >= and <= are also useful for determining whether two values are equal or at least in a certain relationship to each other. For example: 3 <= 3 yields true because both values are equal. The same applies to 4 >= 5, which returns false since 4 is less than 5.
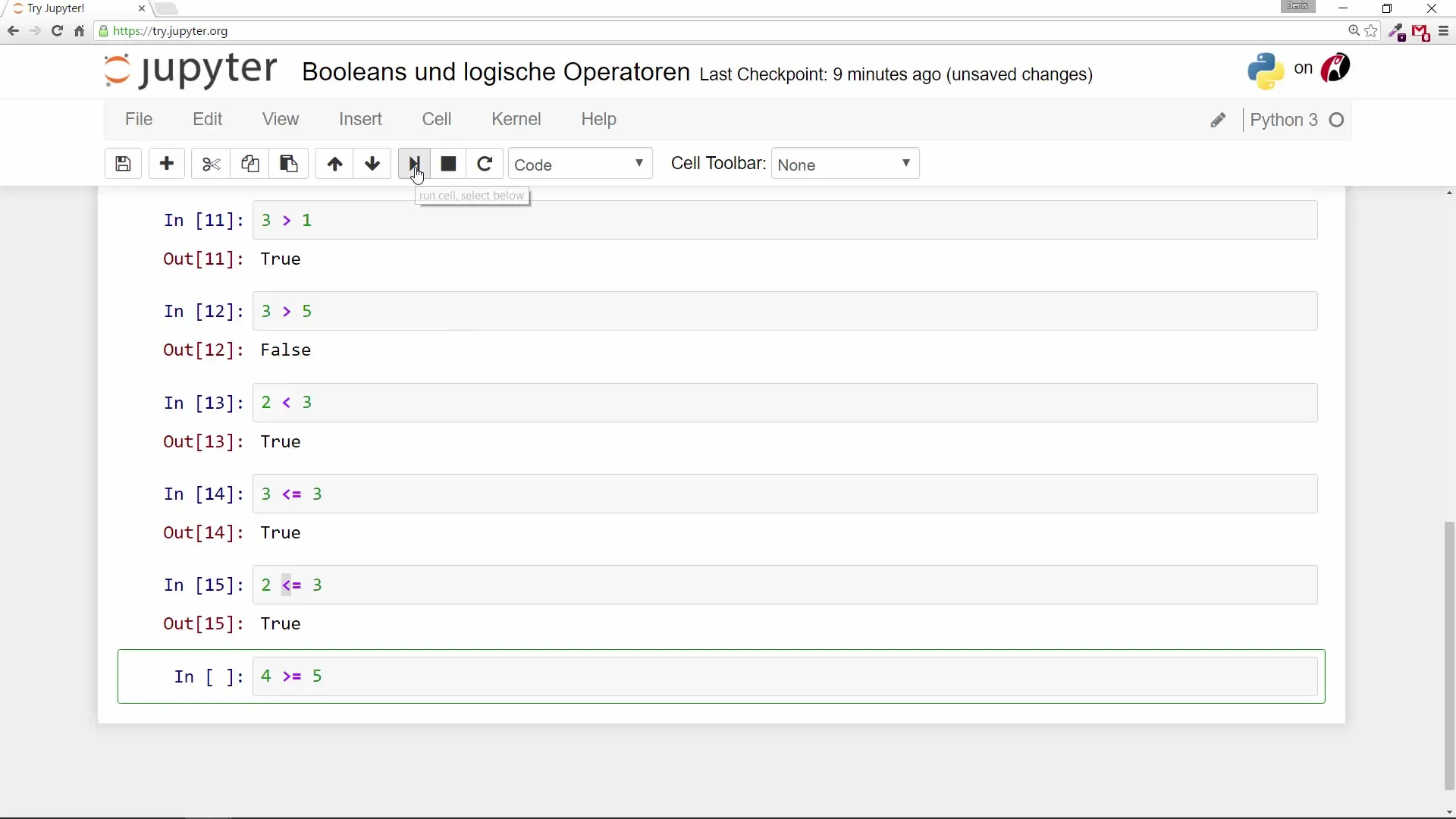
When you combine these different types of comparisons, you have the opportunity to control logical flows in your program and use logical operators for your needs.
Conclusion: Booleans and Logical Operators in Programming
In summary, it is very important that you understand the concept of Booleans in Python and how to apply the different logical operators. Booleans help store states within your applications, and the logical operators assist you in making comparisons and decisions. With these two foundations, you can effectively implement programming logic to create better software solutions. Move on to advanced programming in the next step by mastering conditions and decision structures.
Summary – Using Booleans and Logical Operators in Python Effectively
Frequently Asked Questions
What are Booleans?Booleans are variables that can only take the values true, false, or none.
How do I use logical operators in Python?Logical operators help you make comparisons between values to produce truth values.
What is the difference between == and!=?== checks if two values are equal, while!= checks if they are unequal.
When should I use the value none?none is used to indicate that a variable has no value.
How do Booleans influence decisions in programs?Booleans determine whether a condition is met, which governs the execution or omission of certain code sections.