The ability to implement mathematical concepts like the greatest common divisor (GCD) with Python is a valuable skill for any programmer. In this guide, you will learn how to write a program using While loops that calculates the GCD of two numbers. You will be guided step by step through the process, so that by the end you can independently create a working program.
Key Insights
- The greatest common divisor (GCD) can be determined by repeatedly applying the modulo operator.
- While loops are an effective means of performing repeated calculations as long as a certain condition is met.
Step-by-Step Guide
Step 1: Preparing the Variables
First, you define two numbers as variables that you want to work with. These values will be used in the subsequent calculations.
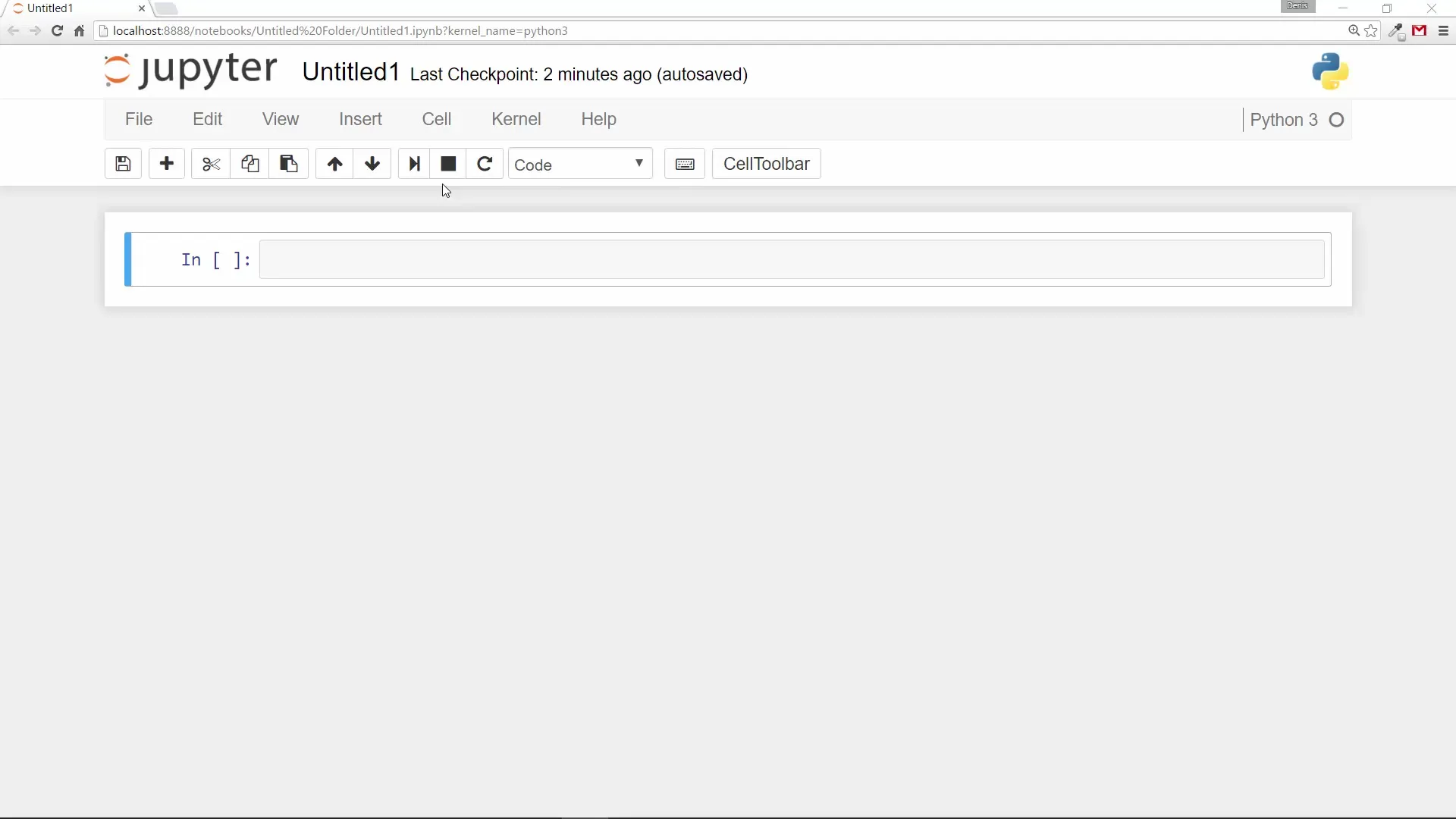
Step 2: Initializing the GCD
You initially set the value of the gcd variable to the second number. This is the starting point for the calculation.
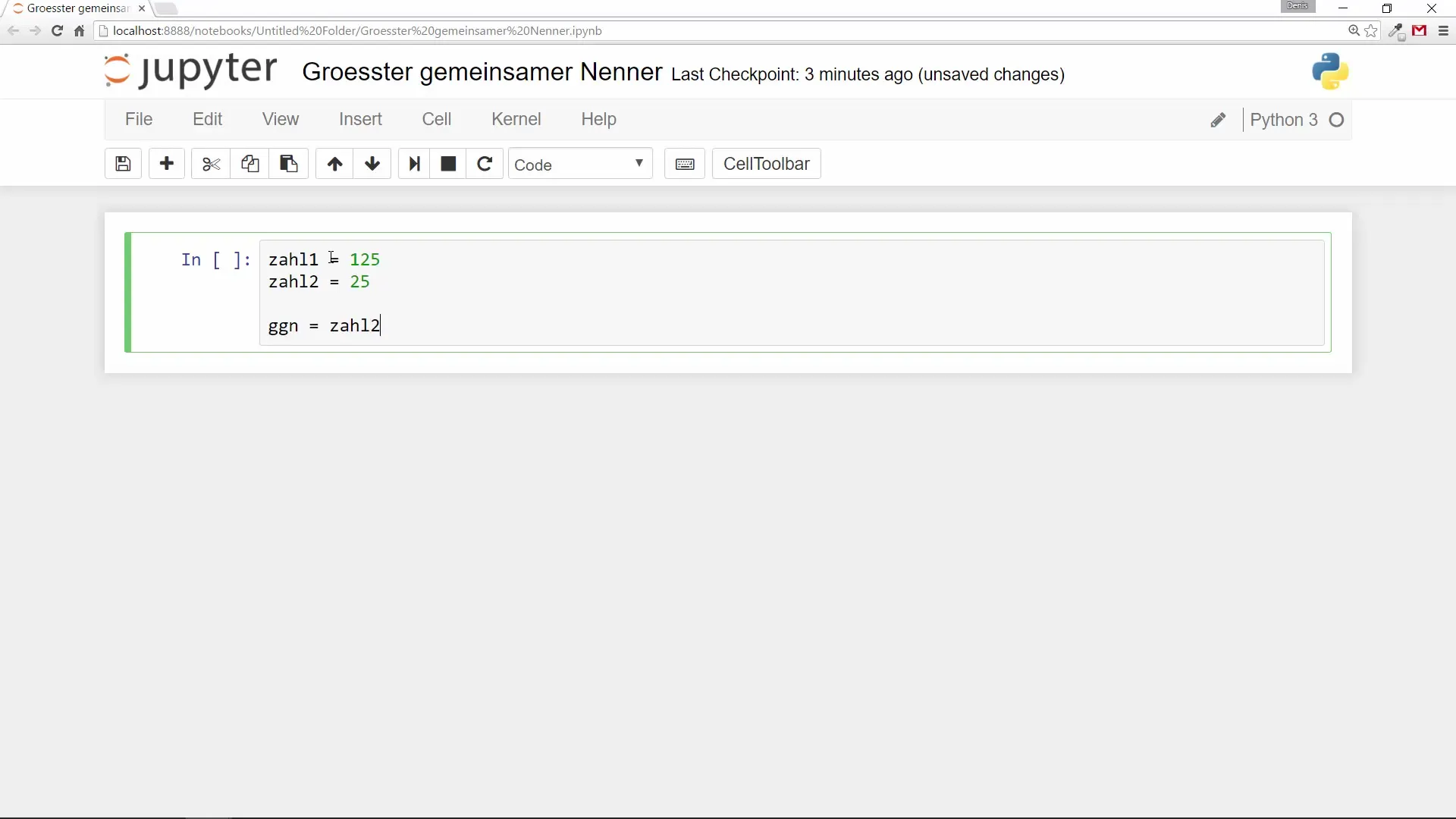
Step 3: Creating the While Loop
Now you create a While loop that continues as long as the first number is greater than zero. The main logic for calculating the GCD occurs within this loop.
Step 4: Applying the Modulo Operator
Within the loop, you replace the value of the first number with the current value of the second number and the second number with the remainder of the first number divided by the second number (modulo calculation).
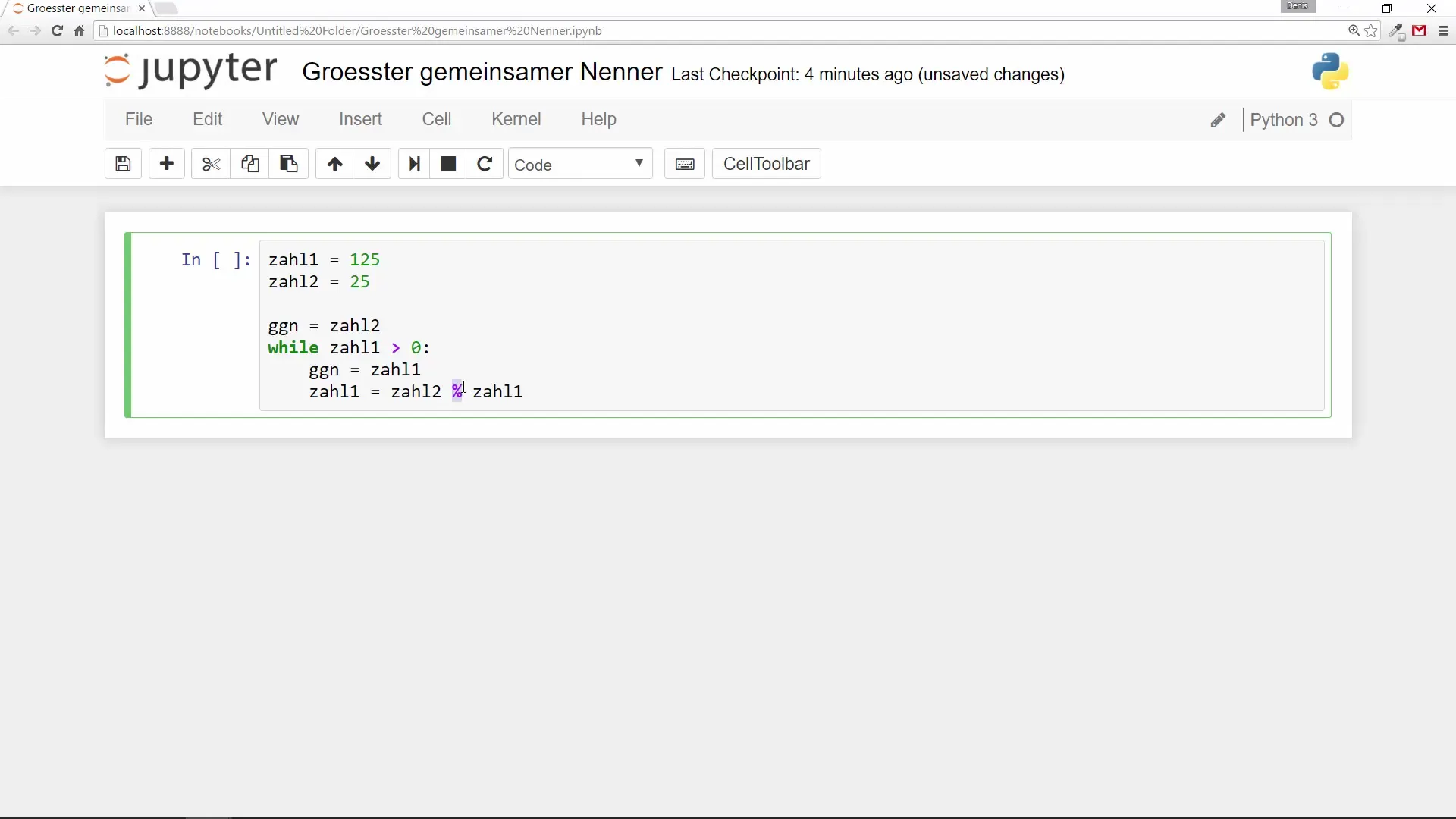
Step 5: Outputting Intermediate Values
It's useful to output the values of both numbers in each loop iteration. This way, you can track how the values change.
Step 6: Calculating the Result
When the loop ends, you output the result with a print command that shows you what the greatest common divisor is. The gcd variable is used here to store the value of the greatest common divisor.
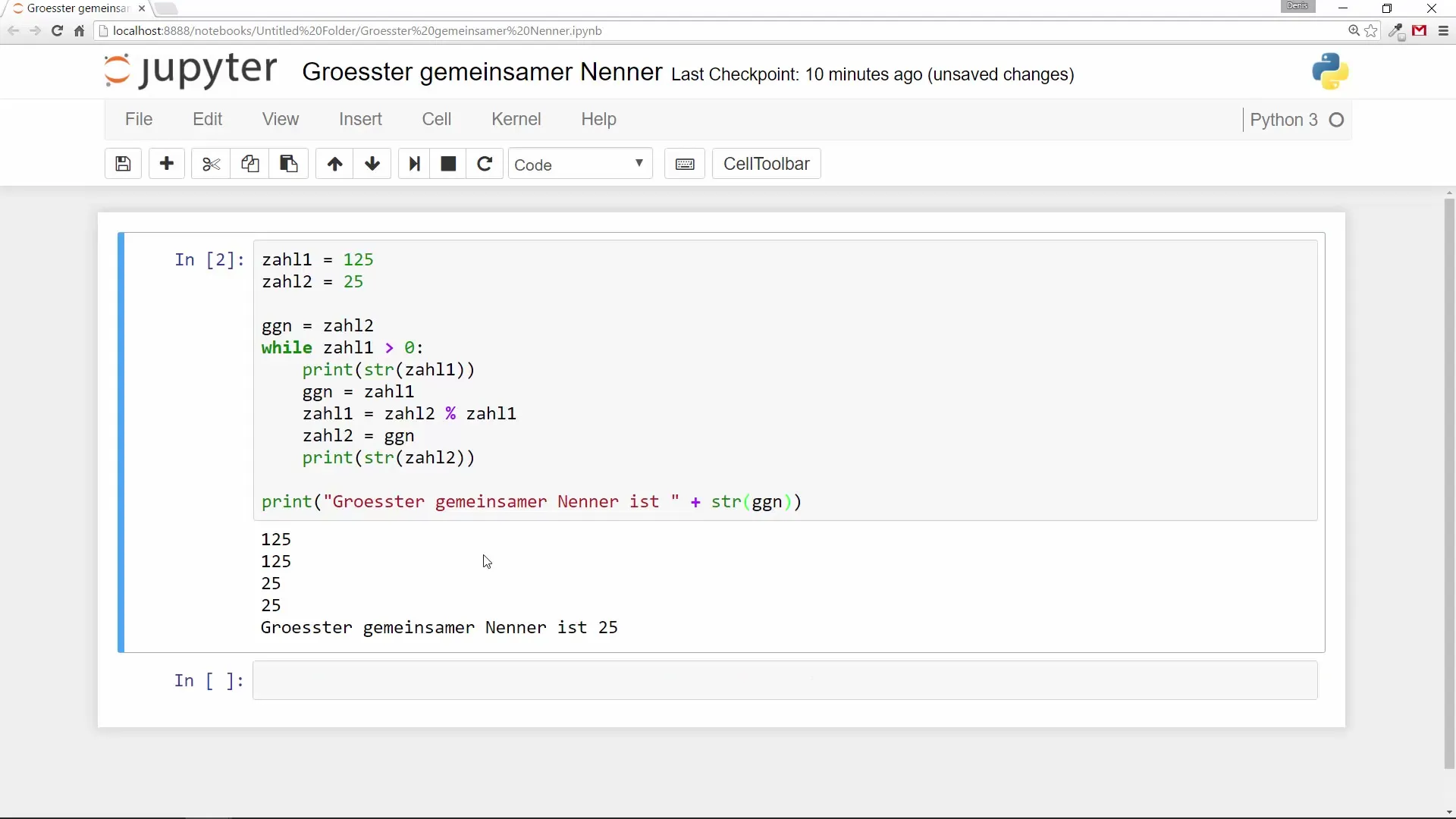
Step 7: Testing with Different Values
Test your program with different numbers to ensure that it works correctly. You can start with smaller numbers and then move on to larger and more complex numbers. Check the outputs for your different test cases.
Step 8: Analyzing the Program
Why does the program work? The logical structure you have developed is designed to find smaller numbers sequentially that meet the conditions for the greatest common divisor until this exact number is found.
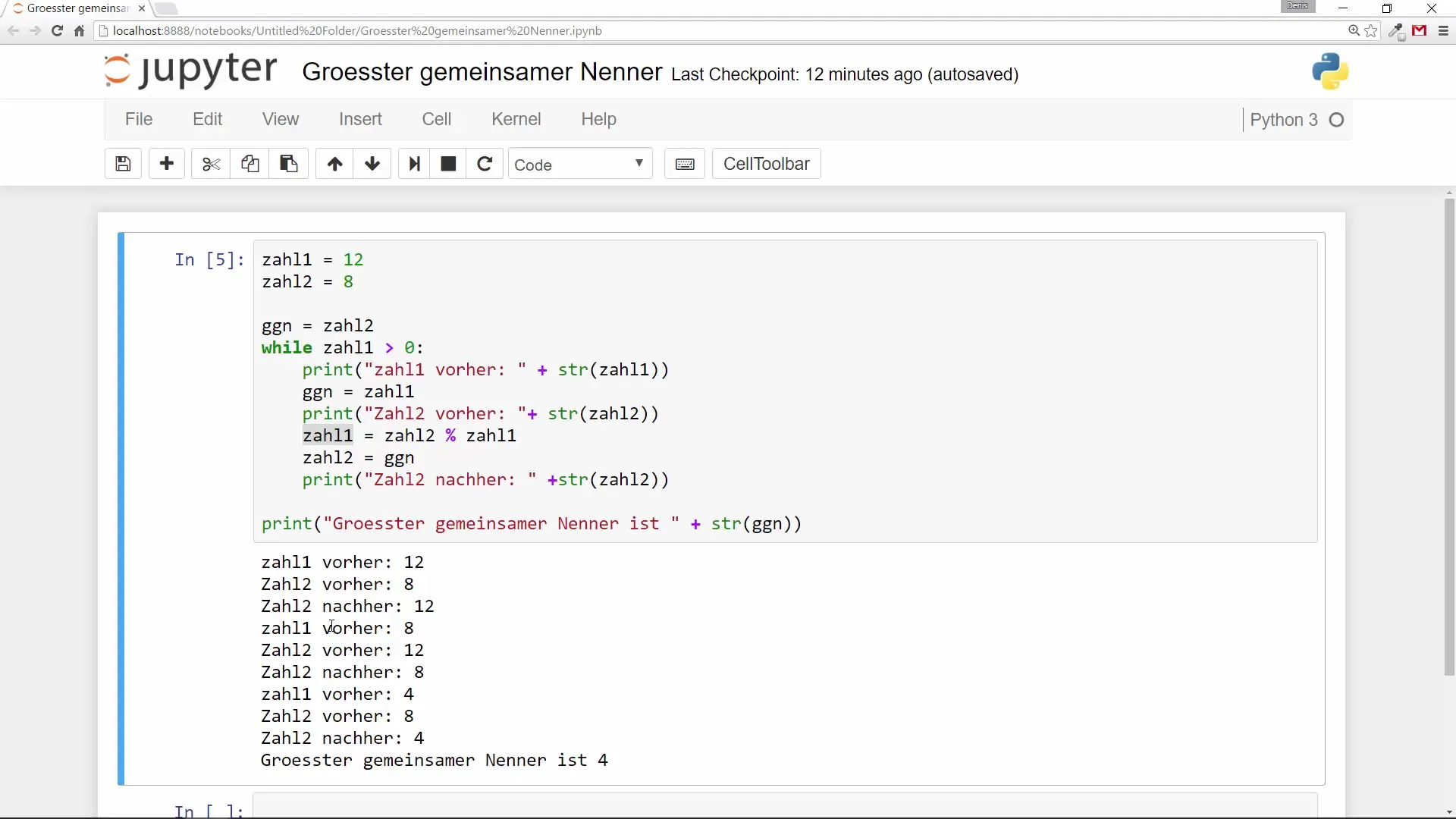
Summary – Greatest Common Divisor with Python: A Step-by-Step Guide
In this guide, you have learned how to calculate the greatest common divisor of two numbers with Python and a While loop. Using the modulo operator allows you to determine the GCD incrementally while iterating through the loop.
Frequently Asked Questions
What is the greatest common divisor?The greatest common divisor (GCD) is the largest number that can divide both numbers without a remainder.
How does the modulo operator work?The modulo operator returns the remainder of a division.
What is a While loop?A While loop executes a block of code as long as a certain condition is met.
How can I test my program?Change the values of the two numbers and call the program again to determine the GCD for different inputs.
Could I also use the program for more than two numbers?Technically, yes, but it would require different logic to handle multiple numbers.