If you work with Python, you will often encounter lists. Lists are flexible and versatile data types that allow you to store multiple items in a single variable. This guide will introduce you to the various methods you can use with lists. It’s important to know the common functions to enhance your programming skills and develop effective, clever solutions.
Main Insights
- Lists can be modified and edited using various methods.
- You can add, delete, modify, and analyze items in lists.
- Python provides a variety of functions that significantly ease working with lists.
Step-by-Step Guide
Creating a Simple List
First, you can create a simple list. For example, your list can contain the letters A, B, and C. These values are defined in Python as follows:
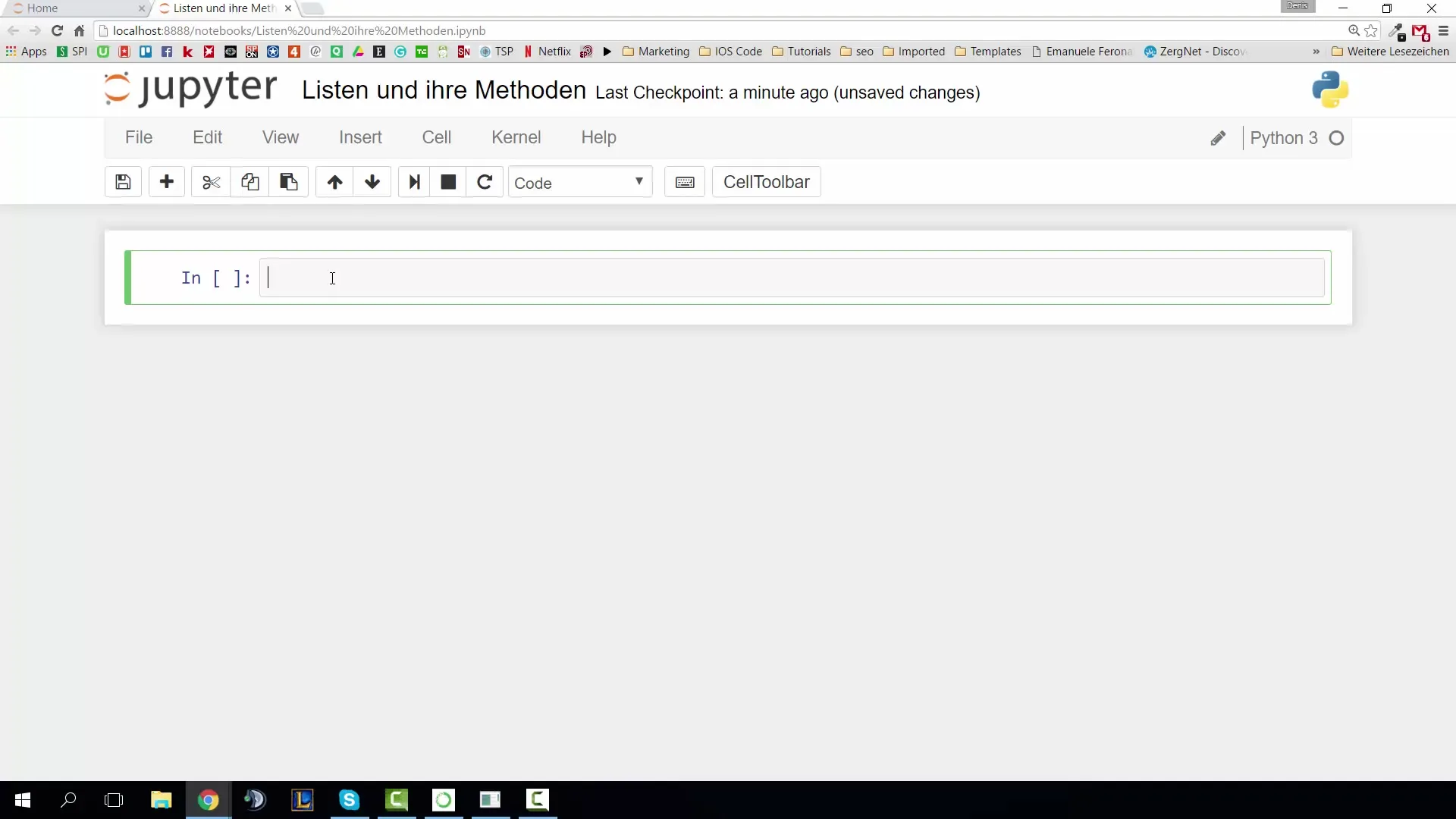
Adding Elements with the append() Method
To add a new value to a list, you can use the append() method. This appends an element to the end of the list. When you print the list, you will see that the new value has been added correctly.
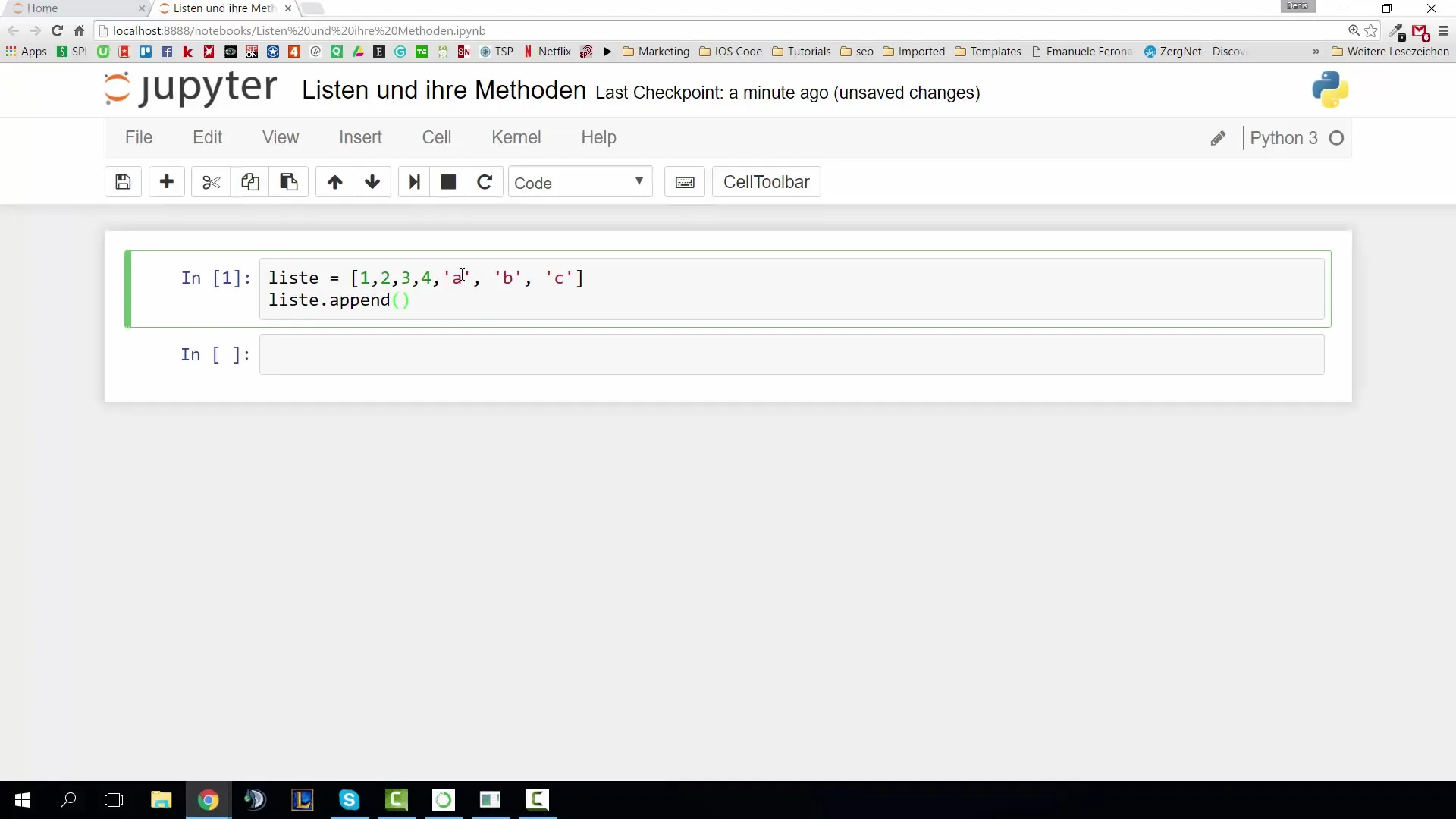
Combining Lists with extend()
If you want to combine two lists, you can use the extend() method. This function extends the first list with the elements of the second. Note that the first list will be overwritten and no new list is created.
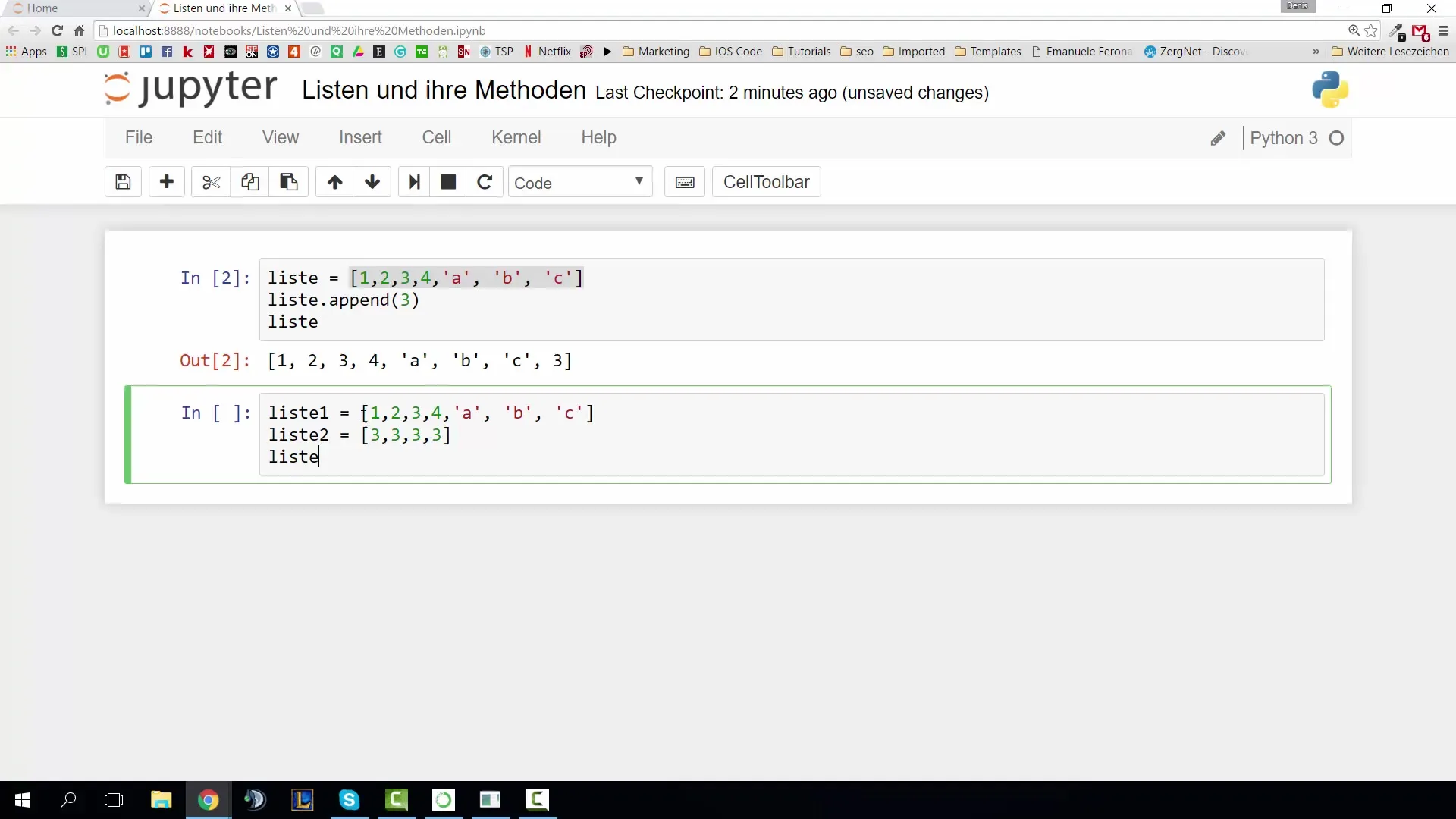
Inserting Elements with insert()
If you want to insert an element at a specific position in the list, the insert() method is ideal. You need to specify the exact position at which the element should be inserted. It’s important to note that the position does not correspond to the index.
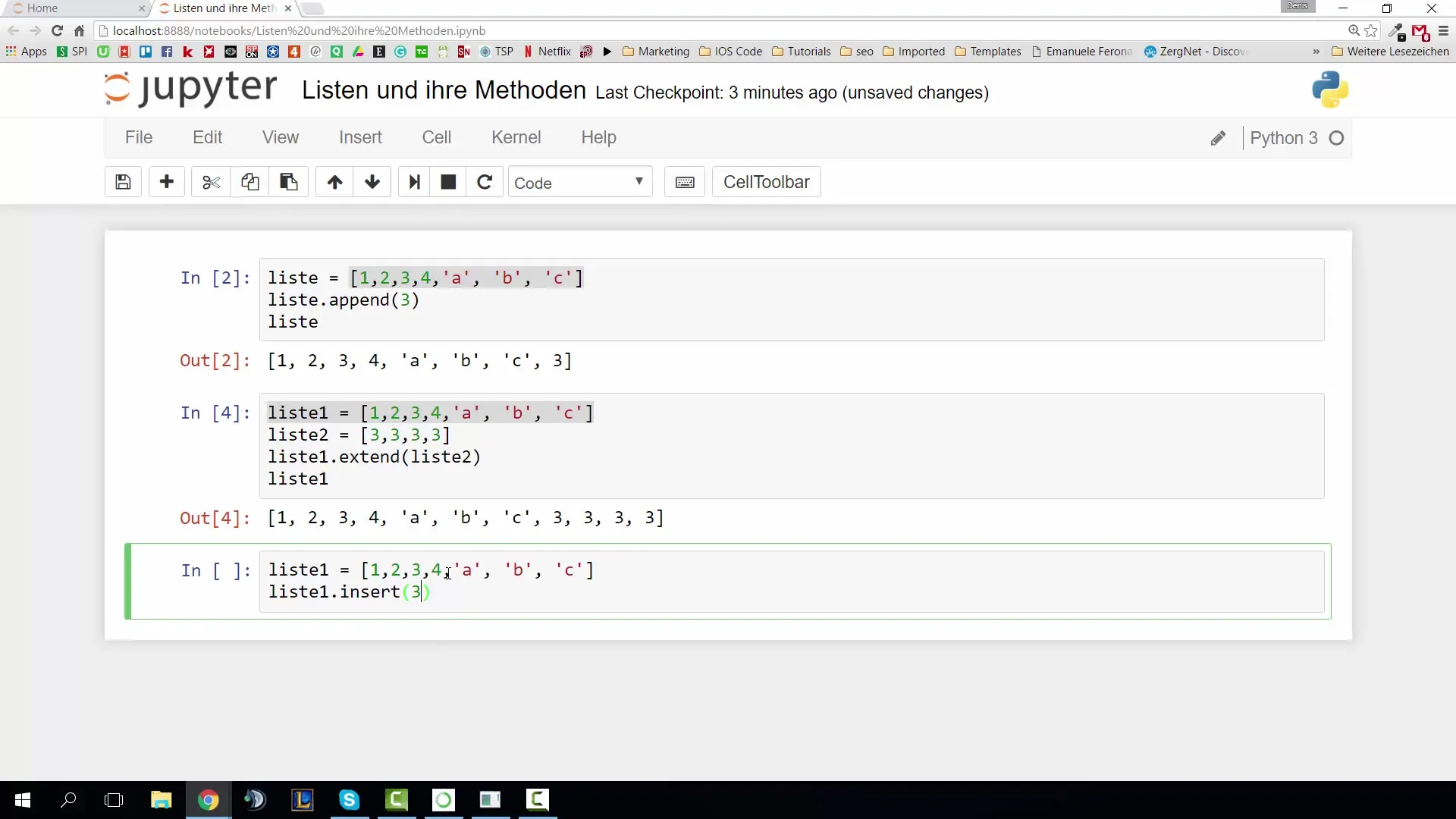
Removing Elements with remove()
To remove a specific element from a list, you use the remove() method. This deletes the first matching entry. If you try to remove an element that does not exist, an error will be raised.
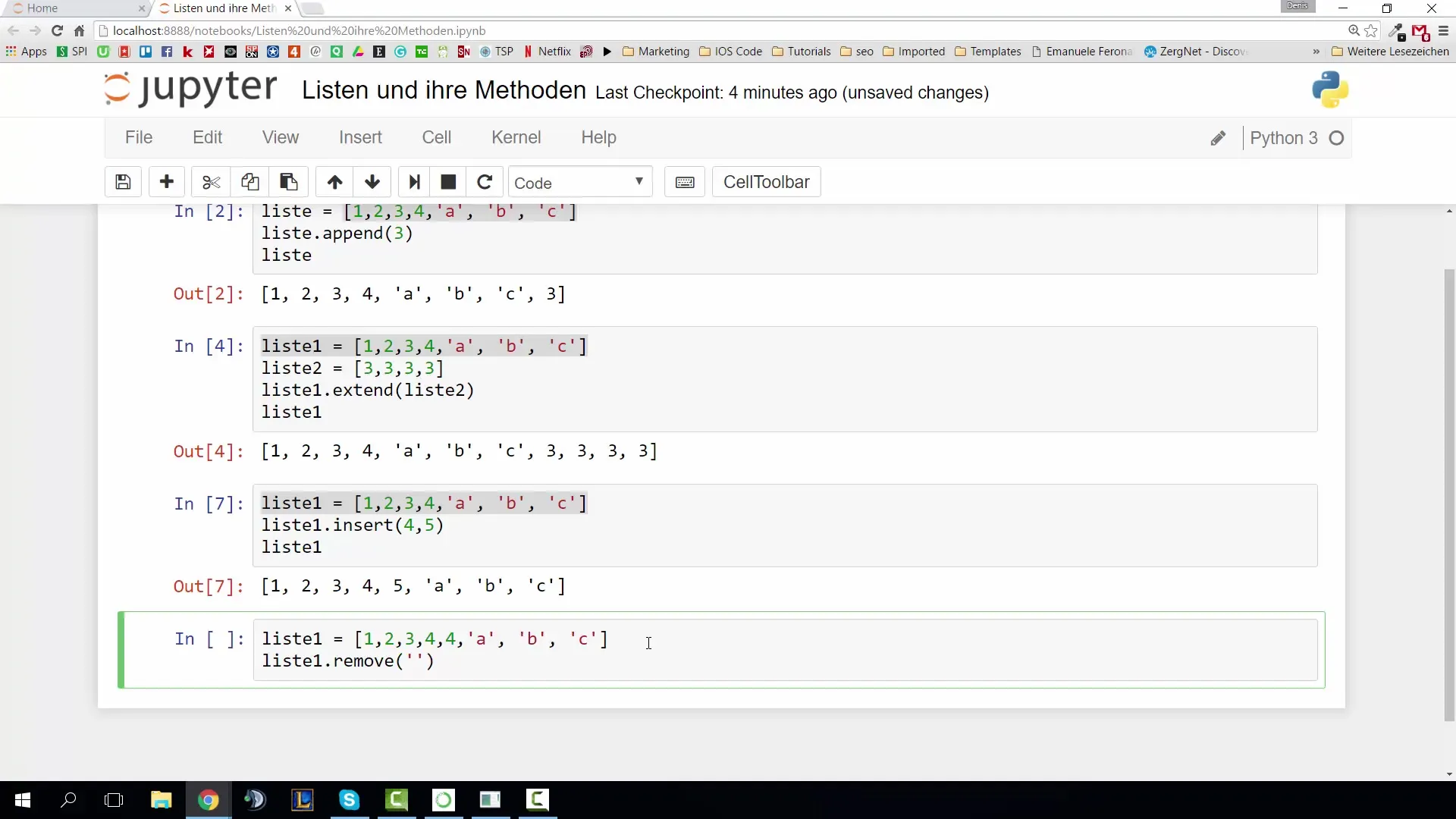
Removing an Element at a Specific Index with pop()
If you want to remove an element at a specific index, you can use the pop() method. This not only removes the element but also returns it.
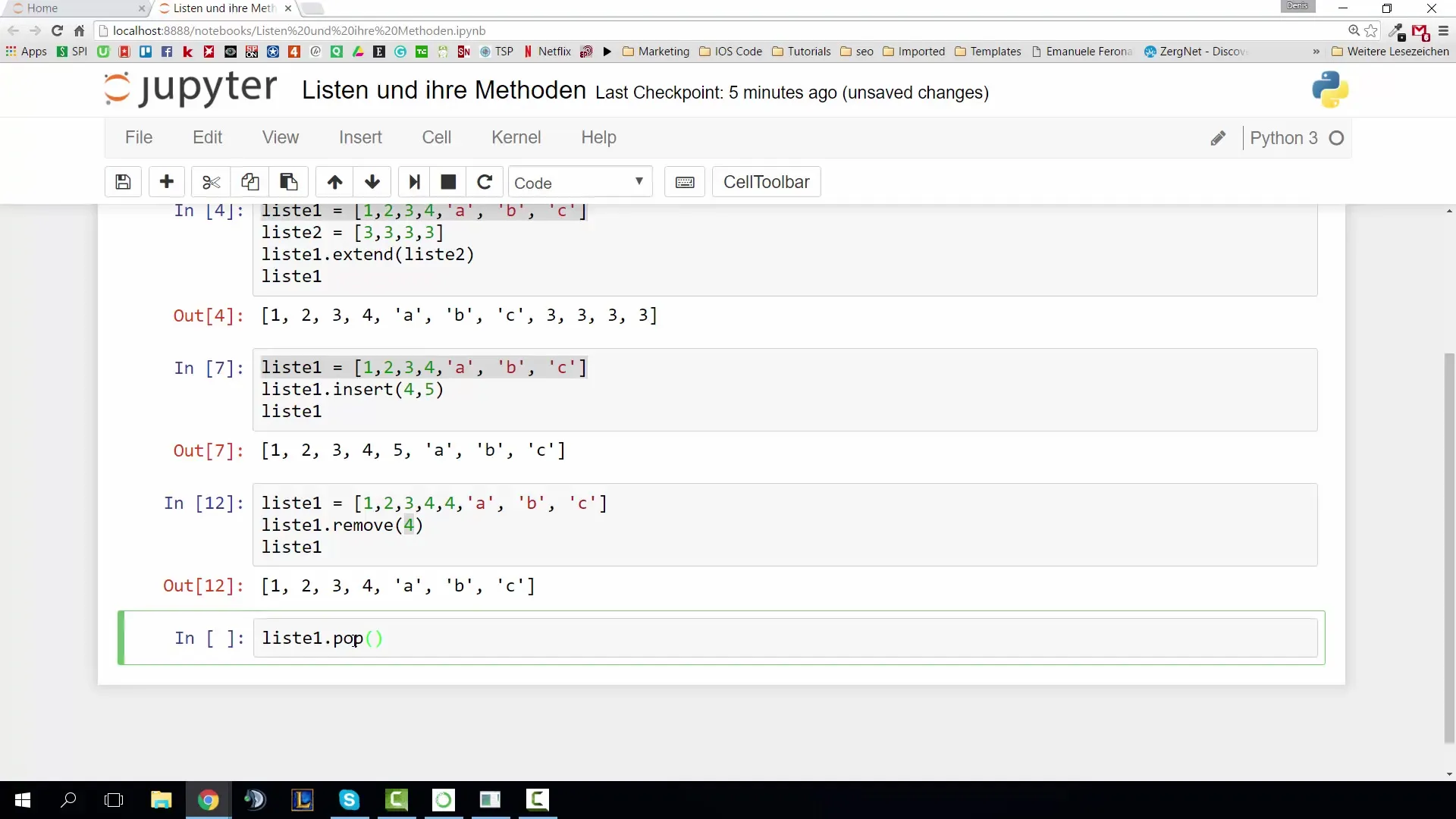
Finding the Index of a Value with index()
With the index() method, you can find out at which position a specific value is located in the list. This method returns the index of the first occurrence.
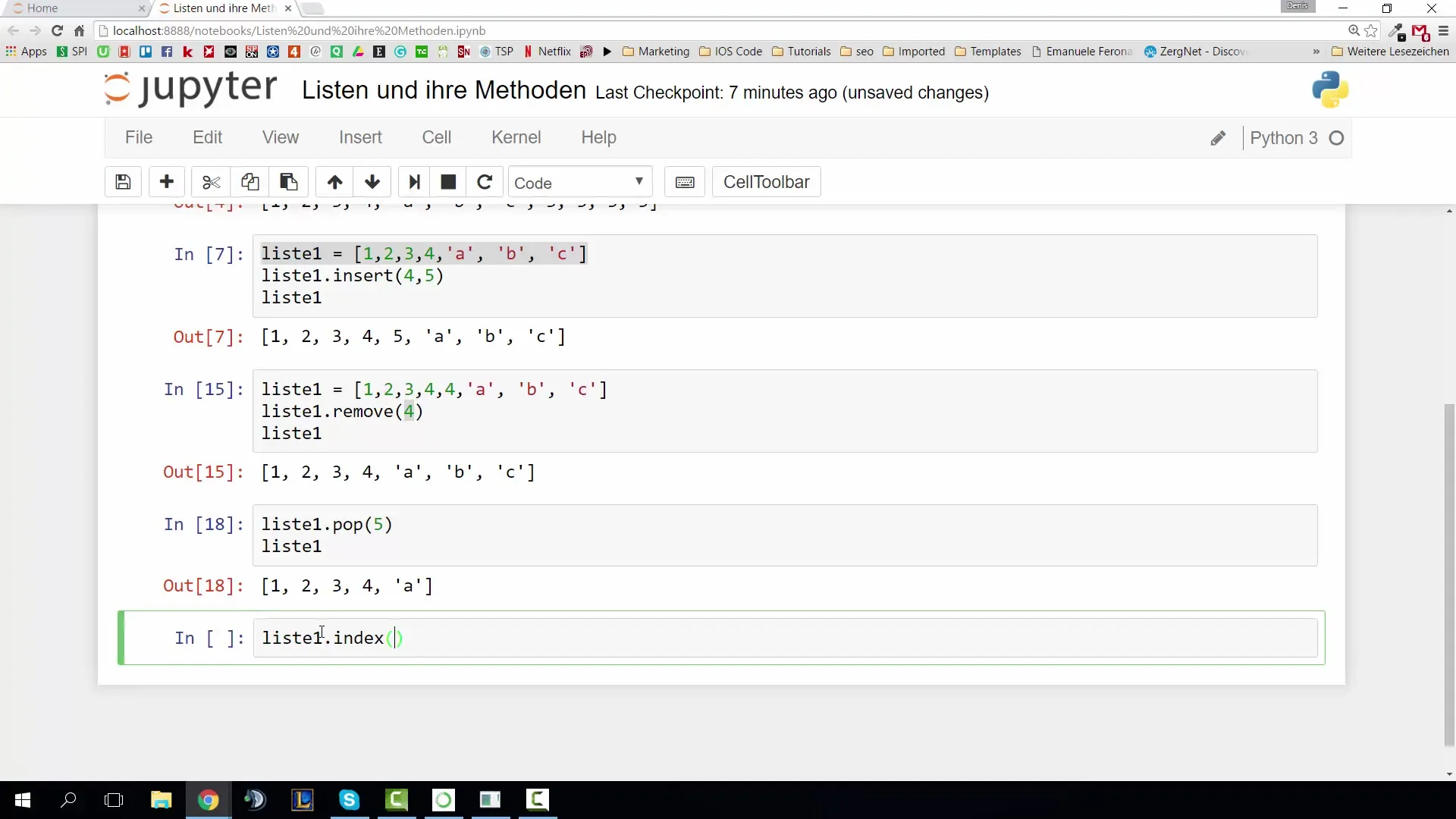
Counting Elements with count()
If you want to know how often a specific value occurs in a list, the count() method is useful. It returns the number of occurrences of the specified value.
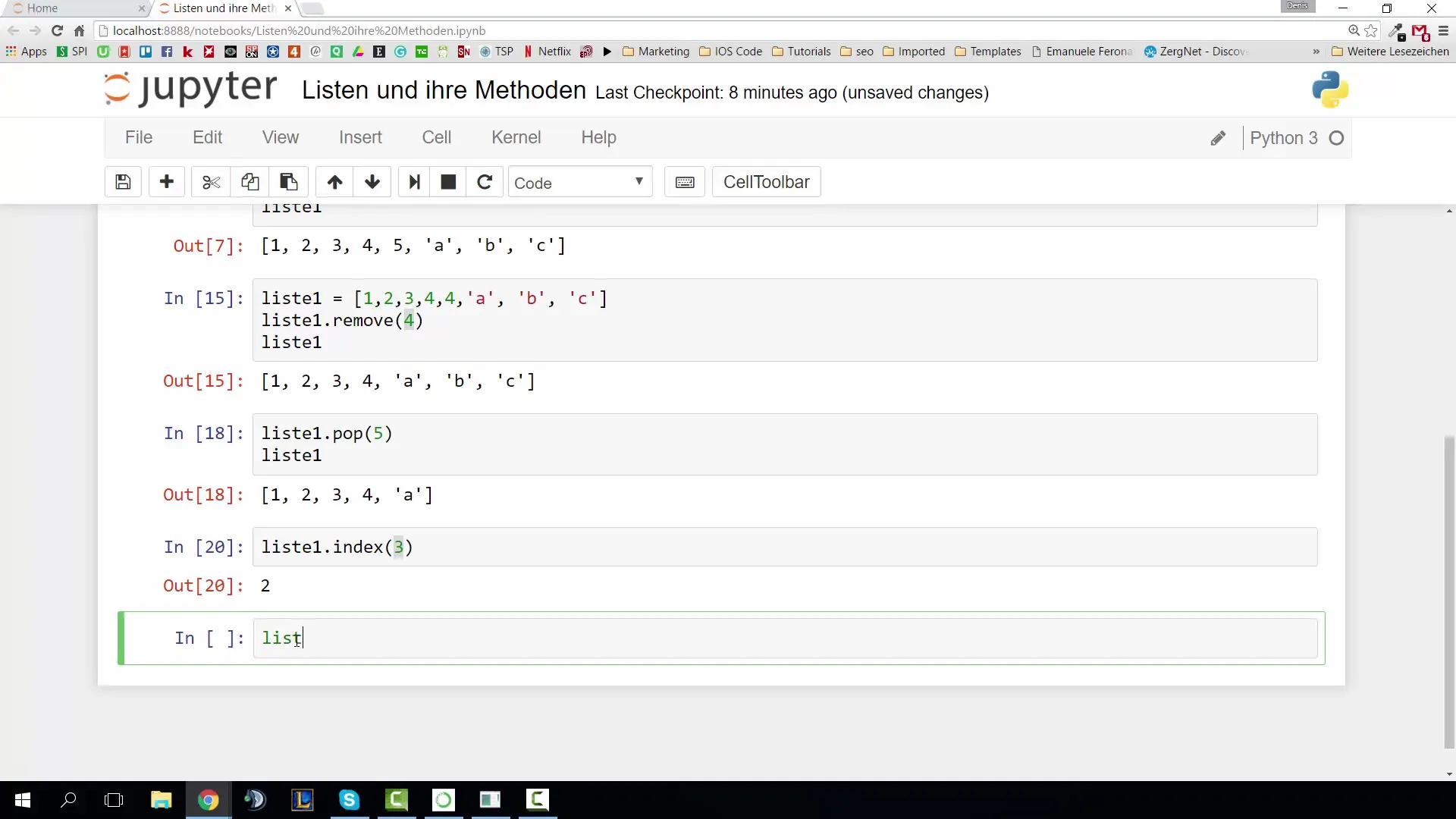
Sorting Lists with sort()
You can easily sort lists by using the sort() method. However, it is important that all elements in the list are of the same data type; otherwise, an error will occur.
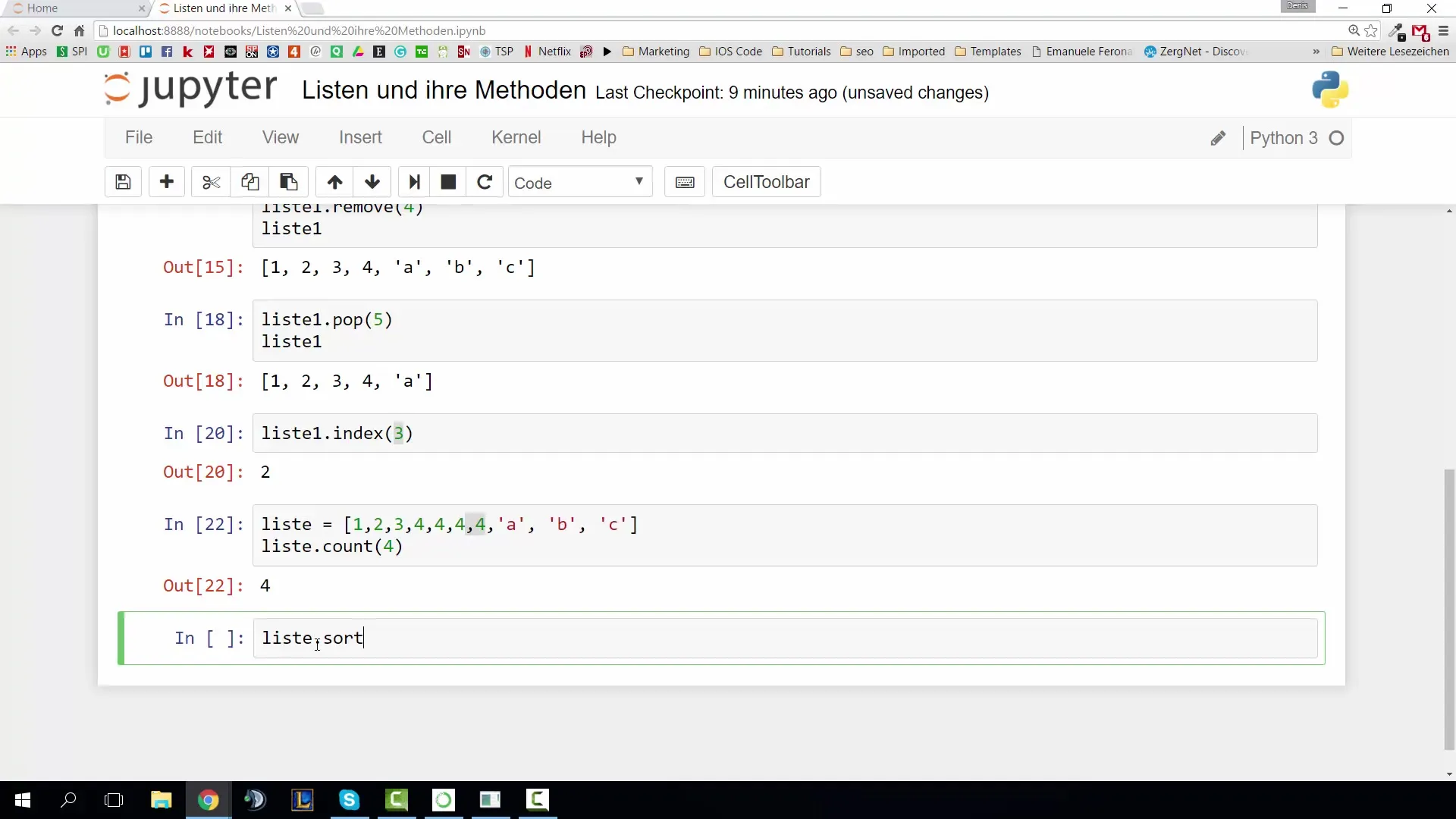
Reversing the Order with reverse()
To reverse the order of elements in a list, you can use the reverse() method. This causes the first element to become the last and the last to become the first.
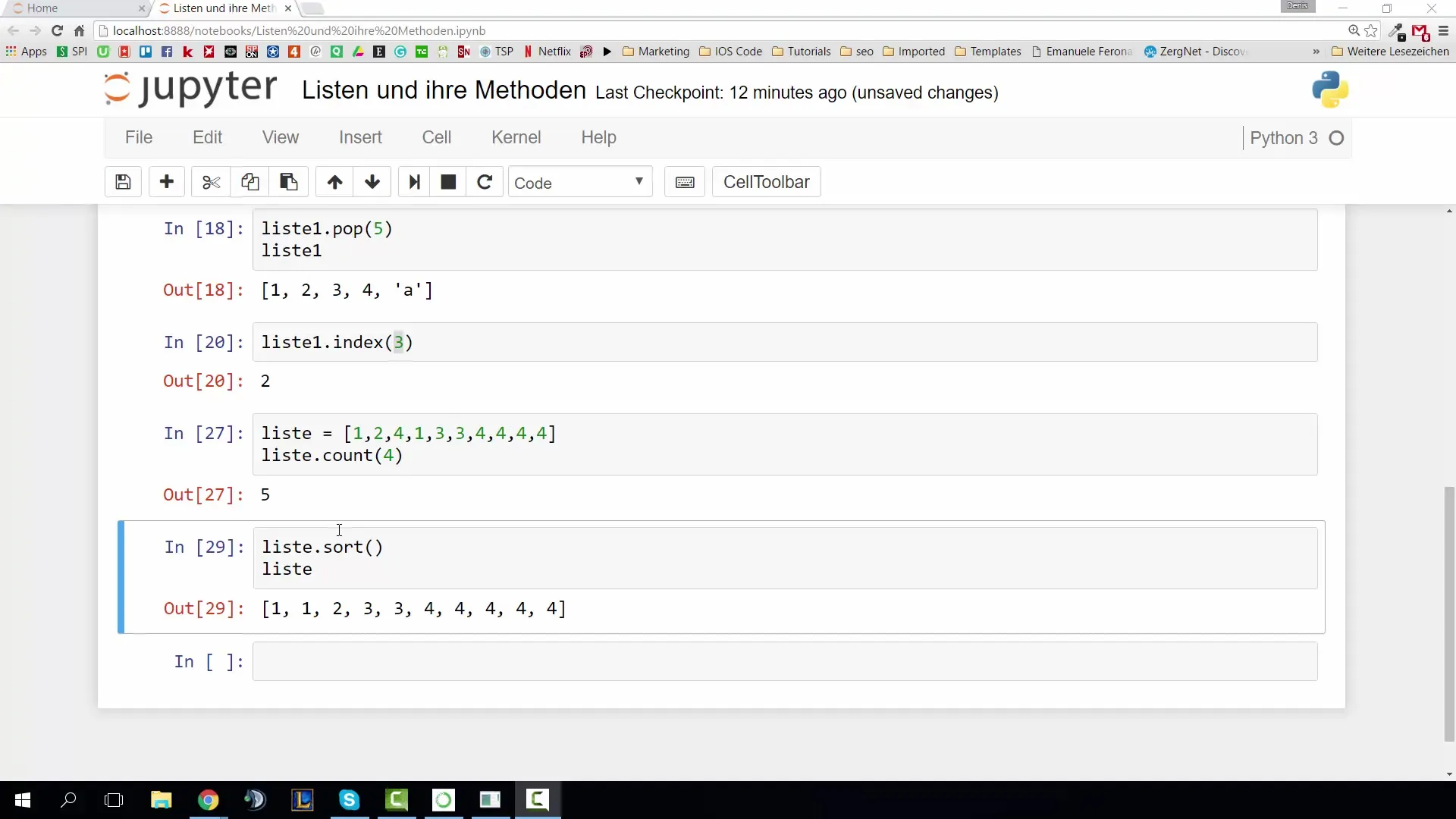
Summary – Python Lists and Their Methods
In this guide, you have learned how to work with Python lists, including adding, removing, and analyzing elements. Understanding these methods is crucial for developing efficient and effective programs.
Frequently Asked Questions
What are lists in Python?Lists are data types that allow you to store multiple values in a single variable.
How can I add an element to a list?You can use the append() method to add an element to the end of a list.
What does the extend() method do?The extend() method is used to append the elements of a second list to a first list.
How can I insert an element at a specific position?Use the insert() method and specify the desired position.
How can I remove an element from a list?Use the remove() method to delete a specific element.