Variables are the heart of every program in Java. They allow you to temporarily store and process data. Once you understand the basics of variables, you lay the foundation for more complex programming. In this tutorial, you will learn about the different data types, the significance of memory requirements in RAM, how to declare variables, and how to assign values to them.
Key Insights
- Variables are volatile memory areas in RAM.
- The data type determines the required memory space.
- The declaration and value assignment of variables are central components of programming.
Step-by-Step Guide
1. Introduction to Variables
At the beginning, it is important to understand what variables are. Variables serve as storage locations in the memory that can hold volatile data. Unlike permanent storage on hard drives, this data is temporary and exists only during program execution. For example, if you want to store a user input like a number, this is done in a variable.
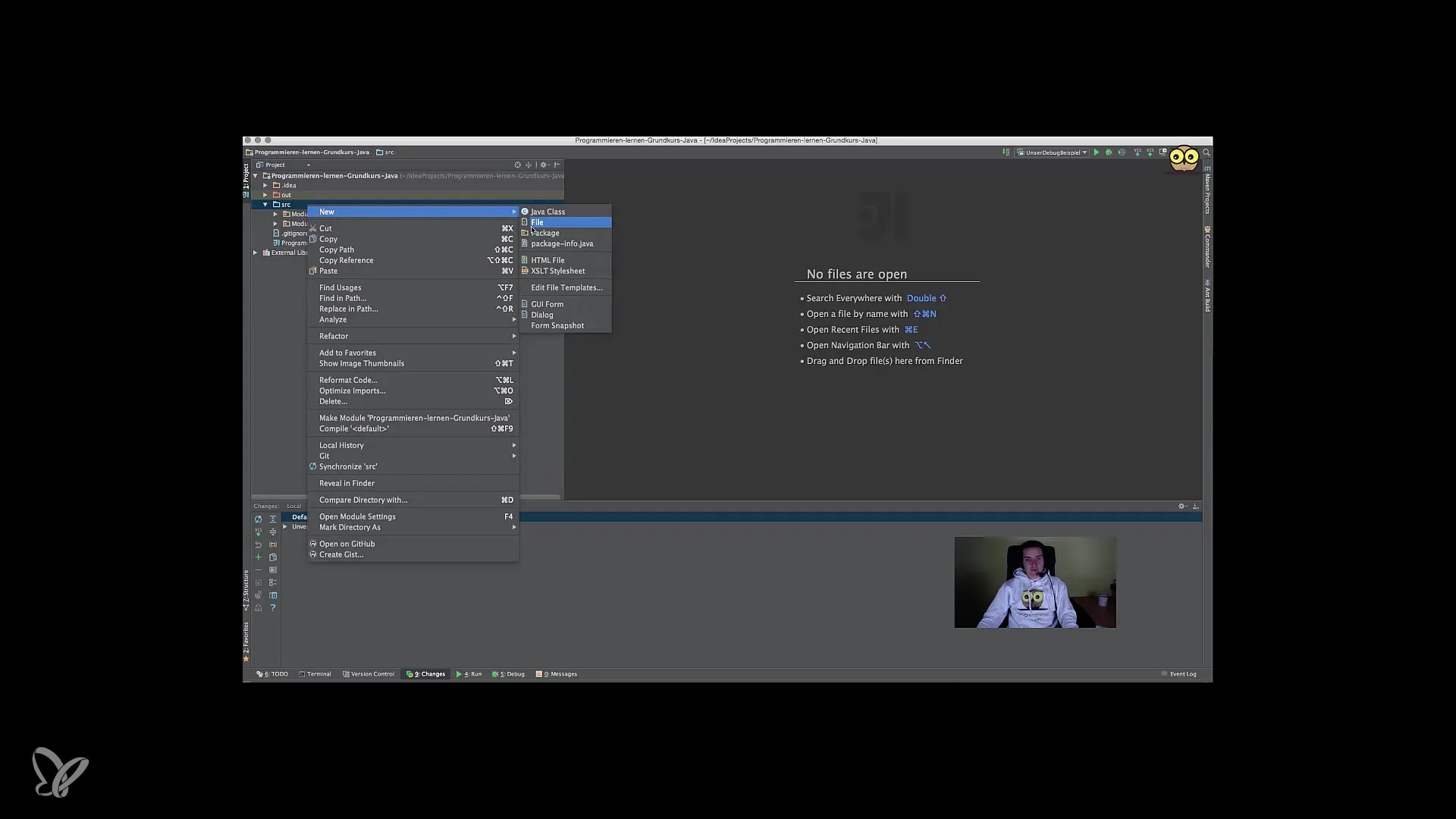
2. Creating a New Module
To start programming, you create a new package and a class. For your first example, you can create a package named "Module4" and a subname "Video1". This structure helps you keep your projects organized and find corresponding modules easily.
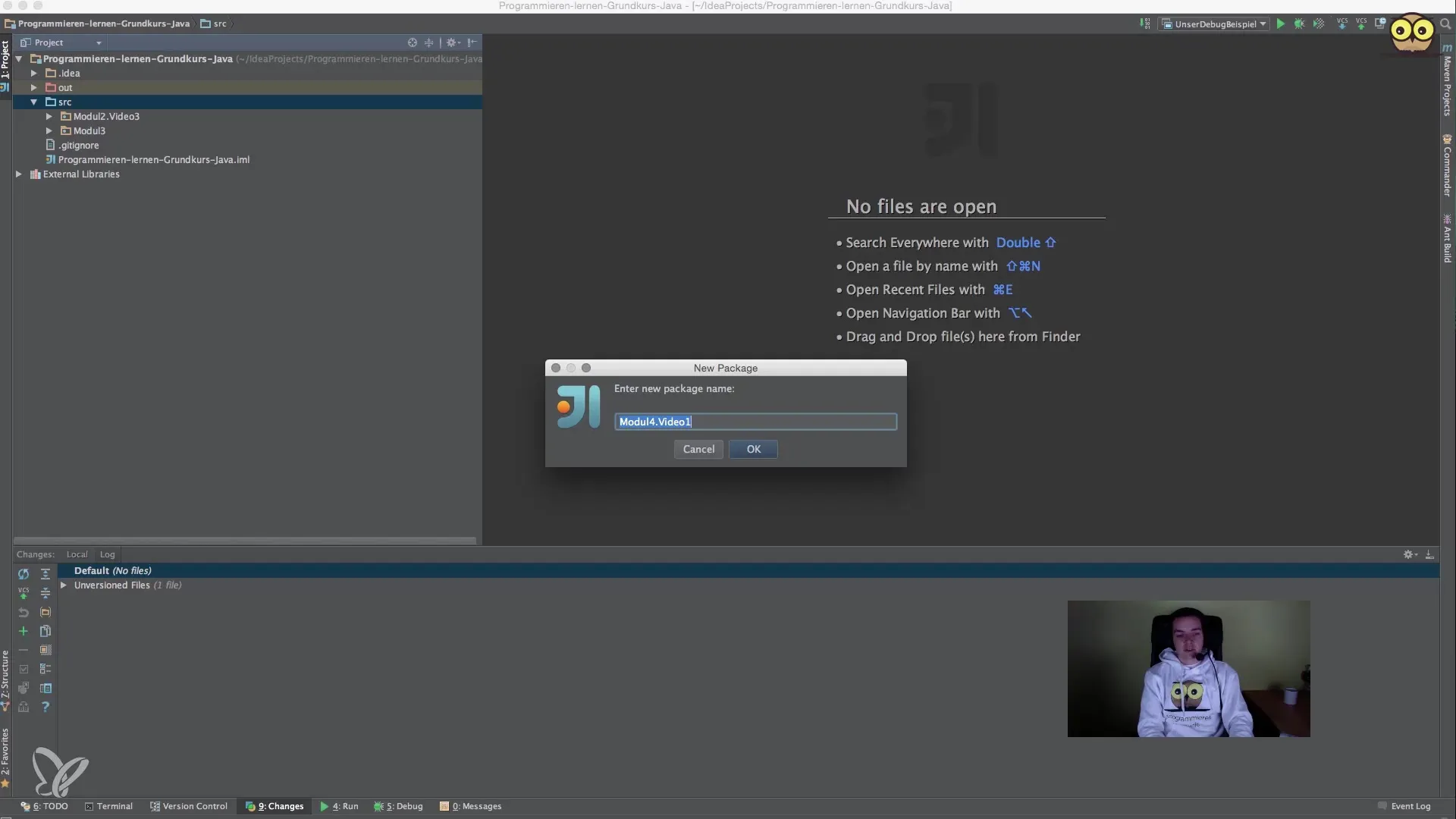
3. Declaring a Variable
Once you have created your package, the next step is declaring a variable. A variable is defined by its data type and a variable name.
With this, you inform Java that a new integer variable is being created, but the memory space is only assigned when you set a value.
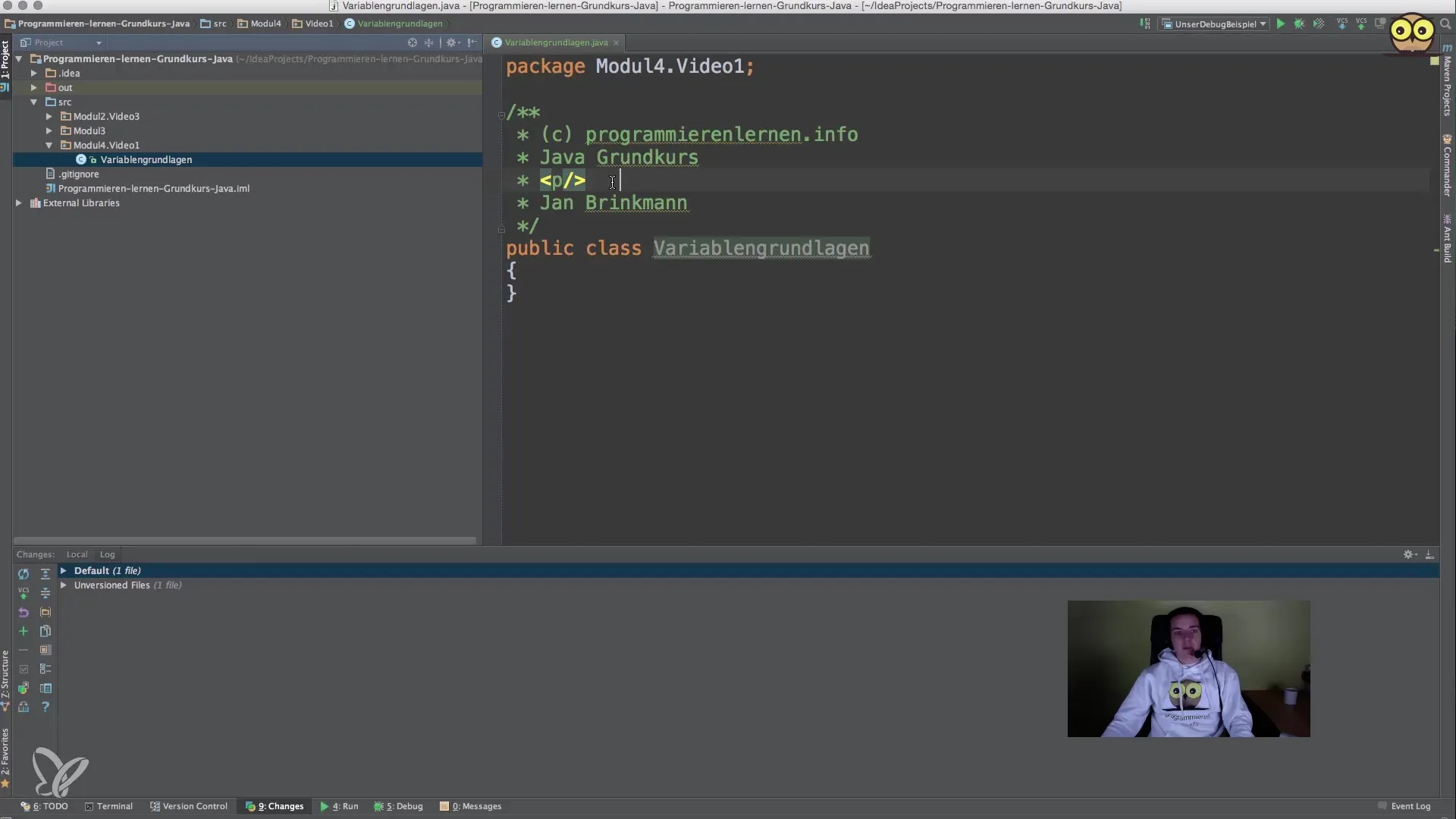
4. Assigning a Value to a Variable
After declaring a variable, you can assign it a value. This is done using the simple equals sign.
In this step, you have successfully declared the variable and assigned it a value.
5. The Importance of Data Types
The data type you choose for a variable affects the amount of memory required. An integer requires more memory than a byte, meaning for smaller values, you should also use smaller data types. This is especially important if you want to save memory in larger programs.
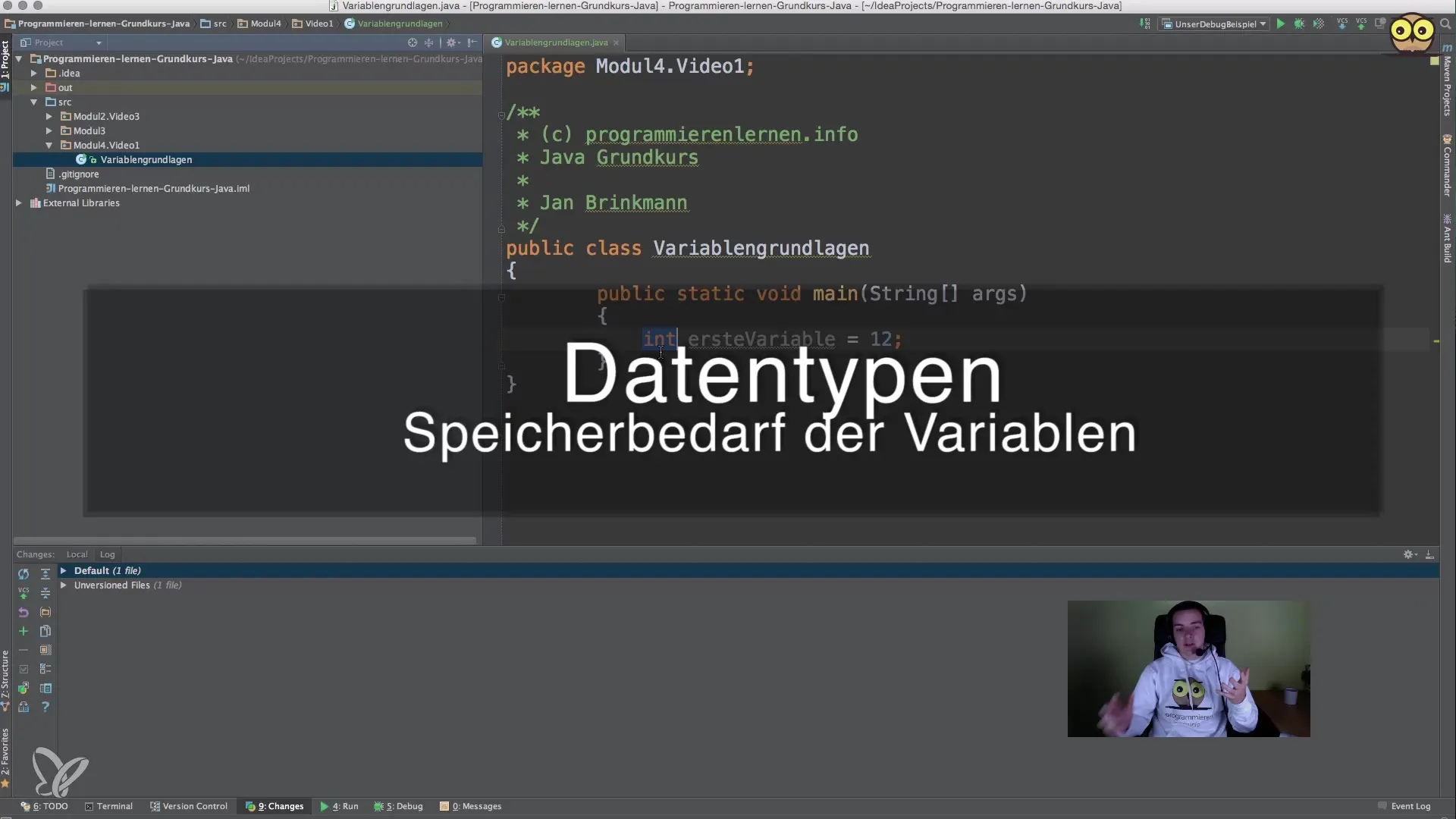
6. Differences in Data Types
Data types in Java are different and allow for various ranges of values. For example, integer values can be over 2 billion, while byte values can only go up to 127. For values that do not exceed these limits, it is more efficient to choose the appropriate data type.
7. Efficient Use of Memory
When writing programs that require many variables, it is advisable to pay attention to memory requirements. For example, if you only need values between 0 and 10, a byte is entirely sufficient. This way, you can minimize your memory usage and increase the efficiency of your programs.
8. Example of Simultaneous Declaration and Value Assignment
A commonly used method in Java is the simultaneous declaration and assignment of a variable.
This way, you have declared the variable "countdown" simply and effectively while simultaneously setting its value.
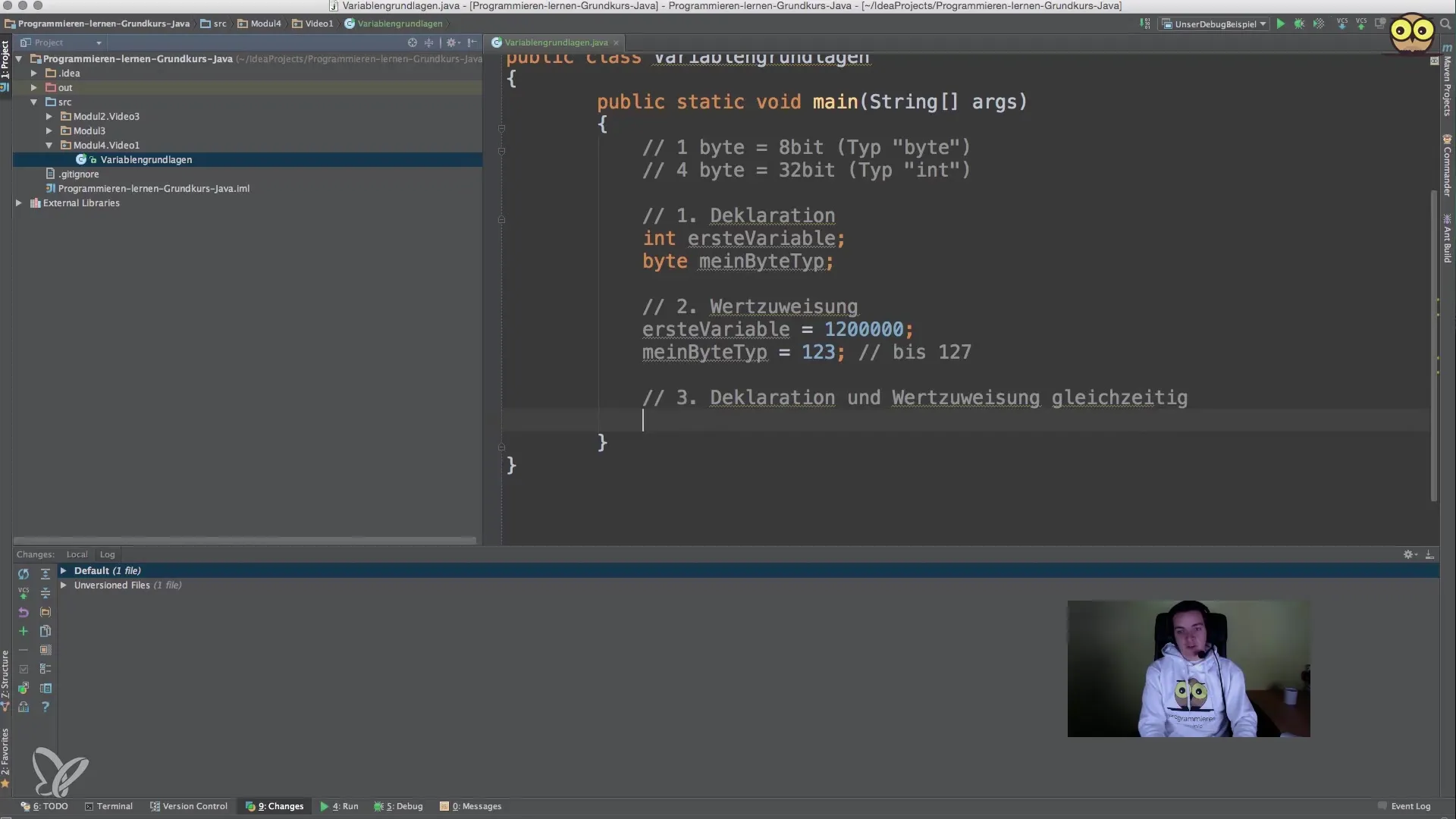
Summary – Variables in Java: Basics and Application
In this guide, you have learned the fundamental aspects of variables in Java. You have seen how to declare variables, assign values to them, and recognize the importance of the right data type. These skills are essential to deepen your programming knowledge.
Frequently Asked Questions
What are variables in Java?Variables are volatile memory areas in RAM where temporary data is stored.
How do I declare a variable?You declare a variable by writing the data type followed by the variable name.
Why are data types important?The data type determines the required memory space and the values that the variable can hold.
Could I always use integer variables?That would be inefficient, as smaller data types require less memory and are sufficient in many cases.
How do I perform simultaneous declaration and assignment?This is done using the syntax data type variableName = value;.