The for() loop is an important control tool in programming with Java. It provides a structured way to iterate through a collection of values or a specific number of iterations. In this guide, we will take a closer look at how the for() loop works, compare it with while()-loops, and also learn how nested loops function.
Key takeaways
- The for() loop is excellent when the number of iterations is known in advance.
- The loop counter is typically initialized, checked, and manipulated in a single expression in the for() loop.
- Nested for() loops allow for complex iterations through arrays and multidimensional data structures.
Step-by-Step Guide
1. The basic structure of a for() loop
The for() loop has a clear structure that differs from the while() loop. It consists of an initialization statement, a condition, and an increment statement.
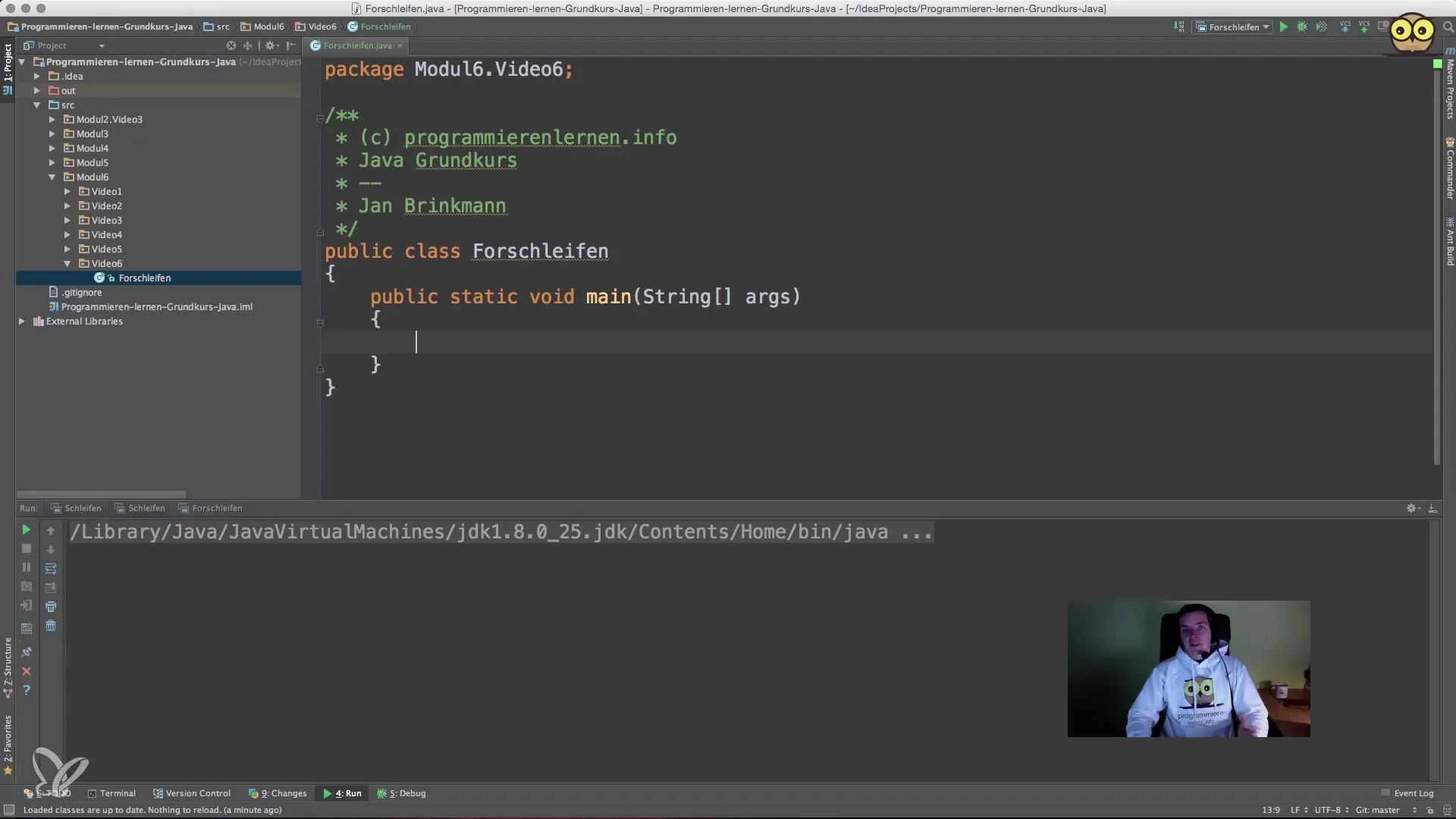
This structure simplifies reading and understanding the code, as all parts of the loop are summarized in one statement.
2. Example: Iterating through a simple array
Let's say you want to iterate through an array of colors. First, you declare the array and initialize it with values.
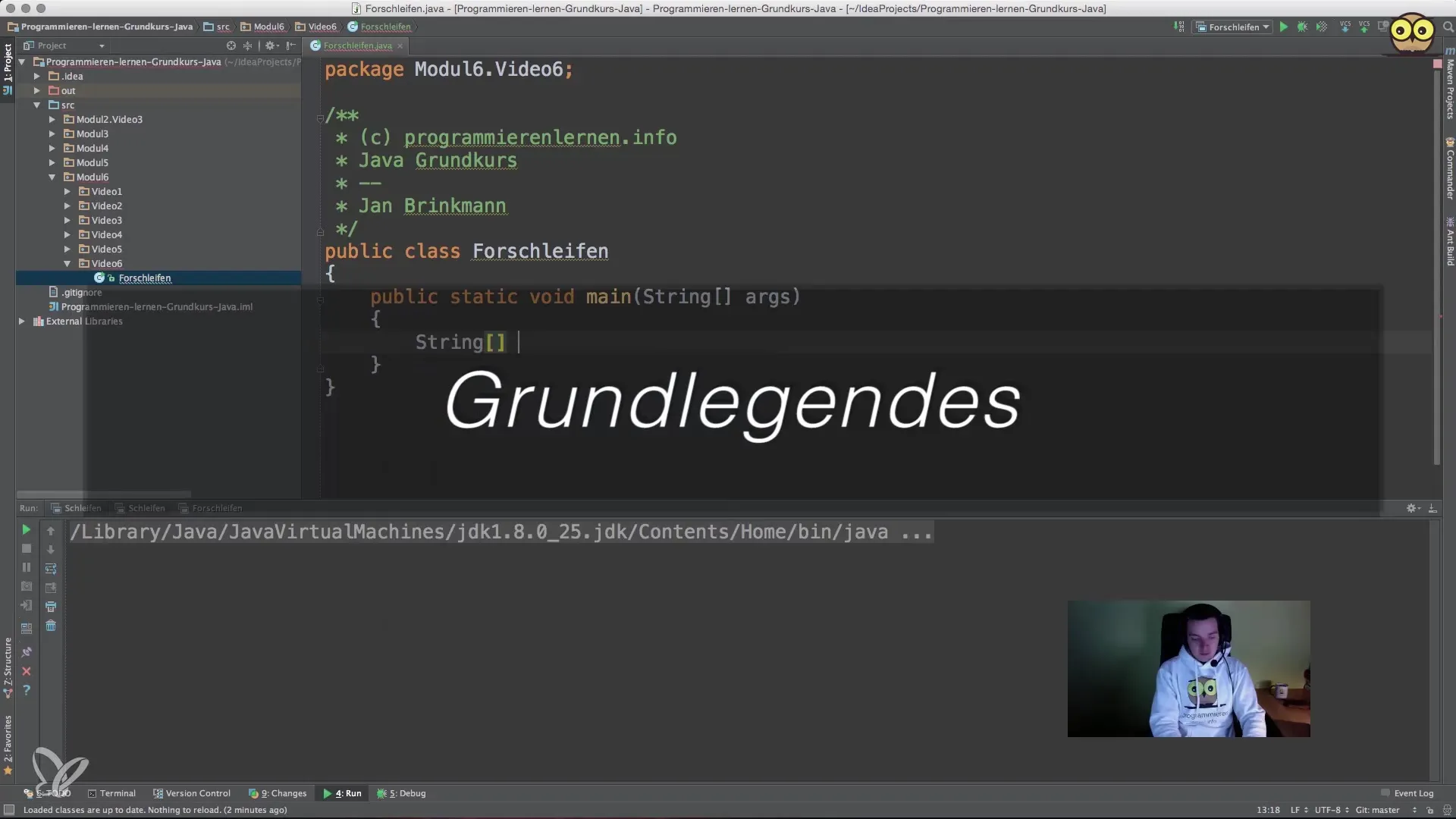
Now you can use the for() loop to access each element and print it in the console.
In this example, you use the length of the array to control the loop.
3. Explanation of the loop counter
The loop counter, also referred to as the index, is typically initialized to 0 in the for() loop. The loop condition is checked, and the loop is executed as long as the condition remains true.
If, for example, i is 0, the loop accesses the first element of the array. You reach the end of the loop when i hits the number of array elements (in this case, 3), meaning the loop ends when i = 3.
4. Doing the opposite: Iterating backwards with the loop
There are situations where you may want to iterate backwards through an array with the for() loop. You can achieve this by setting the initialization value to the length of the array minus one and then adjusting the condition.
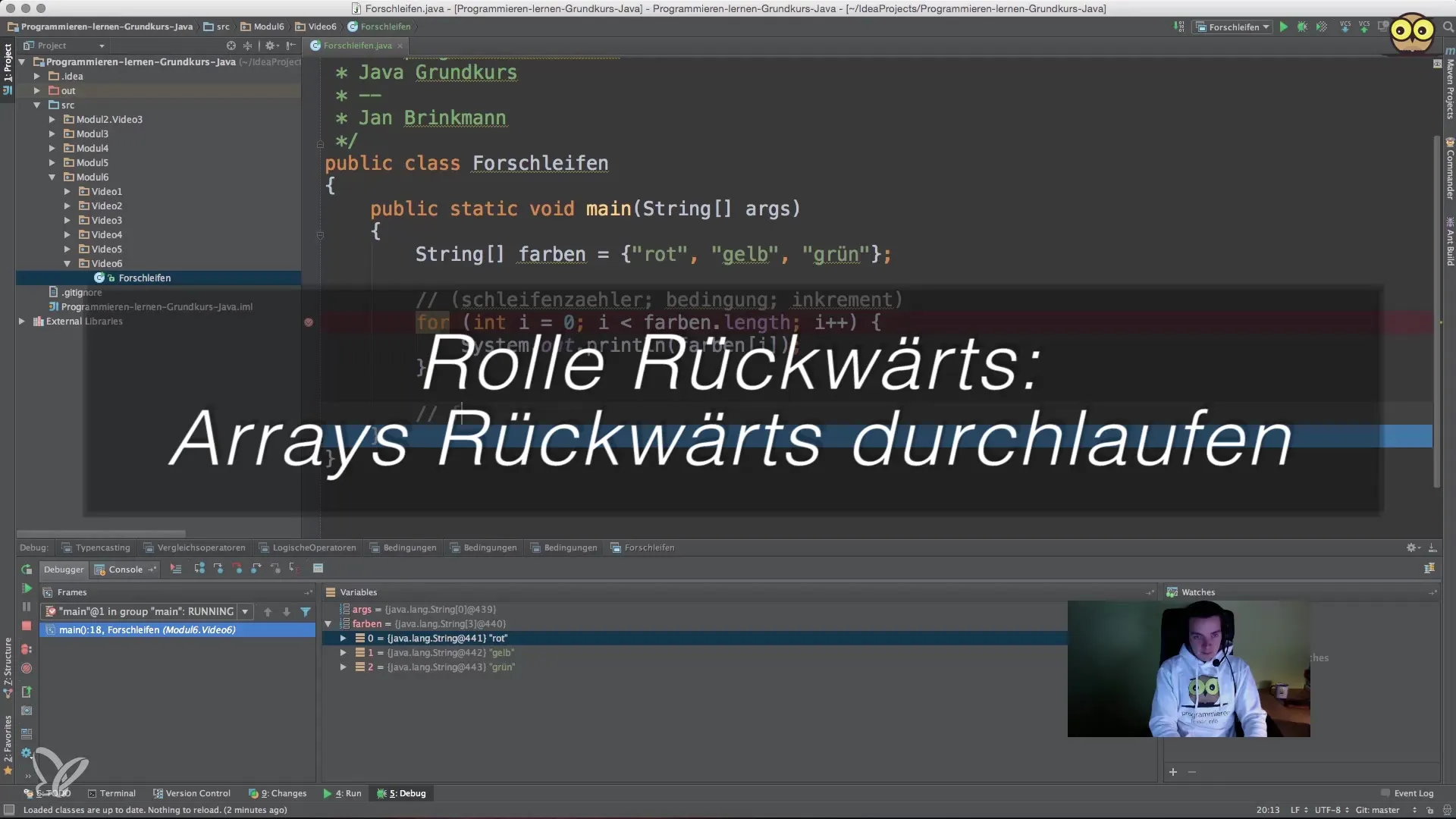
This gives you the elements in reverse order.
5. Nested loops
Now we come to an interesting topic: using nested for() loops. These are helpful when you want to process multidimensional data structures or output a table.
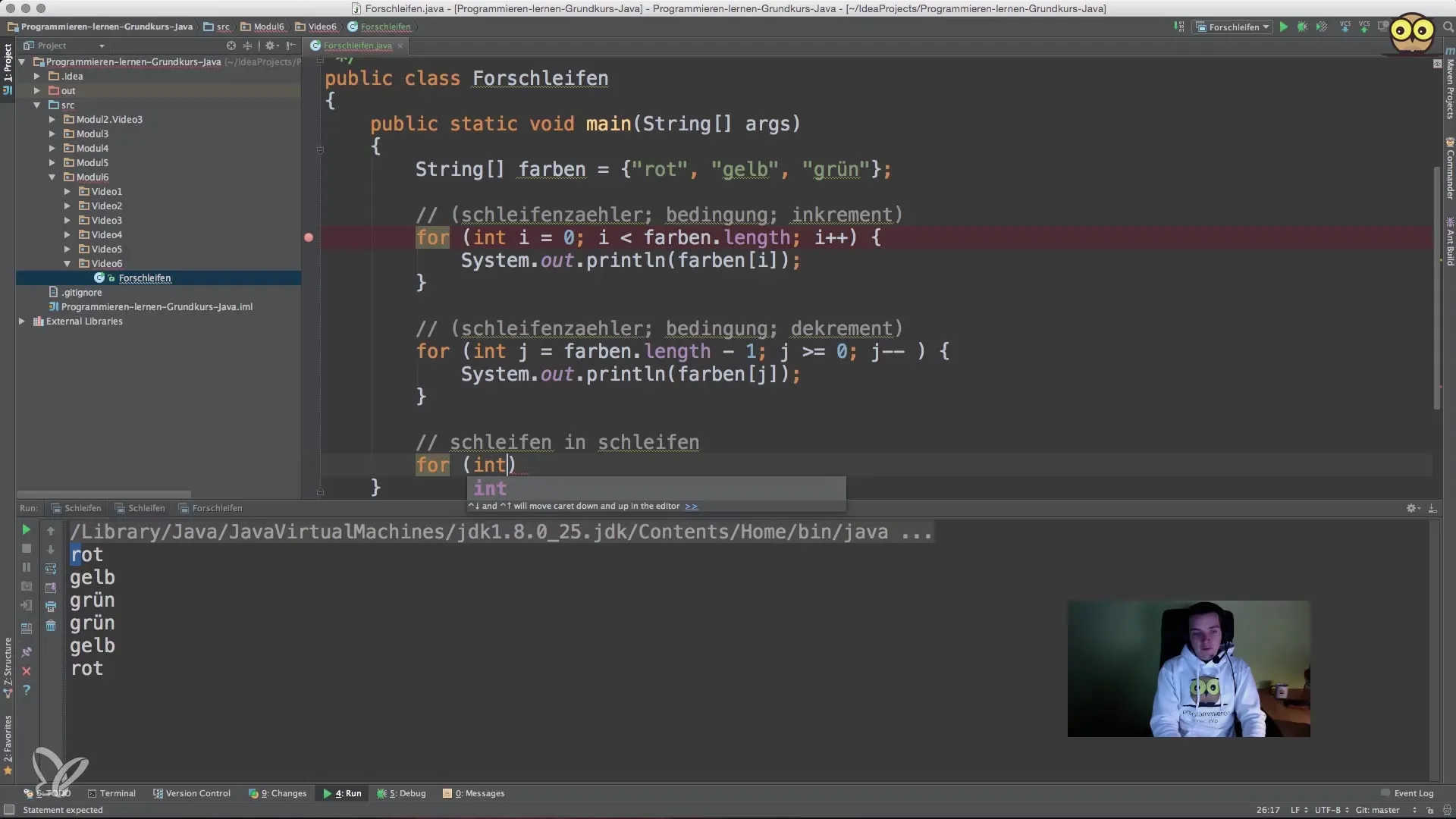
Imagine you want to output a 5x10 grid of X. You could use the outer loop for the rows and the inner loop for the columns.
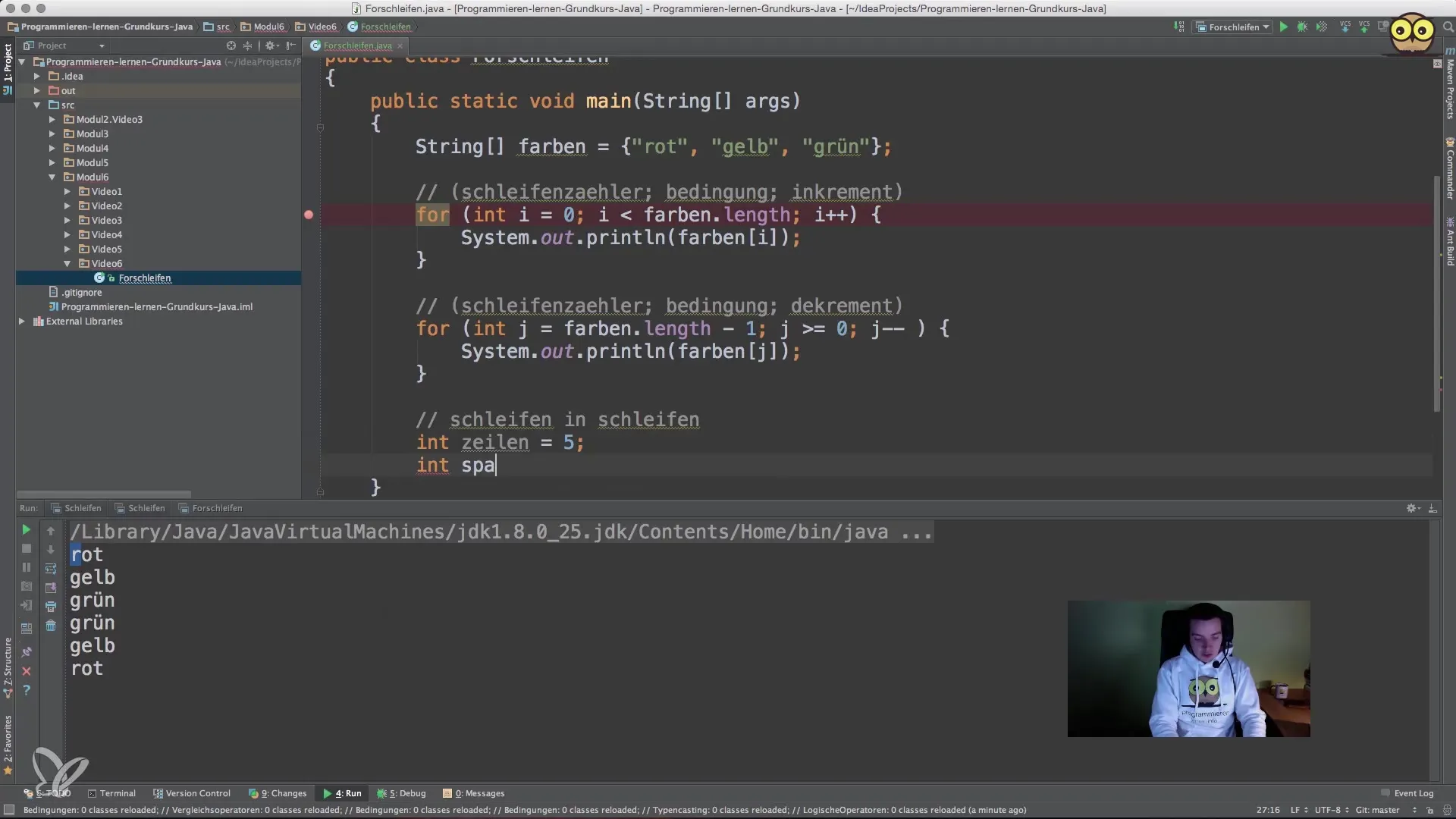
6. Simulating multidimensional arrays
When working with multidimensional arrays, the principle remains the same. You need a loop for each dimension.
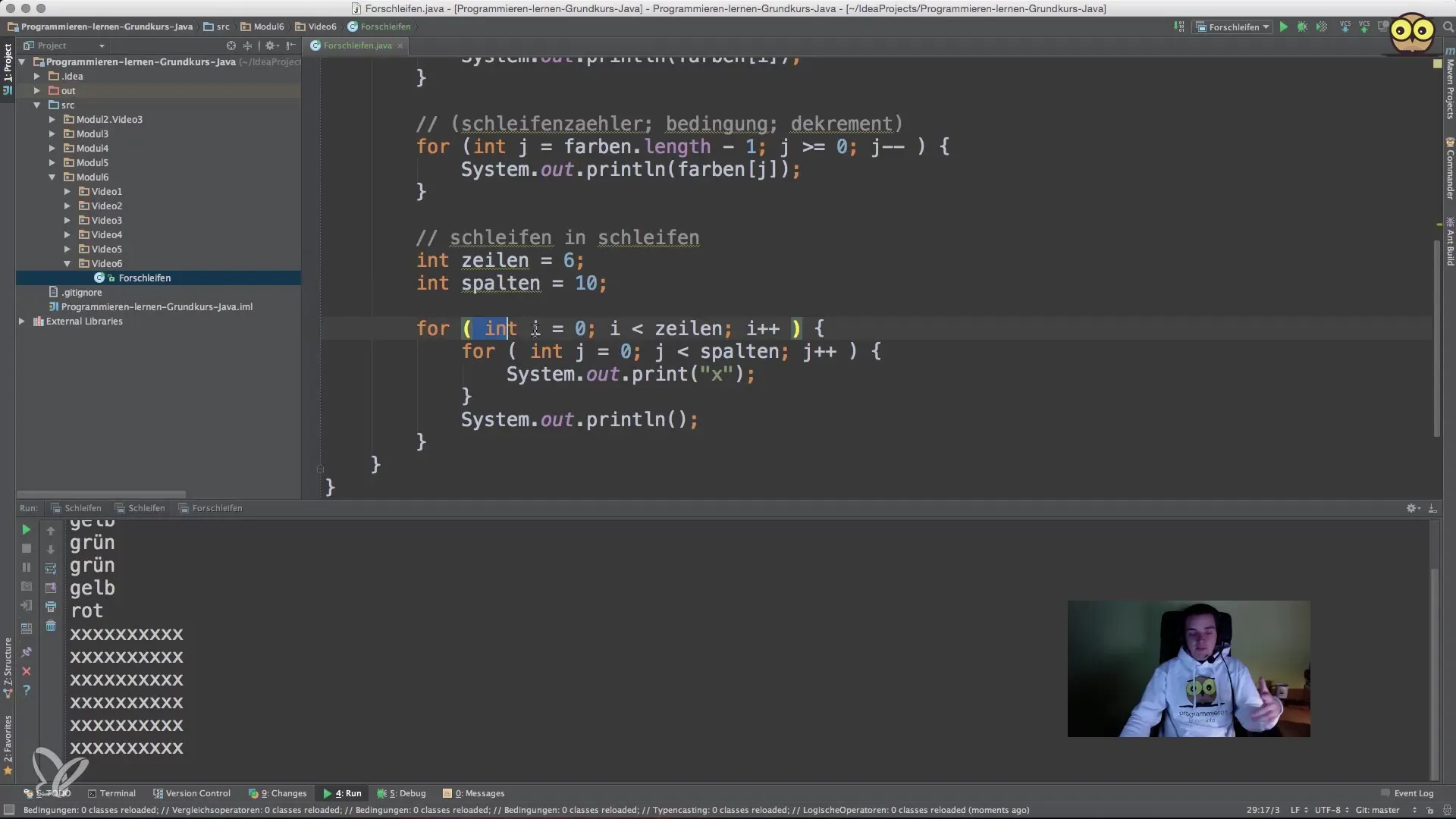
7. When should I use a for() loop?
The for() loop is particularly suitable when the number of iterations is known or well definable. For example, if you know the length of an array or specifically need a certain number of iterations, the for() loop is the right choice.
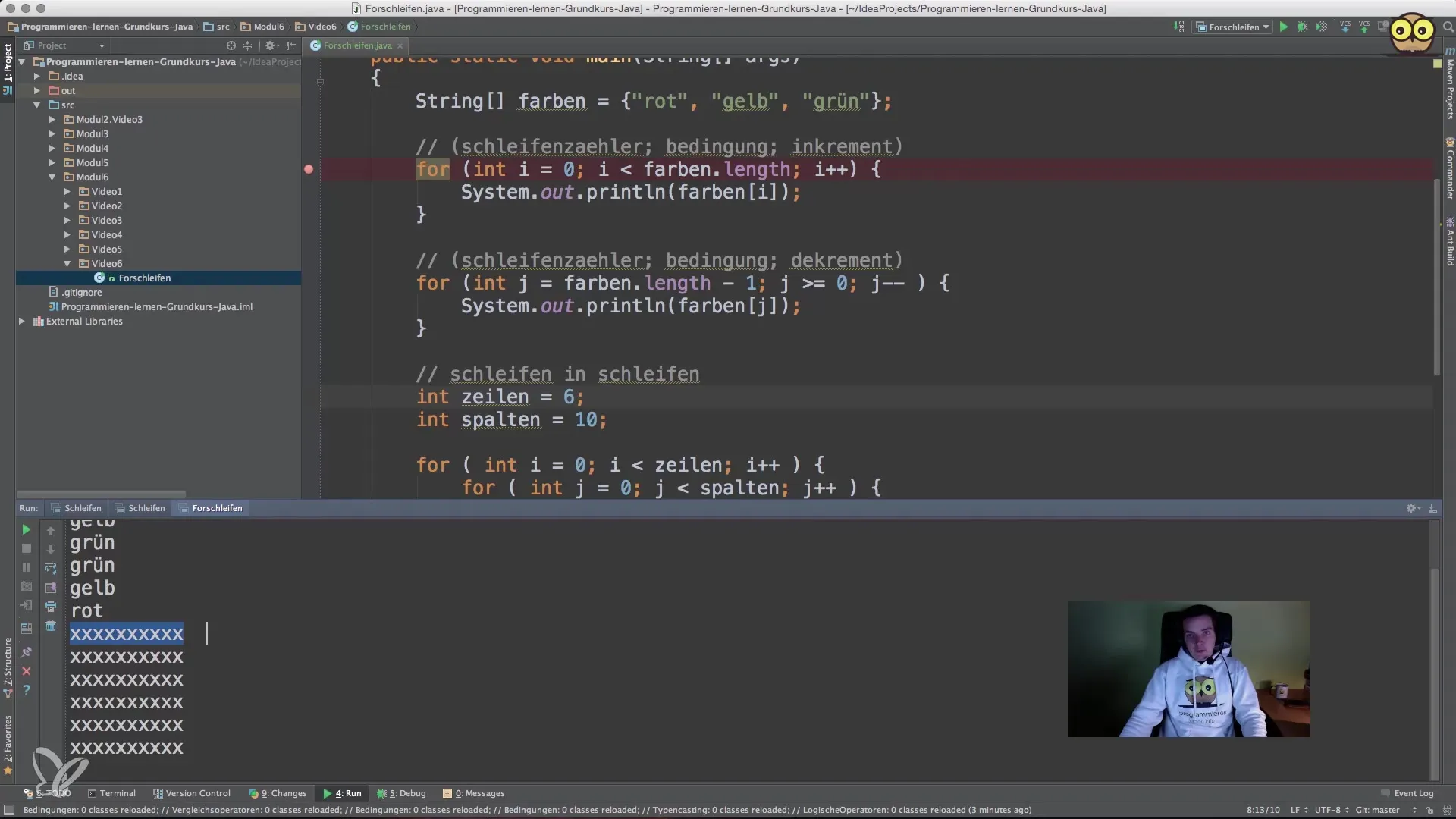
For undefined iterations, such as searching for a specific value, a while() loop may provide a better solution since it is more flexible regarding condition checking.
Summary - Java for Beginners: Understanding for() Loops
The for() loop is an effective tool in Java for performing structured iterations. Through nesting, it can be used to handle more complex data structures. This guide provides you with the necessary foundations to use and apply for() loops correctly and confidently.
Frequently Asked Questions
How do I define an array in Java?An array in Java is created with the type followed by square brackets and an initialization, e.g., String[] colors = {"red", "yellow", "green"}.
What is the difference between for() and while() loops?The for() loop is ideal when the number of iterations is known in advance, while the while() loop is more flexible for an undefined number of iterations.
What are nested for() loops?Nested for() loops are loops defined within another loop to iterate through multidimensional data structures.
How can I reverse a for() loop?By adjusting the initialization argument to the length of the array minus one and reversing the condition accordingly.
How many times is the body of a for() loop executed?The body of the loop is executed as many times as the condition is true. When the condition becomes false, the execution of the loop stops.