When programming with Java, it quickly becomes clear that the concepts of classes and objects are of central importance. They form the core of object-oriented programming and are indispensable for understanding Java. In this tutorial, you will learn how to create classes, instantiate objects, and particularly, how to work with the keyword this.
The principles are illustrated by a practical example that revolves around a vehicle. You will be guided step by step through the process to master both the theoretical foundation and the practical implementation in Java.
Key Insights
- Classes are blueprints for objects.
- Objects are instances of classes.
- The variable this references the current object and is needed to distinguish between instance variables and local variables.
Step-by-Step Guide
1. Basics of Classes
First, you should create a class in Java. This can be done easily with the class keyword, followed by the name of the class. Make sure that the class name and the file name match. In our example, we name the class firstClass.
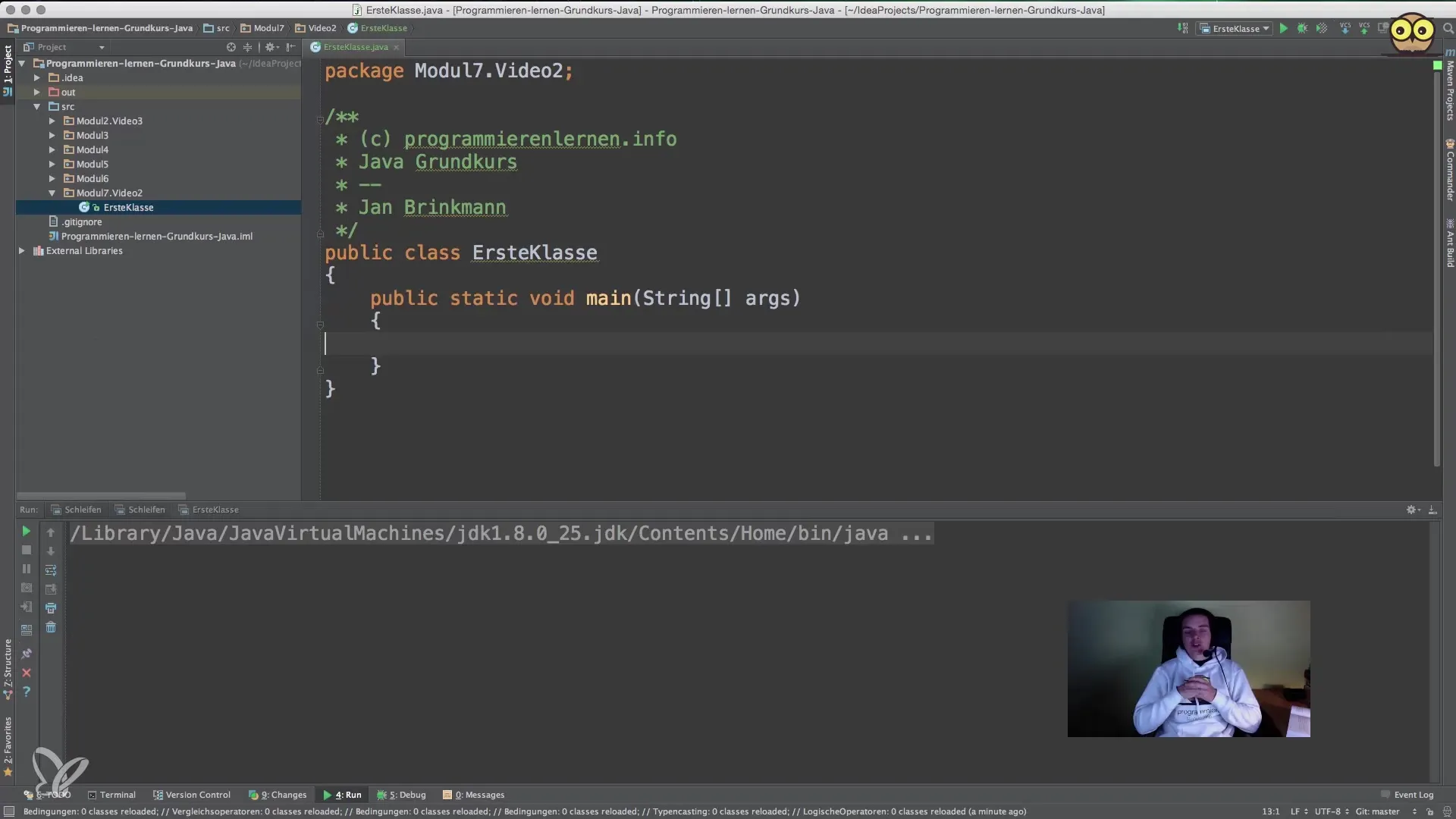
This basic structure is important because the main method is the entry point for any Java program.
2. Creating a Second Class
Now you create a second class, which we will call Vehicle. This class will define the properties and methods that are relevant to our vehicle. Again, use the class keyword and the name accordingly.
3. Defining Attributes
In the Vehicle class, you will define attributes that are important for a vehicle, for example, the speed. These attributes are declared outside of the methods and are of type Integer. Here you define the speed:
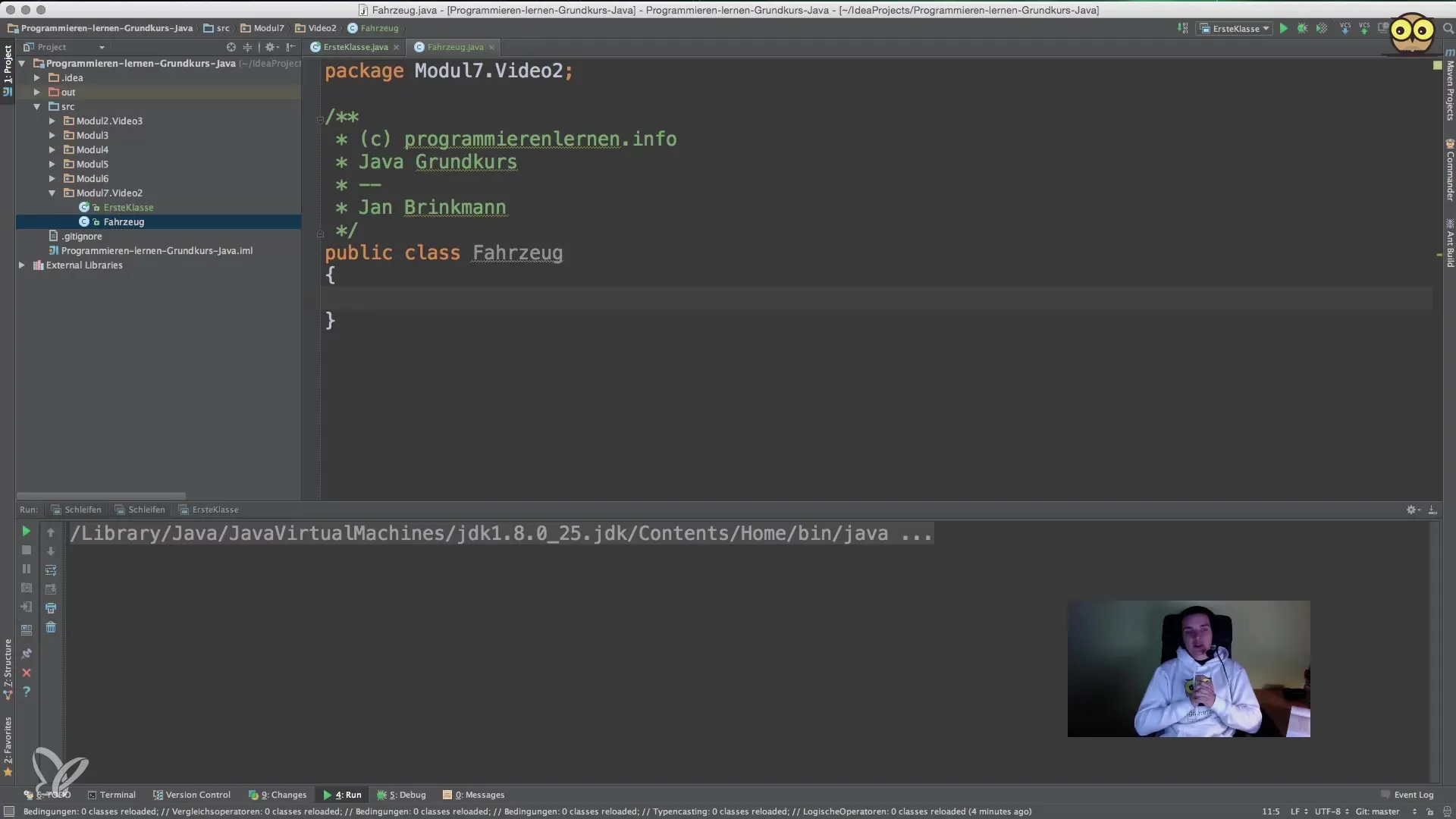
4. Creating a Method for Speed Initialization
Now you need a method that allows you to set the speed of the vehicle. We will name this method drive. The method declaration follows the pattern you know from the main method.
5. Accessing Attributes with this
To access the instance variable speed, you use the keyword this. This ensures that you are accessing the instance variable and not a local variable.
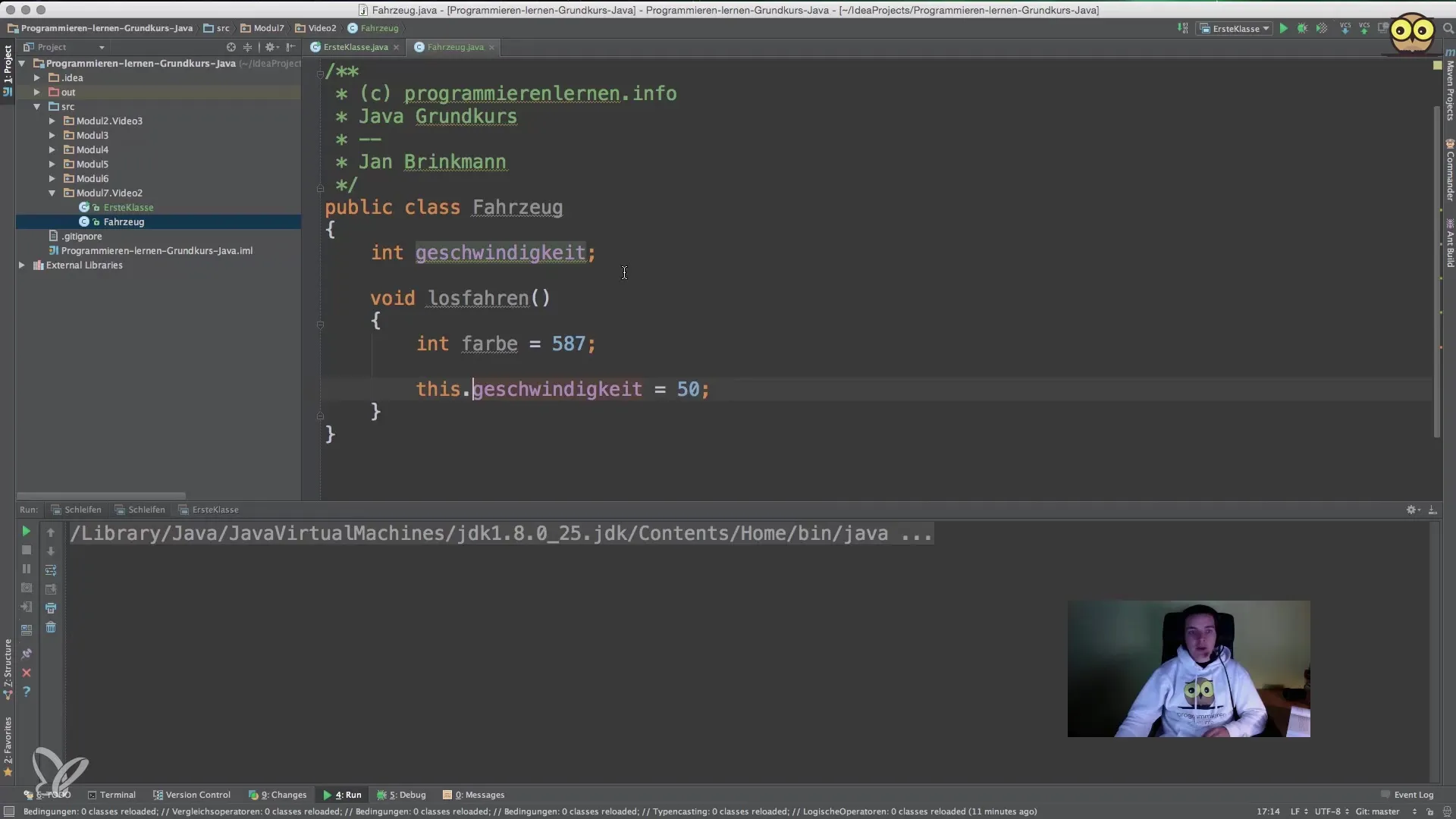
6. Creating an Object of the Vehicle Class
Now that your classes and methods are defined, it is time to create an object of the Vehicle class. This takes place in the main method of firstClass. You can do this with new.
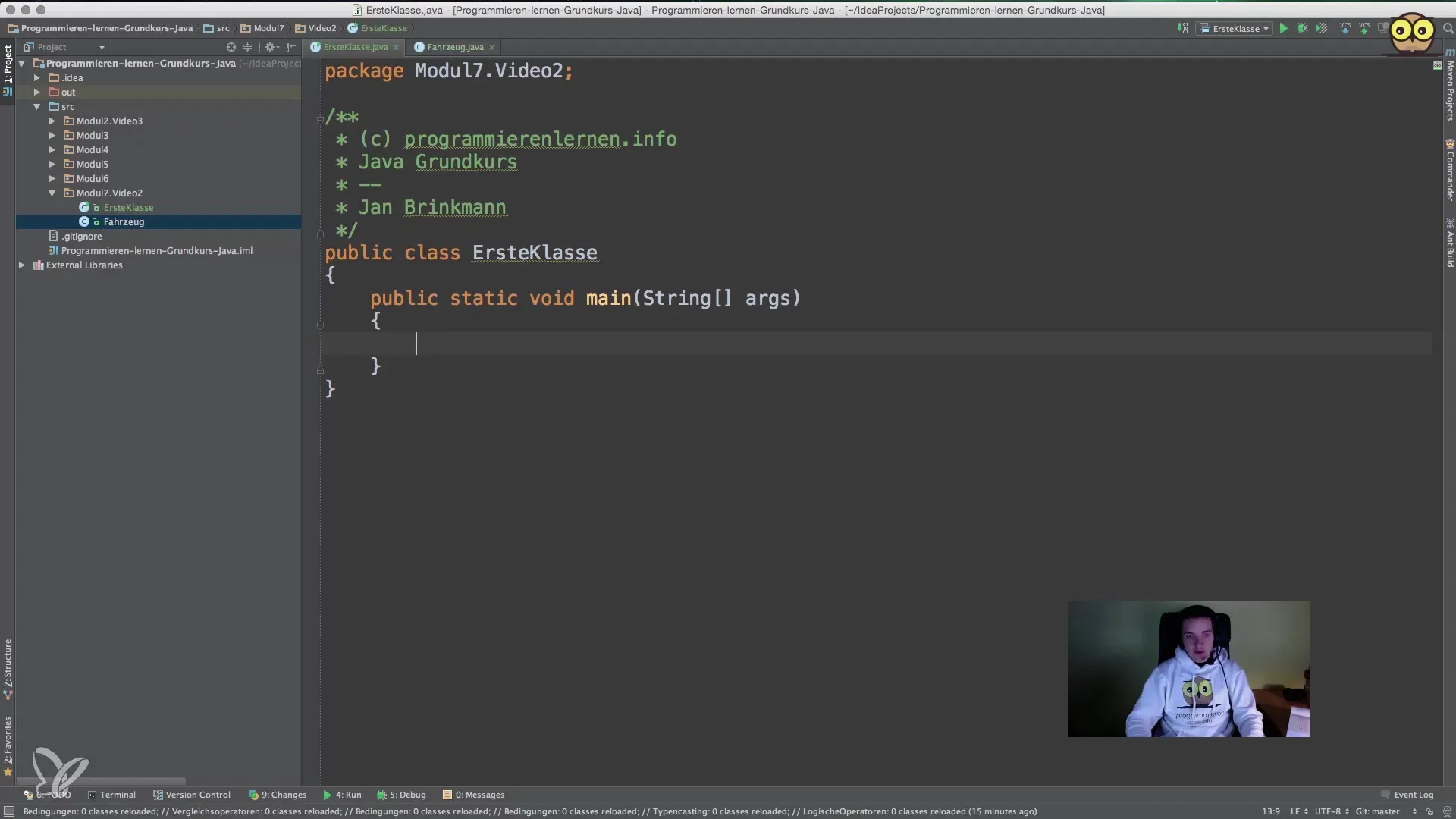
Here a new object myVehicle is created, and the method drive is called.
7. Running the Program
Now run your program. You should see the output “I have started driving” in the console. This confirms that your vehicle has been successfully set in motion.
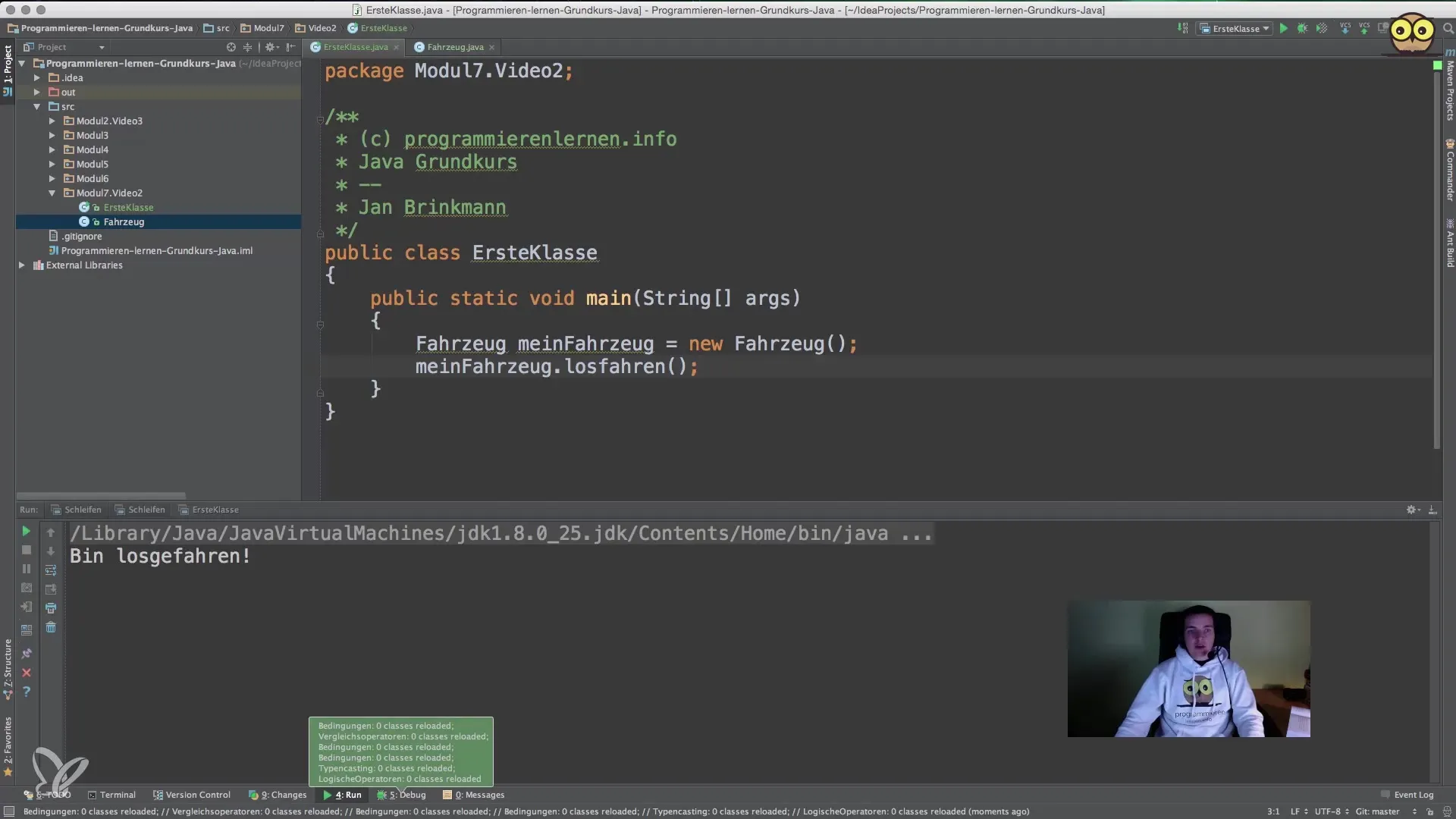
8. Debugging for Verification
If you want to learn more about the flow of your program, you can work with a debugger. Set breakpoints in your drive method and observe the values of your variables.
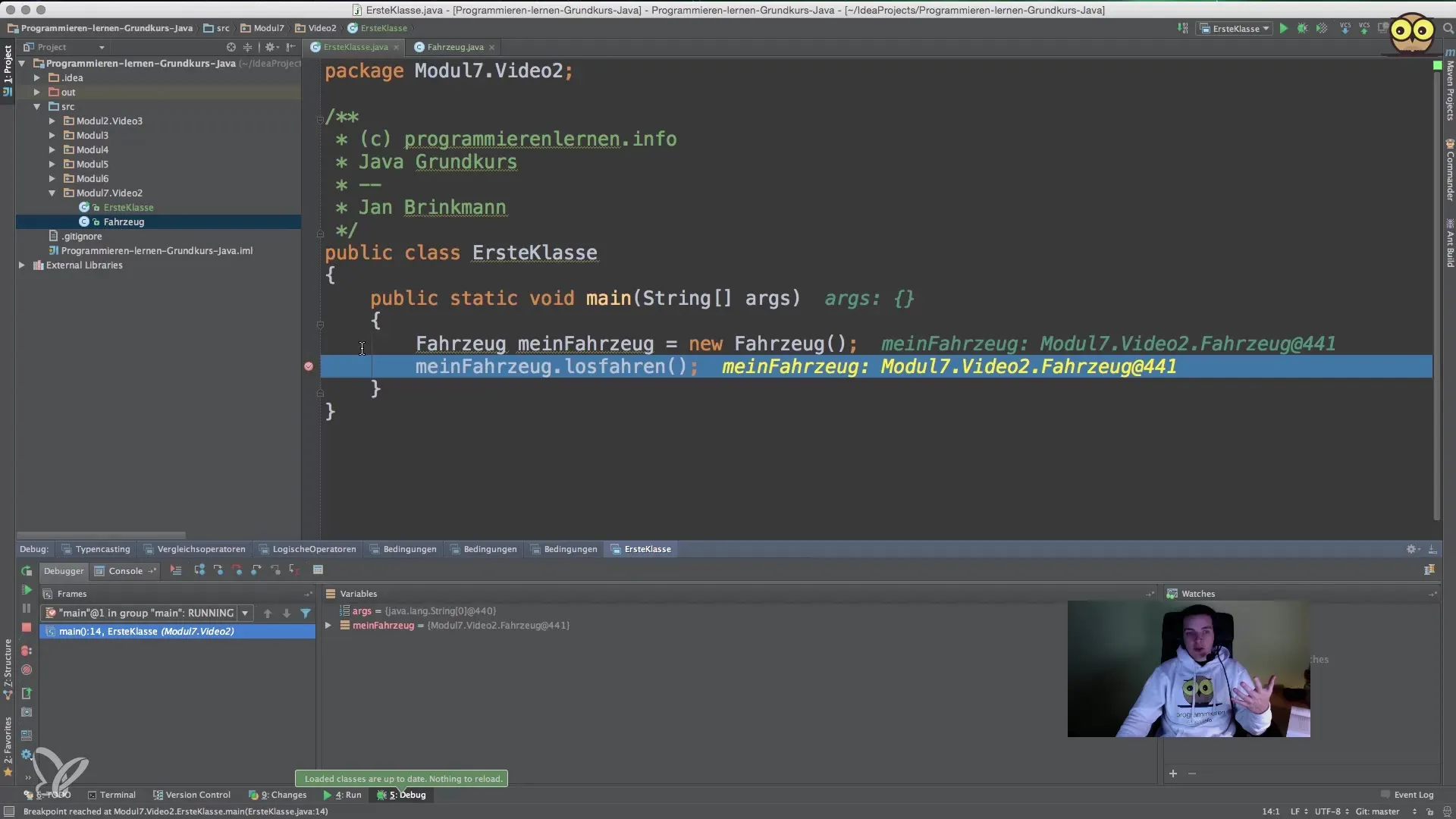
This way you can see how the speed and other values are set during the execution of the program.
Summary - Java for Beginners - Classes, Objects, and the Variable “this”
In the course of this tutorial, you have learned fundamental concepts of object-oriented programming in Java. You learned how to create classes, instantiate objects, and work with the this variable that helps you distinguish between instance variables and local variables. With the example of a vehicle, you could practically experience how classes and methods interact.
Frequently Asked Questions
How do I declare a class in Java?You use the class keyword followed by the name of the class in CamelCase.
What is the difference between instance variables and local variables?Instance variables are attributes of a class that are available to all methods in the class. Local variables are only visible within the method in which they were declared.
How do I access instance variables within a method?You use the this keyword to access the instance variable unambiguously.
How do I run my Java program?Make sure the main method is present, and click on “Run” in your IDE.
What is the keyword new in Java?new is used to create a new object of a class.