Are you ready to delve deeper into the basics of programming? Logical operators are a central component of every programming language, including Java. They allow you to link multiple conditions and create more complex decision structures. In this guide, you will learn how logical operators work in Java, and you can effectively expand your programming skills.
Key Takeaways
- Logical operators are crucial for linking conditions.
- The main logical operators in Java are: NOT, AND, and OR.
- The NOT operator negates the truth value of a condition.
- The AND operator requires both conditions to be true.
- The OR operator allows at least one of the two conditions to be true.
Understanding Logical Operators
1. The NOT Operator
The NOT operator, represented by the symbol! in Java, is used to negate the truth value of a condition. If you apply the concept correctly, understanding it will be easier.
You would formulate an if condition to check if a statement is true. If it is presented, you can use the NOT operator to test the opposite.
Here is the basic example:
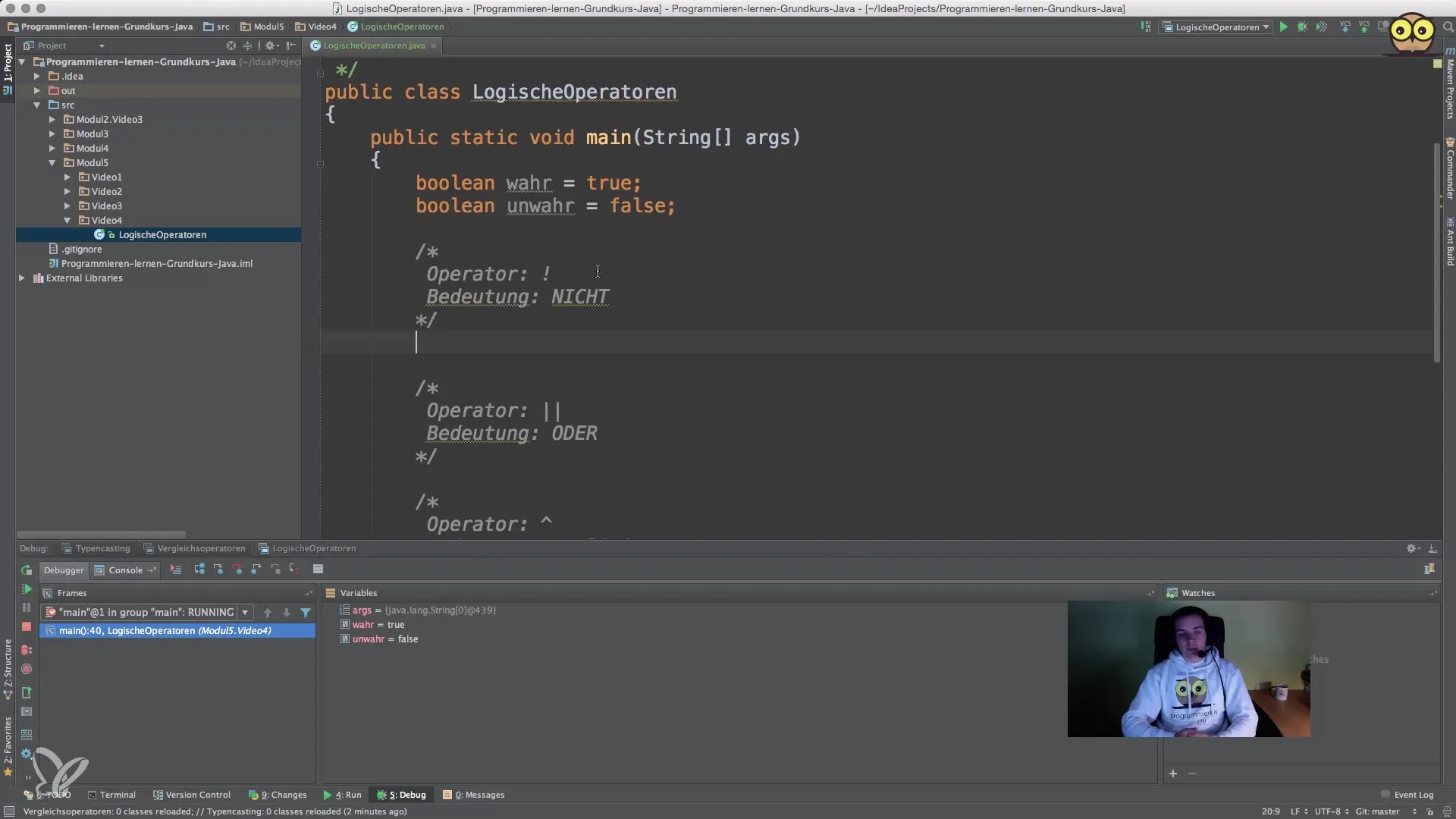
If the original condition W has the value true, the program outputs "Statement 1 is true." However, if you want to test the negation, you can use!W. If W is now false, the ELSE branch will be activated.
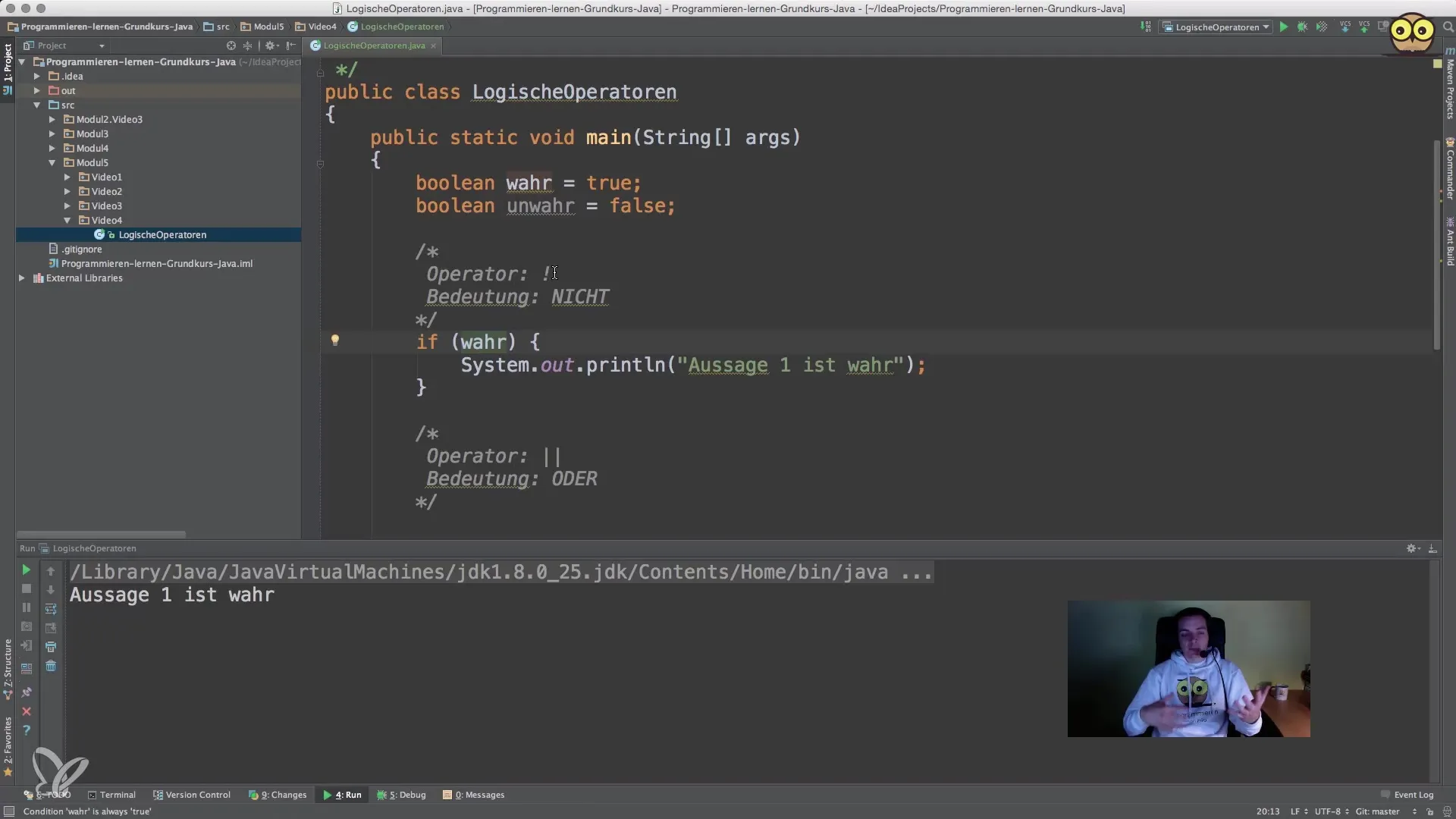
Make sure to always use the correct expressions and conditions to avoid confusion.
2. The OR Operator
The OR operator is represented by the symbols || in Java. If at least one of the two conditions is true, the if condition is considered fulfilled. It is great for creating alternatives.
In this case, your code might look like this:
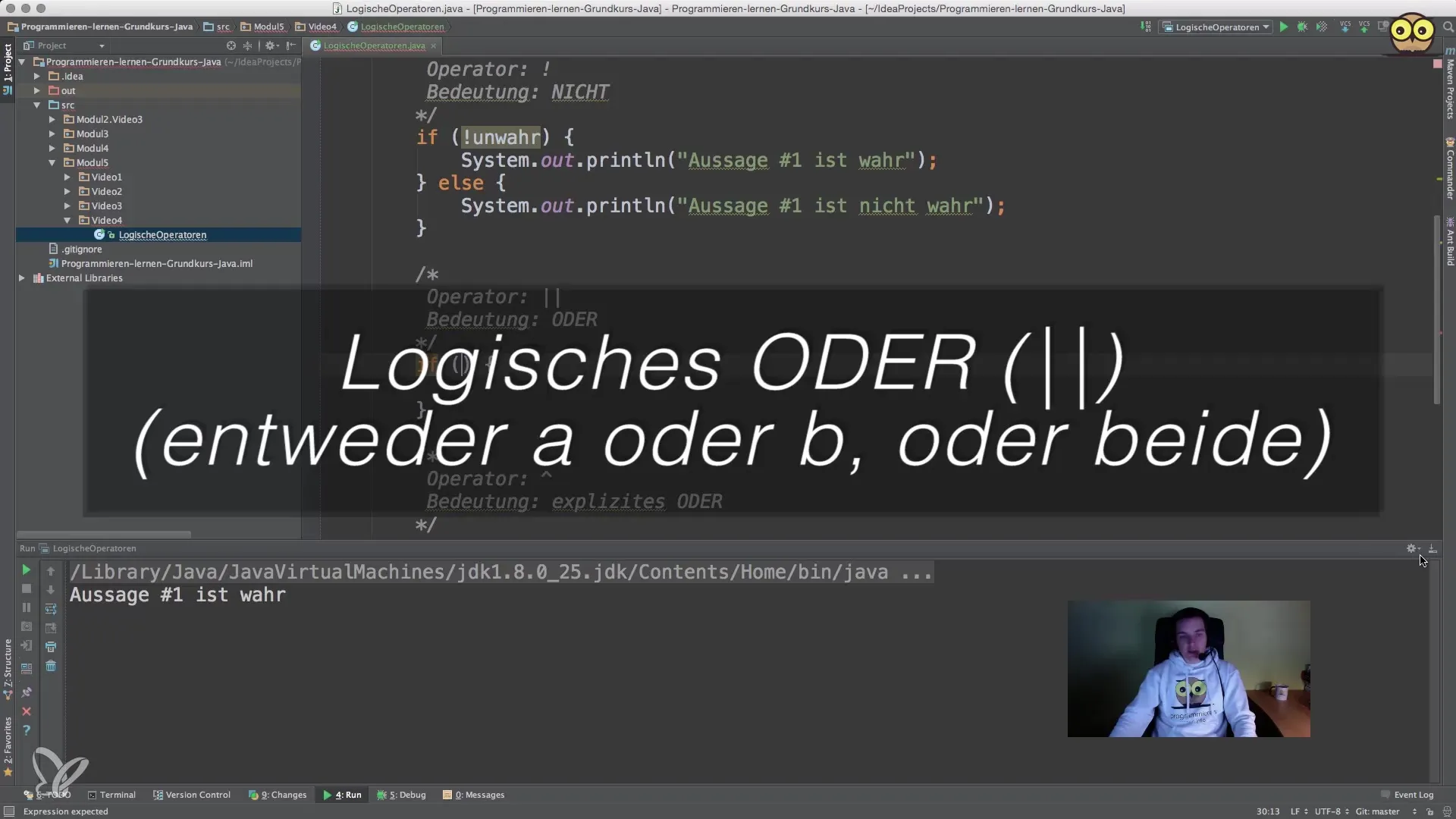
Here you check two conditions. If one of them is fulfilled, the program prints "or has triggered." If both conditions are false, then the statement "or has not triggered" is output.
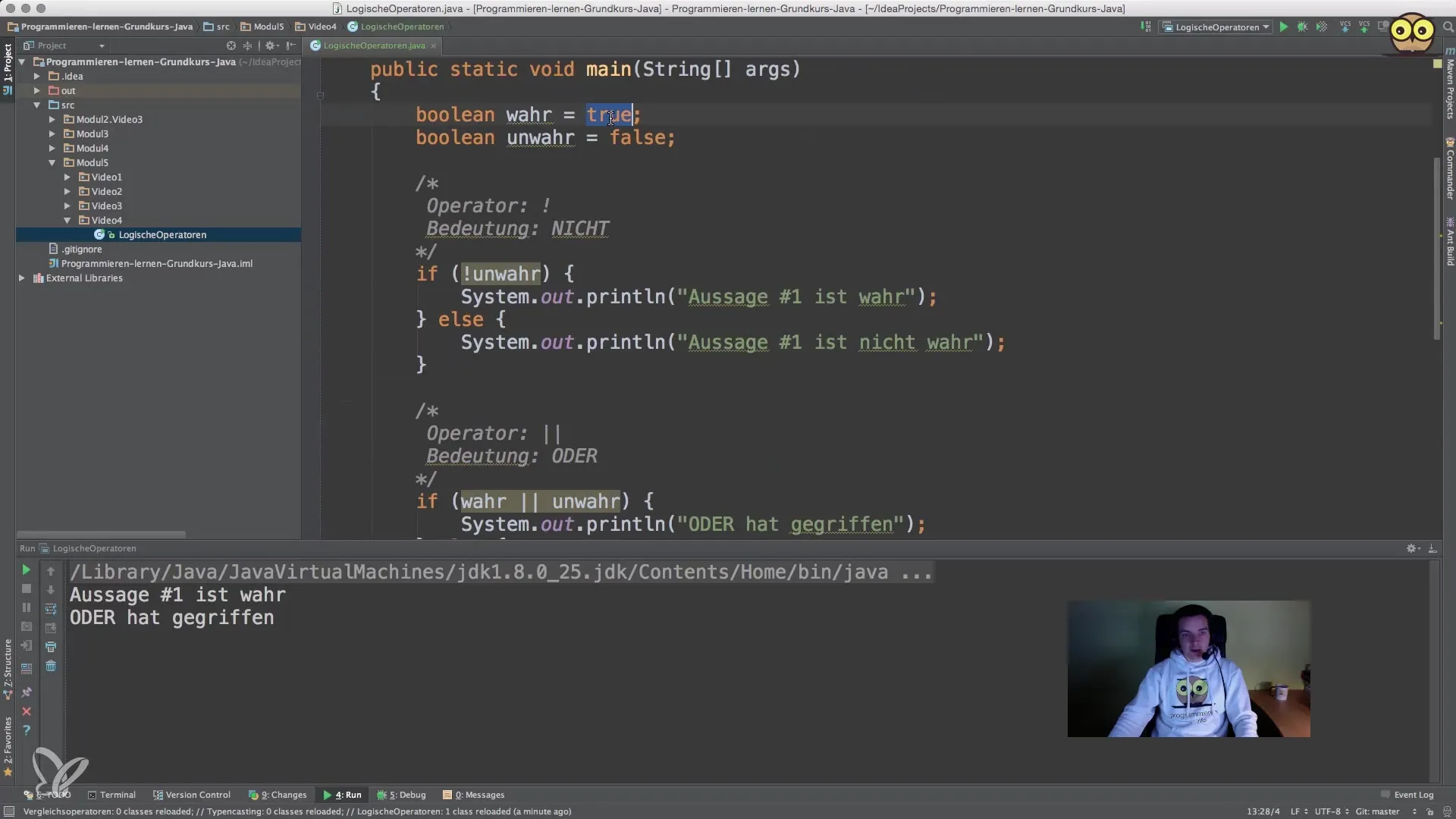
This is particularly useful for combining different scenarios that do not all need to be fulfilled at the same time.
3. The AND Operator
The AND operator is used in Java with the symbol &&. Here, both conditions must be true for the entire condition to be considered true.
Here is an example of how you apply the AND operator:
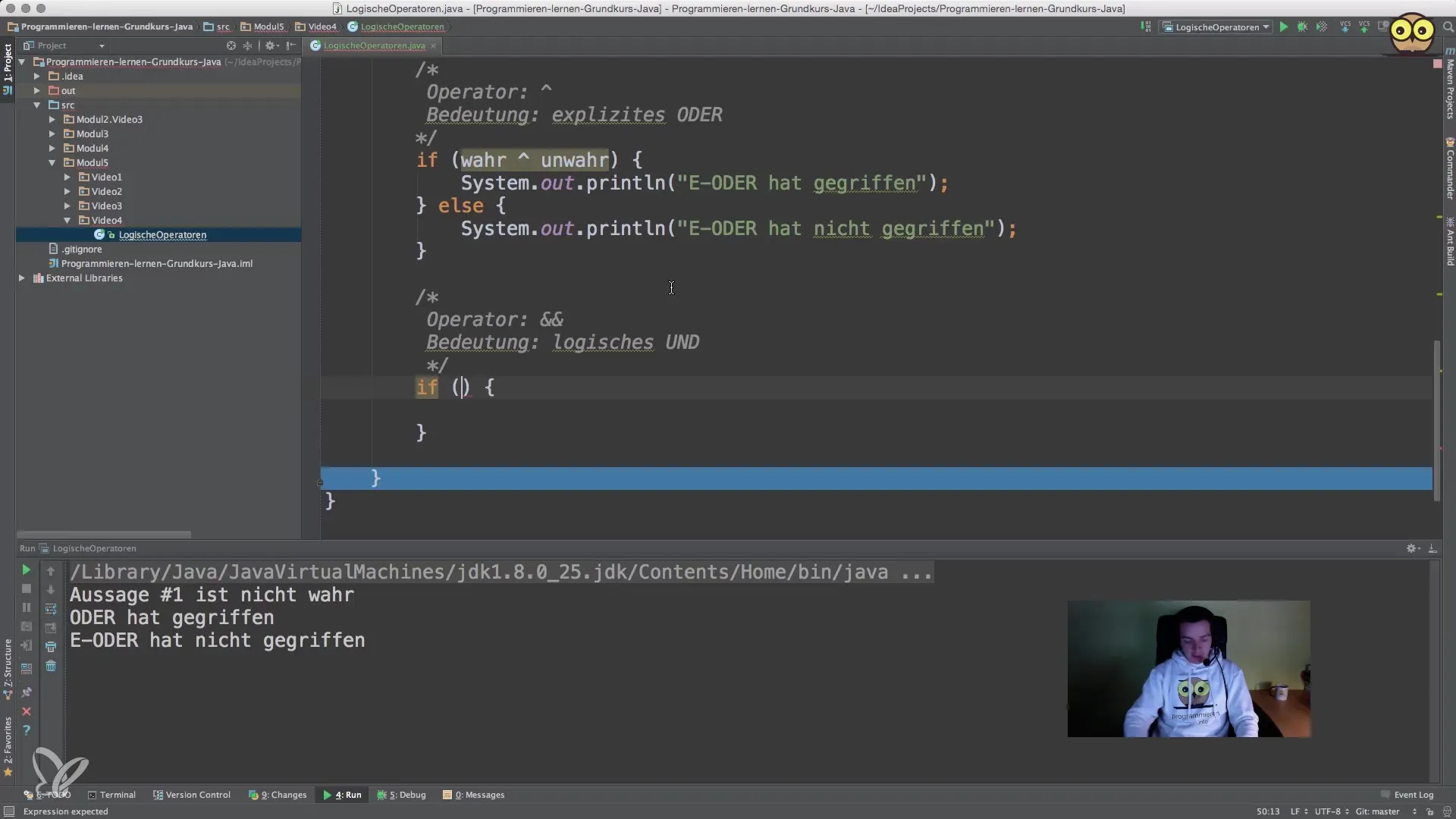
In this example, you check if both conditions are fulfilled at the same time. If they are, "and has triggered" is output. Otherwise, the program indicates that it has not triggered.
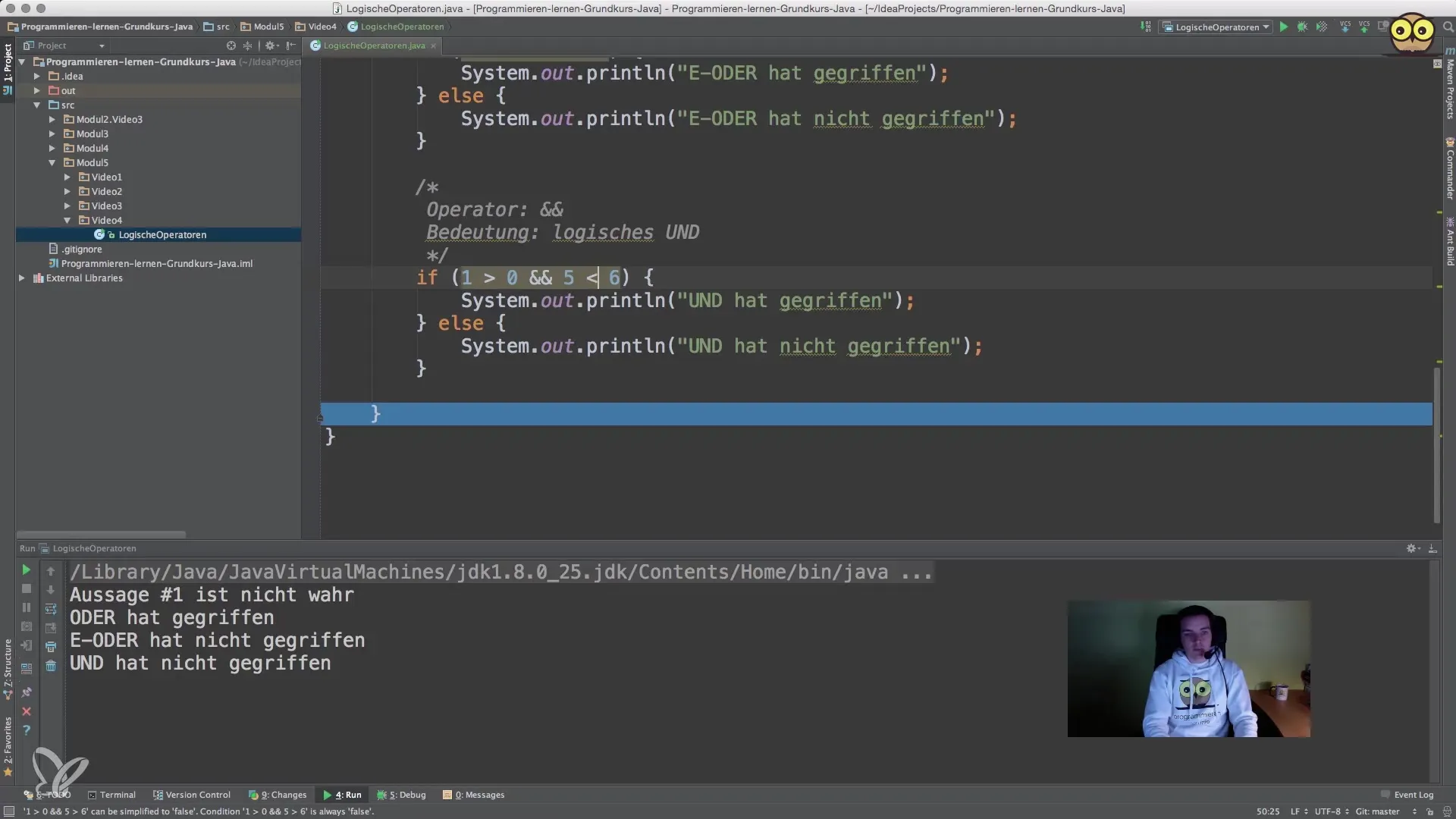
This is particularly relevant when you want to ensure that multiple conditions are fulfilled at the same time.
Applying Logical Operators in Complex Conditions
Logical operators can help you create multifaceted conditions by combining them. You can even use parentheses to control the priority of conditions. This allows for finer control of your logical expressions.
4. Combining Operators
With clever combinations of logical operators, you can formulate very complex queries. This includes not only linking AND and OR conditions but also incorporating comparisons.
Your code might look something like this:
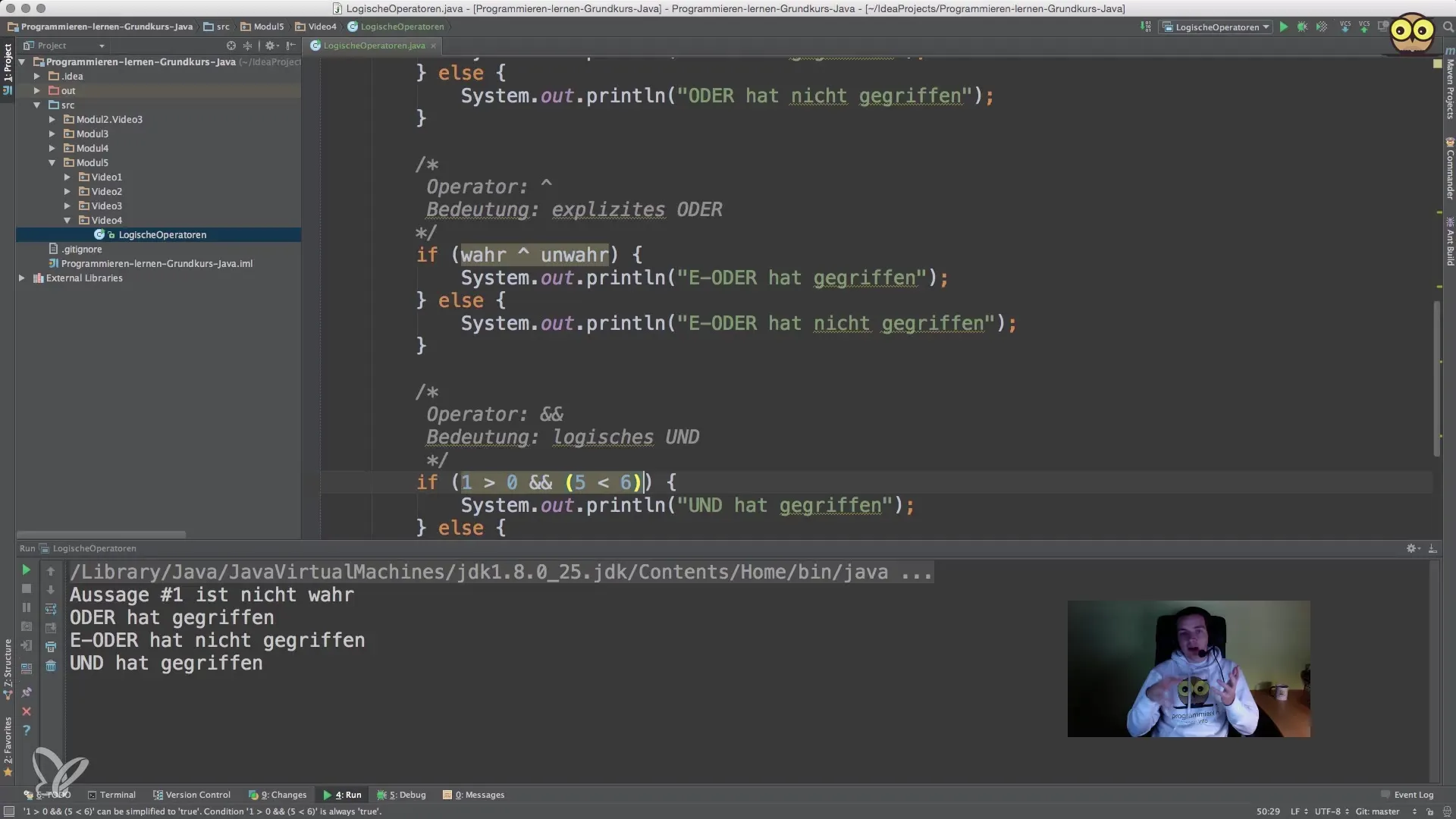
With such code, you check multiple criteria at once. Make sure that the individual conditions are clearly formulated to avoid misunderstandings.
Summary – Logical Operators in Java
Logical operators are essential for programming in Java. By using NOT, AND, and OR, you are able to create logical queries that cover a variety of use cases. You now have the tools to write complex programs based on your conditions.
Frequently Asked Questions
How does the NOT operator work in Java?It negates the truth value of a condition, e.g.,!true becomes false.
When do I use the AND operator?Whenever multiple conditions must be true at the same time.
How do I use the OR operator?Allow at least one of the conditions to be true to fulfill the entire expression.
Can I combine logical operators?Yes, you can combine AND, OR, and NOT to create complex logical queries.
How important are logical operators in programming?They are fundamental as they help you make decisions in your code.