Java is an object-oriented programming language that supports both primitive and complex data types. When programming in Java, you will quickly encounter the need to use complex data types. These allow you to access a variety of functions and methods associated with individual values. In this guide, you will learn the basic concepts of complex data types, particularly the wrapper classes for primitive data types, as well as practical examples.
Key findings
- Complex data types in Java are classes that extend primitive data types.
- Primitive data types like int only store values, while complex data types like String provide methods.
- Wrapper classes allow you to treat primitive data types as objects and provide additional functionalities.
Introduction to complex data types
A complex data type in Java is a collection of properties and methods that build on primitive data types. While primitive data types like int, float, or char only store values, complex data types, also known as reference data types, can provide additional methods and properties.
To clarify the difference, let's take a few examples:
Primitive data types
Imagine you have a primitive data type int. When you store a value, you are merely storing that value without being able to access it or do anything with it, except to use it or pass it on.
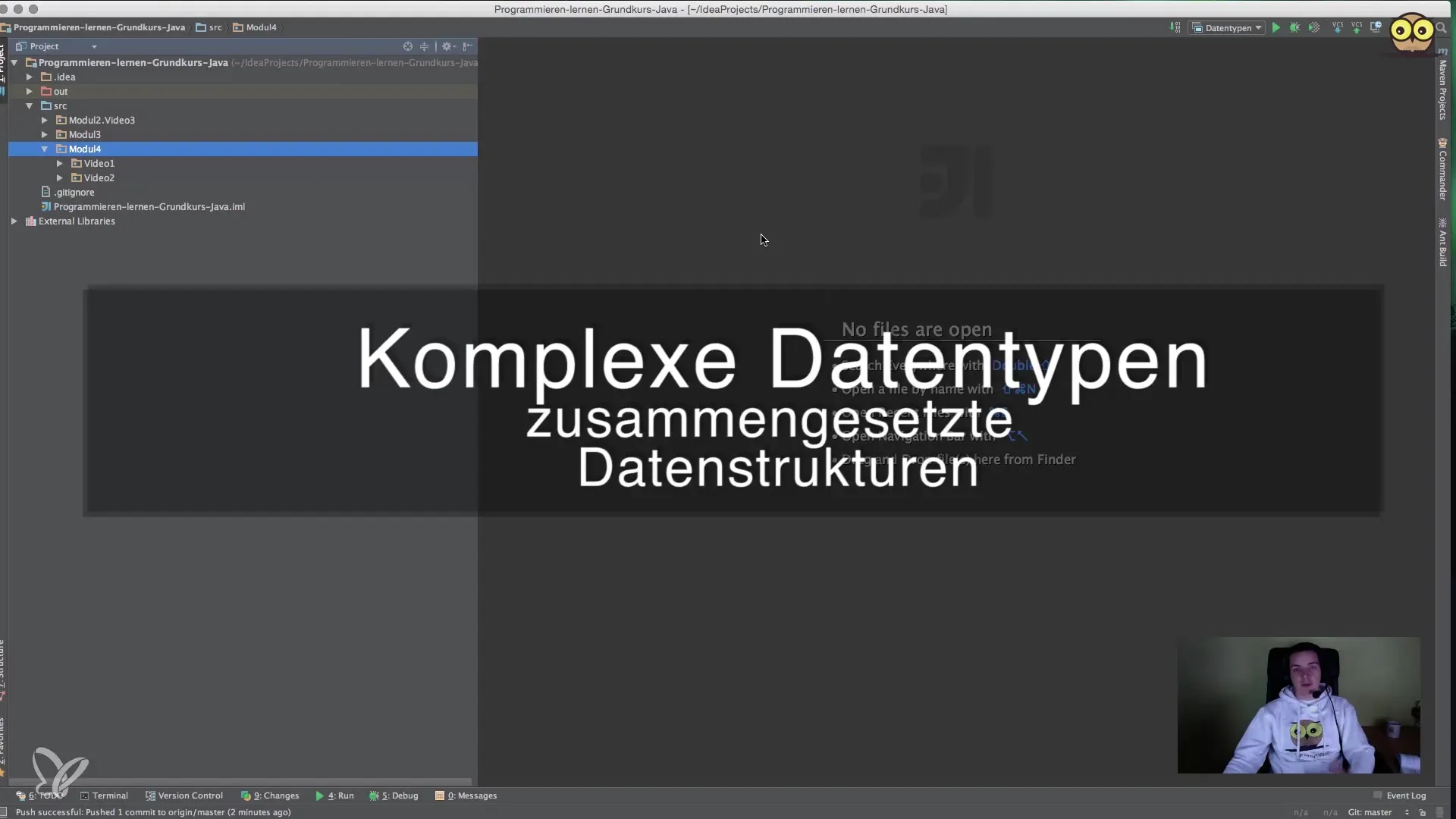
Complex data types
A complex data type like String, on the other hand, offers you many useful methods. You can perform operations such as comparing strings, calculating the length, or searching the string. With these methods, you can work more comprehensively.
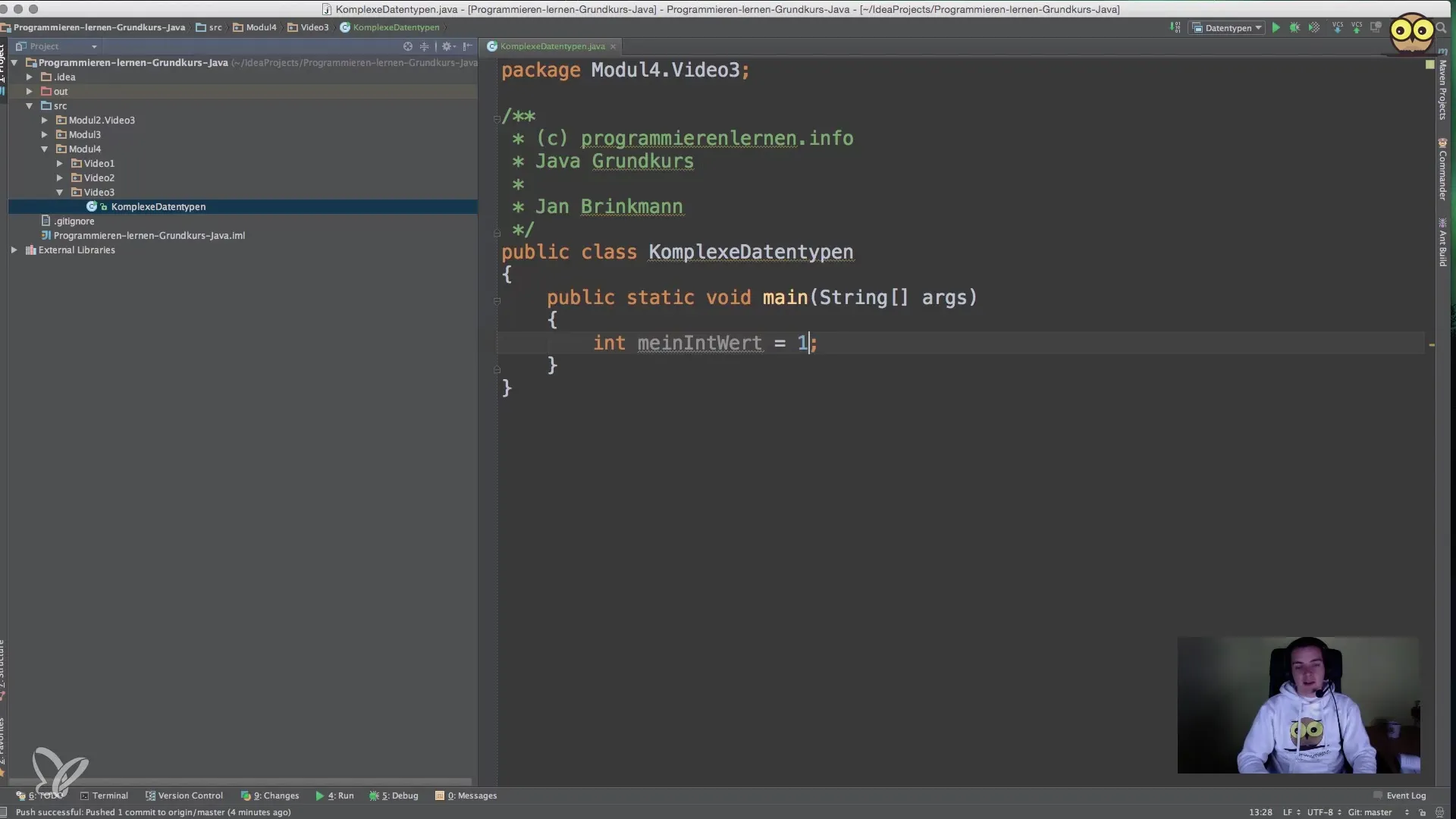
Step-by-step guide to using complex data types
1. Create a new Java project and a package
For the first step, create a new Java project and a package in which you can store your classes. This is usually done through the IDE of your choice. Right-click on the source folder to create a new package.
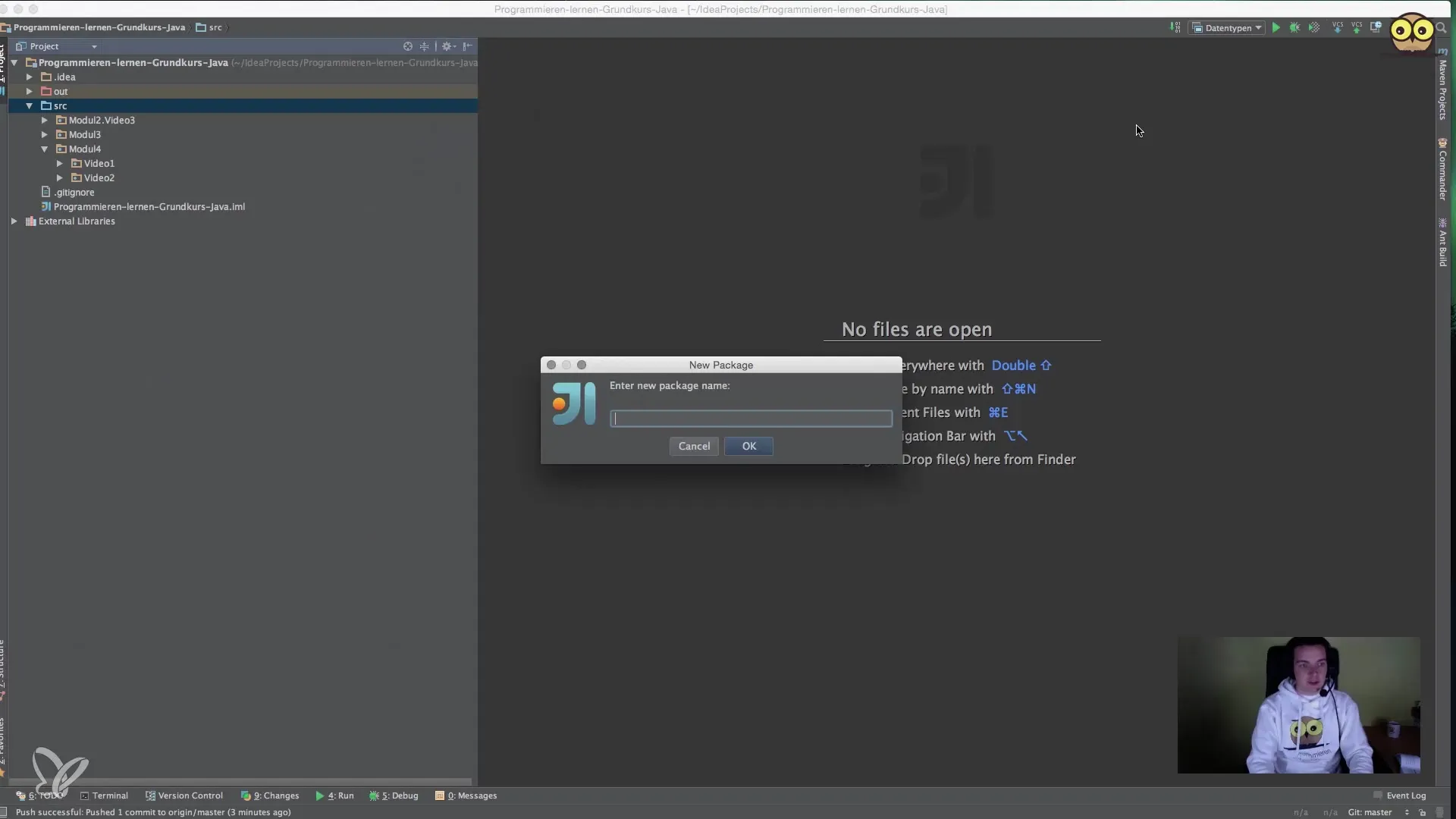
2. Create a class for complex data types
After creating your package, create a new class that represents complex data types. This is done in the same package.
3. Define the main part of your class
Now add the main method. Within this method, you can later experiment with the different data types and their methods.
4. Use a primitive data type
Create a variable of the primitive type int and assign it a value. You can store the value, but you will find that you cannot call methods directly on this variable.
5. Utilize a complex data type with String
Now create a String variable and assign it a value. Note that you can call many methods here, such as length(), equals(), and many others to work with the string.
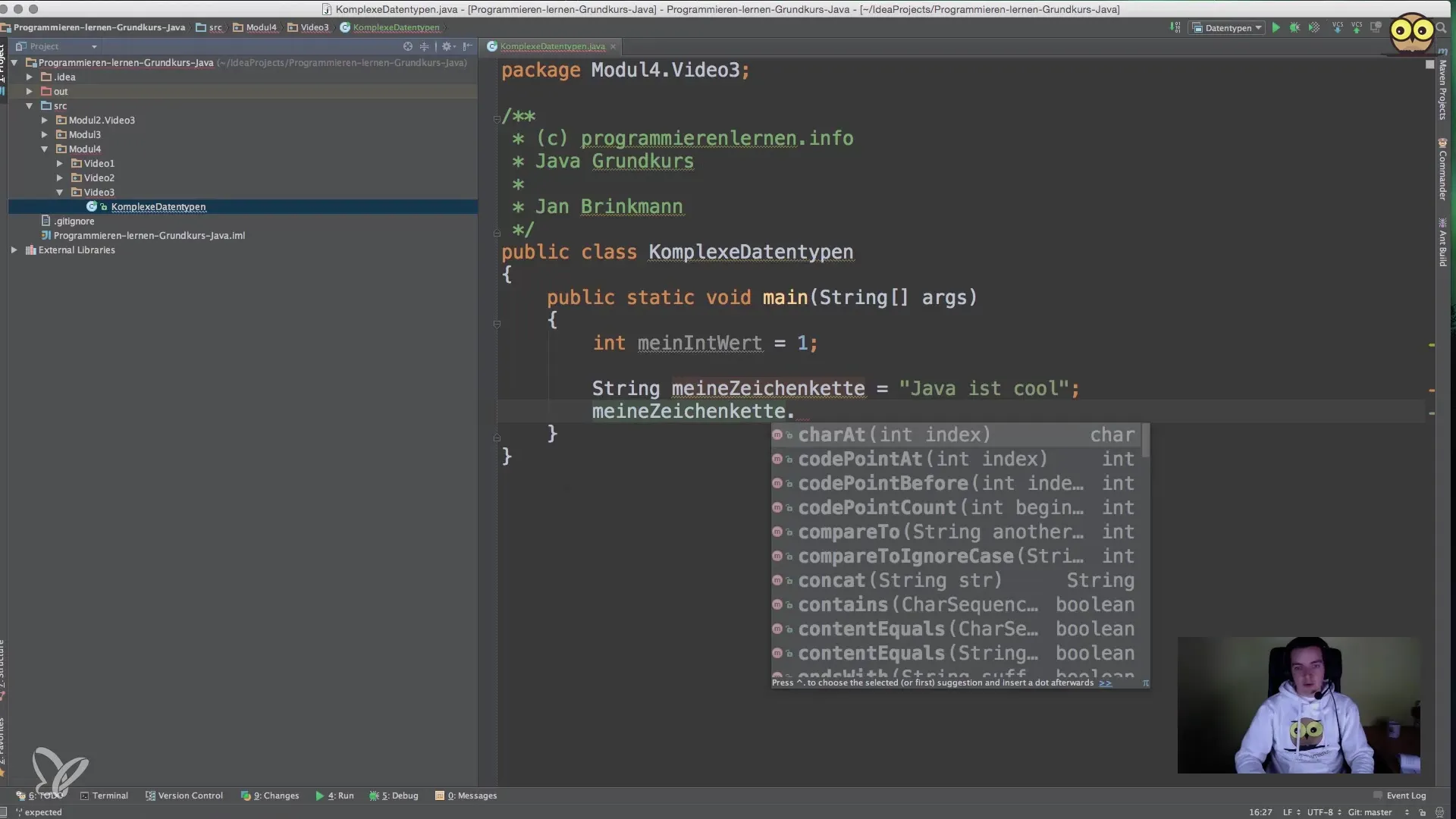
6. Display the length of a string
To display the length of the string value, use the length() method. It gives you a clear idea of how many characters your string contains.
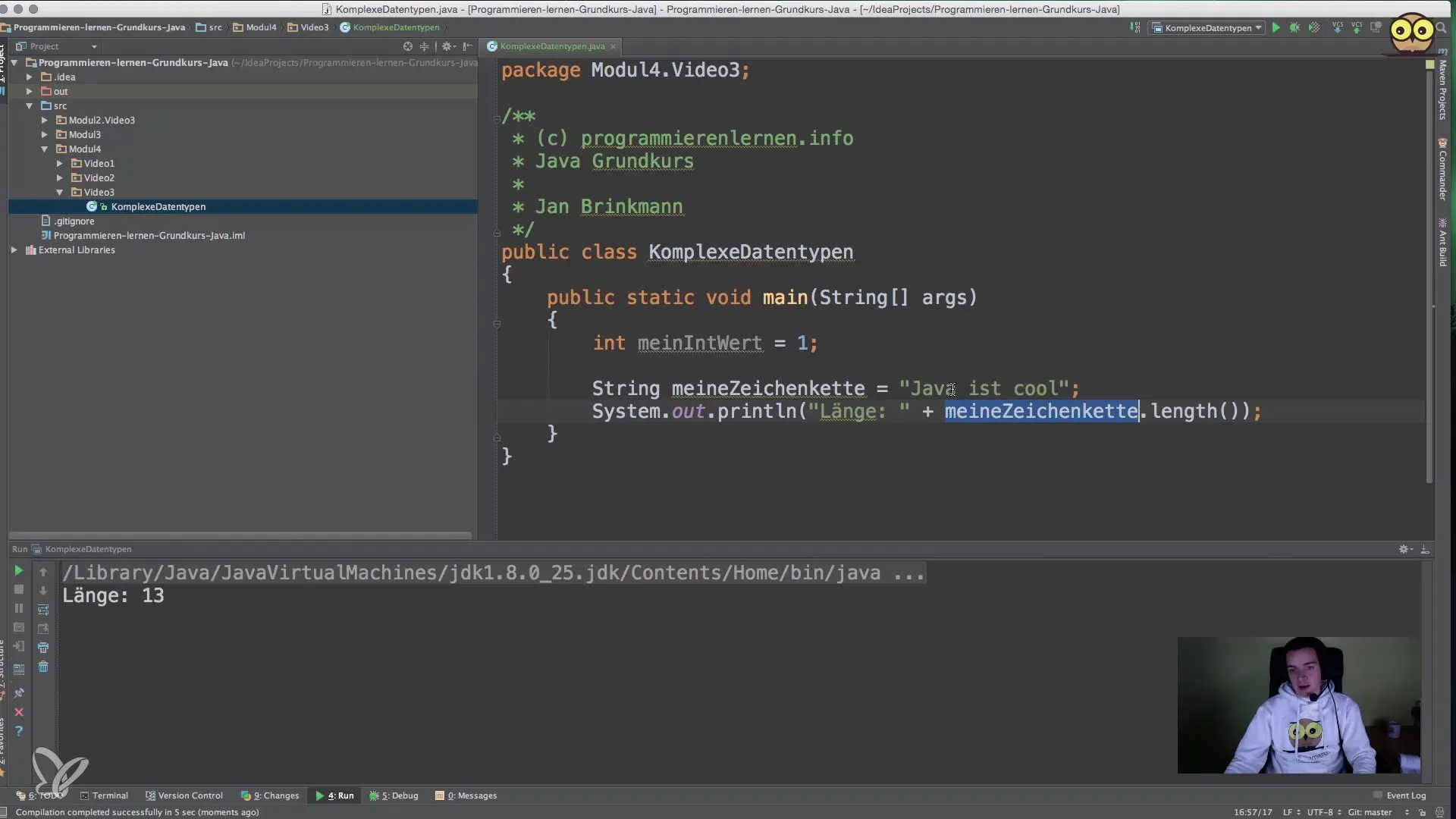
7. Introduction to wrapper classes
Java provides wrapper classes for all primitive data types. You will learn how to create an instance using the wrapper class Integer that allows you to treat the int value as an object.
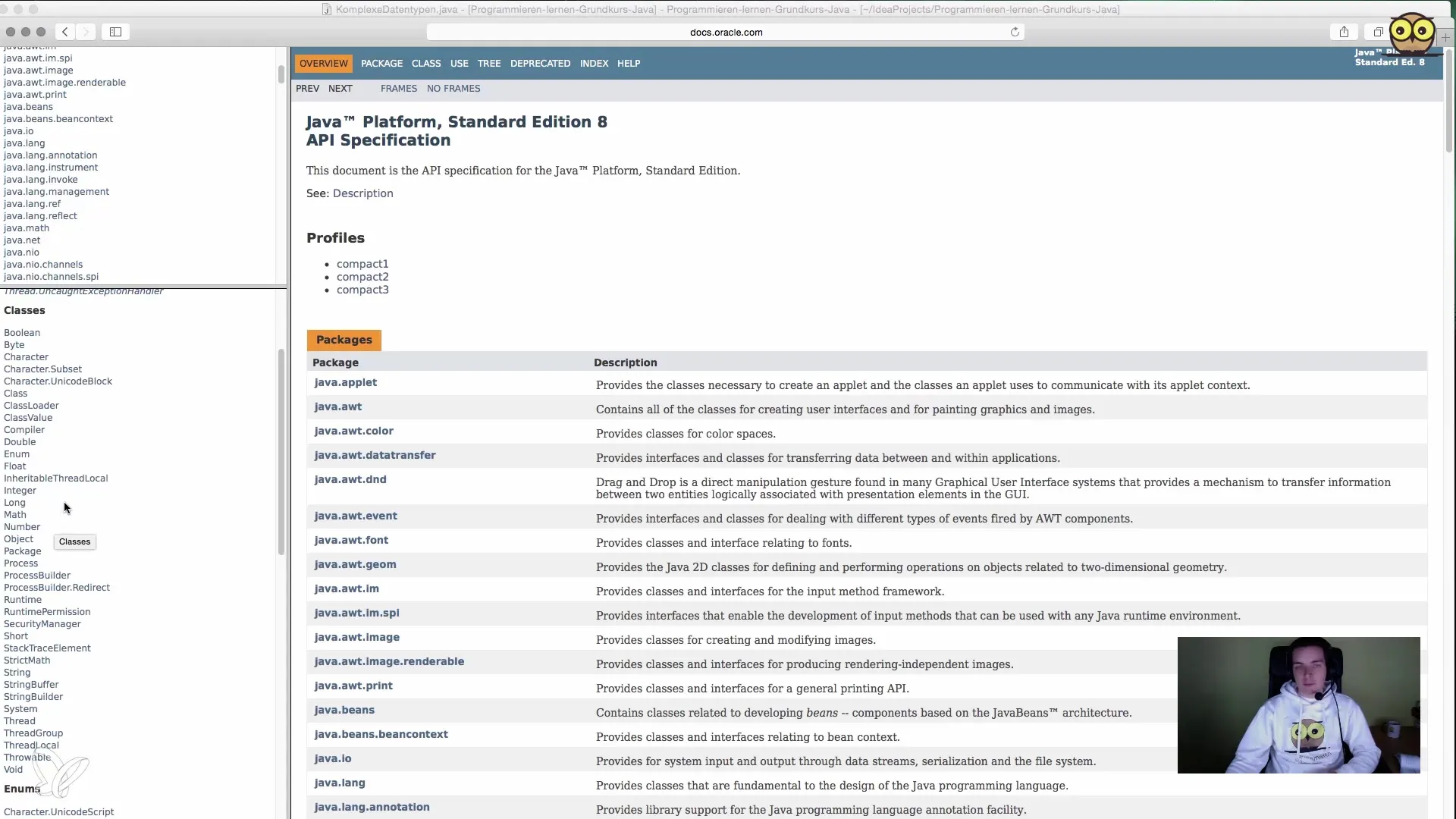
8. Create a wrapper object
You can create a wrapper object by instantiating the Integer class. This allows you to call additional methods that help you obtain the value as a Double or other formats.
9. Output as Double value
If you use a method like doubleValue() for your Integer wrapper object, you can output the value as a Double. This is particularly useful when working with different data types in your calculations.
Summary – Learning about complex data types in Java
Complex data types are an essential element in Java as they enable you to work with methods and properties that go beyond simple values. By using wrapper classes, you can extend primitive data types and leverage the benefits of objects. Understanding and effectively utilizing these data types is crucial for programming in Java.
Frequently Asked Questions
What are complex data types in Java?Complex data types are classes that enable the use of methods and properties based on primitive data types.
How do primitive and complex data types differ?Primitive data types only store values, while complex data types provide methods and functionalities.
What is a wrapper class?A wrapper class is a class that converts a primitive data type into an object, providing additional methods and functionalities.
Why do I need wrapper classes?Wrapper classes allow you to handle primitive data types as objects and give you access to useful methods.
How do I use complex data types in Java?Complex data types are defined by classes like String or wrapper classes like Integer and provide methods to efficiently process data.