If you want to work with graphical user interfaces in Java, you are exactly right here. In this section, we will learn the basics of responding to user input and expand a simple program that reacts to button clicks. Through the practical examples, you will understand how to handle inputs in your Java application project.
Key Insights
- Handling JFrame and JPanel to create a user interface.
- Implementing event listeners to respond to user interactions.
- Using ActionListener to respond to button clicks.
- Applying structured programming to organize your Java application.
Step-by-step Guide
First, you should ensure that your development environment is ready for programming in Java. After setting up your project, follow this guide to respond to inputs.
1. Create a Main Class
Start by creating a main class that extends JFrame. This allows you to create a window that represents your application. Set the necessary components and pass the title via the constructor.
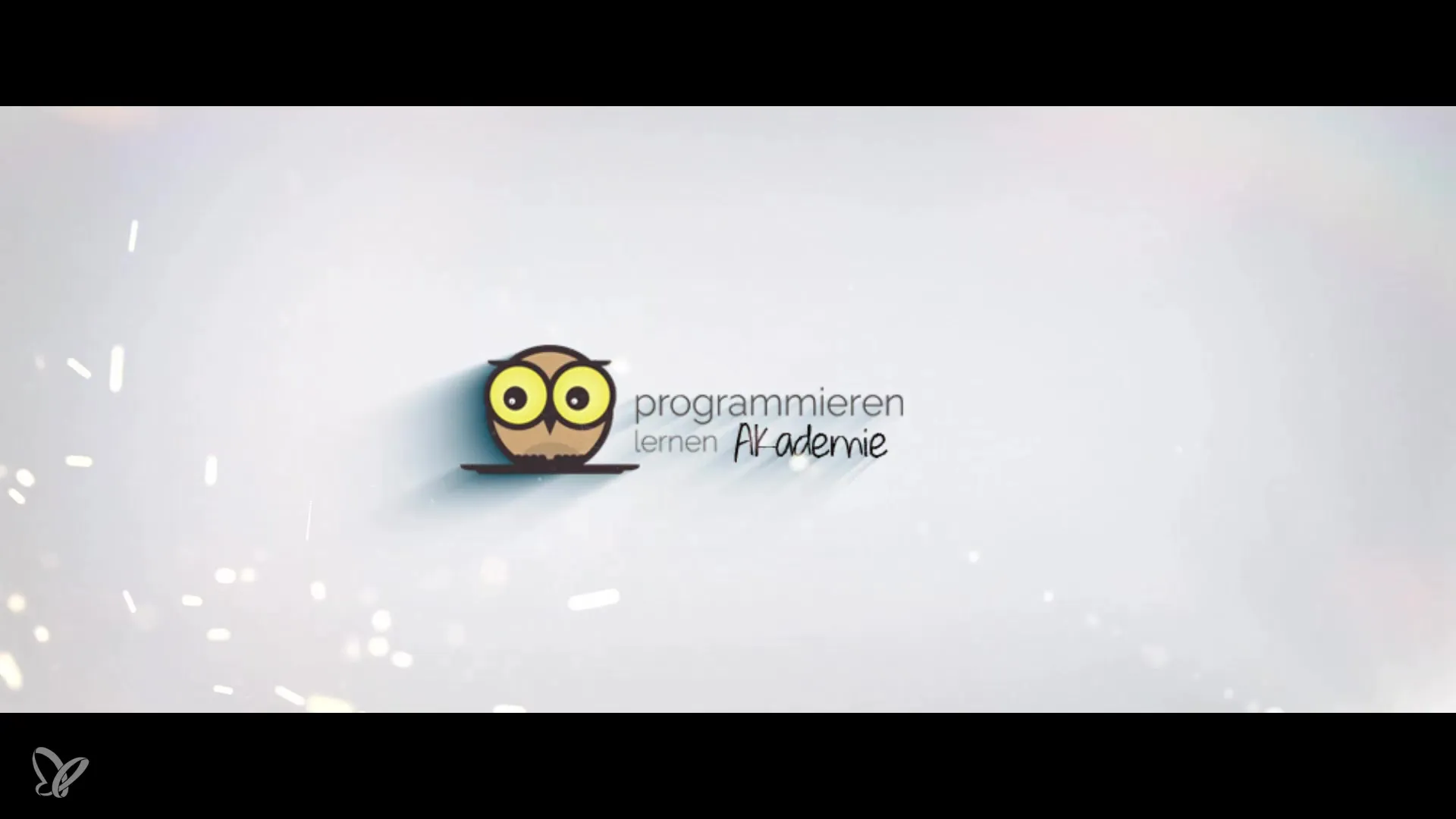
Make sure your JFrame is set to visible by calling setVisible(true) in the constructor.
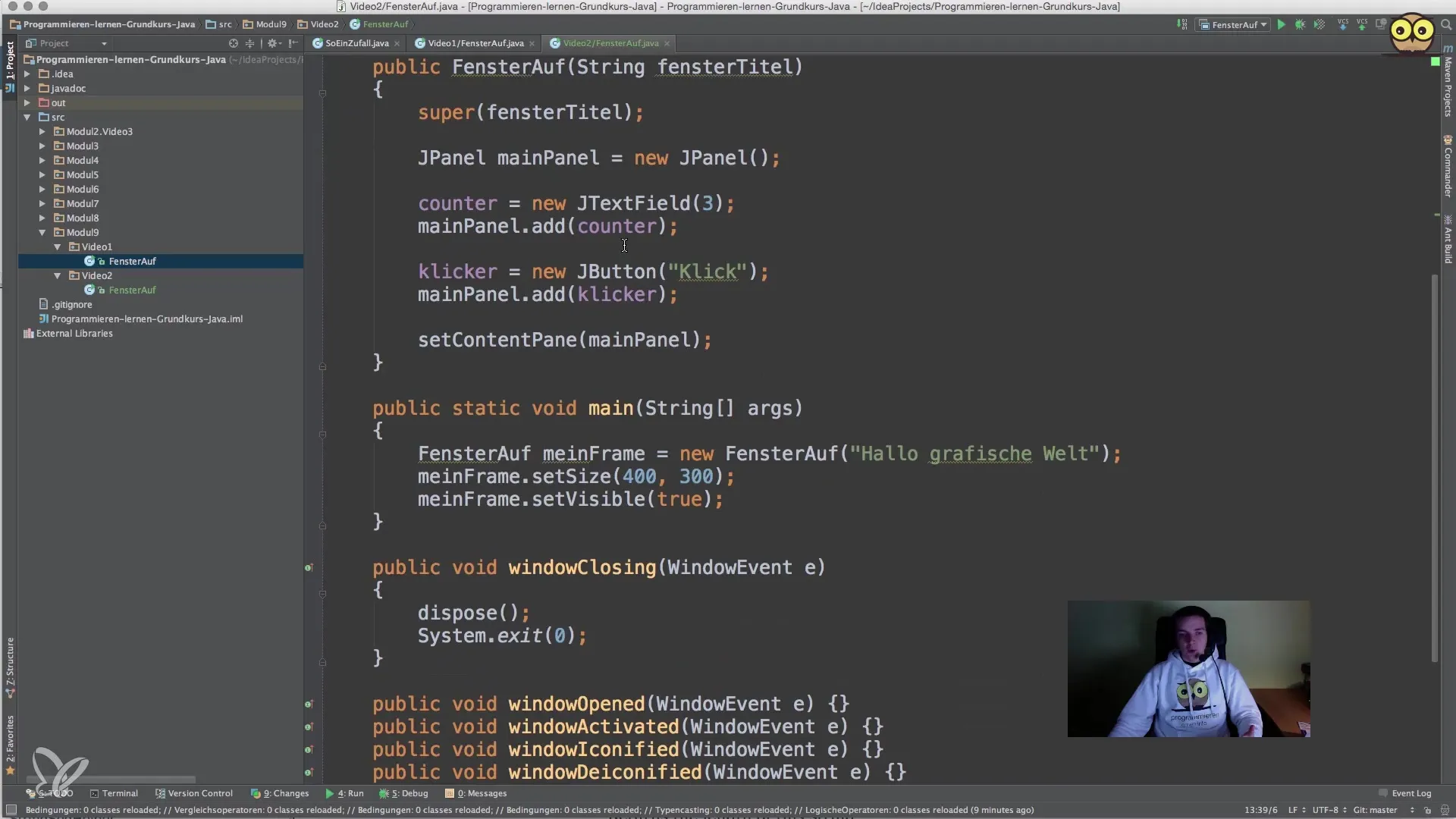
2. Adding a Panel
Create a JPanel that serves as a container for the controls you want to add. These components can be text fields, buttons, or other GUI elements.
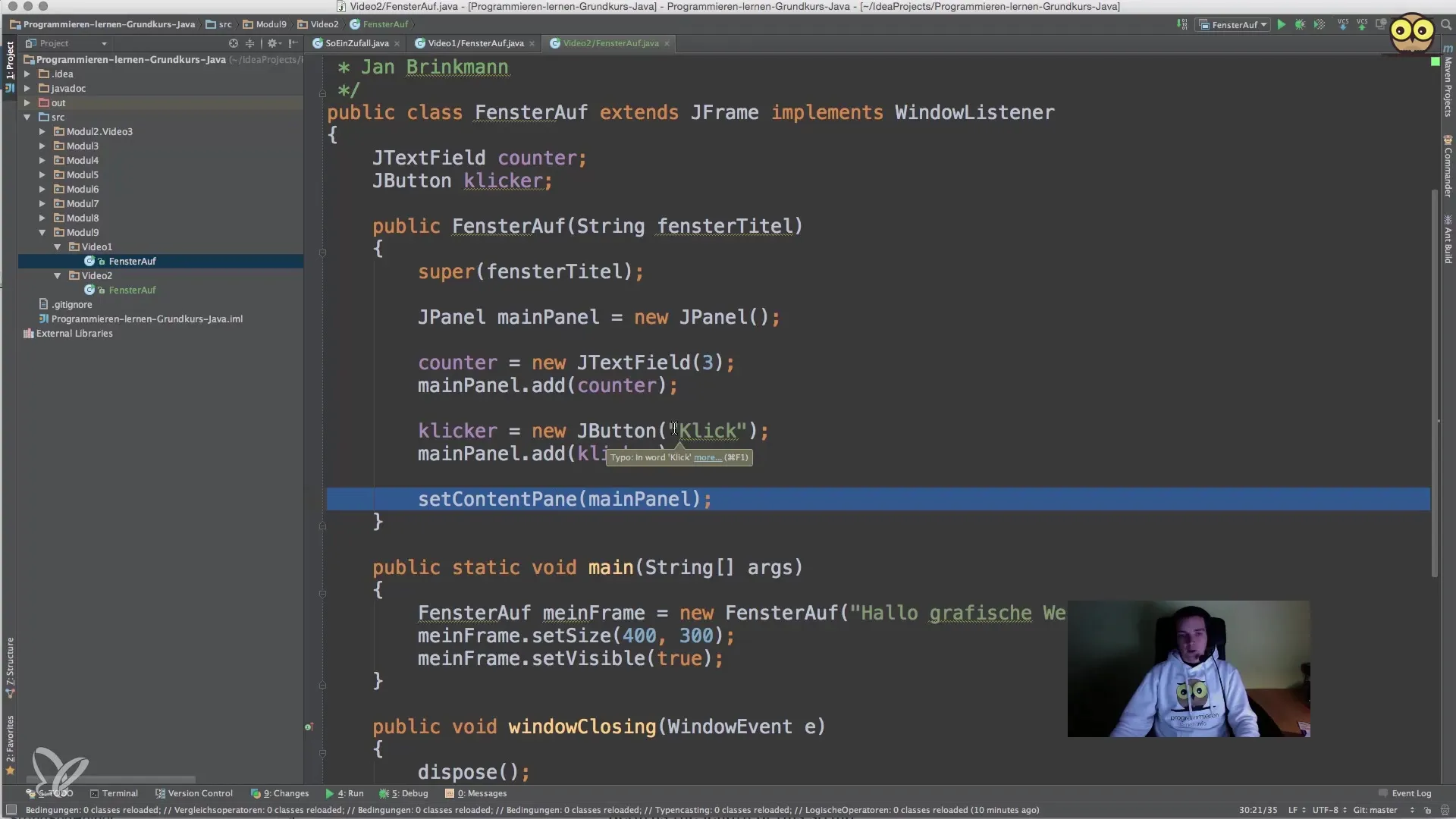
3. Creating a Counter and a Button
Choose a variable to count the clicks. In our case, we use an int type to store the number of clicks.
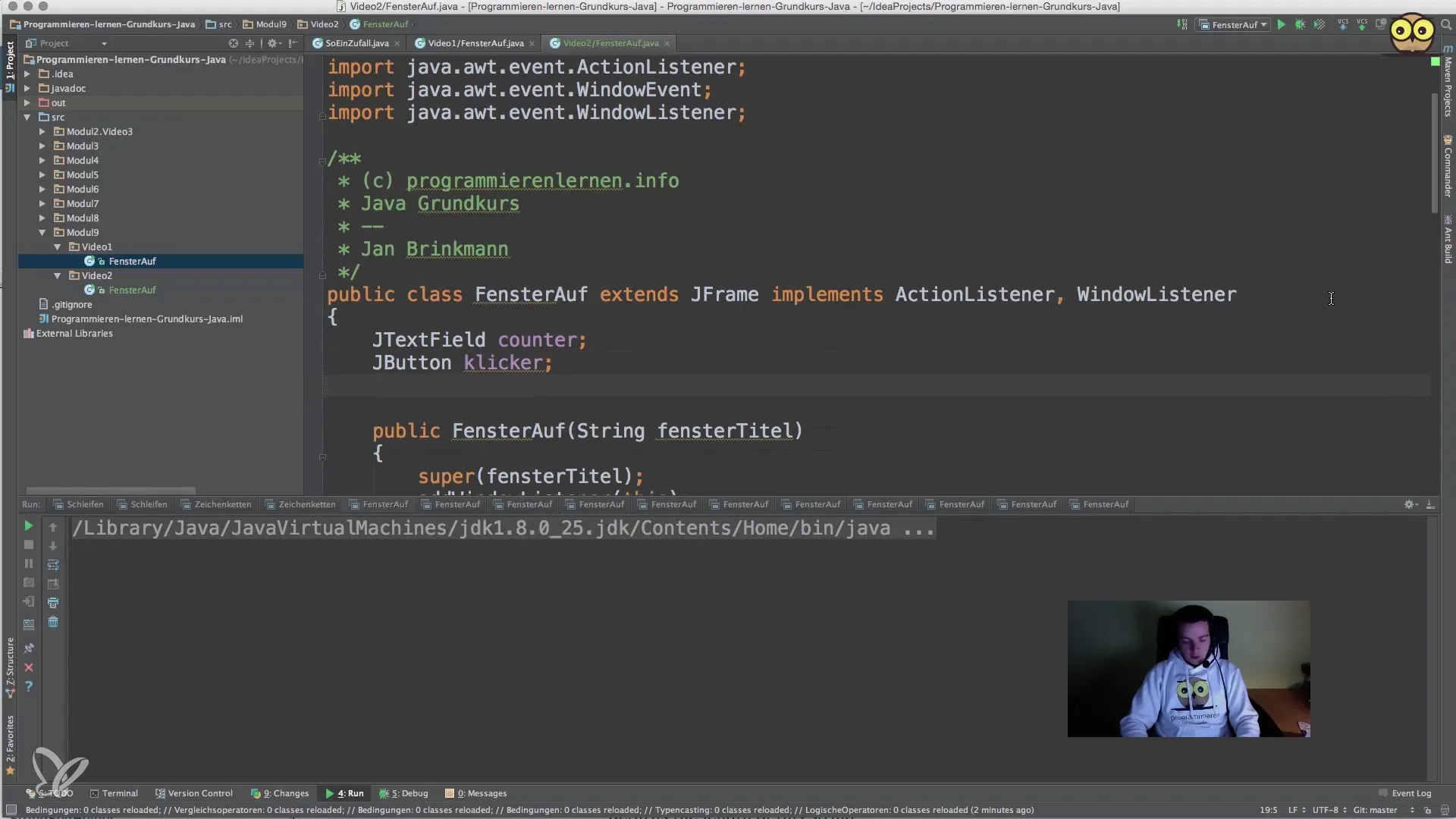
Initialize the counter in the constructor of the class to ensure it is set to zero when the application starts.
4. Implementing the ActionListener
To respond when the button is clicked, you need to link the button with an ActionListener. This listener will be called when the button is pressed.
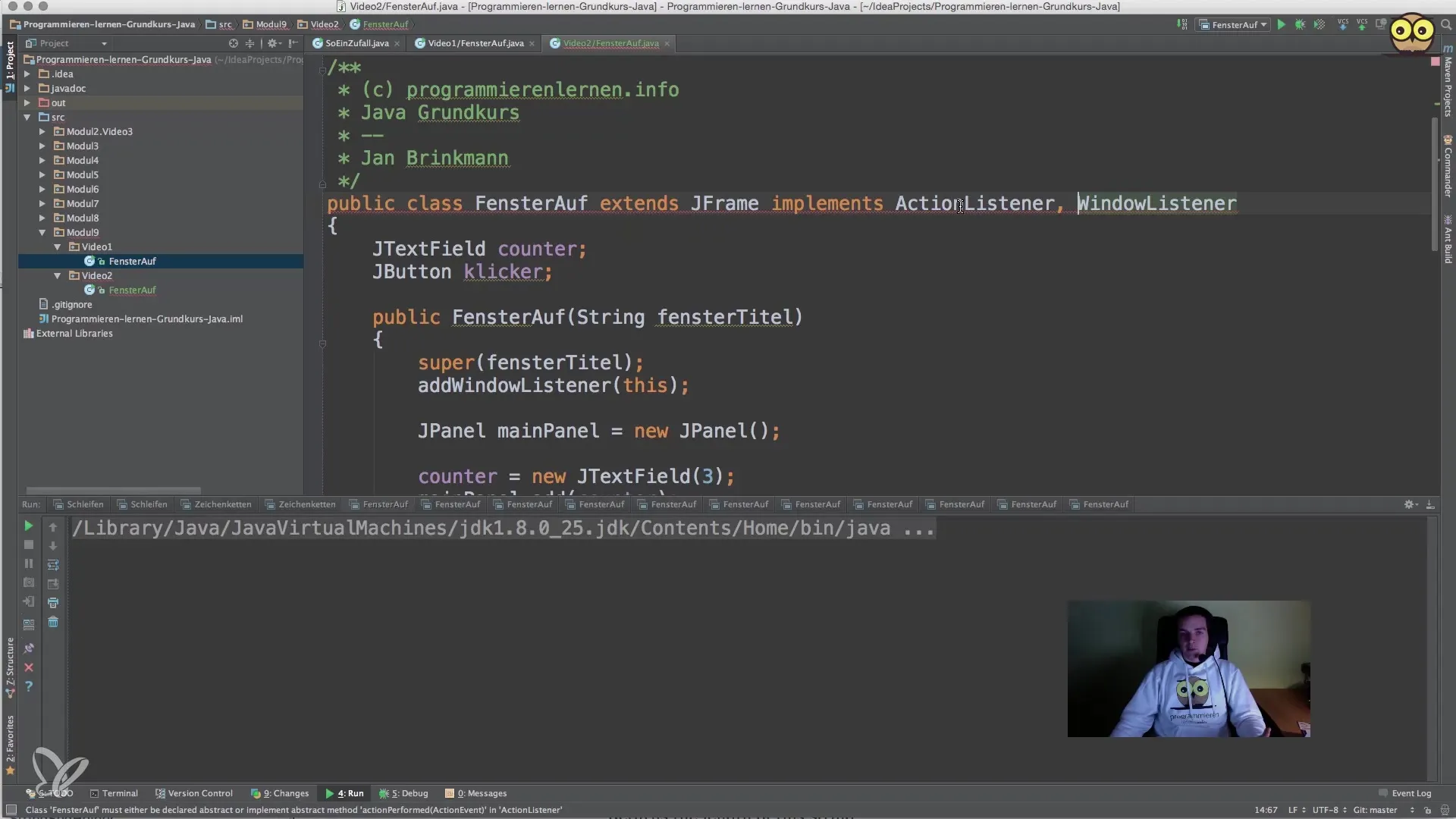
5. Displaying the Counter in the Text Field
Create a text field that displays the current value of the counter. You can update the content of the text field when the button is clicked.
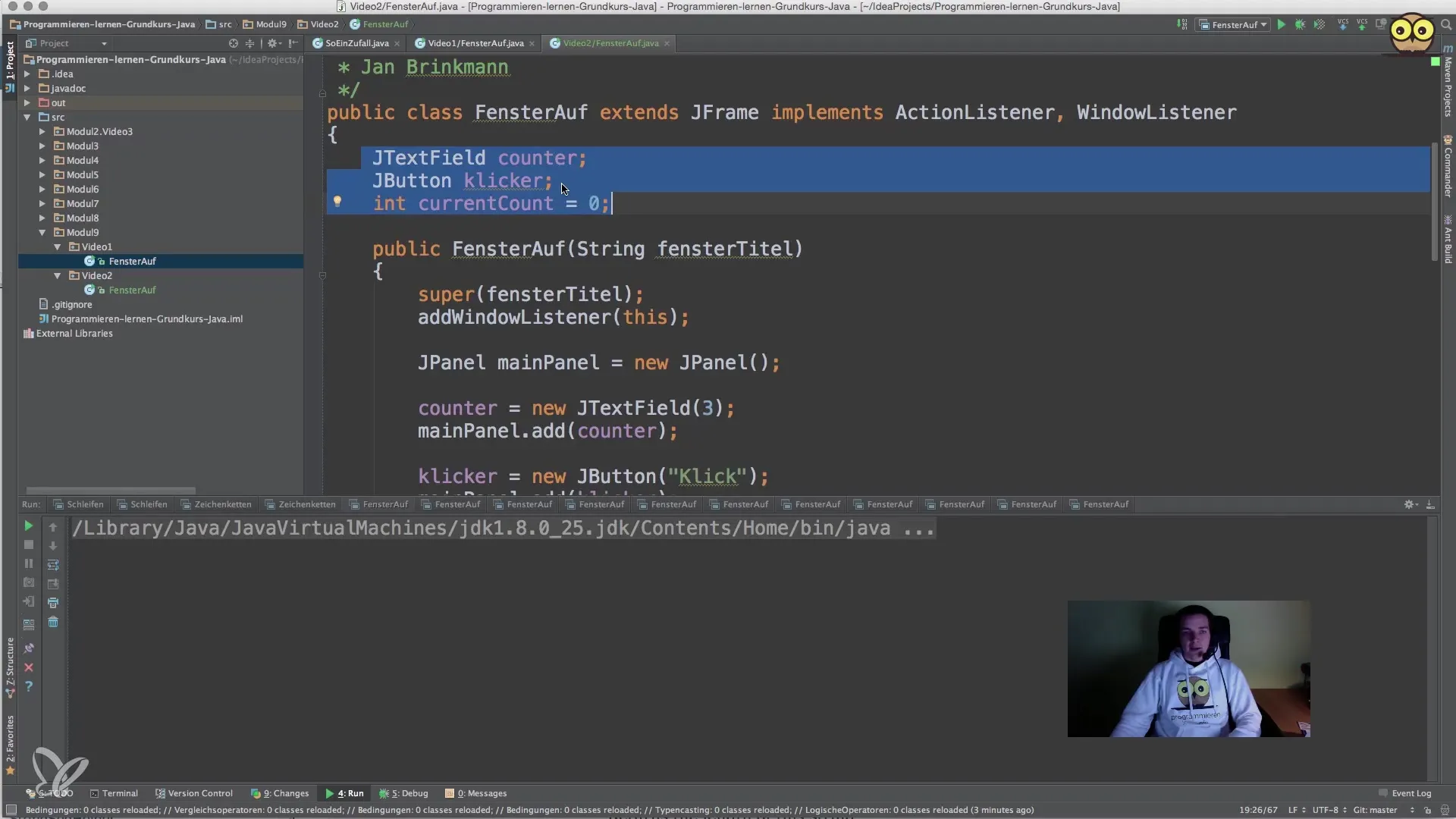
6. Managing the Closing of the Window
To ensure that the Java process is terminated when the window is closed, you should implement the WindowListener. Define what happens when the close icon (x) in the title bar of the window is clicked.
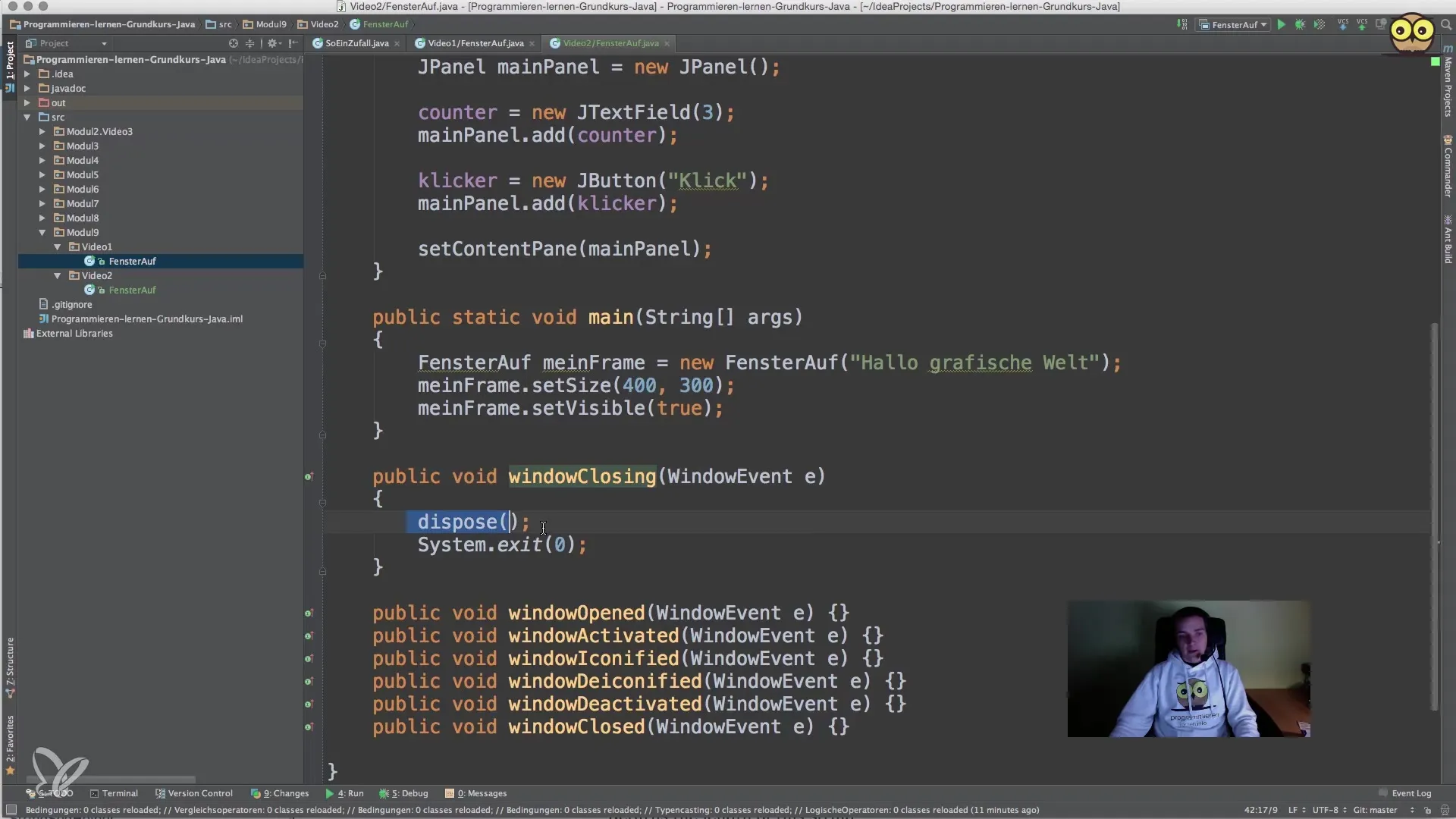
7. Testing the Application
Run your program and test if the button clicks are correctly counted and displayed in the text field. Click the button and observe how the counter increases.
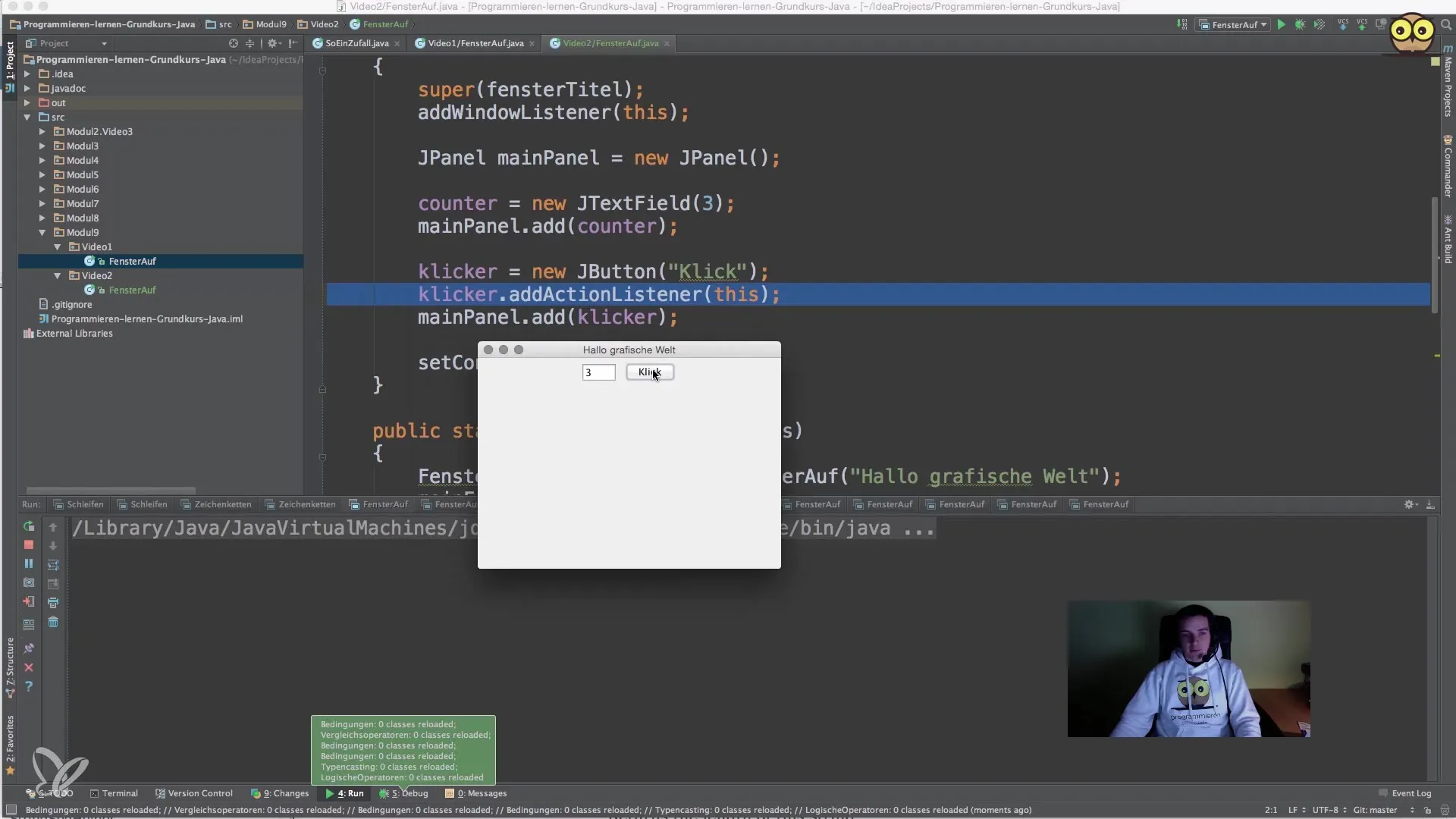
If you implemented everything correctly, the value should increase with each click. You have thus successfully responded to inputs in your Java application.
Summary – Java for Beginners: Responding to User Inputs
You have learned how to create a simple Java application that responds to user inputs. You were able to create a window, implement button and text field interactions, and manage the state of your application. These foundational concepts form the basis for more complex applications that you can develop in the future.
Frequently Asked Questions
How do I implement a button in a Java GUI?You can create a button using the JButton class and add it to your JPanel.
What is an ActionListener?An ActionListener is used to respond to actions such as button clicks and defines what should happen when the action occurs.
How can I display the counter in the text field?Set the text of the JTextField to the current counter value using the setText() method.
What happens when I close the window?If you use setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE), the application and the Java process will be terminated when the window is closed.
How can I add multiple buttons?Simply create multiple JButton instances and add them to your JPanel, each with its own ActionListener.