Before you dive into the fascinating world of programming, it is crucial to understand the concepts of code blocks and scopes – also known as "Scopes". These fundamentals are not only important for Java, but for many programming languages. This knowledge becomes especially important when you come from another programming language, as there are often specific challenges waiting here. Let’s explore together how code blocks are structured and what rules apply to scopes.
Main insights
- Code blocks are groups of statements enclosed in curly braces {}.
- The scope of a variable determines where it can be used in the code.
- Variables defined within a code block are only visible within that block, but an inner block has access to the variables of the outer block.
Step-by-Step Guide
Step 1: Definition of code blocks
You can think of code blocks as containers for your statements. In Java, they are always enclosed by curly braces. A simple example is the main method, where your code is executed. Any code within the curly braces of this method will be executed in sequence.
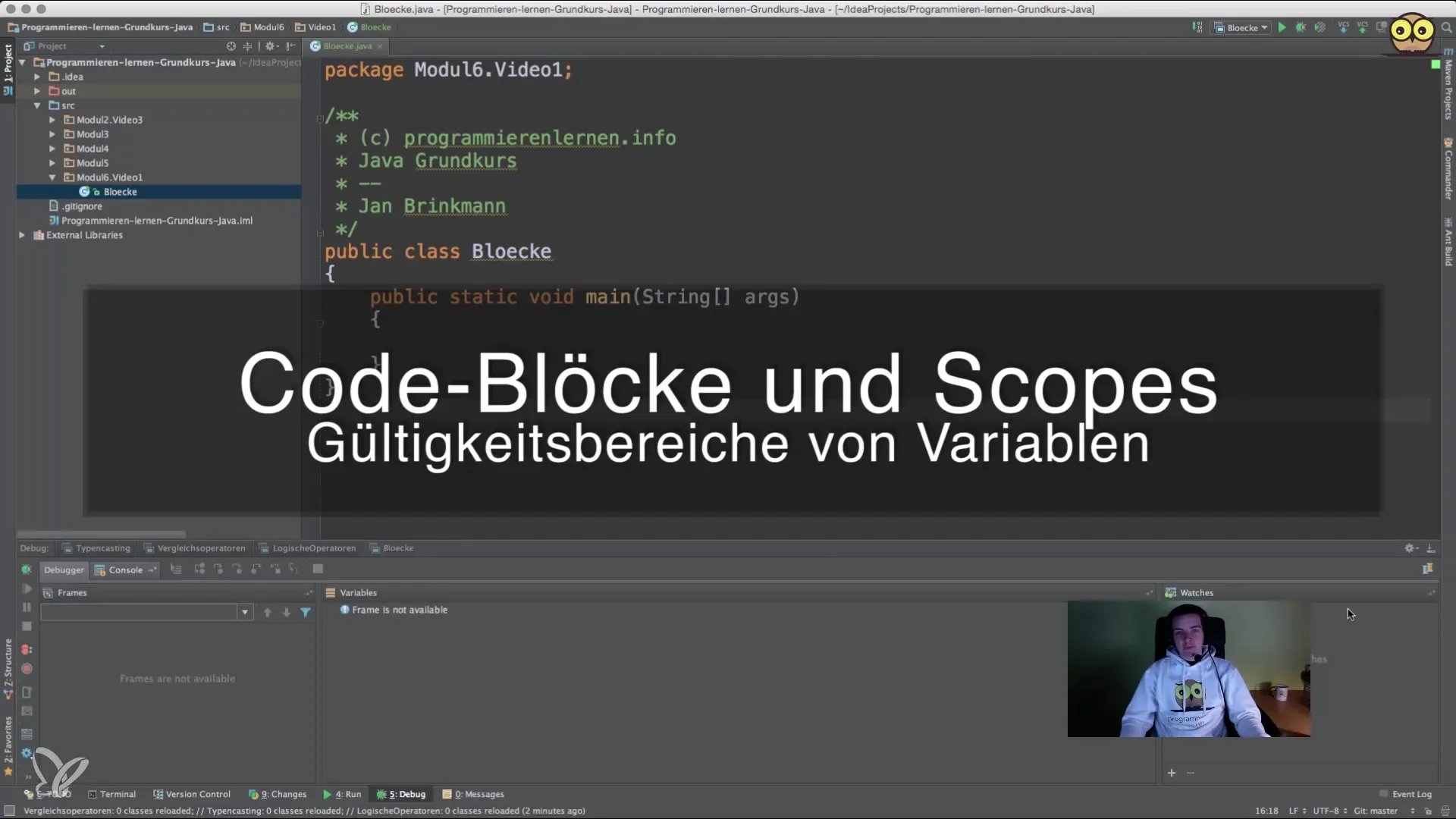
Step 2: Executing code in the main method
For example, if you place a simple print statement like System.out.println("first line"); in the main method, this line will be printed. If you have multiple statements, each will be executed in the order they are defined.
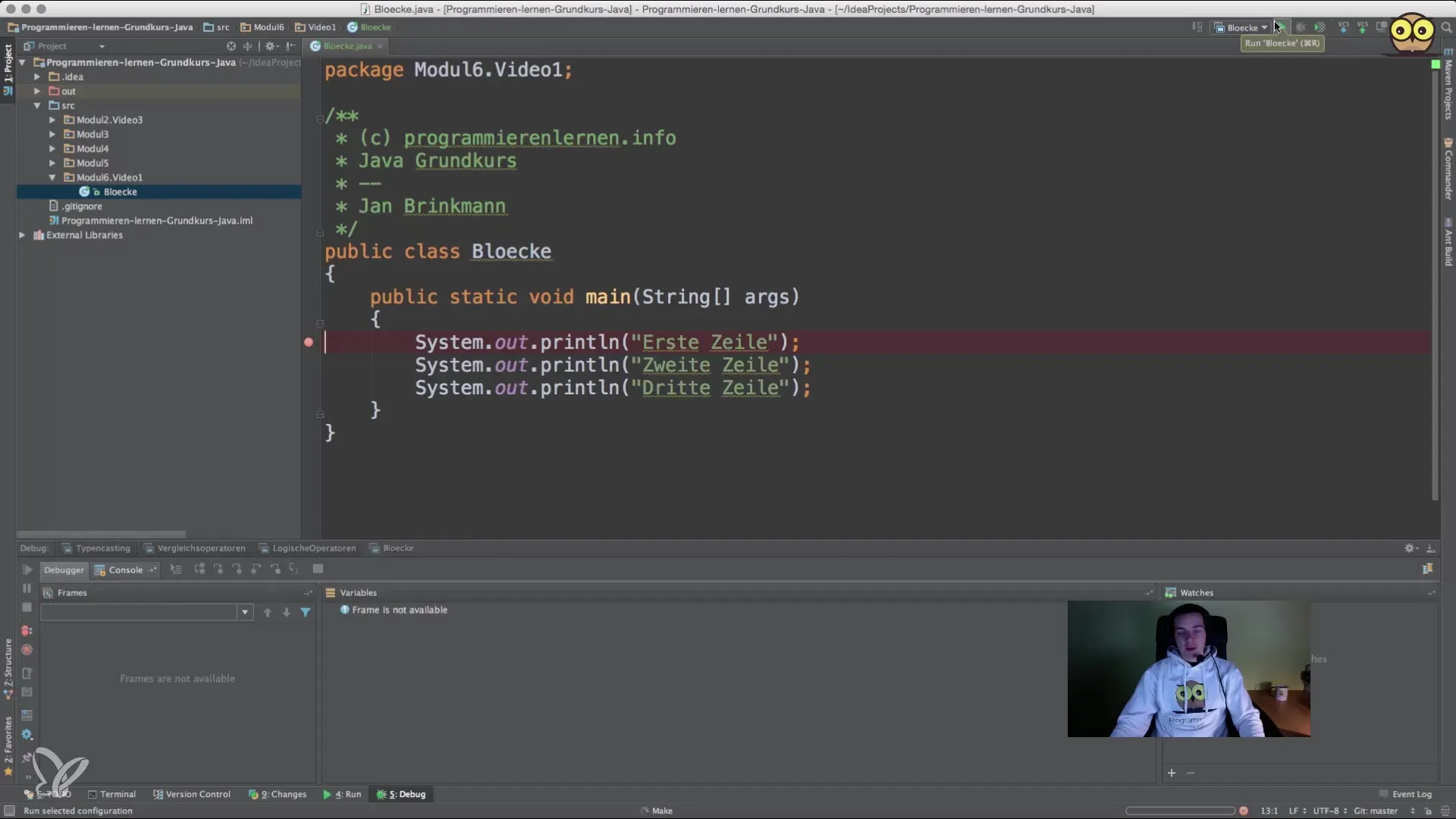
Step 3: Understanding scopes
A crucial aspect in Java is the scope of variables. When you define a variable within a block, it is only valid in that block. For example: If you define a variable int lineNumber = 1; within the main method, you can only access this variable within that method.
Step 4: Creating conditions
You can also insert conditions into your code to control the logic. For example, if you want to check if a number is smaller than another, you use an if condition. In an example, you could use if (2 < 3) {...} to enter a specific code block.
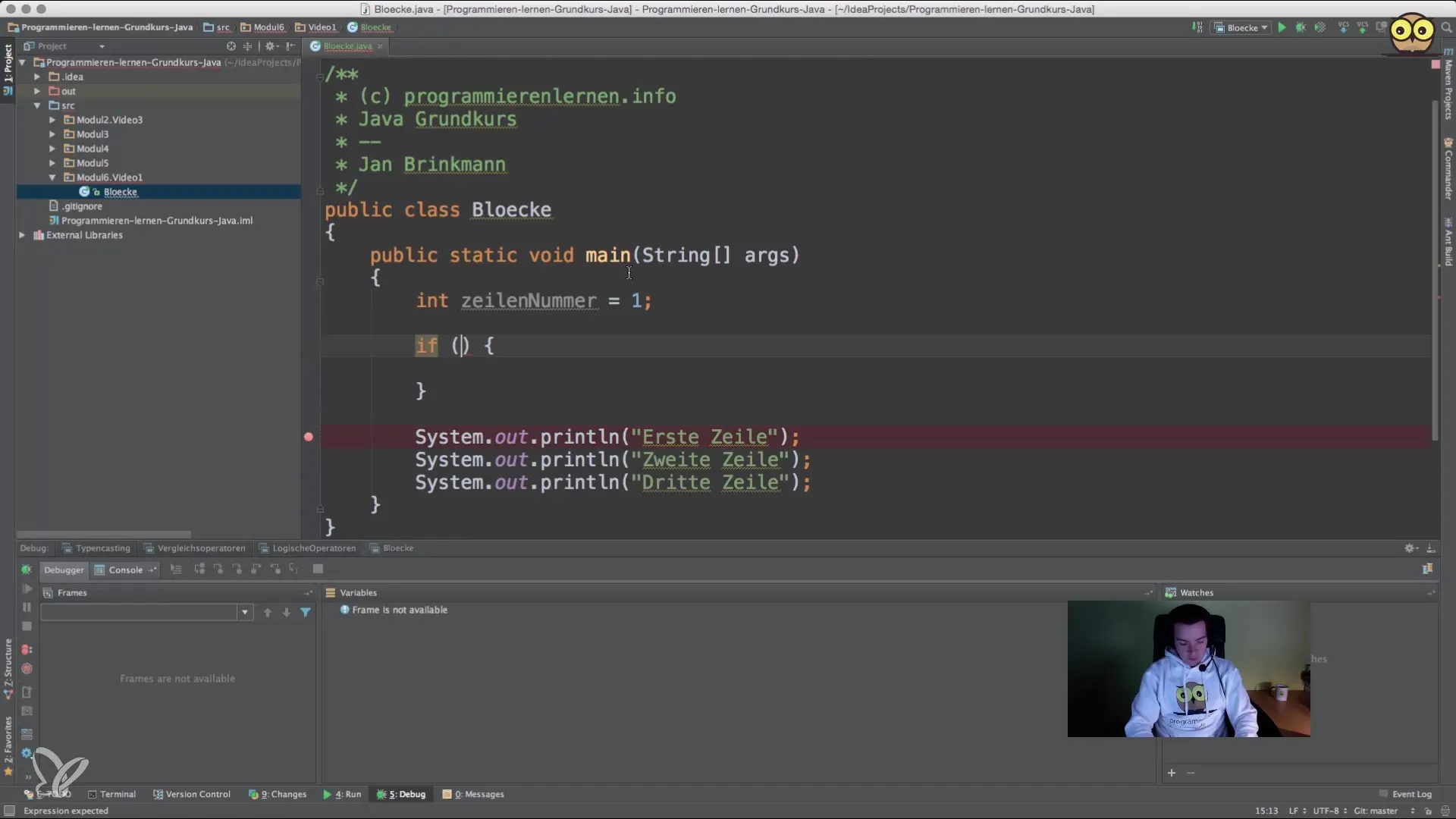
Step 5: Working with variables within blocks
Within a block, such as in an if statement, you can also define variables. However, these variables are only visible in that block. If you try to access this variable from outside, it will not work.
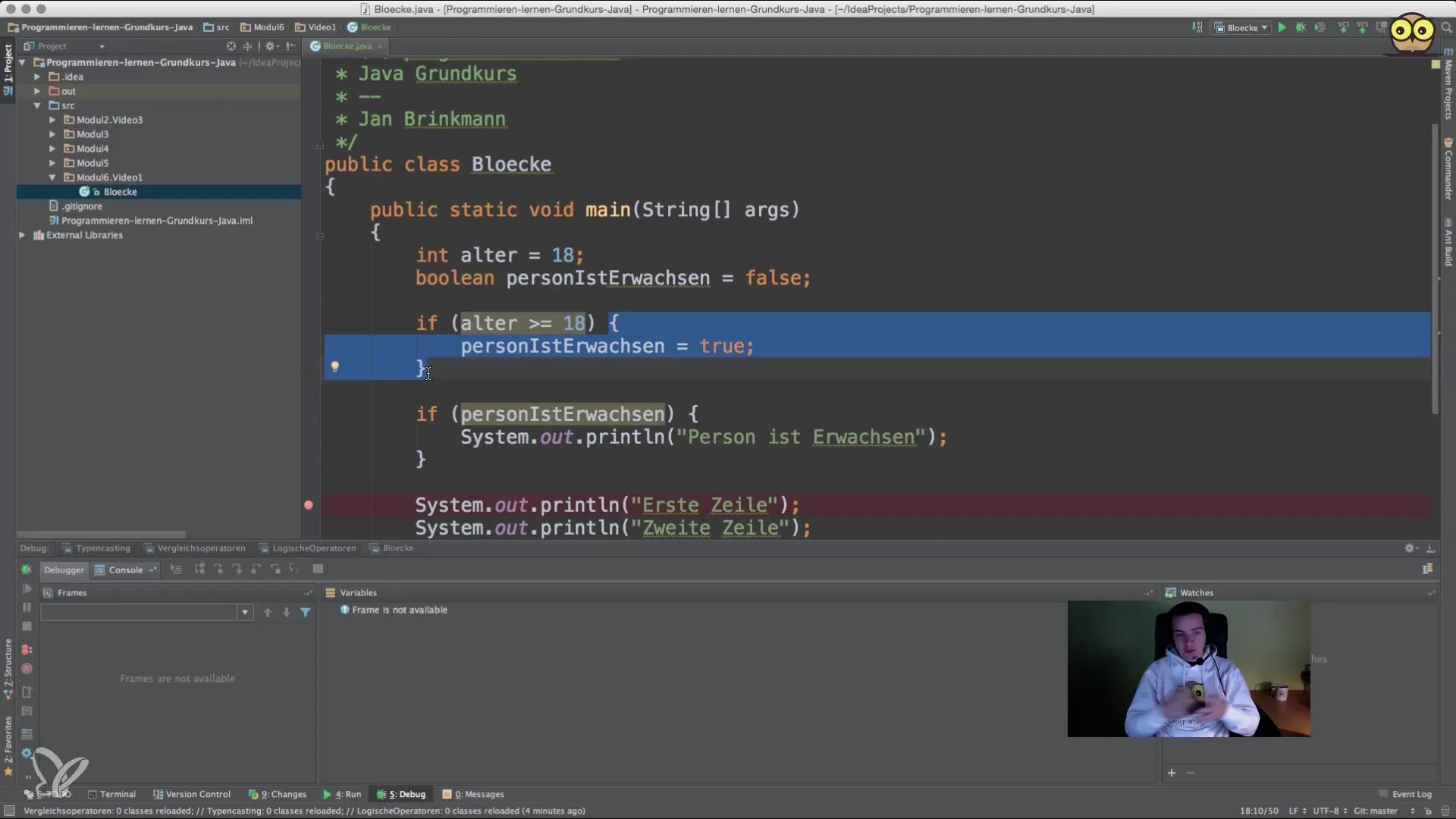
Step 6: Delegating values between blocks
Understanding access to variables between blocks is essential. An inner block can access the variables of its parent block, which makes handling values between different control structures easier. You could set the value of a variable in an inner block and then use it in the outer block.
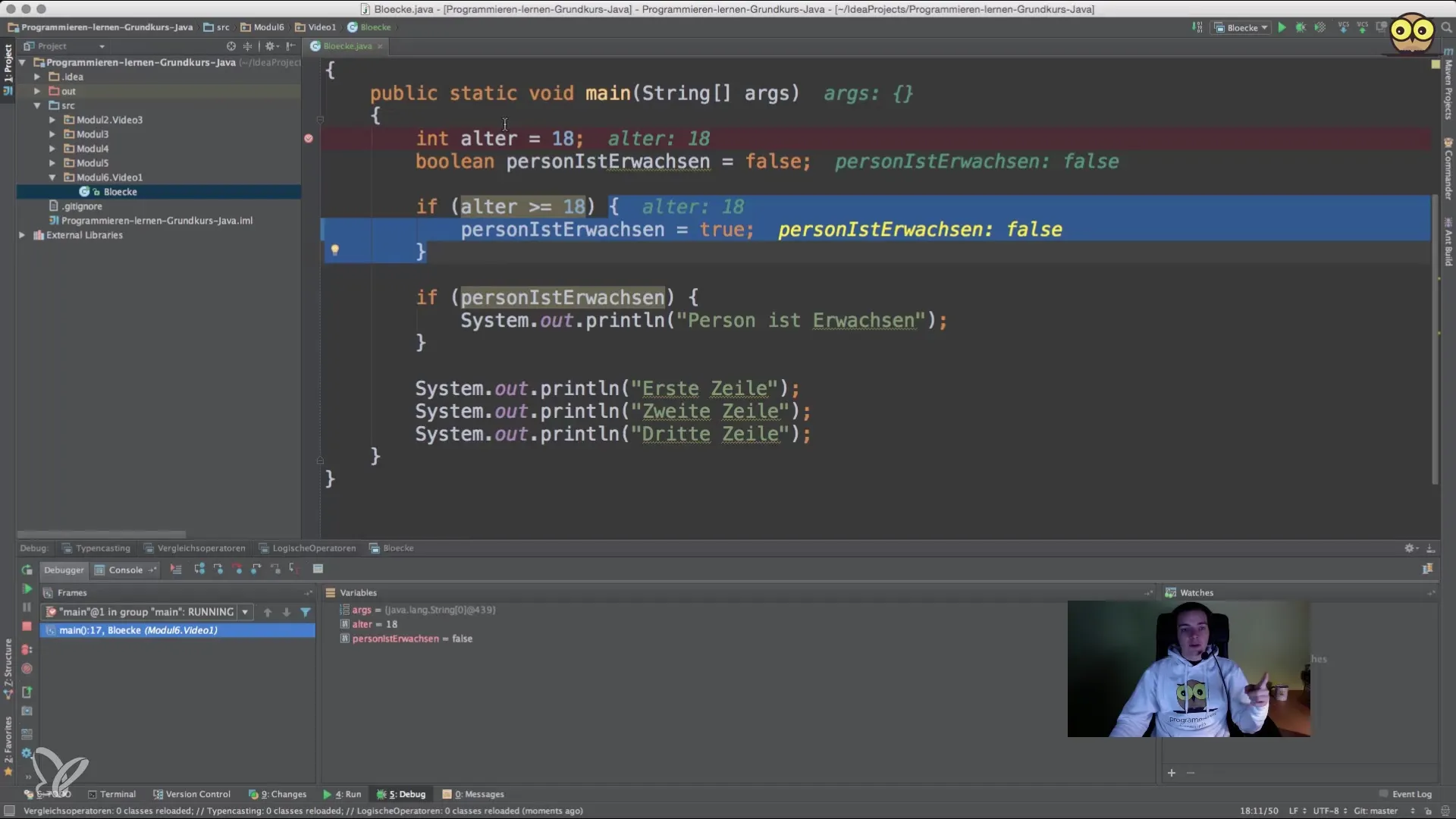
Step 7: Errors with variable visibility
Deliberate limitations on visibility can lead to errors in your code. For instance, if you try to access a variable defined within an inner block, it will not be recognized in the outer block. This can be useful for making certain variables available only within a specific scope.
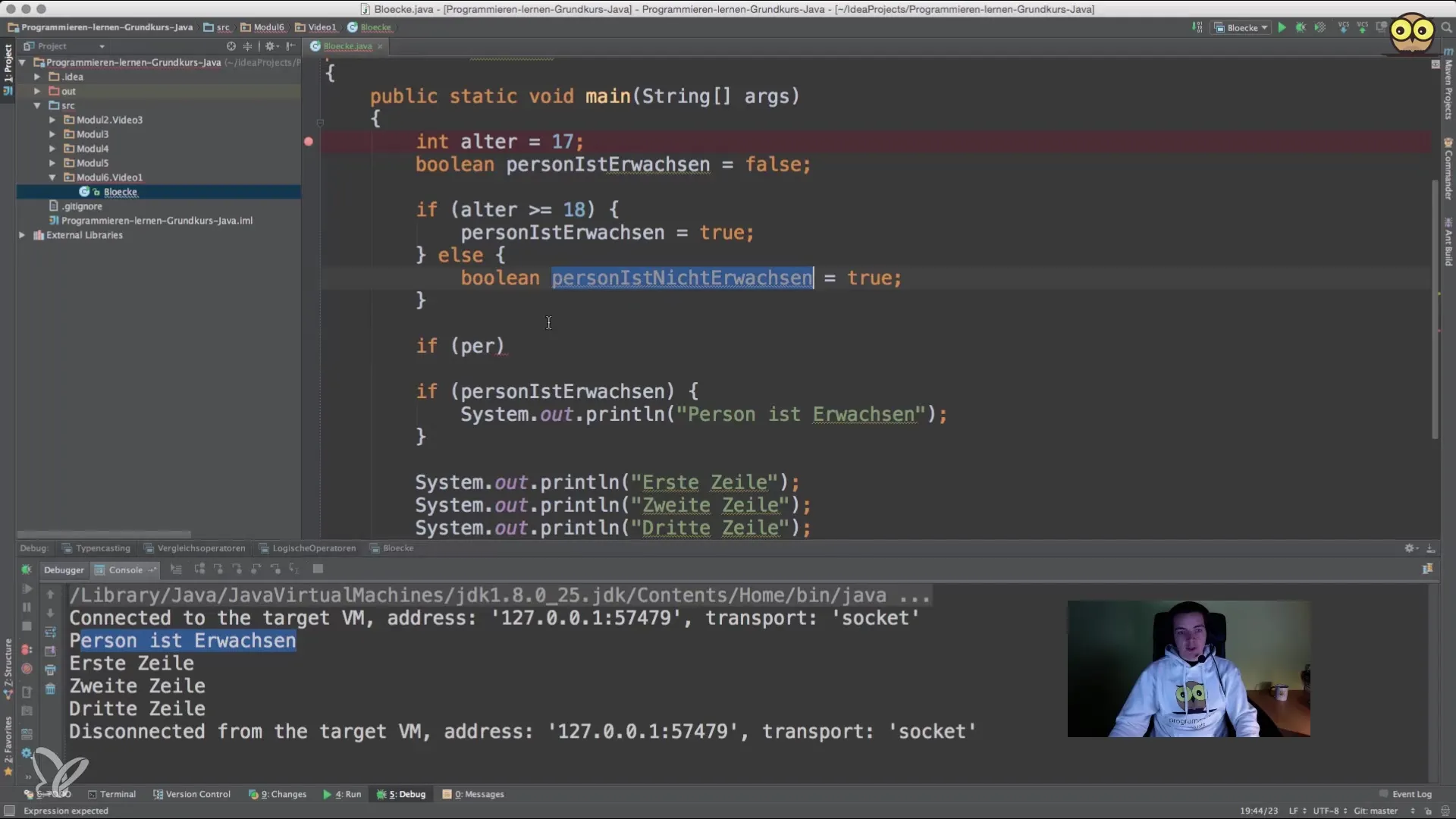
Summary – Understanding code blocks and scopes in Java
By understanding the structure and logic of code blocks and scopes, you lay a solid foundation for your Java programming. The practice of using blocks and understanding scopes enables you to write clearer, safer, and better-structured code.
Frequently Asked Questions
How do I define a code block in Java?A code block is defined by curly braces {}, inside which statements are written.
Can I access variables within an inner block from an outer block?Yes, an inner block has access to variables defined in its outer block.
What happens if I try to access a variable outside its scope?You will receive a compilation error, as the variable is not visible outside its defined scope.
How can I control the scope of variables?You can control the scope by defining variables in different code blocks.
Are code blocks only available for methods?No, code blocks can also be defined in other control structures, such as if statements and loops.