In object-oriented programming, interfaces play a crucial role, especially when it comes to writing well-structured and maintainable code. Interfaces help organize and define the interactions between the various parts of a software solution, specifying which methods a class must implement to fulfill the desired contract. In this text, you will learn what Java interfaces are, how they work, and how you can implement them in your own projects.
Main Insights
- An interface defines which methods a class must implement without providing the implementation itself.
- Interfaces promote the modularity and reusability of code.
- You can create multiple classes that implement the same interface, with each class having its own specific logic.
Introduction to Interfaces
Interfaces in Java are like contracts between classes. When a class implements an interface, it commits to providing a specific set of methods. This allows you as a developer to ensure that all classes implementing this interface offer the same set of methods without having to worry about the specific implementation.
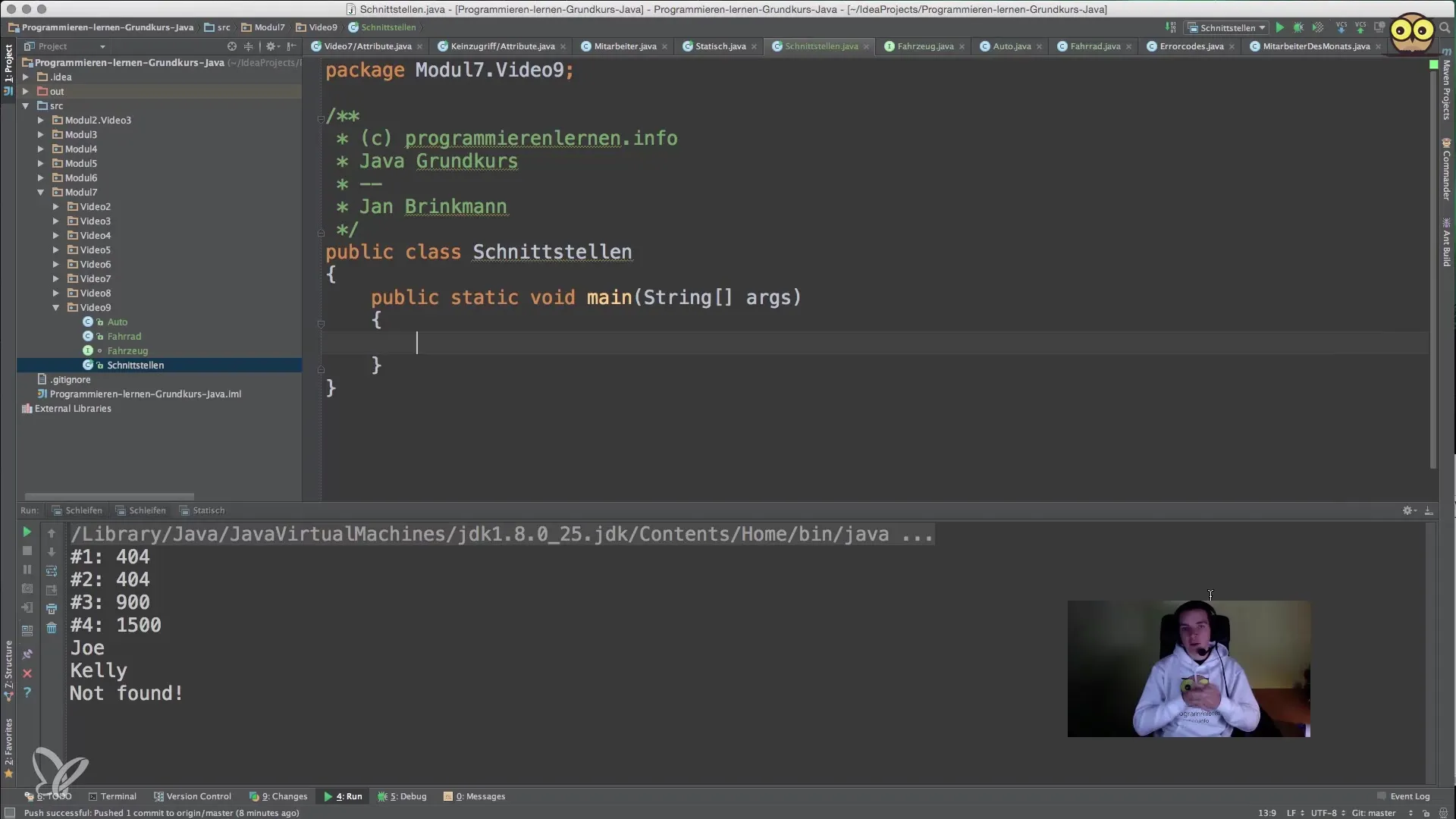
Definition of an Interface
To create an interface in Java, you use the keyword interface. An interface contains only method declarations and no implementations. This means that you must provide the logic of the methods in the classes that implement the interface.
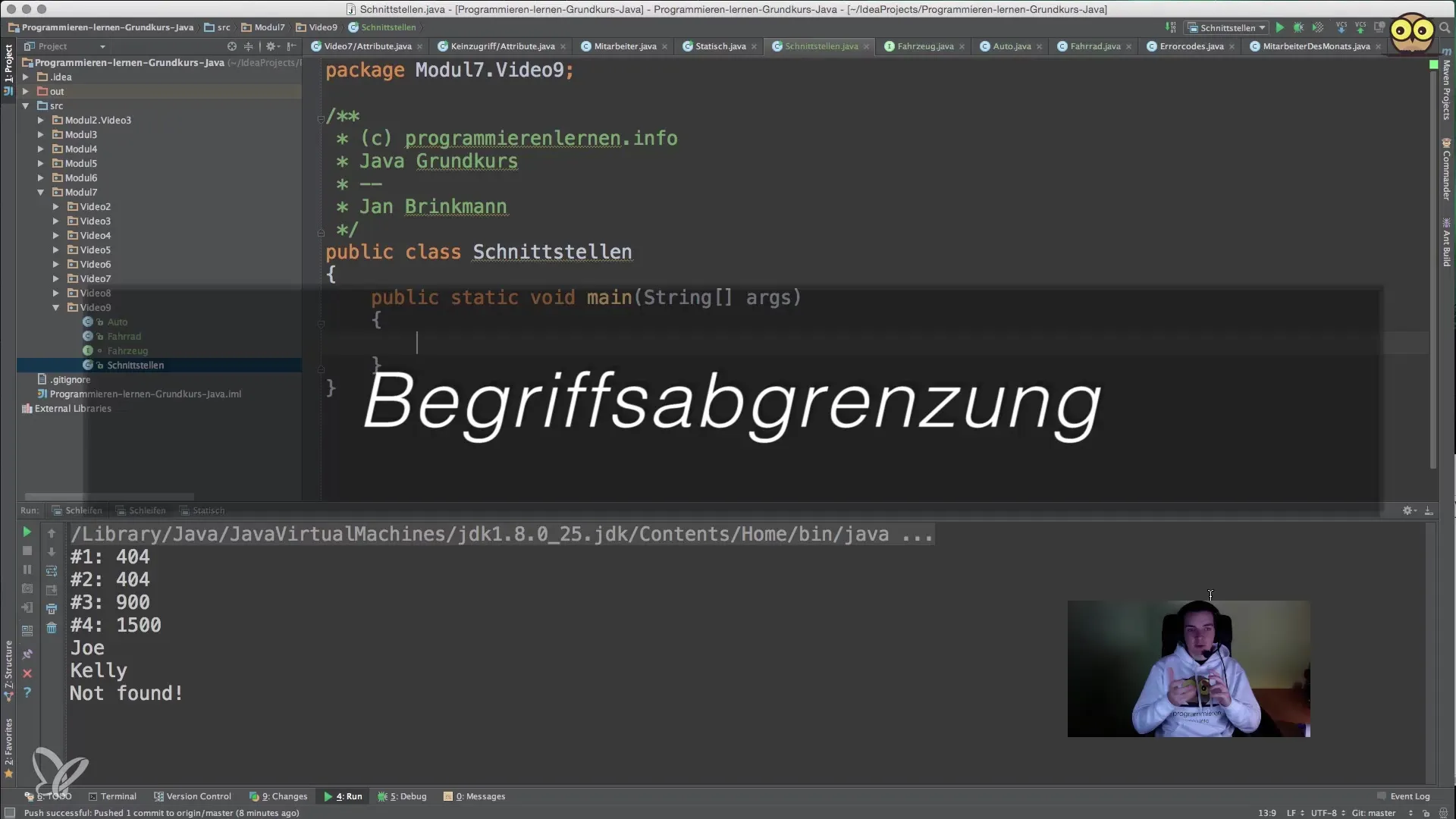
In this example, an interface Vehicle is created, defining two methods: accelerate() and brake().
Implementing an Interface in a Class
After defining an interface, you can implement it in a class. You use the keyword implements for this.
Using Interfaces
A significant advantage of interfaces is the flexibility they offer. You can create multiple classes that implement the same interface, and each of these classes can provide the same methods with different implementations.
Here, you have a way to use both bicycle and car while still leveraging the methods accelerate and brake, without having to worry about the specific implementation of the class.
Generalized Methods with Interfaces
Another advantage of interfaces is that you can create methods that only accept objects of a specific interface. This enhances the modularity and maintainable structure of your software.
Example of a General Method
Now you can address both a car and a bicycle through this method. This gives you the flexibility you need in your code to handle different vehicle types.
Conclusion
Interfaces are a central concept in Java that help you to keep your code clear, efficient, and maintainable. They allow you to define a contract between different classes, ensuring that those classes implement certain methods. The result is a well-structured code that is easy to maintain and extend. With the knowledge of interfaces, you can sustainably improve your software architecture and better respond to your project's requirements.
Summary — Fundamentals of Java Interfaces
In this guide, you learned:
- What interfaces in Java are and what they are used for.
- How to define an interface and implement it in classes.
- The possibilities that arise from using interfaces to write flexible, maintainable code.
Frequently Asked Questions
What is an interface in Java?An interface is a contract that contains defined methods that must be provided by implementing classes.
Why should I use interfaces?Interfaces promote modularity and reusability of code and simplify the maintenance and extension of software projects.
Can a class implement multiple interfaces?Yes, in Java a class can implement multiple interfaces, allowing for very flexible software design.
What happens if a class does not fully implement the interface?The class must be declared as abstract, or a compilation error will be generated.
How does an interface differ from an abstract class?An interface can only contain methods without implementation, while an abstract class can contain both methods with and without implementation.