Working with conditions in Java is one of the fundamental concepts of programming. In fact, most software solutions are based on decision structures. Here you will learn how to efficiently use conditions in Java. This guide will help you deepen your understanding of conditions and expand your Java know-how.
Key Insights
- Conditions are essential for the logic of programs.
- The order of conditional checks plays a significant role.
- The use of if, else if, and else is crucial for the program structure.
- The power of debugging tools: they help to monitor the execution of conditional elements.
Step-by-Step Guide
To effectively use conditions in Java, you should familiarize yourself with the syntax and functionality. Let's start with basic conditions.
Here I will show you the application of conditions using an example where we evaluate a person's age for an age rating check.
Step 1: Set up a basic if example You want to check if a person's age is suitable for a film rating. Start by defining a variable for the age. Here we are setting the age to 18, for example.
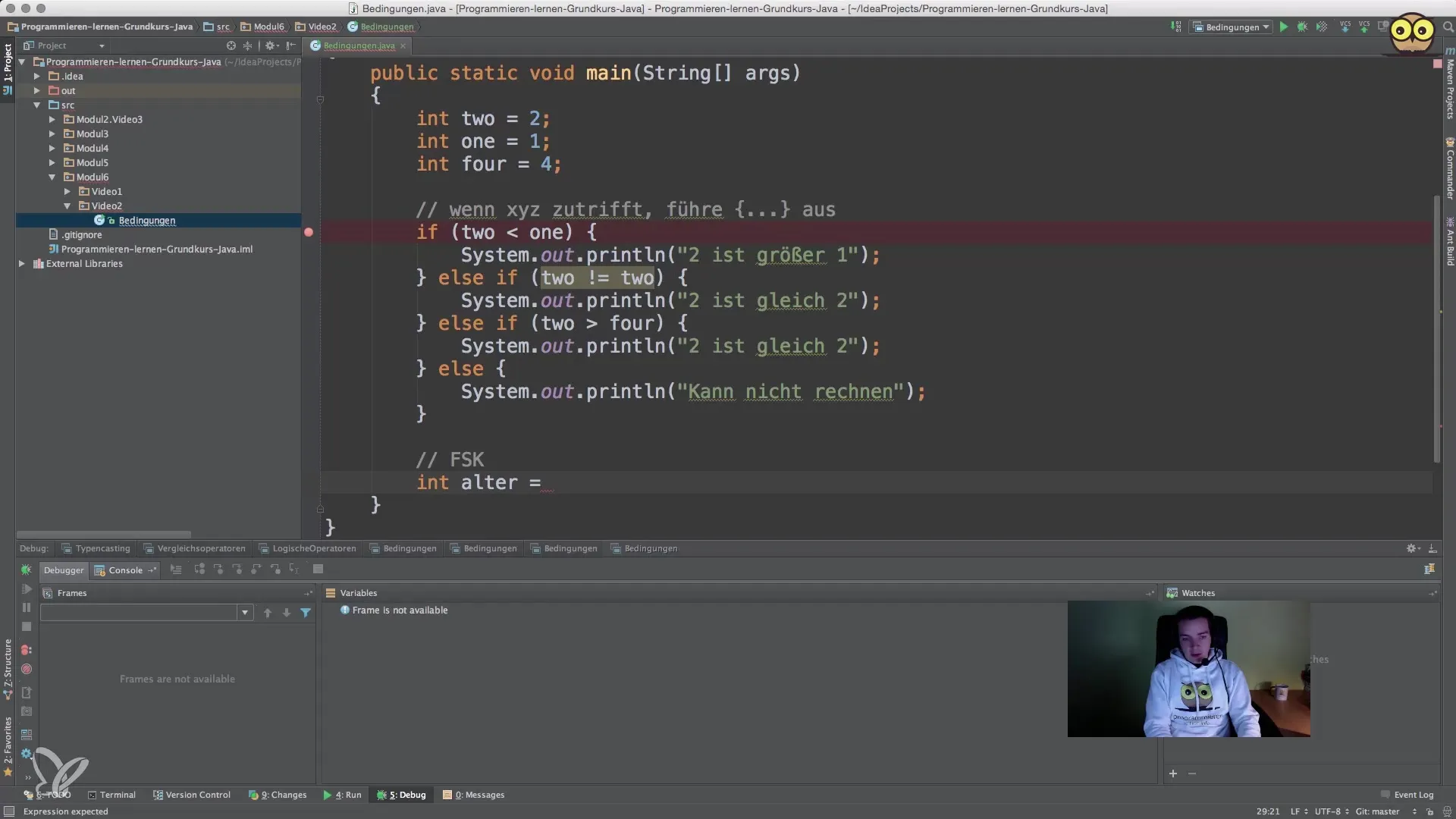
Now we use the if statement to check if the person is 6 years old or older.
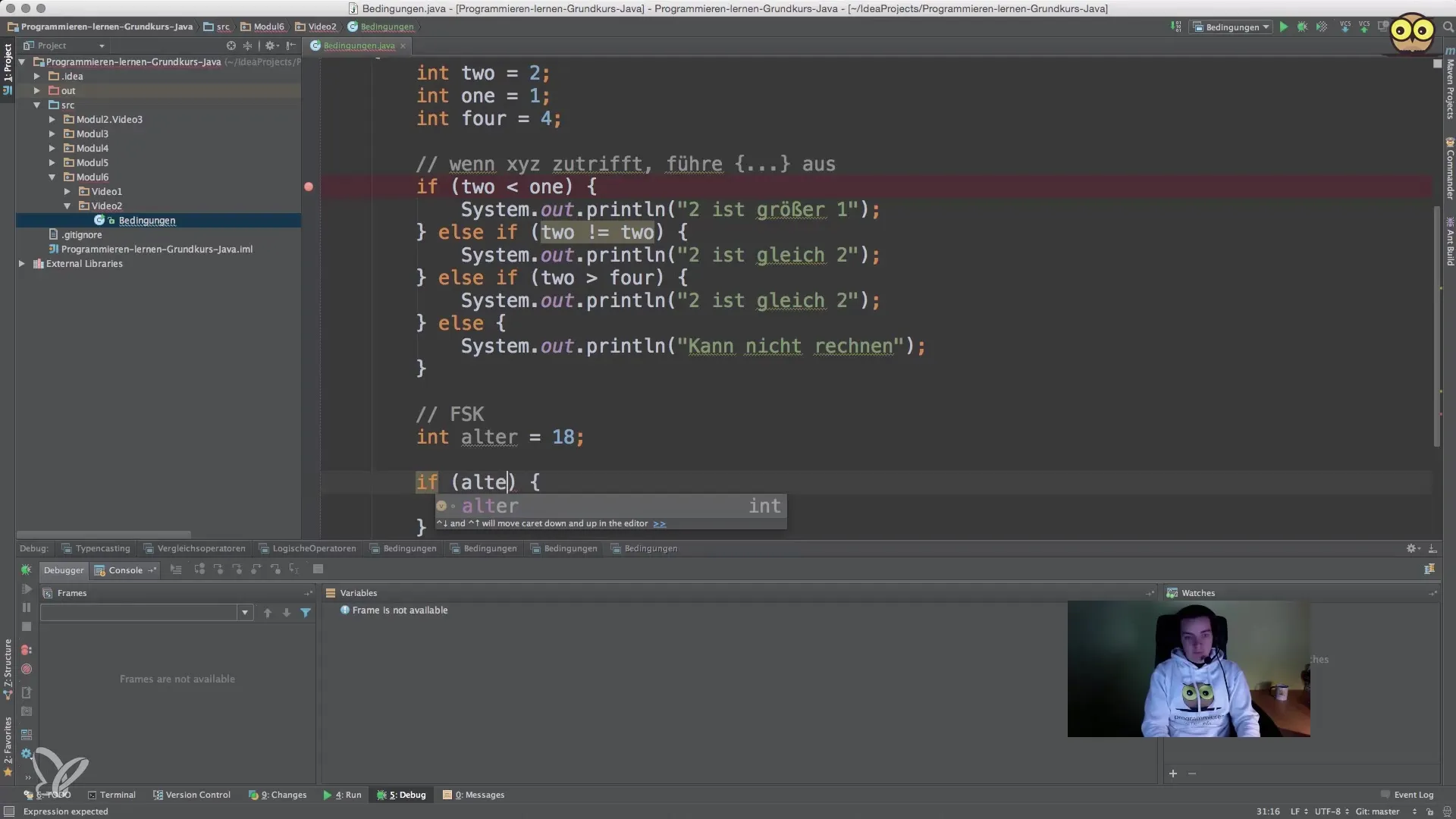
If this is true, we output a corresponding text for the age rating 6. Use the System.out.println method for this.
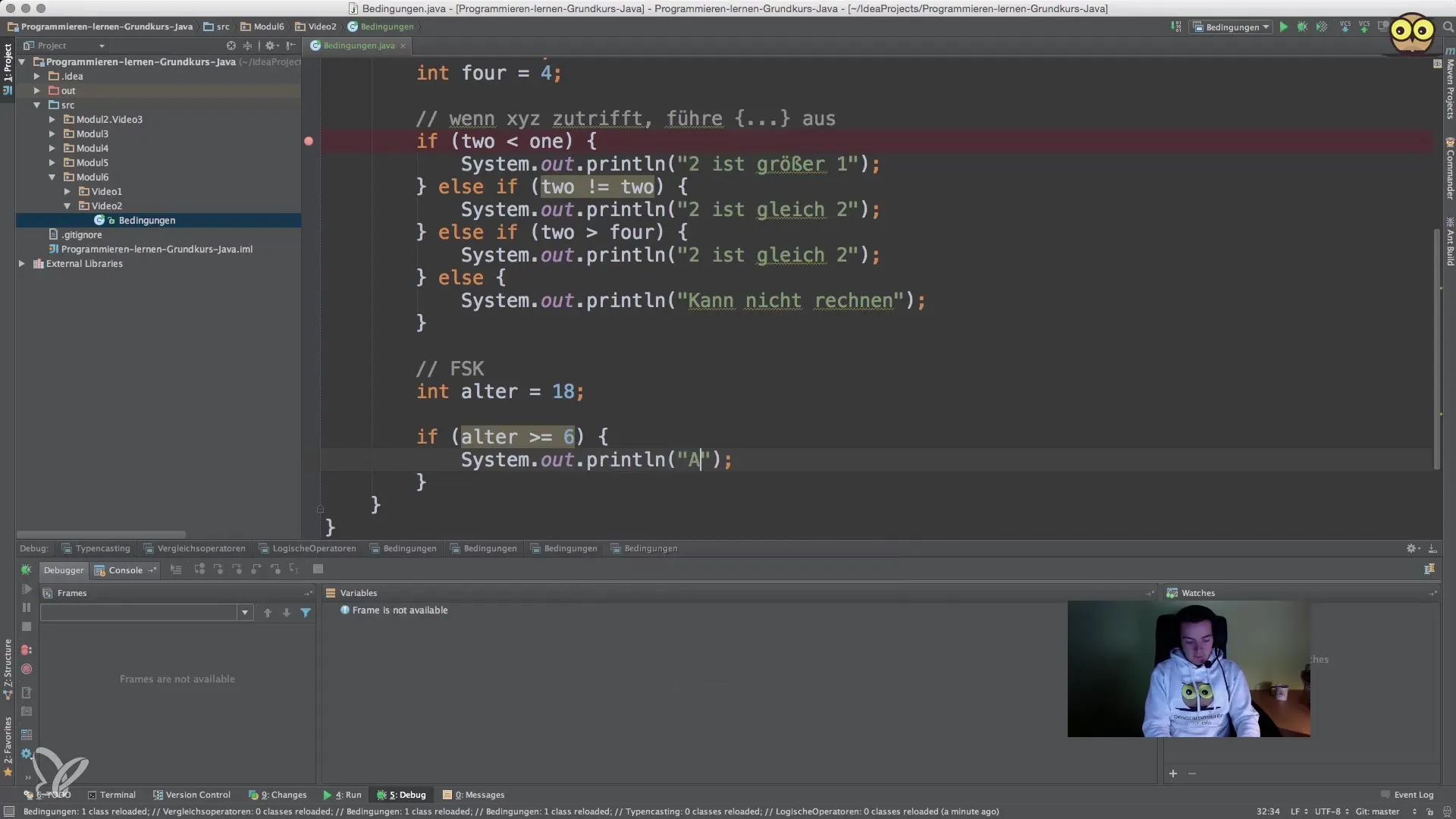
Step 2: Expand the conditions Once the first if statement is set, you can add more conditions. For example, we check if the age is greater than or equal to 12 to output the age rating 12.
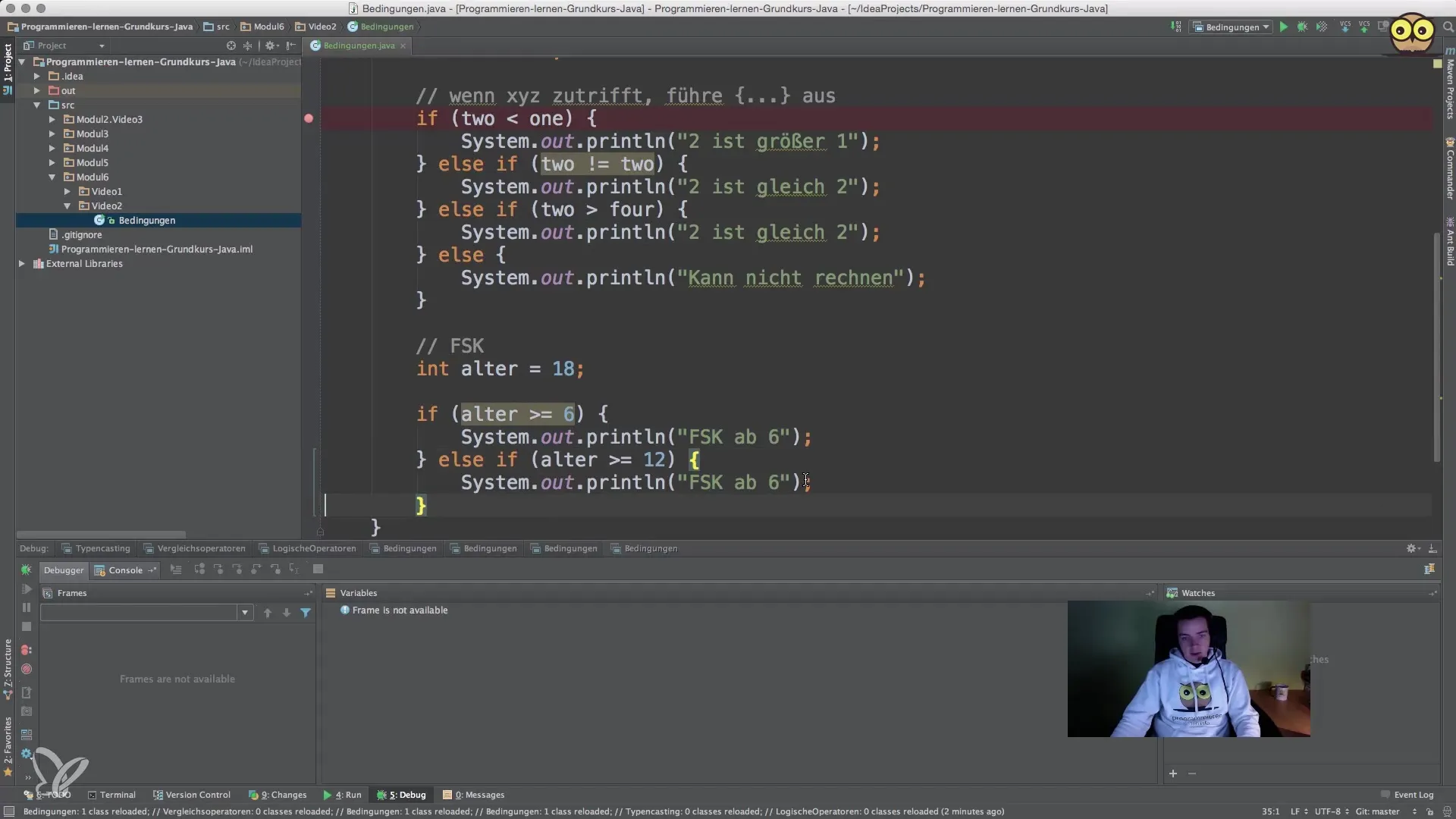
Also introduce the conditions for age ratings 16 and 18. The order here is crucial since the first hit can ignore subsequent conditions.
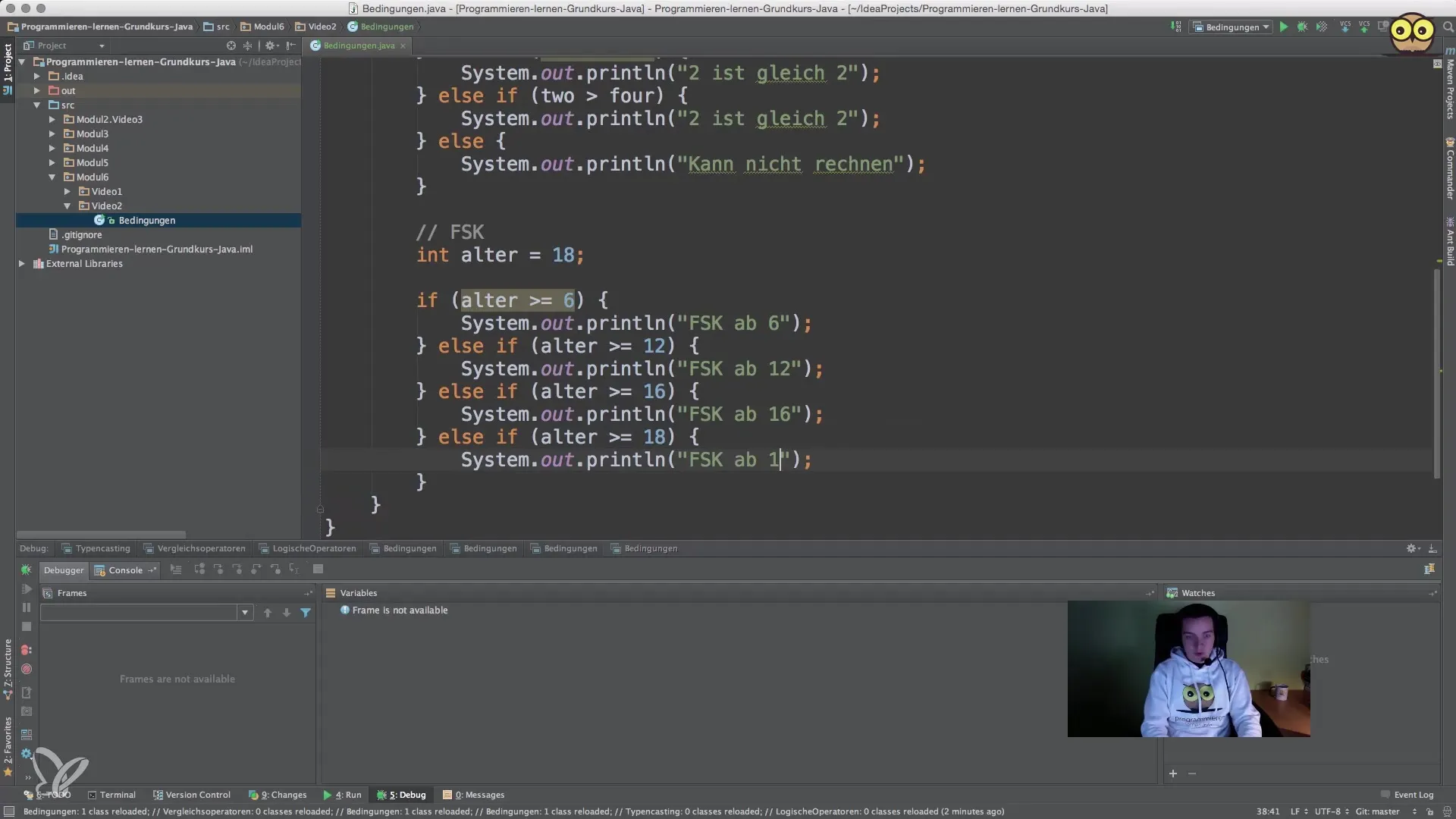
Step 3: Activate debugging Use a debugging tool to monitor the flow of your conditions. Set breakpoints at key points — particularly in the if conditions. This helps you see if all conditions are functioning as expected.
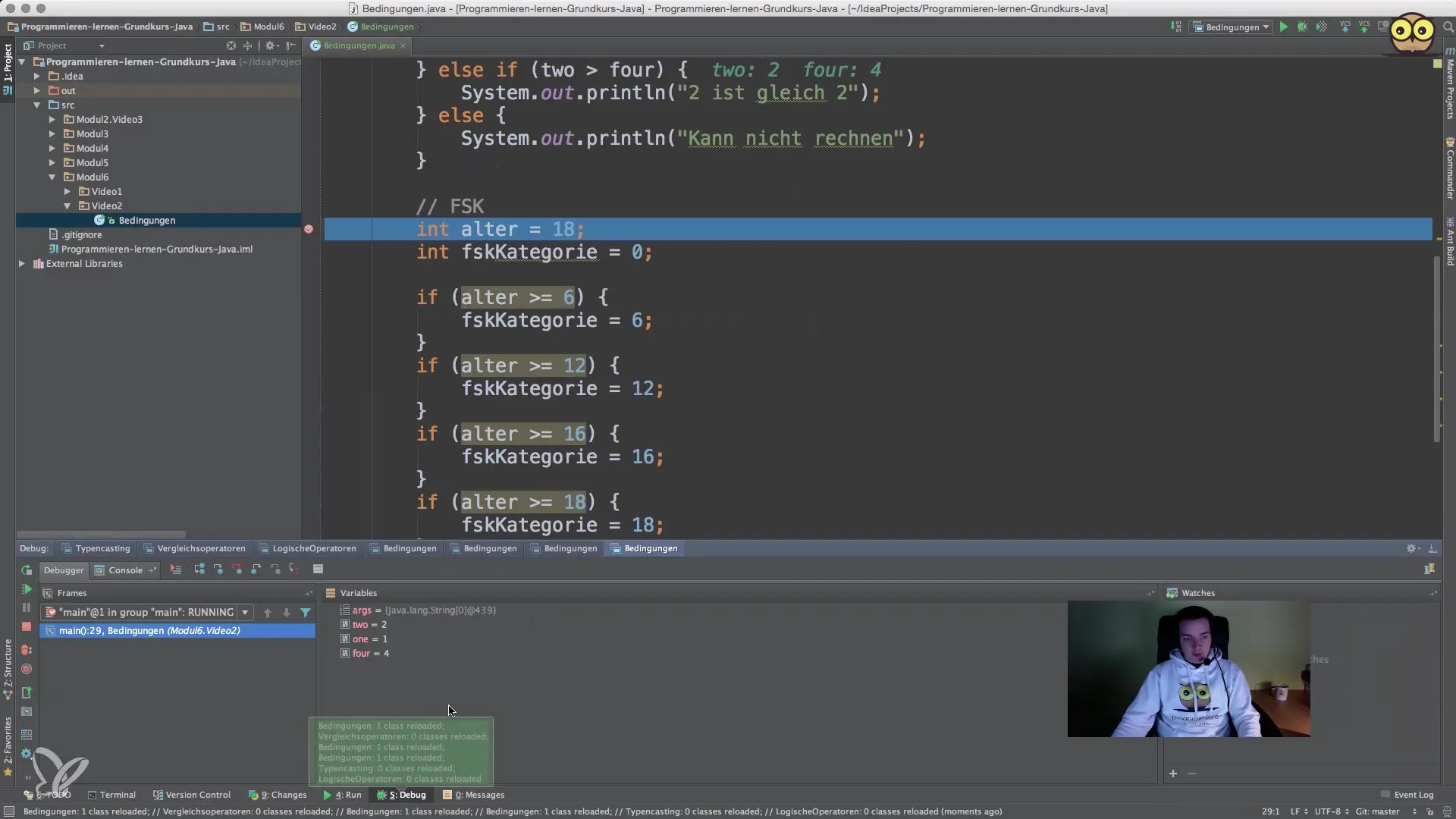
Check the outputs to ensure that the correct age rating categories are displayed.
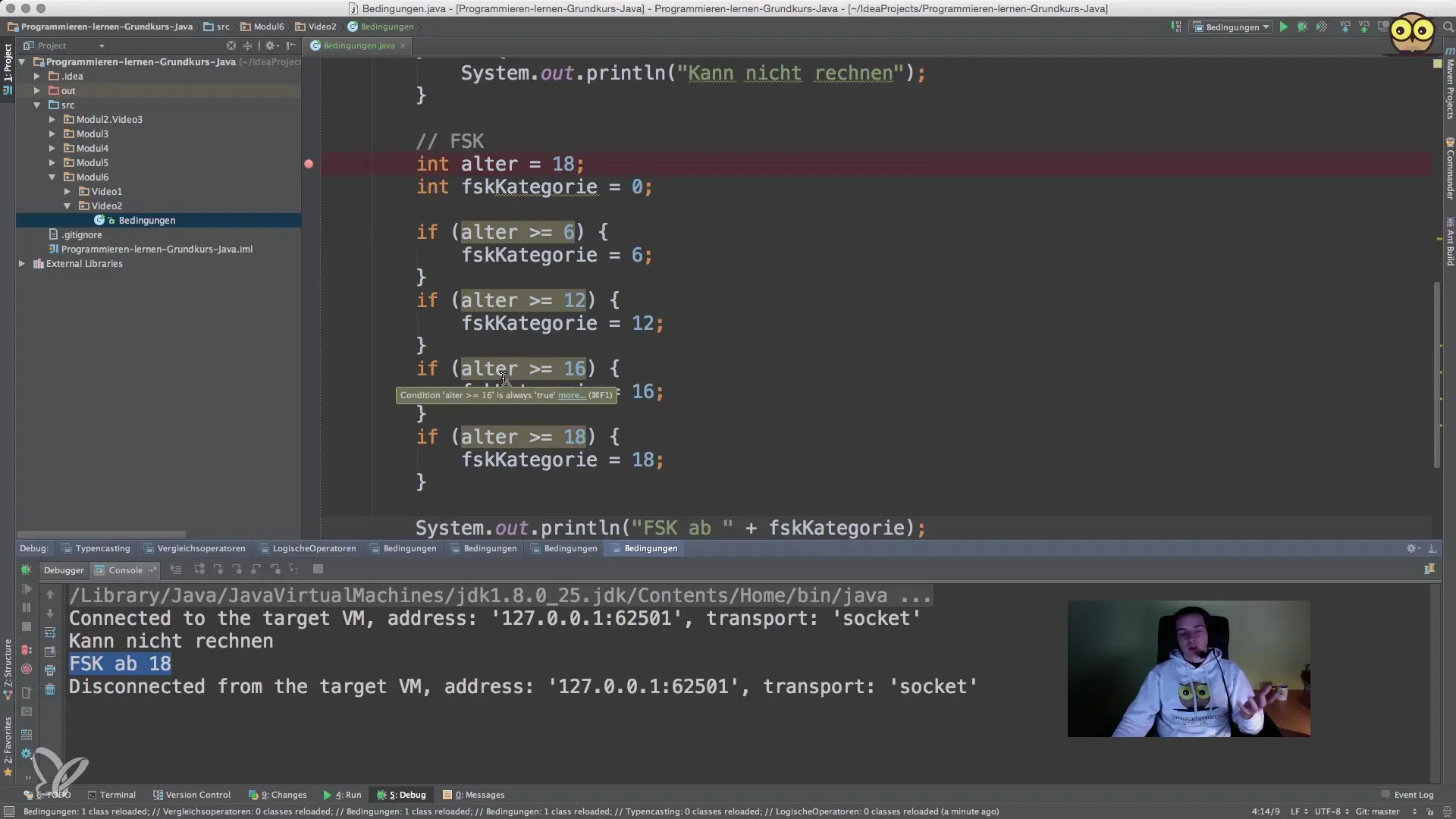
Step 4: Use alternative structures Instead of nested if statements, separate if conditions can be used to make each condition more independent. You can experiment with this to improve readability and efficiency.
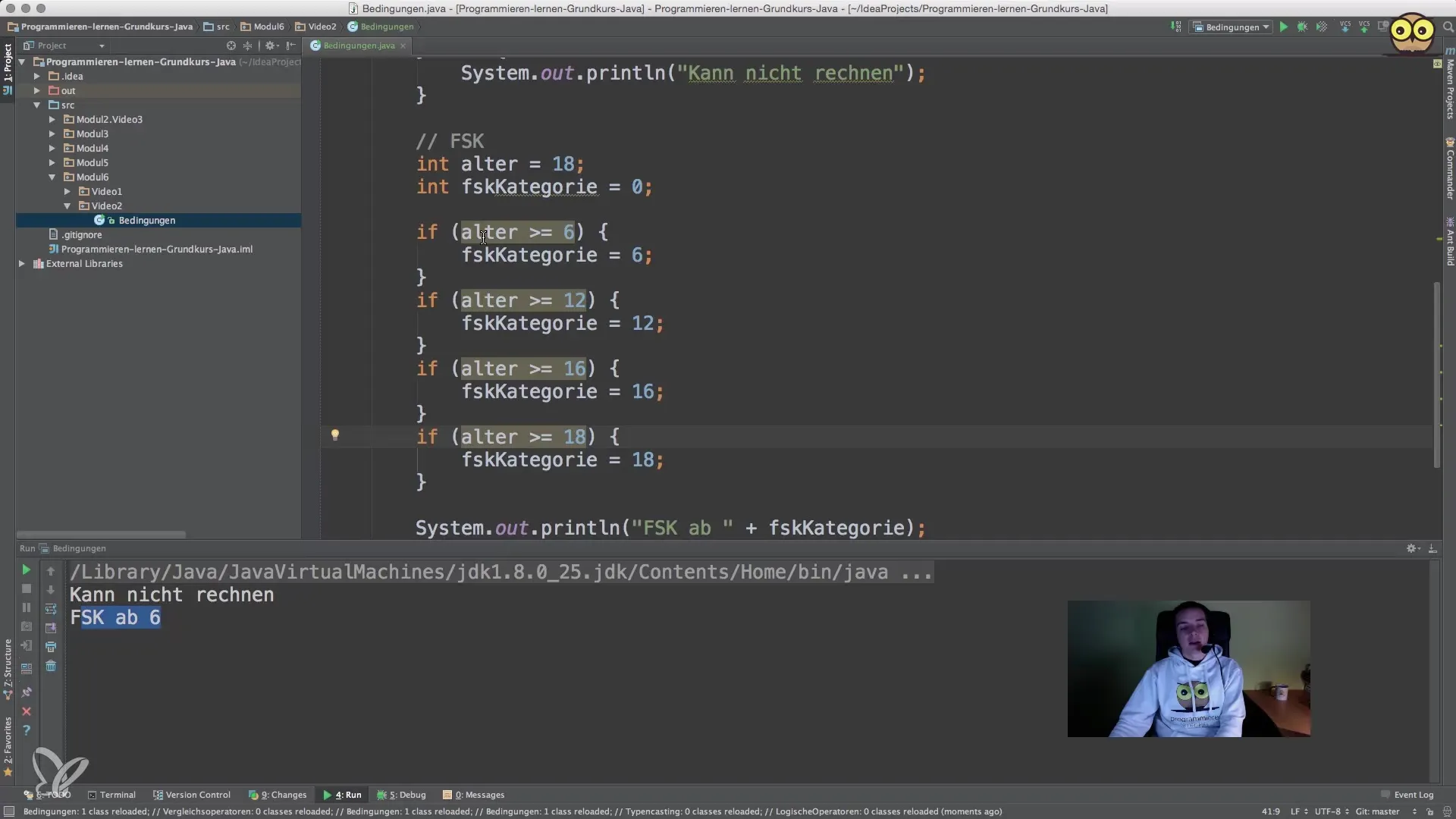
If a condition is true, you can manage variable values together to determine the last valid age rating value.
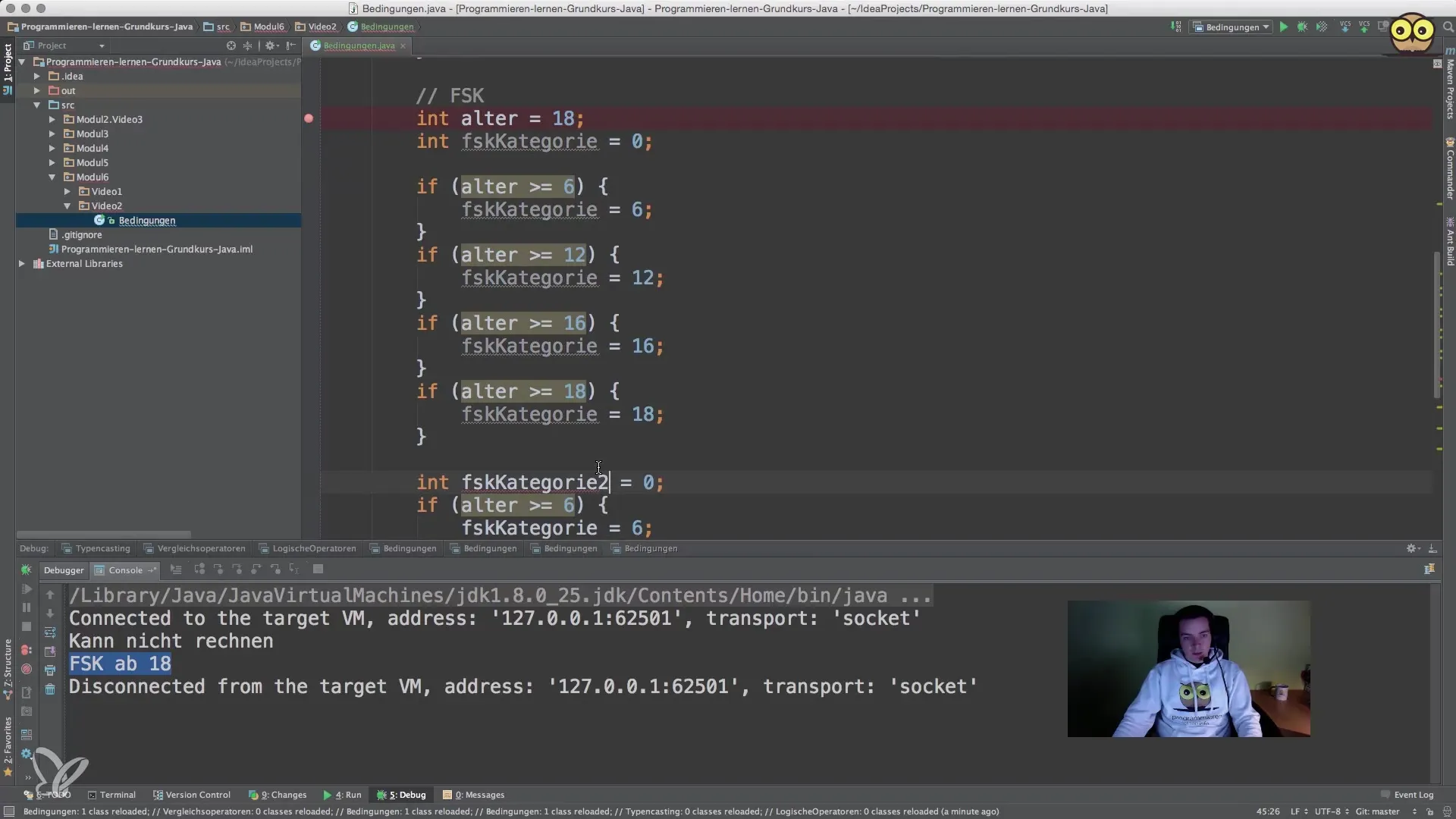
Step 5: Pay attention to the order Be mindful of how you rearrange your if and else if statements right from the start. Begin with the higher age groups to narrow down the targets.
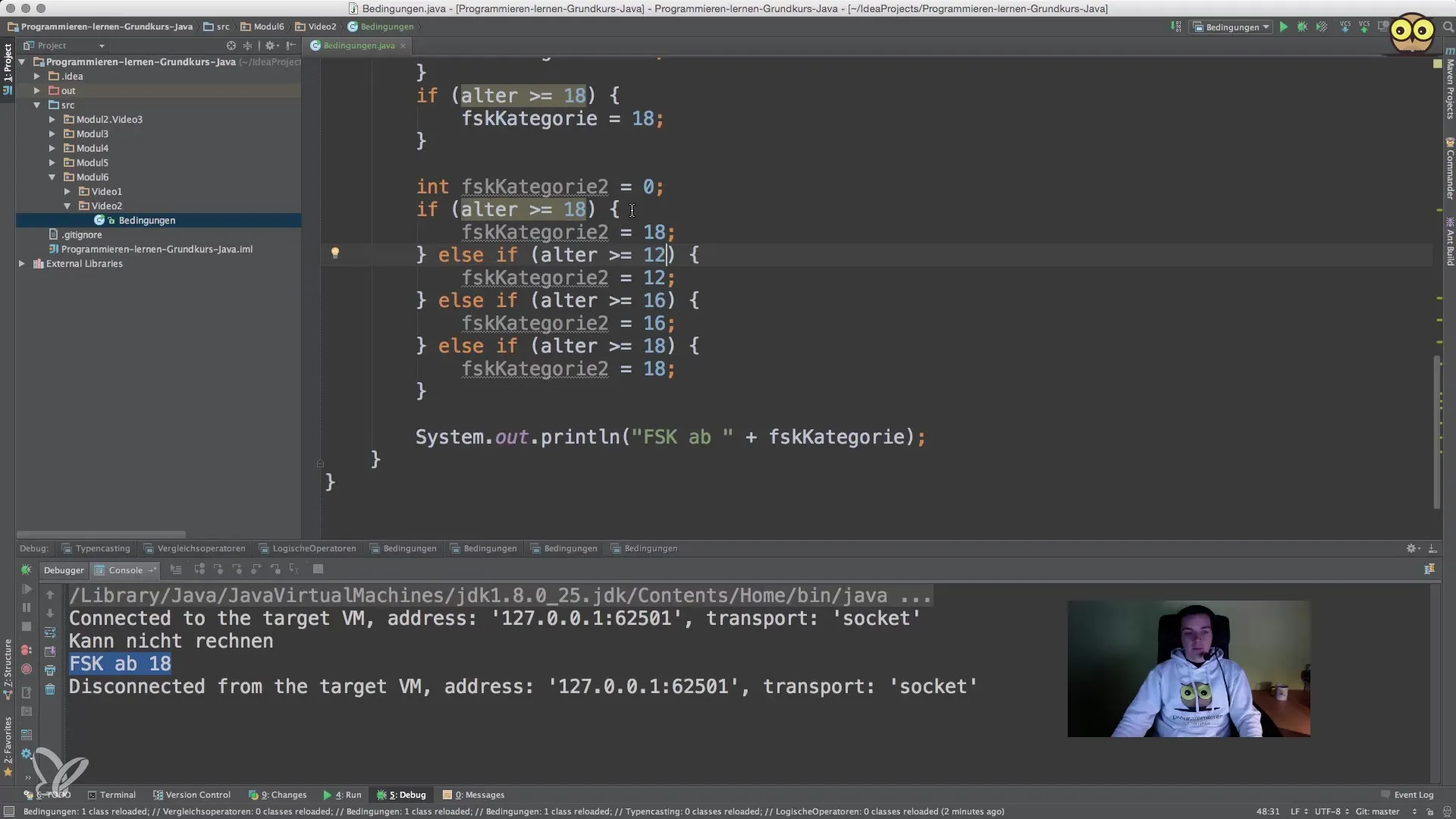
If you know that a person is, for example, 18 years old, you can grant direct access to the age rating 18 information without going through other checks.
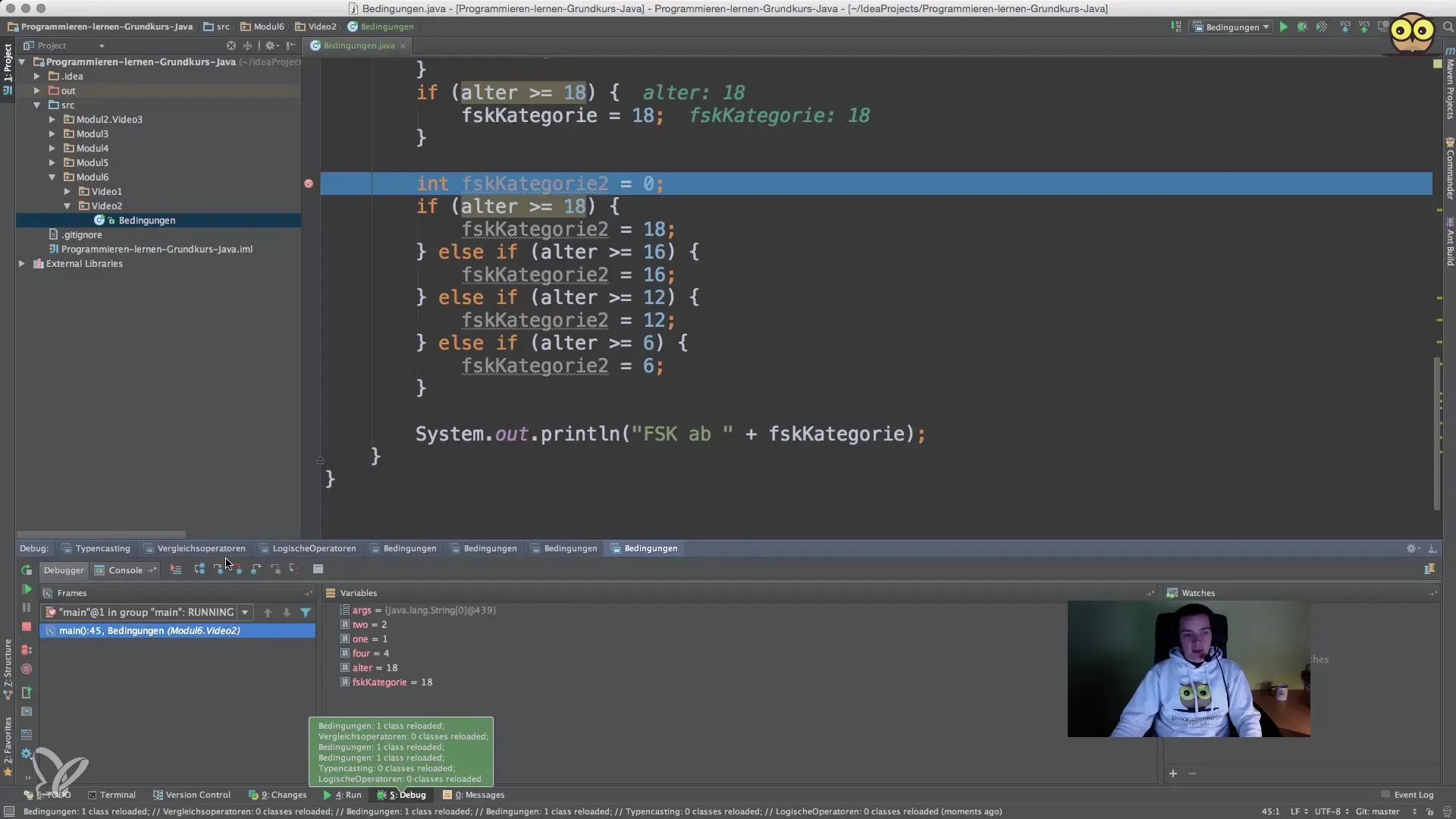
Summary – Tips on Conditions in Java
The importance of conditions in Java cannot be overstated. They are the backbone of every software logic. Pay attention to the order of your checks and utilize debugging tools to ensure your code functions as intended. Experiment with different conditions to gain the best possible understanding.
Frequently Asked Questions
What are conditions in Java?Conditions in Java allow your program to perform different actions based on certain truth values.
How do I use if, else if, and else?With if you evaluate a condition, with else if you can check alternative conditions, and with else you perform an action if no previous conditions were met.
How can I avoid errors in condition checks?Make sure to consider the order of conditions and use debugging tools to track which conditions are true or false.
What is the benefit of debugging?Debugging helps you analyze the control flow of your program and determine where conditions are not met or incorrect outputs are generated.