Array lists are one of the most flexible and commonly used data types in Java. They allow you to create a dynamic list of elements that can be adjusted as needed. Unlike traditional arrays, array lists provide the capability to add, remove, and dynamically change the size of the list. In this guide, you will learn the basics of array lists and how to effectively use them in your Java projects.
Key insights
- Array lists are generic data types that allow the storage of objects of different types.
- They offer many useful methods such as add(), get(), size() to work with elements.
- The difference from arrays lies in the flexibility and dynamic size of array lists, which gives them an advantage in many applications.
Step-by-Step Guide
To provide you with a practical understanding of array lists, we will cover some basic steps.
1. Creating an Array List
To create an array list in Java, you first need to import the ArrayList class. Here is an example:
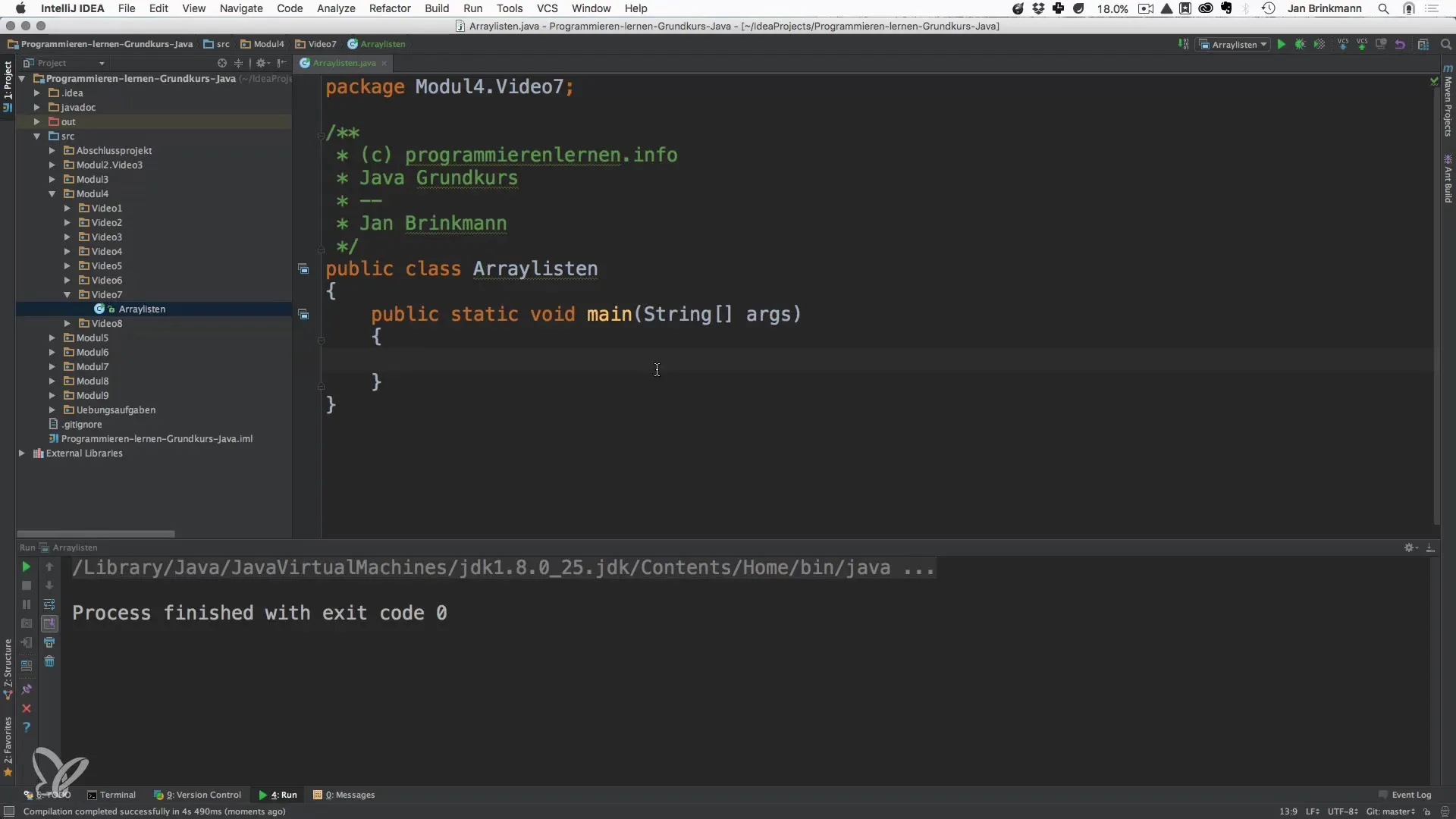
In this example, you declare an array list for integers. It is important to note that you must specify the data type in angle brackets to indicate which type of objects you want to store in your list.
2. Adding Elements to the Array List
An essential aspect of array lists is the ability to add elements. You use the add() method to add new values to your list.
Here we see that we are adding multiple integer values to the integer list. With each add() call, a new element is inserted into the list, effectively expanding the list.
3. Outputting the Number of Elements
An important method that helps you find out the size of your array list is size(). This method returns the number of elements currently stored in the list.
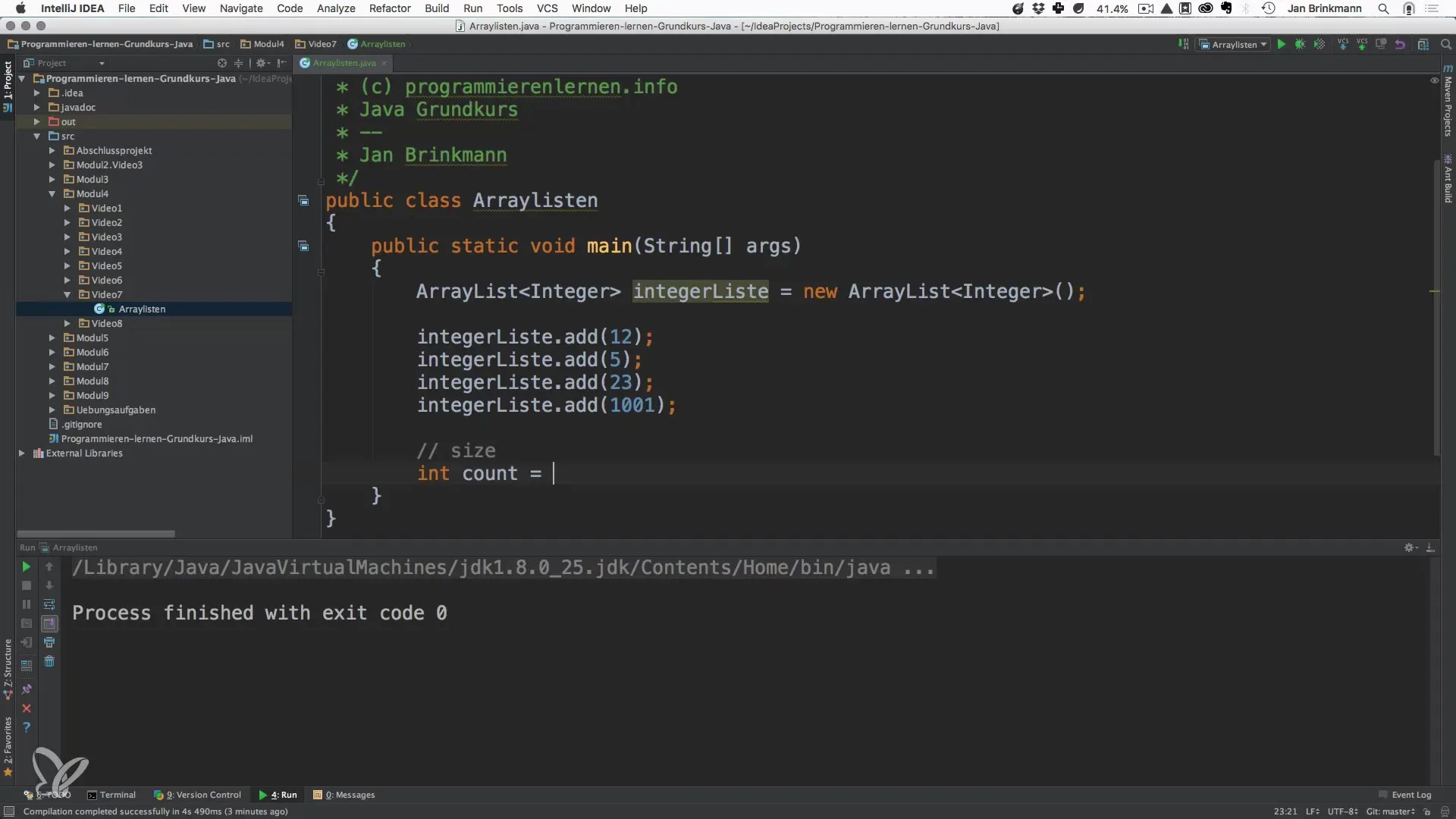
In this step, we create a variable to store the number of elements and then use System.out.println() to output the number of added elements.
4. Iterating Over the Array List
Another useful aspect of array lists is the ability to iterate through the list. You can do this with a loop. Here we use a simple for loop.
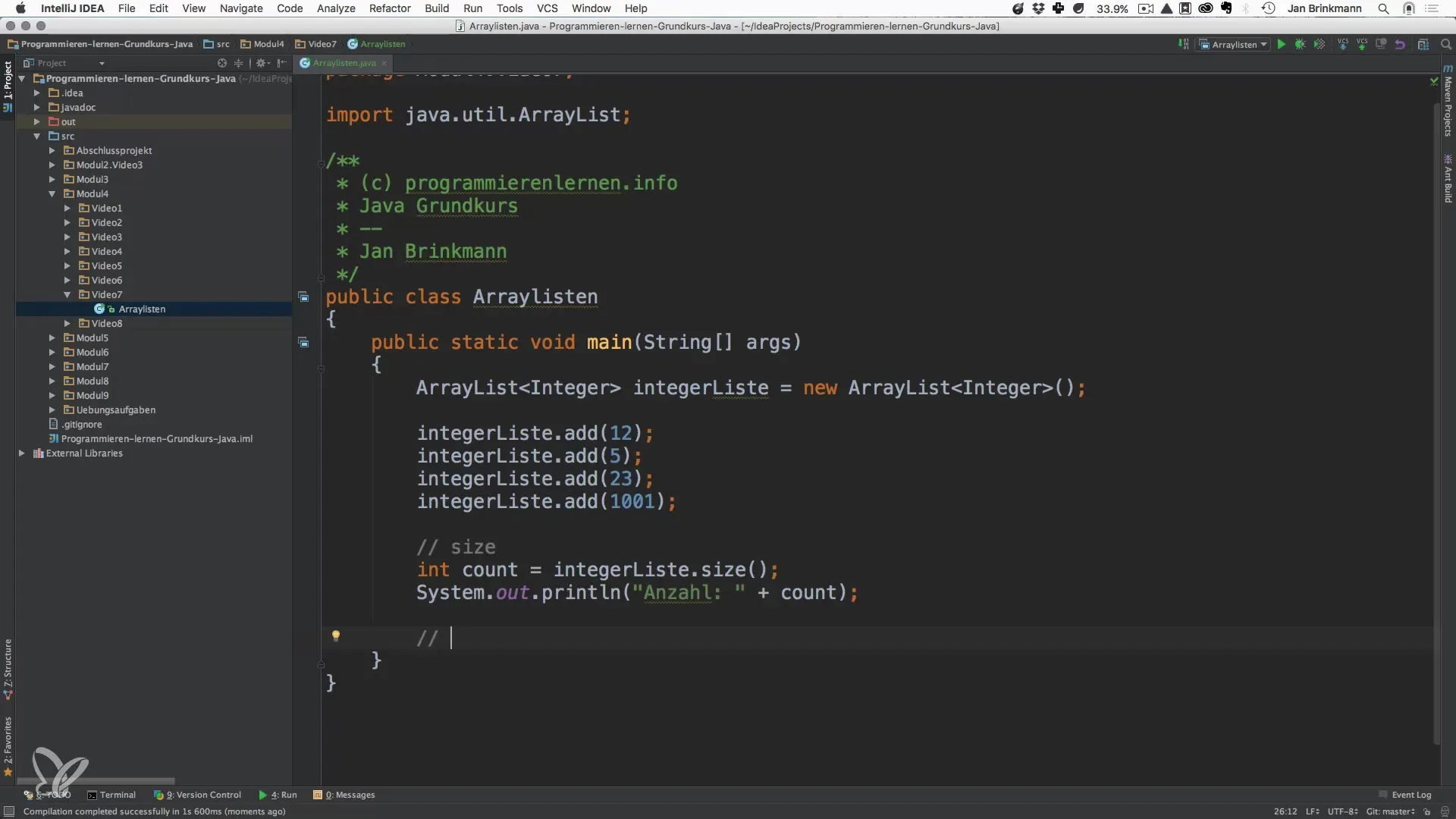
The loop runs as long as the number of elements in the list is reached. In each loop iteration, you can retrieve the current element using the get() method.
5. Using Generics
Another concept you should pay attention to is the use of generics. They allow you to check types at compile time, making data handling in your array list safer.
Generics are an important tool in Java programming as they enable higher code quality and catch errors at compile time rather than at runtime.
6. Flexibility of an Array List
A major advantage of array lists compared to arrays is their flexibility. An array has a fixed size, while an array list can adapt its size dynamically.
Distinct examples or scenarios illustrate when array lists should be preferred, such as in applications where the number of needed elements is unknown at the outset.
Summary – Array Lists in Java: A Comprehensive Introduction
Array lists offer an excellent way to create dynamic data structures in your Java applications. Their flexibility and the variety of useful methods make them an indispensable tool in software development. If you master the concepts and techniques described above, you are well on your way to using array lists effectively.
Frequently Asked Questions
How do I create an array list in Java?Use ArrayList name = new ArrayList(); and specify the desired data type.
What methods are available for array lists?Important methods include add(), remove(), get(), and size().
What are generics in Java?Generics allow the definition of classes or methods with placeholders for data types at compile time.
How can I iterate through an array list?Use a loop to iterate over the indices and use the get() method to retrieve the elements.
Why should I use array lists instead of arrays?Array lists are more flexible and can dynamically adjust the data structure, whereas arrays have a fixed size.