Programming with Java often begins with the most fundamental building blocks: the primitive data types. These data types are essential as they form the basis for storing and manipulating data in Java. In this guide, you will learn about the primitive data types Java offers, how to declare them, and where their typical application areas lie.
Key Insights
- Java distinguishes between primitive and complex data types.
- The primitive data types include int, boolean, char, byte, short, long, float, and double, among others.
- Each data type has its own size in memory and its range of values.
Step-by-Step Guide
1. Creating the Java Class
Start by creating a new Java class for your project and name it "DataTypes", for example. This will help you approach the task in a structured manner.
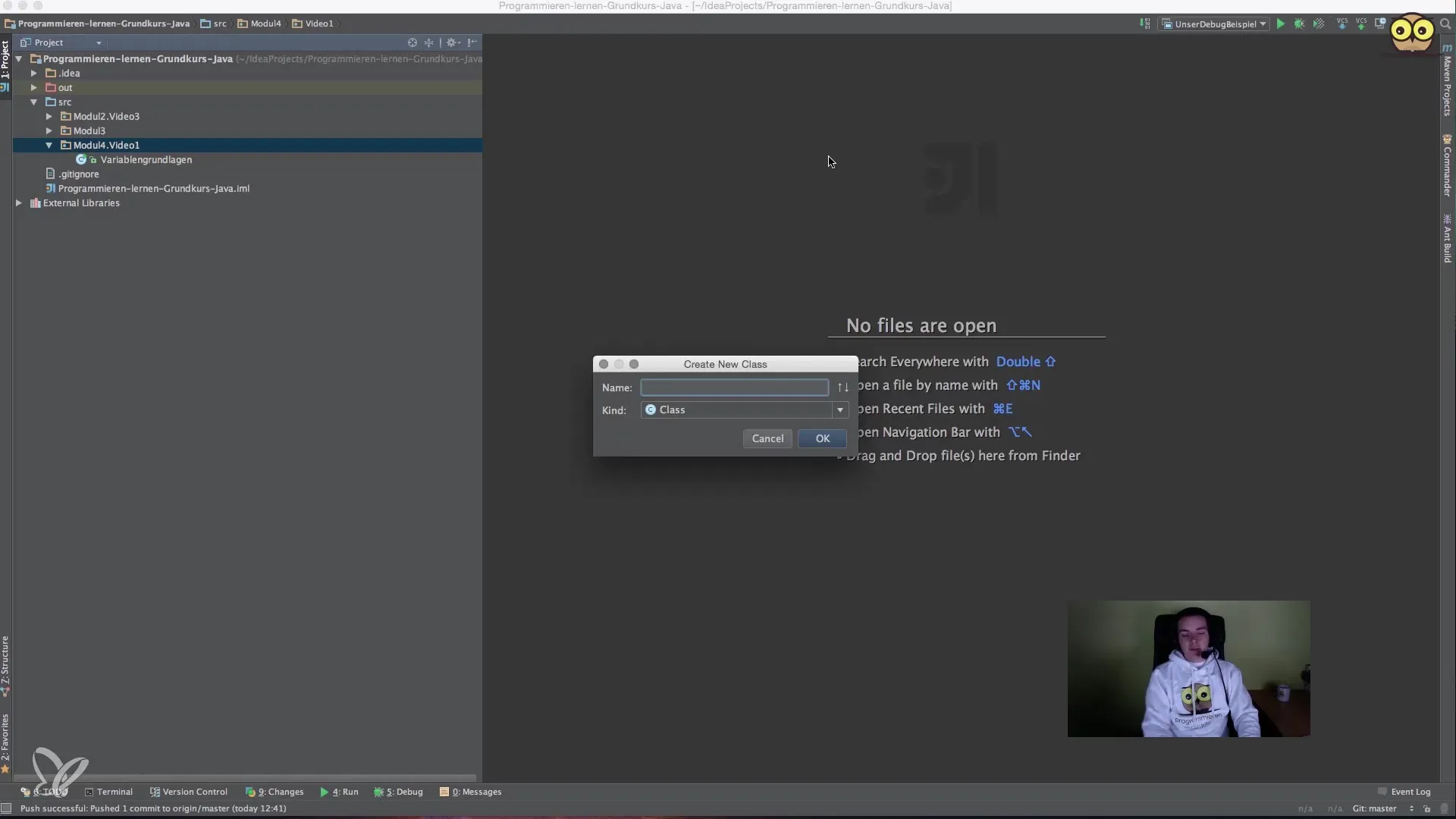
2. Declaring Variables
Once you have created your class, declare your first variable. In Java, the declaration follows a specific pattern: first the data type and then the name of the variable. It looks like this: DataType variableName;
To declare an int variable, for example, you could write the following:
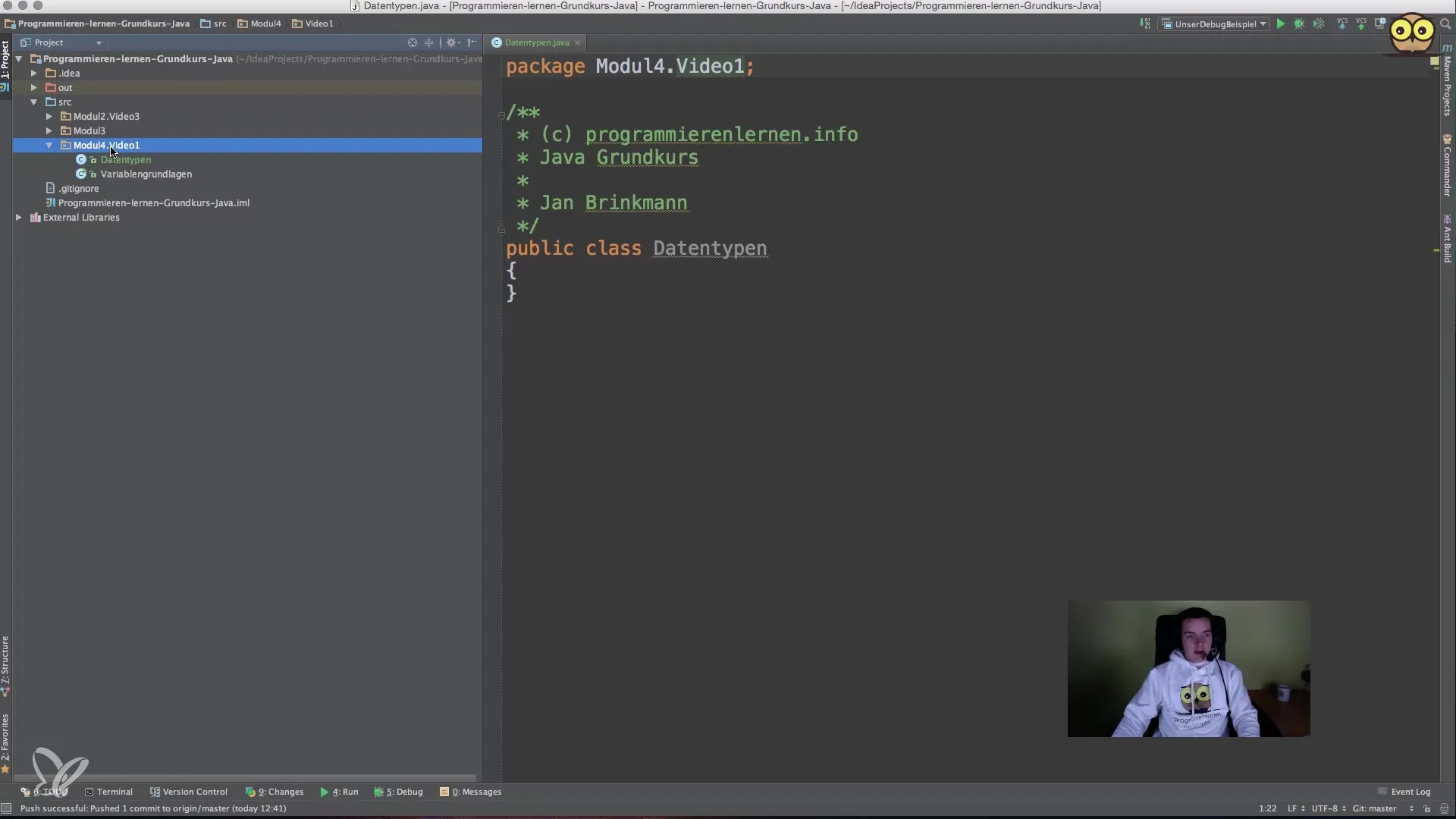
3. Introduction to Primitive Data Types
Now that you have a variable, let’s look at the various primitive data types. First, we start with the boolean data type. A boolean value can be either true or false. This is crucial for checking conditions and making decisions in your code.
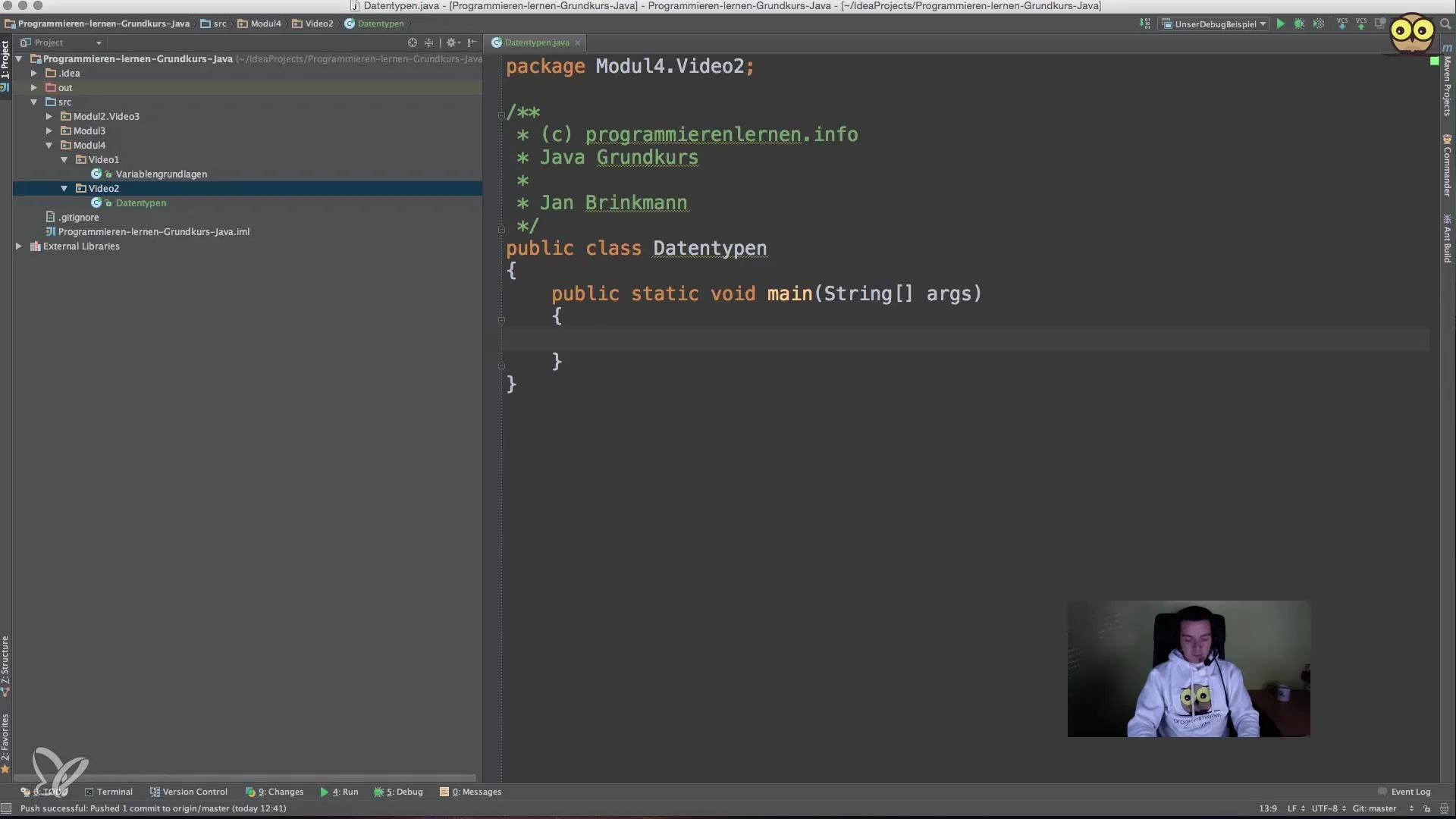
4. Understanding the Char Data Type
Another important primitive data type is char, which stands for character. A char stores a single character and is actually treated as a number in the background. For instance, the letter A is represented by 65 in the ASCII table. This allows you to store letters and other characters efficiently.
5. Integer Data Types
Now let's take a look at the different integer data types: byte, short, int, and long. Each of these data types has a specific range of values and memory requirement. A byte occupies 1 byte and ranges from -128 to 127, while a long is larger and can store significantly more values.
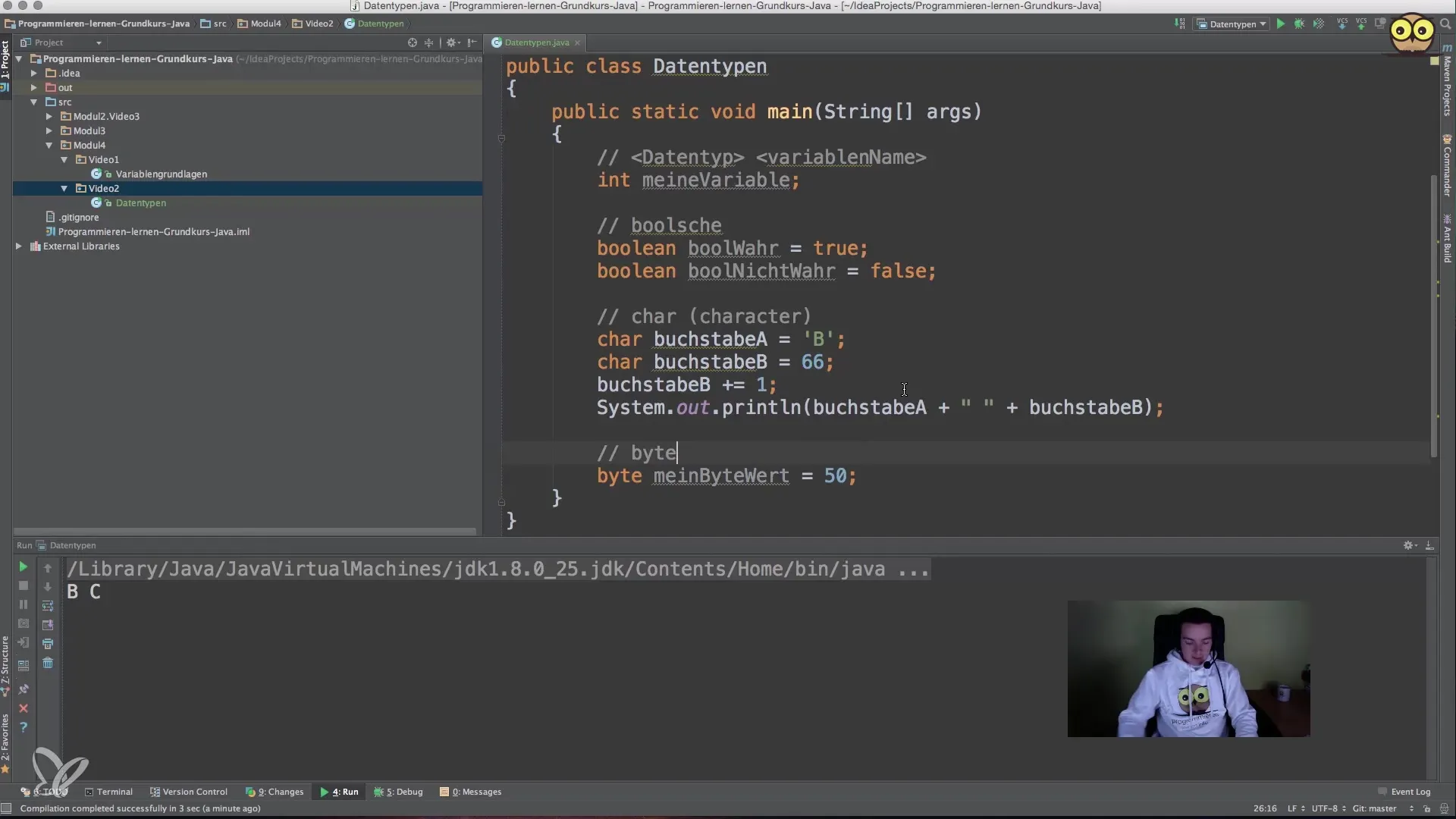
6. Floating-Point Data Types
After understanding the integer types, we move on to the floating-point data types: float and double. The float data type offers an accuracy of about 7 decimal places, whereas double allows for up to 15 decimal places, making it ideal for more precise calculations.
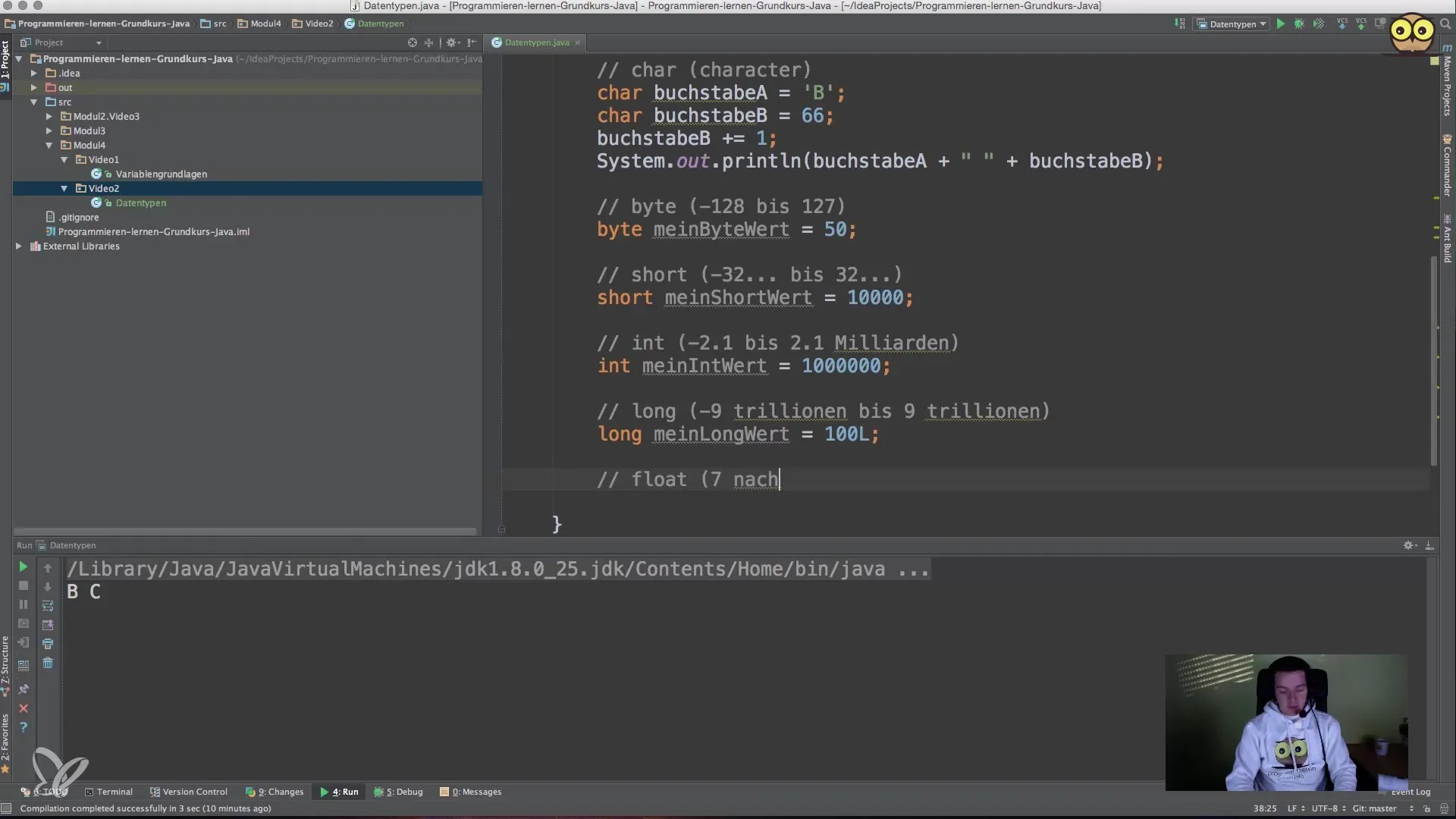
7. Summary of Data Types
Overall, you have now gained knowledge about the different primitive data types in Java, their usage, and their range of values. It is important to know when to use what, especially concerning memory usage and accuracy of the data.
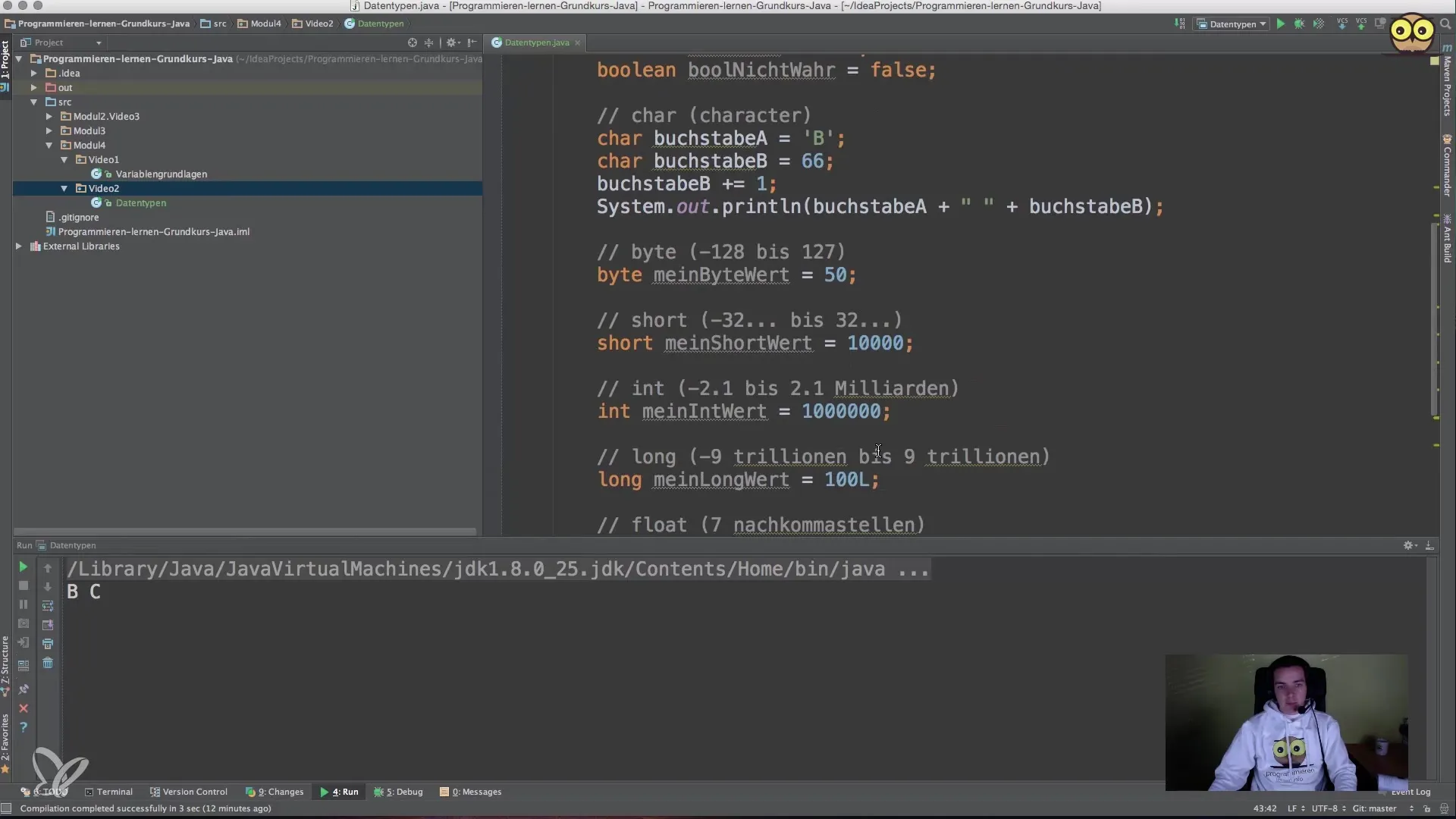
Summary - Understanding Primitive Data Types in Java
This guide has introduced you to the basic primitive data types in Java and shown you how to declare, initialize, and use them. You have learned that choosing the correct data type is crucial for programming efficiency.
Frequently Asked Questions
What are primitive data types in Java?Primitive data types are the simplest data types in Java, including int, boolean, char, byte, short, long, float, and double.
What is the range of values for an int data type?The int data type ranges from -2,147,483,648 to 2,147,483,647.
When should I use float instead of double?float should be used when you need a smaller amount of storage and less precise calculations.
How can I declare a String variable in Java?A String variable is treated as a complex data type and is declared as follows: String variableName;.
Why is it important to choose the right data type?The right data type affects memory consumption and the accuracy of values in your programming script.