With this tutorial, you start your exciting journey into the world of programming. Here you will write your first Java program, create your first variable, and output its content. Let’s get started!
Main insights
You will learn how to:
- structure a simple Java program
- declare and initialize a variable of type String
- output content using System.out.println()
- perform basic configurations in an IDE (IntelliJ IDEA)
Step-by-Step Guide
1. Create a new Java class
To write your first program, open your development environment (IDE) such as IntelliJ IDEA. Here, you will create a new project and set up a new Java class. Name it “MeinErstesProgramm” (MyFirstProgram). It is important to use clear naming to keep track later.
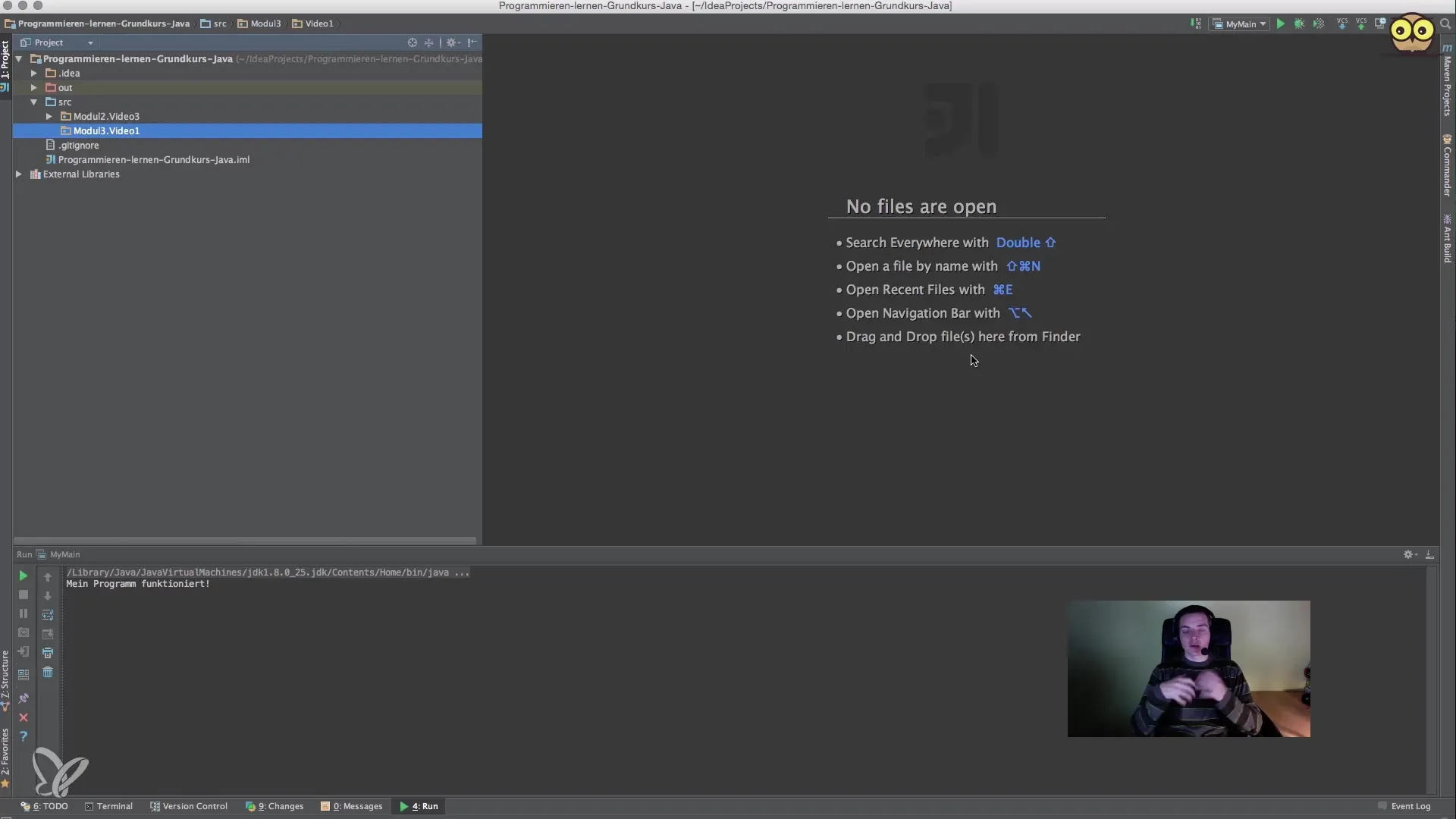
2. Add the main method
In Java, every program needs a main method that serves as the entry point.
Insert this code as a method in your class.
3. Declare a variable
Now we will start creating a variable. We will declare a variable of type String, which we will call “myName”, and set it to your name, for example, “Jan”.
With this step, you have successfully declared your first variable. Make sure that the variable in your code is now highlighted in gray, indicating that it has not been used yet.
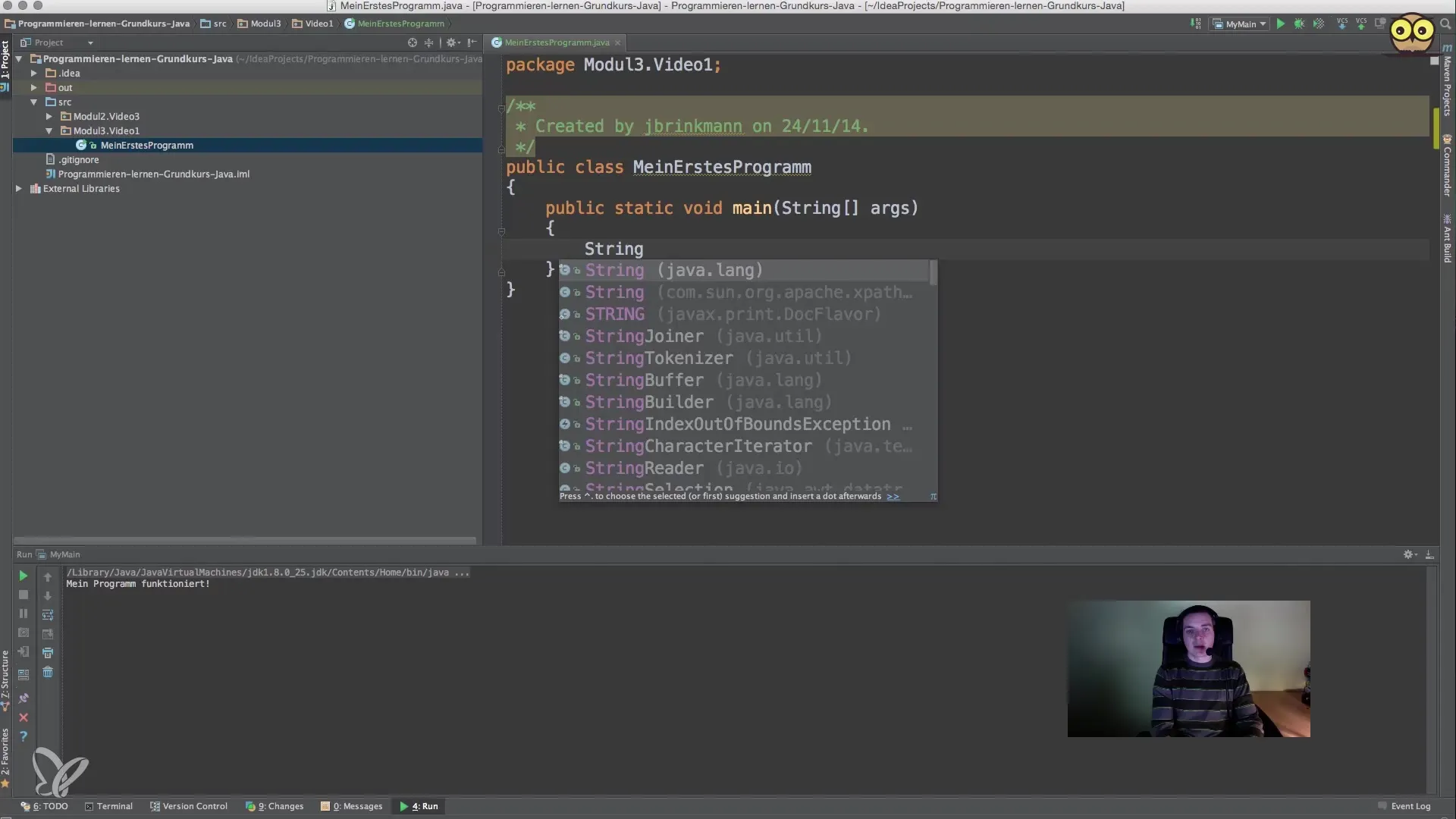
4. Output data using System.out.println()
Now comes the exciting part! To output the content of your variable, you use the method System.out.println().
Here, we use the operator + to concatenate the string “Name:” with the variable “myName”. This is known as string concatenation.
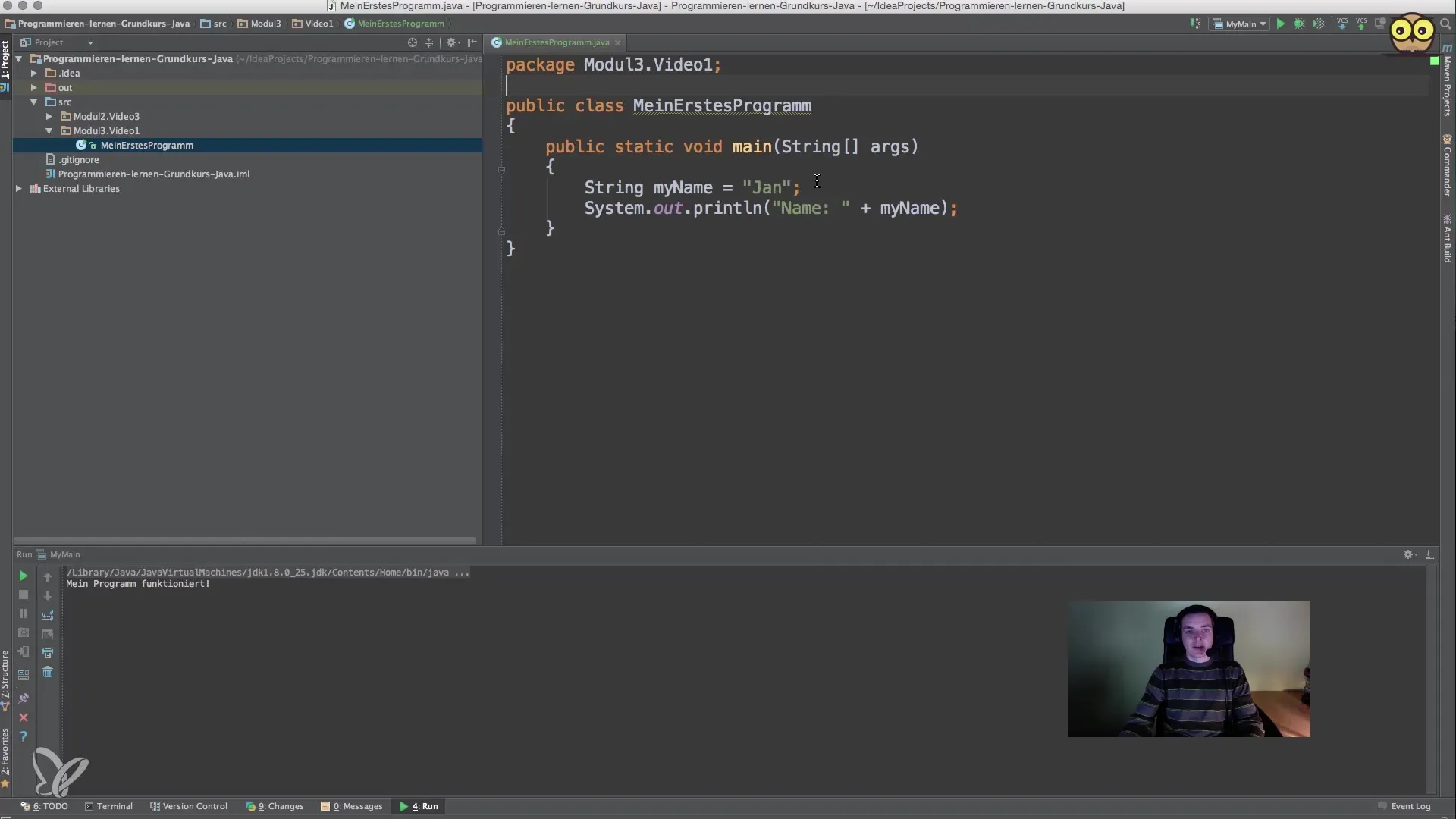
5. Run the program
To run your program, you need to create a configuration in your IDE. Go to the "Run" menu at the top and select "Edit Configurations". Click on the plus sign and choose "Application". A window will open where you can select the name of your class. Choose “MeinErstesProgramm” (MyFirstProgram).
Now press “Play” or “Run” to start your program.
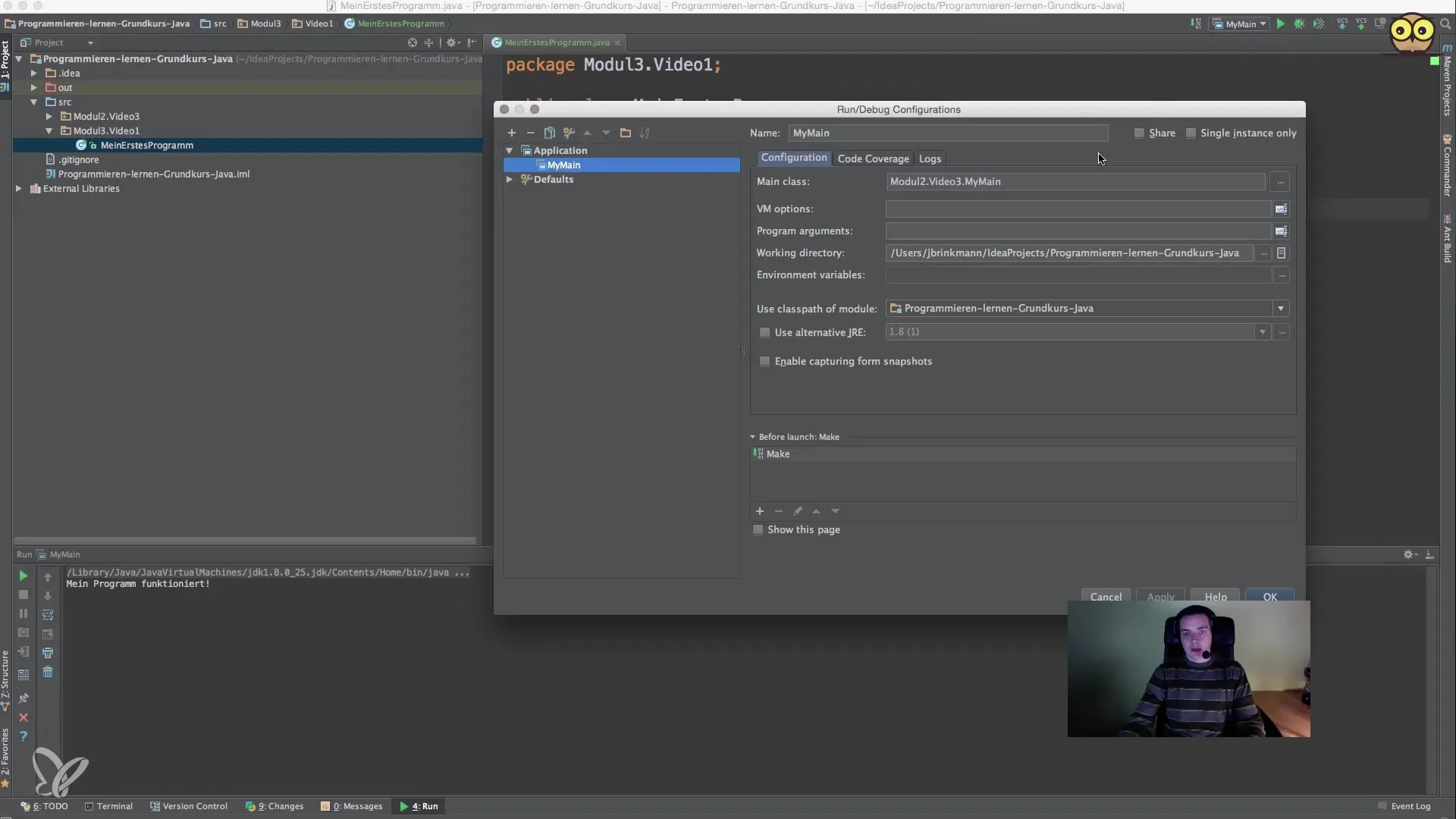
6. Check the results
After running your program, you should see the output in the console, which looks like this:
Name: Jan
If you change your name, for example to “Jan Bringmann”, simply update the value of your variable and run the program again.
7. Adjust configurations
If you want to write and run more programs, it’s wise to organize the configurations in your IDE well. You can create different configurations for different classes. This helps you keep a better overview of your projects.
Summary – Your First Java Program – Step by Step
In this tutorial, you learned how to write your first Java program and create a variable of type String. You tested the output using System.out.println() and made basic configurations in IntelliJ IDEA.
Frequently Asked Questions
What is a variable in Java?A variable is a placeholder for a value that you can store and use in the program.
How do I declare a variable?You declare a variable by specifying the data type, the name, and optionally a value. For example: String myName = "Jan";.
What is the main method?The main method (public static void main(String[] args)) is the entry point of a Java program, where execution starts.
How do I run a program in IntelliJ IDEA?You create a configuration that points to your main class and then press "Play" or "Run".
How can I change the value of a variable?You can change the value by adjusting it directly in the assignment, e.g., myName = "Jan Bringmann";.