Casting is a central concept in the programming language Java, as it allows you to effectively convert data types. You can switch between different data types such as primitive and complex data types. This guide focuses on casting from data type A to data type B, the syntax, and the associated challenges, particularly the potential loss of information. Let's dive in and explore the basics of type casting in Java.
Main insights
- Casting allows the conversion of data types while keeping the syntax consistent.
- There are different types of casting: implicit and explicit casting.
- During conversion, there can be a loss of information, especially when moving from a larger data type to a smaller one.
Step-by-step guide to type casting
1. Introduction to type casting
Type casting involves transferring one data type into another. This is particularly important when you need to work with different data types and Java needs to understand how to handle the data. You can think of the syntax for casting as simple and consistent.
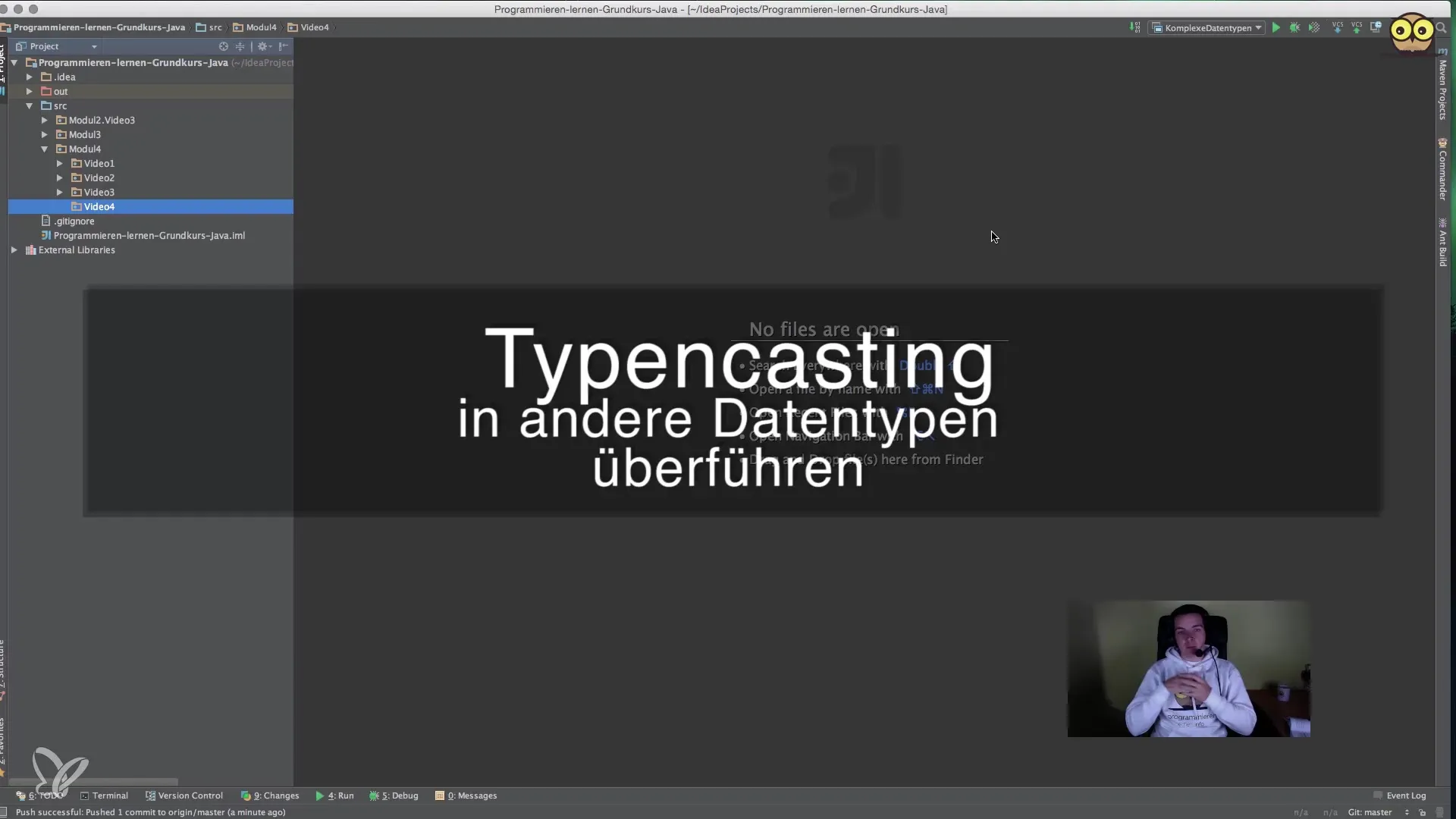
2. Example of implicit casting
Suppose you have a variable of type Integer. If you want to convert this to a Double, you can simply do so by assignment, as Java handles this implicitly. Here, the type automatically represents your data type.
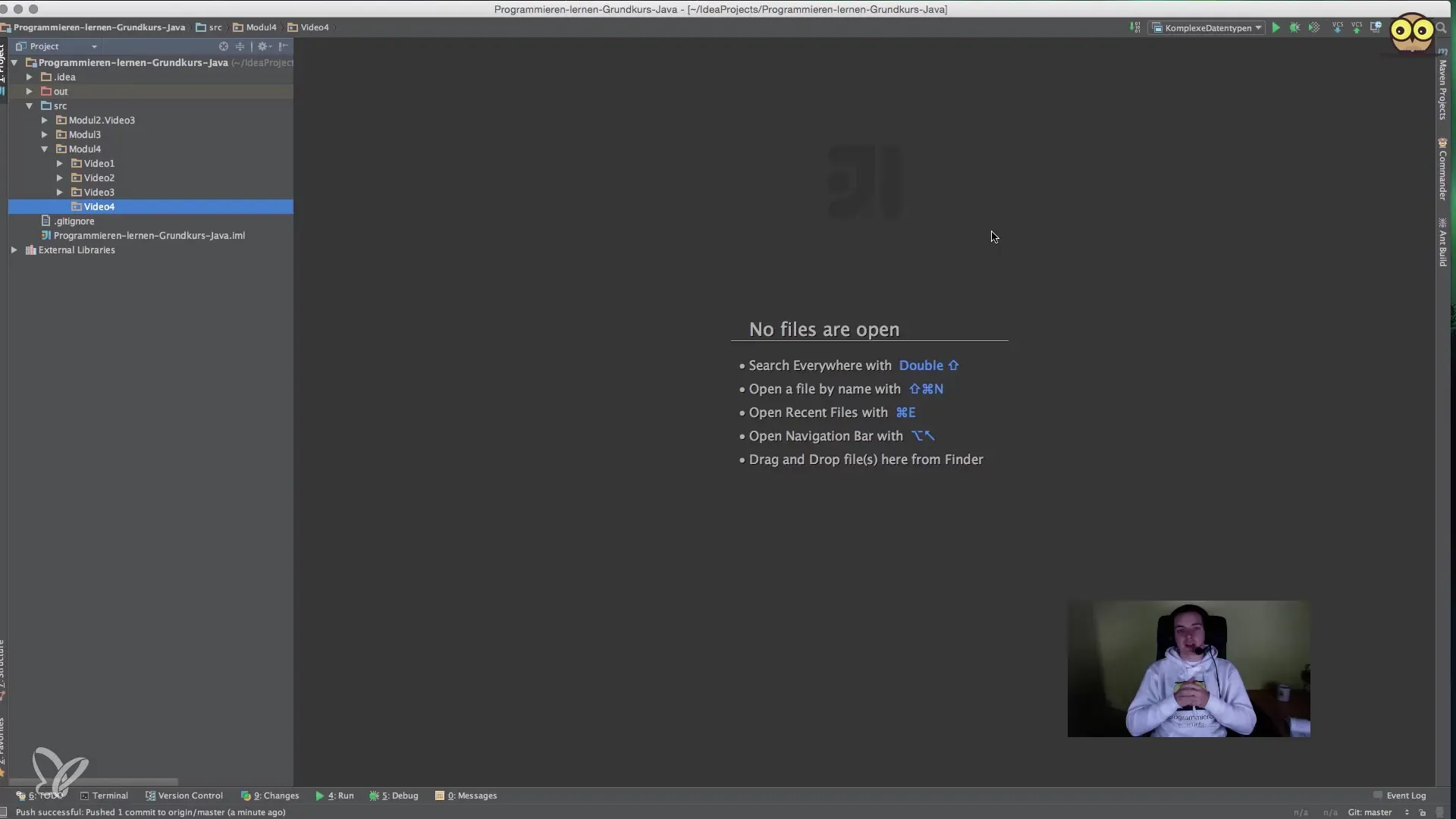
3. Example of explicit casting
Assume you have a Double variable with the value 1.2 and want to convert this value into an Integer variable. In this case, you must cast explicitly, as you are converting from a larger data type (Double) to a smaller one (Integer).
4. Debugging a casting
To verify what actually happens during casting, debugging is an indispensable tool. When you cast from a Double variable to an Integer variable, there may be a loss of decimal places. For example, the value 1.2 becomes 1. Let's debug this step by step:
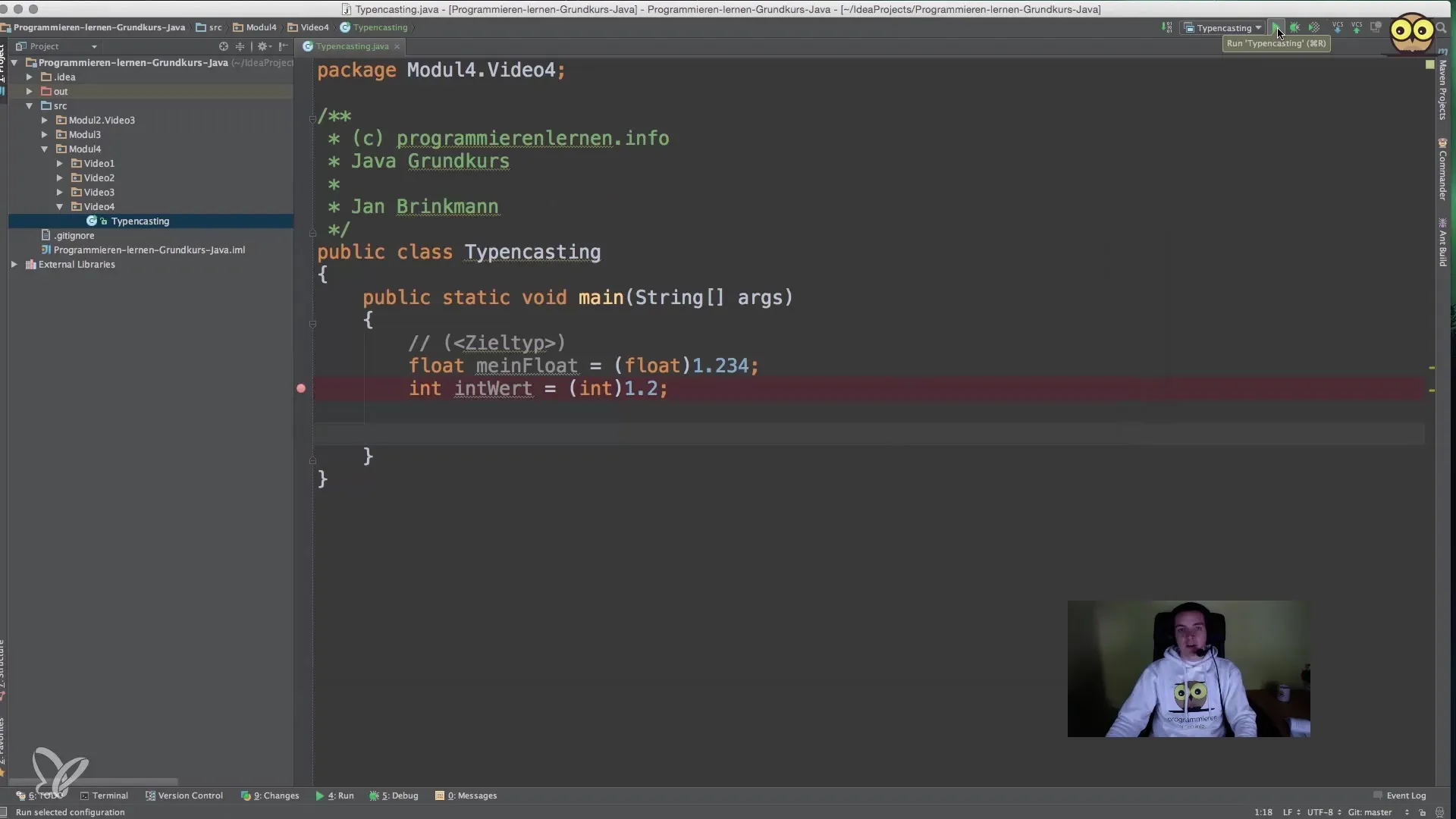
5. Loss of information during casting
When you try to convert a Double value to an Integer, Java truncates the decimal places. As a result, information is lost. We can make this more visible by using more decimal places. If you have a value like 1.23456789, it will be rounded down to 1 when cast. Perform multiple castings and check the values during debugging.
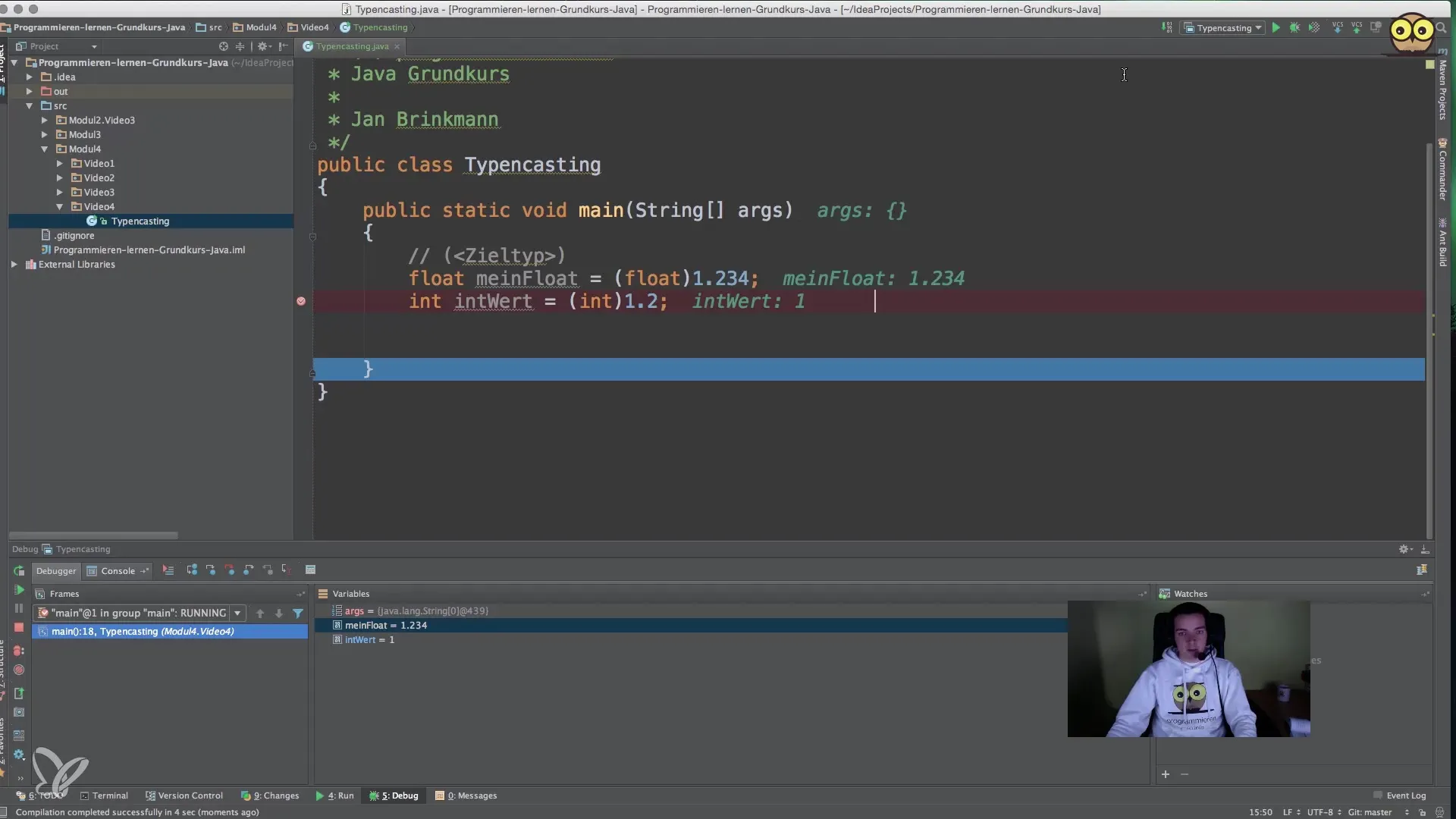
6. Casting from larger to smaller data types
Another important example is when you want to cast an Integer value, which has a large range (e.g., 10,000), into a data type with less memory space (e.g., byte). In this case, further losses may occur. The value may be cut off, resulting in unexpected outcomes.
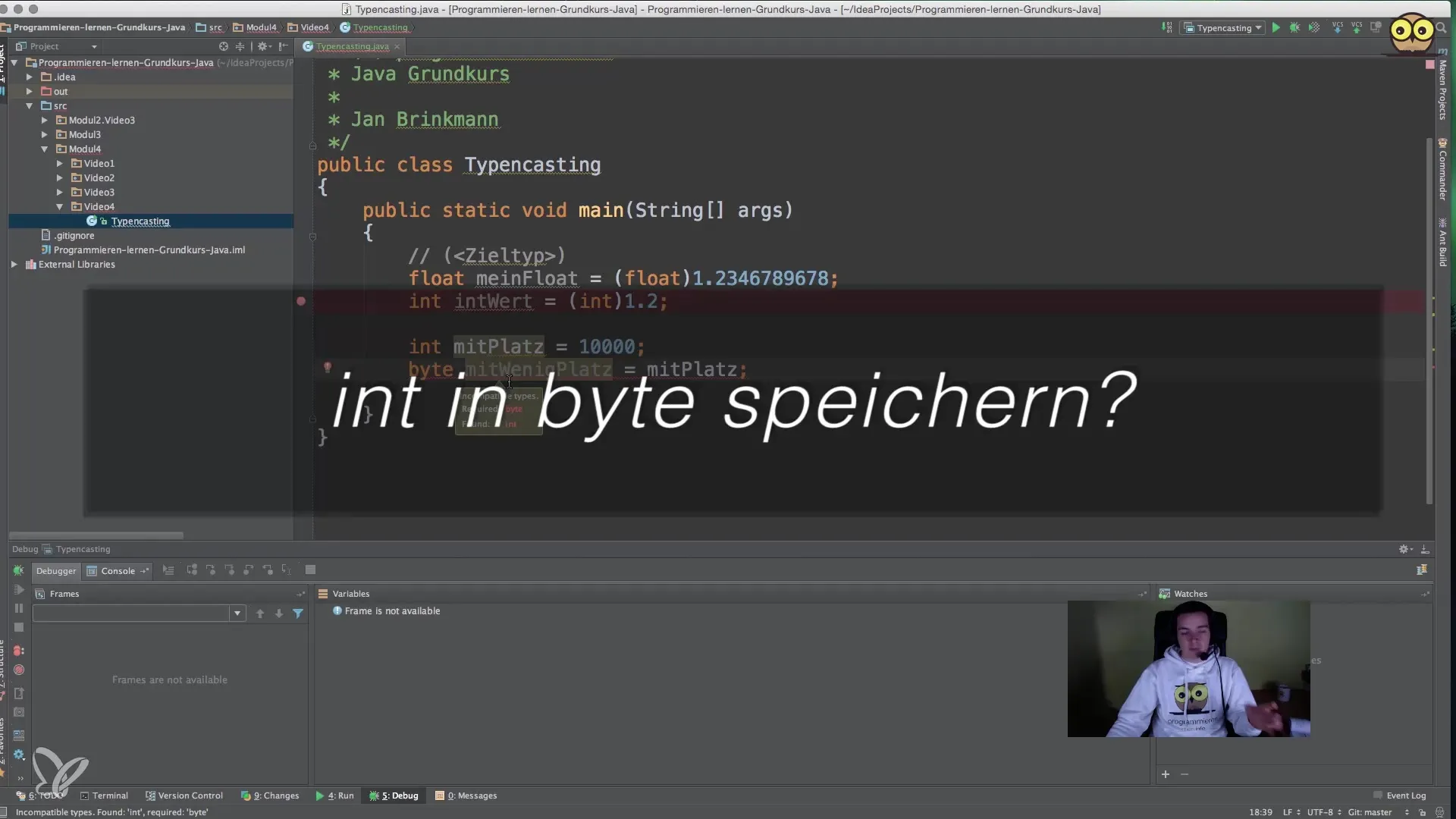
7. High to low data type
Casting from a data type with less memory space to a larger one usually does not cause an error, as long as no information is lost. For example, you can cast a byte value into an Integer without Java raising any issues.
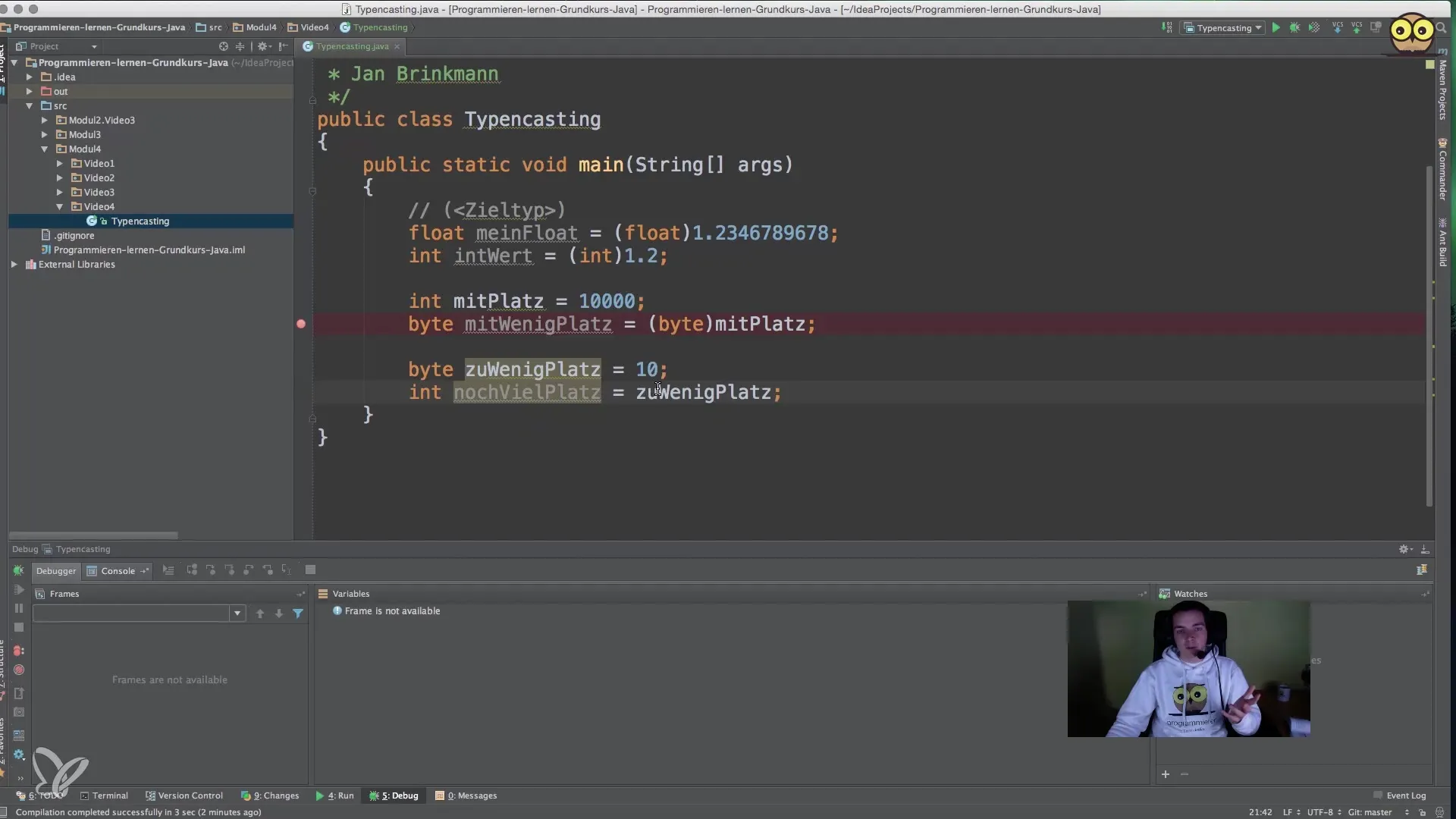
8. Summary considerations
It is crucial to develop a good understanding of data types and their scales to avoid unexpected casting problems. You should always be cautious when attempting to cast from a larger to a smaller data type to ensure that no sensitive information is lost.
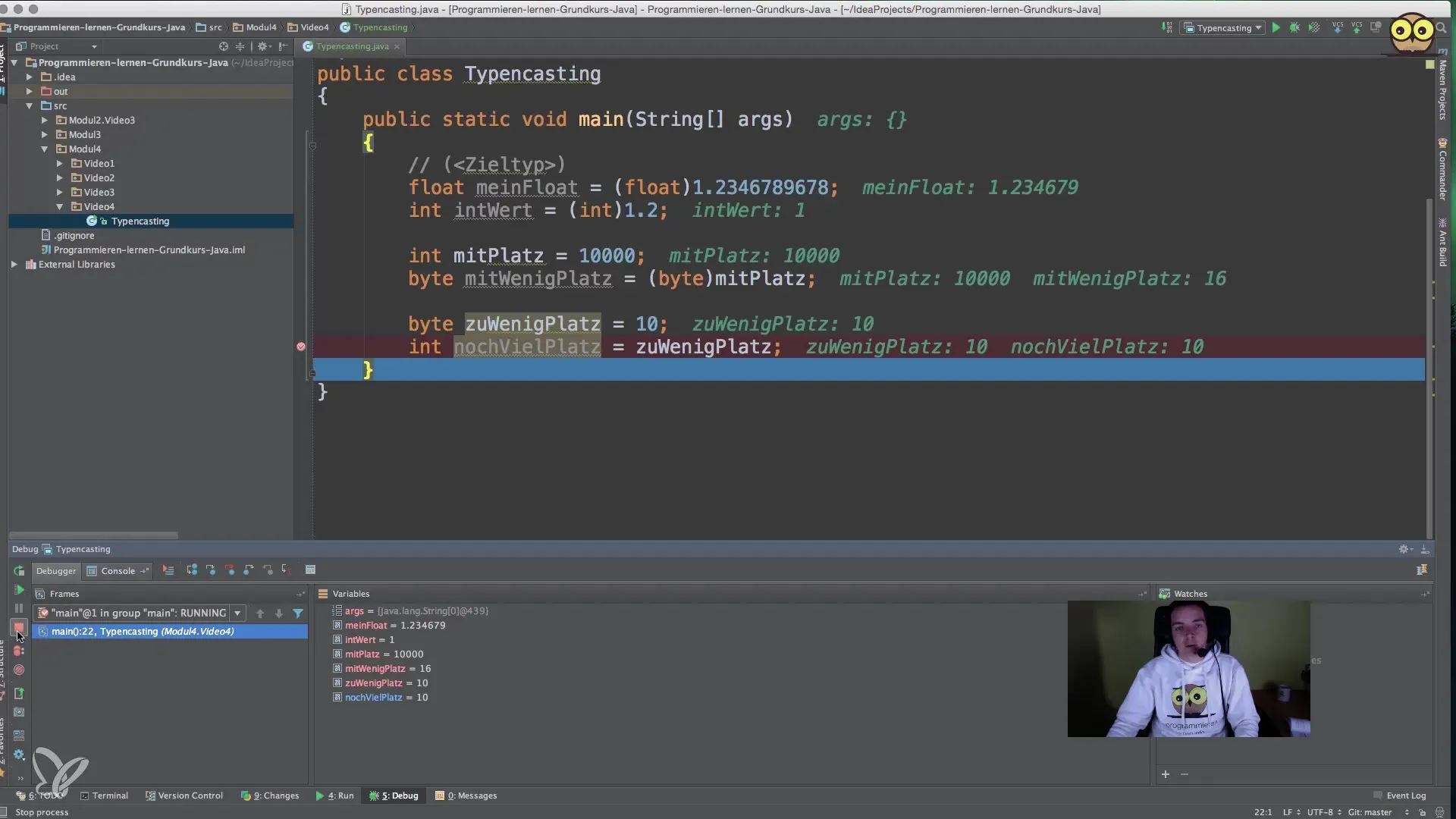
Summary – Type casting in Java
Type casting is an essential skill in Java to switch between different data types. You have learned that information can be lost when you cast from a larger data type to a smaller one. This can easily lead to errors in programming if you are not careful. Therefore, it is essential to understand what happens during casting and how you can utilize it effectively.
Frequently Asked Questions
What is type casting in Java?Type casting is the conversion of one data type into another in Java.
How does implicit casting work?Implicit casting happens automatically when you assign a smaller data type (e.g., Integer) to a larger data type (e.g., Double).
How does explicit casting work?You need explicit casting when you want to convert a larger data type to a smaller one, for example, from Double to Integer, by writing (int) before the expression.
What happens with information loss during casting?When you switch from a larger to a smaller data type, measures such as decimal places can be lost.
Can I cast a byte value to an Integer?Yes, this is possible and generally does not cause information loss as Integers offer more space.