Inheritance is a central principle of object-oriented programming and allows you to extend existing classes and add new functionalities without having to implement everything from scratch. In this guide, you will learn how inheritance works in Java and how to use it effectively.
Key Insights
Inheritance allows you to derive a new class (subclass) from an existing class (superclass). This enables you to reuse properties and methods of the superclass in the subclass and make specific additions. In this tutorial, you will learn the following:
- Creating a base class with common properties.
- Deriving further classes to add specific properties.
- Using methods in the derived classes.
Step 1: Creating the Base Class "Vehicle"
First, we create the base class "Vehicle," which defines common properties such as the number of wheels and the color. This ensures that all vehicles have these attributes.
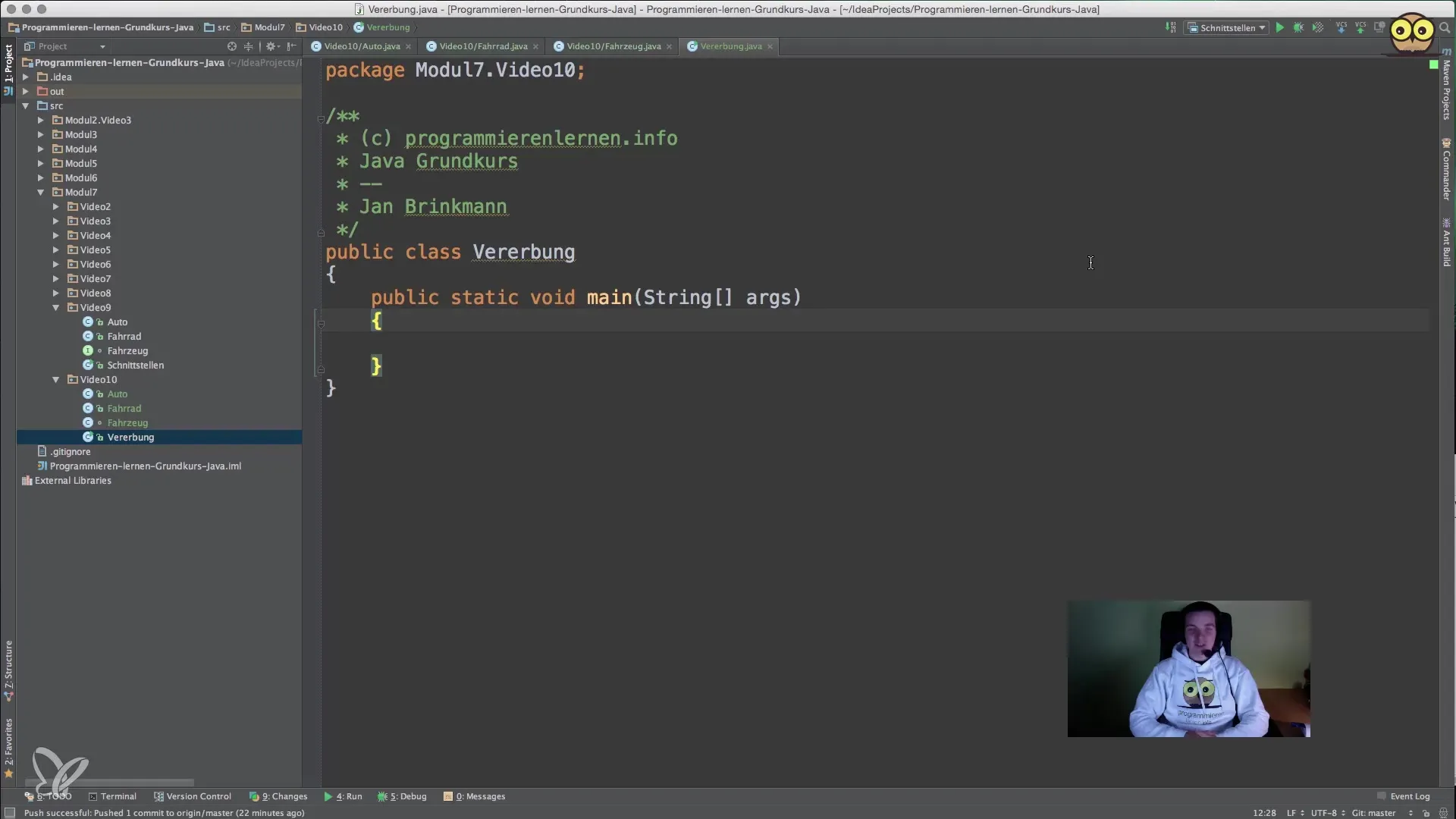
Step 2: Deriving the Classes "Car" and "Bicycle"
Now we derive the classes “Car” and “Bicycle” from the base class “Vehicle.” The keyword extends makes it clear that these classes inherit the properties of the base class.
In both classes, we can add specific methods that are only relevant to each class, such as the method triggerAirbag for the car and ringBell for the bicycle.
Step 3: Instantiation and Usage of the Classes
Now that our classes are defined, we will create instances of “Car” and “Bicycle” and call the inherited and specific methods.
Here you can see that both myCar and myBike use the method setColor, which is defined in the class “Vehicle.” The specific methods triggerAirbag and ringBell are also utilized.
Summary - Inheritance in Java: Basics and Application
Inheritance is a powerful feature in Java that enables you to extend existing classes for specific needs. The ability to inherit methods and attributes from a base class promotes code reusability and reduces redundant implementations. This is particularly useful for creating a clear and easily maintainable software architecture.
Frequently Asked Questions
What is the advantage of inheritance in Java?Inheritance allows you to organize, structure, and reuse code, thereby reducing development effort.
How does the keyword extends work?The keyword extends is used to derive a class from another, thereby inheriting its properties and methods.
Can a class inherit from multiple classes?In Java, multiple inheritance through classes is not possible; however, you can use interfaces to achieve similar functionality.
What happens if I override a method in the subclass?If you override a method in the subclass, the implementation of the base class is replaced by the implementation of the subclass.
What role do access modifiers play in inheritance?Access modifiers determine how properties and methods are visible in subclasses, with protected and public being the most common modes that allow inheriting classes to access them.