Click-events are a fundamental concept in programming with JavaScript. They allow you to respond to user interactions and create dynamic content. In this guide, you will learn how to handle click events efficiently by applying best practices and separating your JavaScript logic from your HTML code.
Key insights
- Directly embedding functions in event handlers should be avoided.
- Separating HTML, CSS, and JavaScript improves code maintainability.
- Using event handler IDs allows for reusability in different contexts.
Step-by-step guide
Step 1: Understand the basics
Dealing with click events simply requires a basic understanding of how JavaScript interacts with HTML elements. First, you should be aware that functions meant to trigger events should not be placed directly in the HTML code. This keeps the code clear.
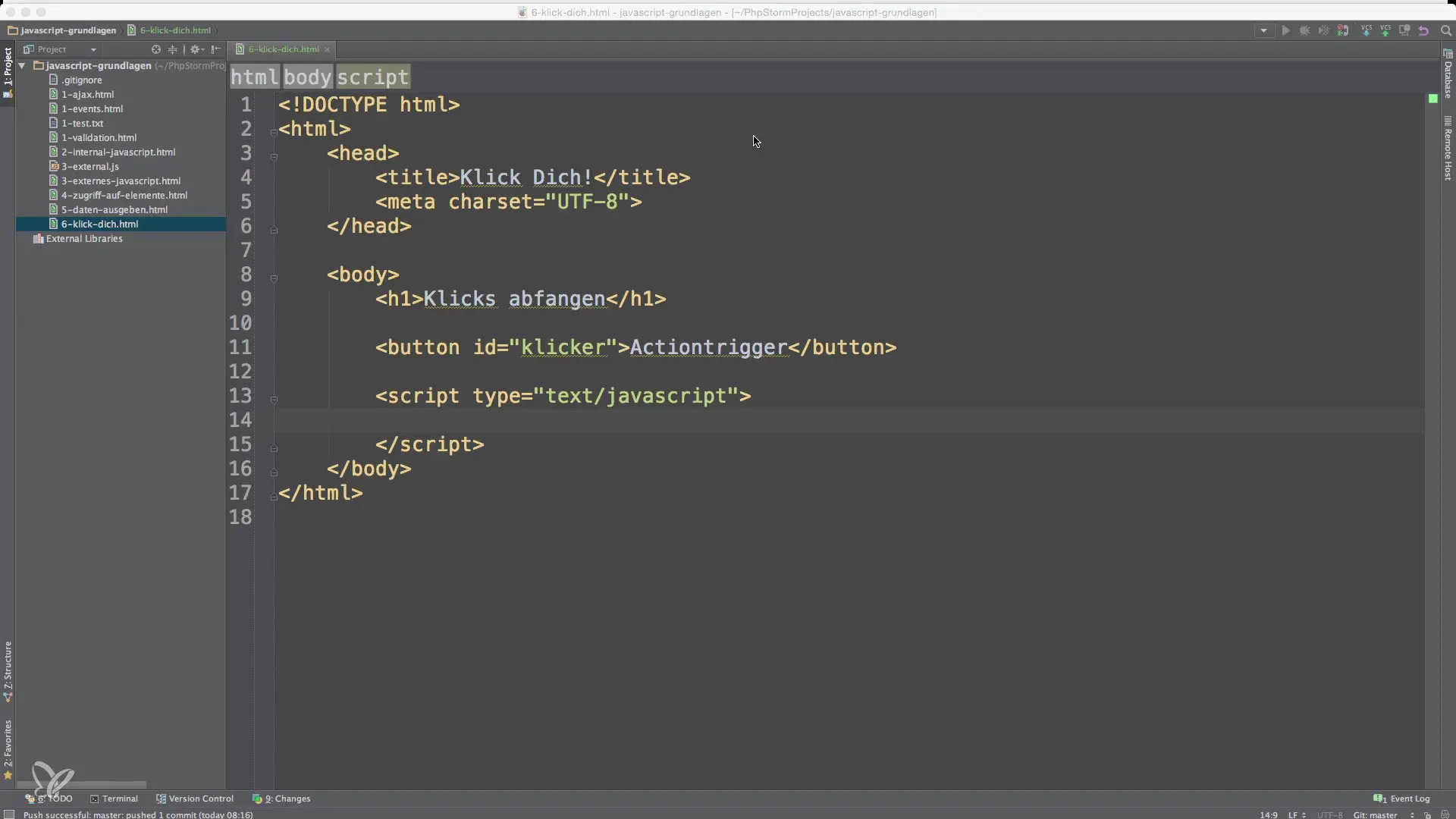
Step 2: Create a simple example
To do this, first, we create a button that changes the URL of the current page. Set the onclick handler directly on the button. Although this seems simple, it can quickly become unclear. Use static links to make the logic more understandable.
Step 3: Separate the function
Instead of writing the logic directly in the onclick handler, create a separate function. Name it something like locationHandler. This function could then provide hints for the user and change the URL.
You can extend the function to also handle AJAX calls or cookies, further increasing reusability.
Step 4: Link the event handler
Now you link the button to your function by using the getElementById method. This allows you to manage the onclick handler better.
Make sure you pass only the function name, not the function call itself. If you attach parentheses, the function will be executed immediately when the page loads, instead of only on a click.
Step 5: Provide user feedback
To improve user experience, you can provide a hint that informs the user before leaving the page. This contributes to user-friendliness and keeps the code clear.
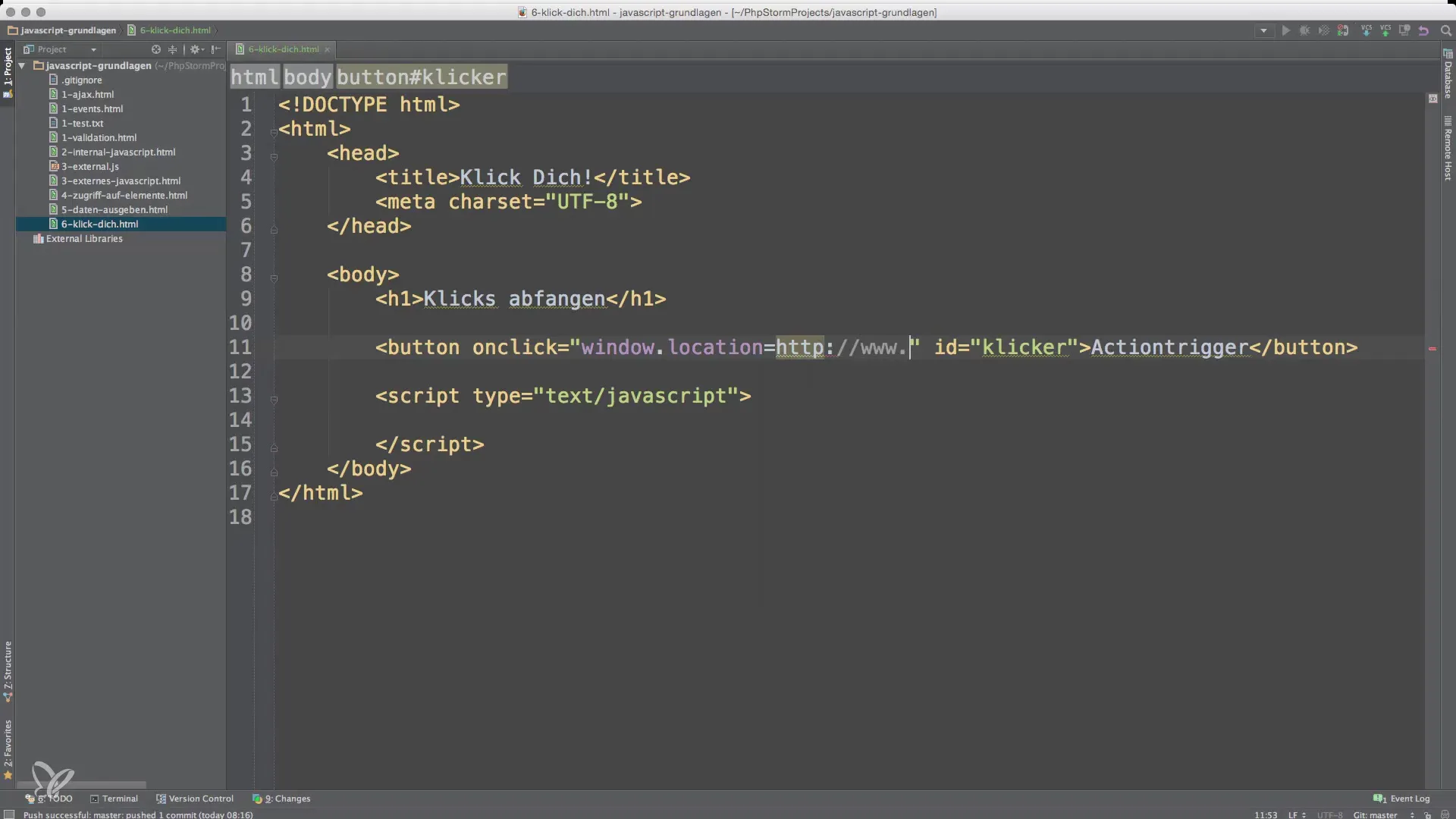
Step 6: Separation of HTML and JavaScript
Another important aspect is the separation of JavaScript and HTML. Ideally, you want JavaScript to be in a separate file to increase maintainability and reusability. Separating into different files is a good principle to follow when programming.
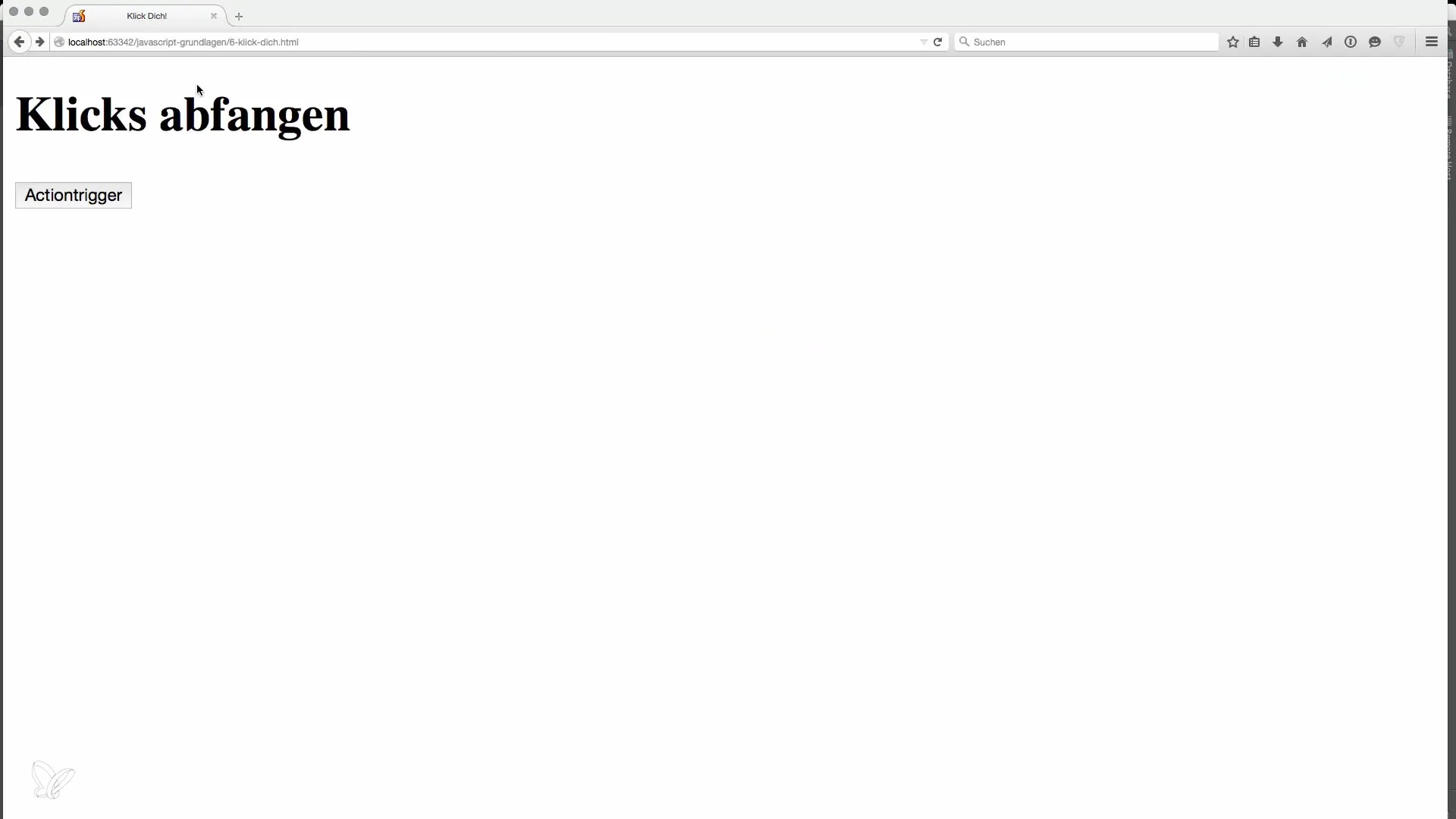
Step 7: Principles of reusability
By linking exactly one handler with different elements, you can improve your code. Instead of repeatedly writing code that calls the same function in different contexts, create a universal handler that responds to clicks on different elements.
This approach promotes clarity and understandability of your JavaScript and leads to a lower likelihood of errors in the long run.
Step 8: Conclusion and next steps
After learning these basics, you will be able to use click events effectively and work with them. It is advisable to apply the learned concepts in a small project to solidify the knowledge.
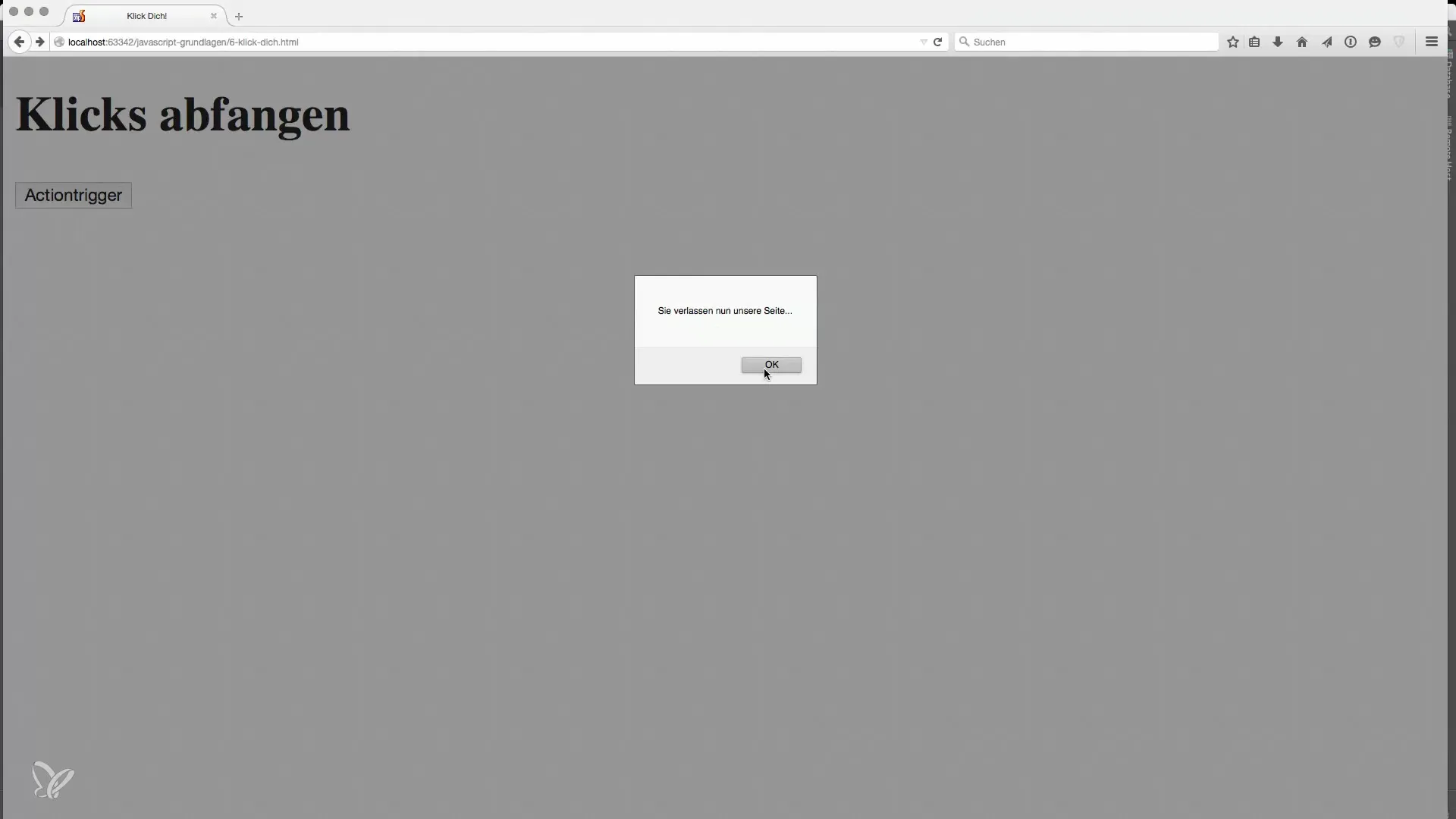
Summary – Fundamentals of JavaScript: Handling click events effectively
In this guide, you have learned the basics of handling click events in JavaScript. You learned how to create event handlers, separate them from HTML, and increase code maintainability. Use the discussed practices to further develop your JavaScript skills.
Frequently Asked Questions
How can I handle multiple click events on different buttons?By assigning the same function to multiple IDs, you allow the same behavior for different elements.
Why should I separate JavaScript from HTML?The separation improves maintainability, readability, and reusability of the code.
What are alternatives to onclick?There are many other events in JavaScript, such as onmouseover, onmouseout, and onchange, that you can use for different user interactions.