String data is an integral part of programming, especially in JavaScript, where strings are frequently used. They can be found in almost every application and play a crucial role in data processing. In this tutorial, we will take a look at how strings work in JavaScript, what problems may arise, and how you can use them effectively.
Main findings
- Strings are simple sequences of characters that are represented in double or single quotes.
- Special characters can mark the end of the string in strings; this is where escaping comes into play.
- JavaScript offers numerous methods to work with strings, such as length, indexOf, toUpperCase, and replace.
Step-by-step guide
1. Introduction to strings
Start with defining a string. In JavaScript, strings can be represented in either single (') or double (") quotes. For example:
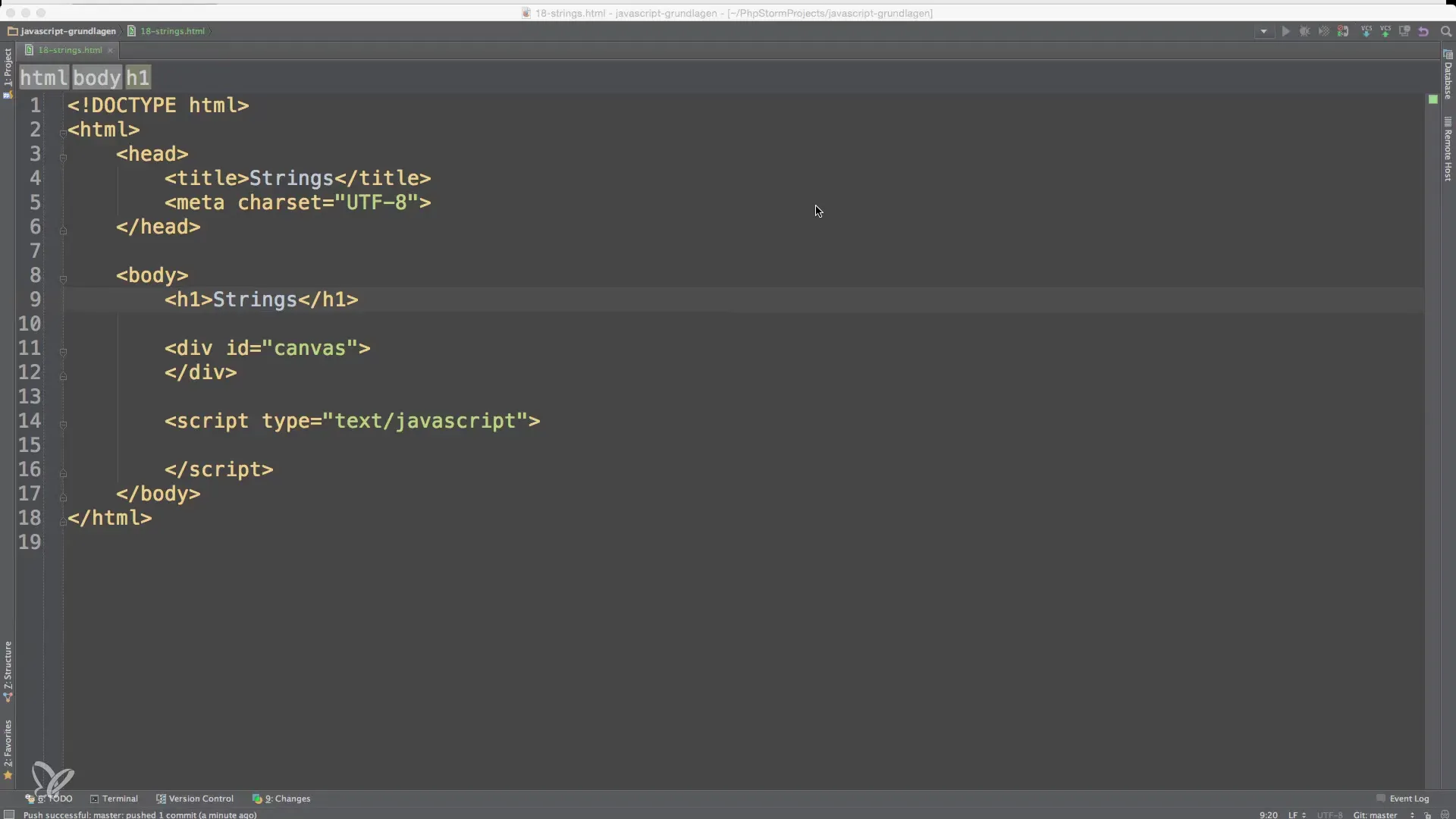
Now you have created a simple string.
2. Issues with special characters
When creating strings, problems may also arise, especially if they contain special characters such as quotes. If you try to create a string with an embedded quote, JavaScript interprets this as the end of the string.
To avoid these problems, you use what's called "escaping." Escaping is achieved with a backslash (\), which tells the JavaScript interpreter to treat the following character as part of the string.
3. Using escape sequences
With escape sequences, you can also insert other special characters into strings, such as the backslash itself.
If you need a backslash in a string, you must use two backslashes to represent one.
4. Methods for strings
Strings in JavaScript are also objects, which means you can use various methods. One of them is length, which returns the length of the string.
Another useful method is indexOf, which returns the first index of a specific character or string within a string.
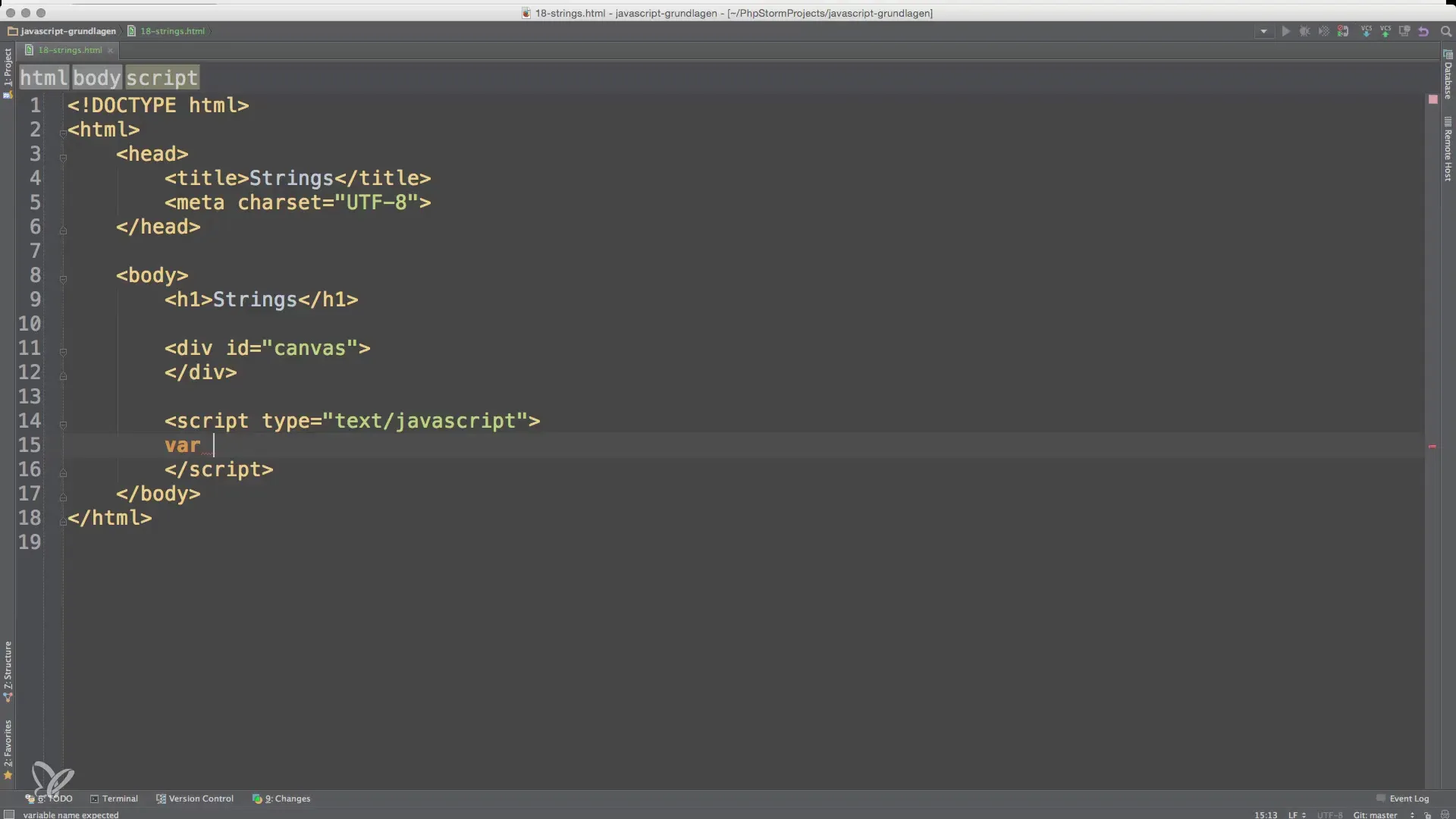
5. Validating email addresses
To check if an email address is valid, you can use the indexOf method together with an if condition. A value that returns null means that the character is not present, making your condition false.
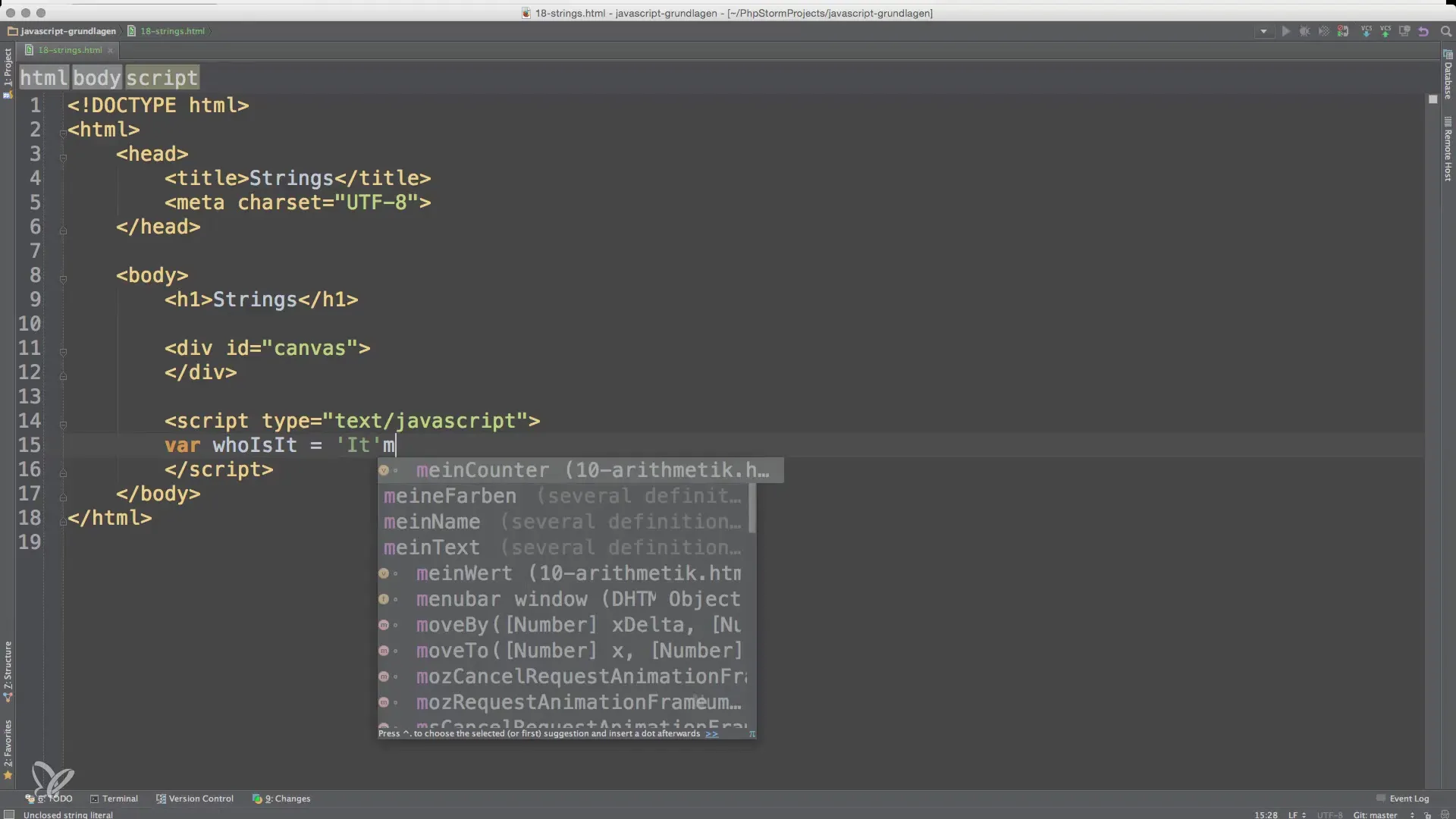
6. Manipulating strings
This leads us to functions like toUpperCase and toLowerCase, which allow you to convert the letters of a string to uppercase or lowercase.
Another operation is replace, which allows you to replace parts of a string. Remember that replace does not change input directly in the variable. You need to store the new value.
7. Dot notation for methods and properties
JavaScript uses dot notation to access methods and properties of an object. With strings, it is important to understand this syntax and use it effectively.
Here,.length returns the number of characters in the string.
Summary - Strings in JavaScript: Basics and Application
In this guide, you learned about the various aspects of strings in JavaScript, from their significance through issues with special characters to functions for manipulation and validation. You saw how to work effectively with strings and which methods can assist you in that.
Frequently Asked Questions
How do I define a string in JavaScript?A string can be created in JavaScript using single or double quotes.
What is escaping?Escaping is the process of treating a character, like a quotation mark, in a string without terminating it.
How can I determine the length of a string?You can get the length of a string by using the length property of the string.
How do I check if a character is present in a string?Use the indexOf method, which returns the index of the character or -1 if it is not present.
How can I replace parts of a string?You can use the replace method to swap parts of a string.