The development of graphical user interfaces (GUIs) with JavaFX is an exciting challenge that allows you to create user-friendly applications. In this tutorial, you will learn how to effectively use text fields (TextField) and layout management with GridPane. You will be guided step by step through the process of creating an application where users can enter their name and age. By the end, you will have basic knowledge of layouts and handling input fields in JavaFX.
Key Insights
- GridPane allows you to arrange controls in a grid.
- TextField is an interactive input field for user data.
- The proper positioning of GUI elements is crucial for usability.
Step-by-Step Guide
Step 1: Clean Up and Create GridPane
First, you clean up your existing code to make space for new elements. Remove all previous scenes and layouts that are no longer needed.
GridPane is an important component as it allows you to organize your UI elements in a structured grid. Cleaning up is essential to ensure clarity.
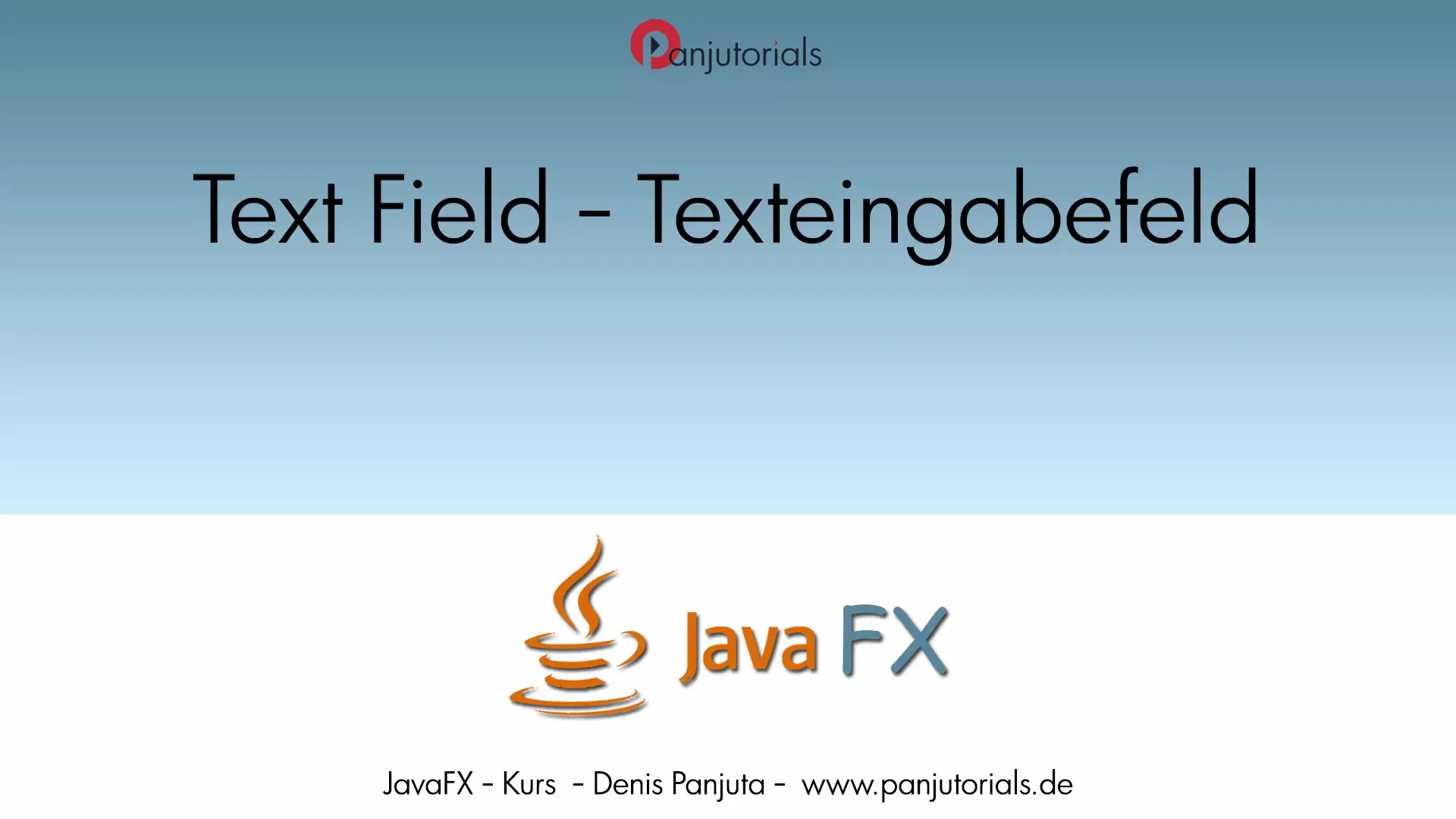
Step 2: Set Padding and Spacing
To ensure that the layout looks as you envision, you can add padding and spacing.
The padding ensures that there are spaces between the edges of the GridPane and the contained elements.
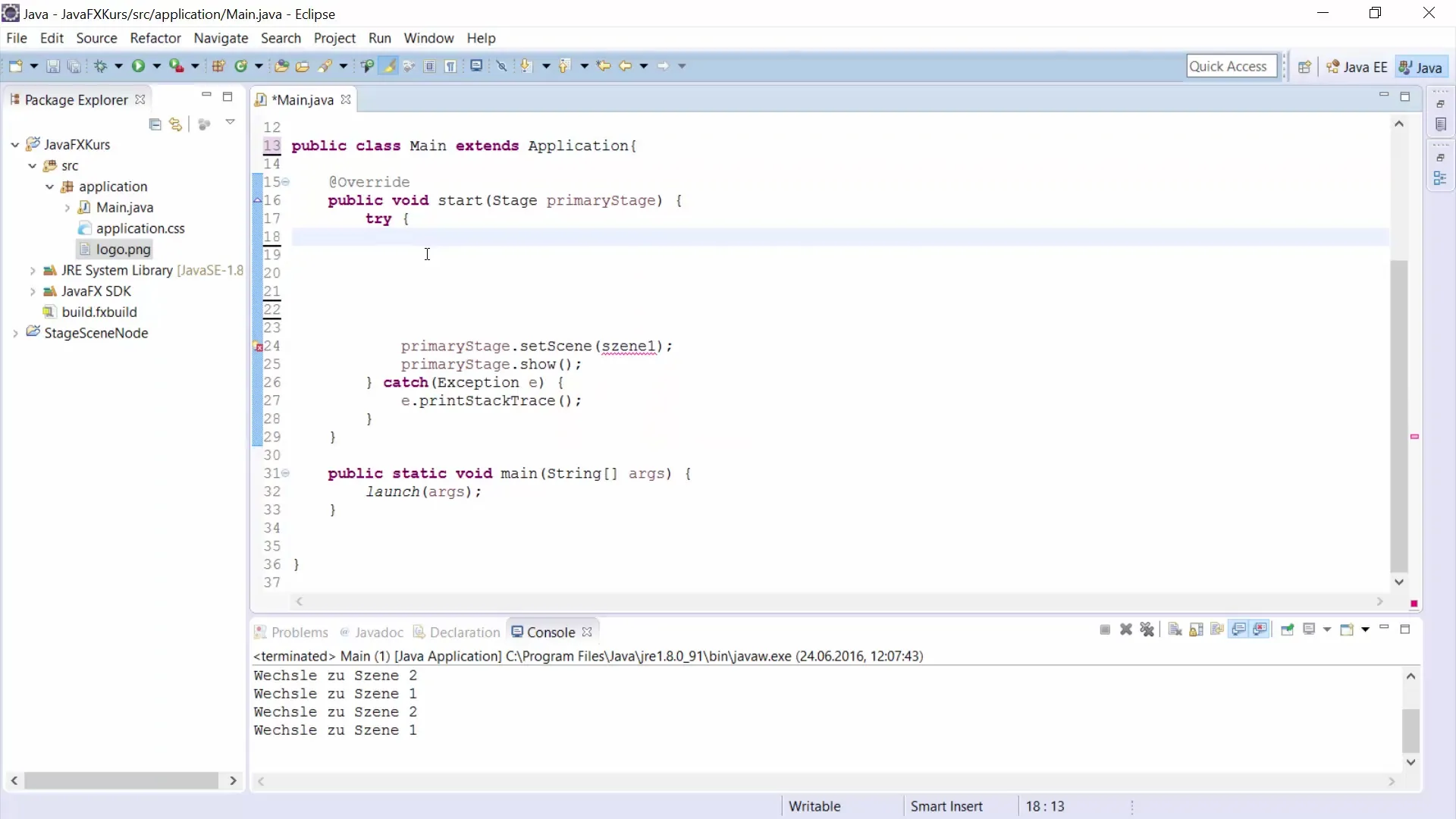
With these values, you ensure a clear and easily readable arrangement of your components.
Step 3: Create Elements in GridPane
Add the labels and text fields that you need.
The prompting in the TextField helps users understand what they should enter.
Here, you define the positions in the grid layout.
Step 4: Add More Elements
Make sure to place the new elements in the correct positions in the GridPane.
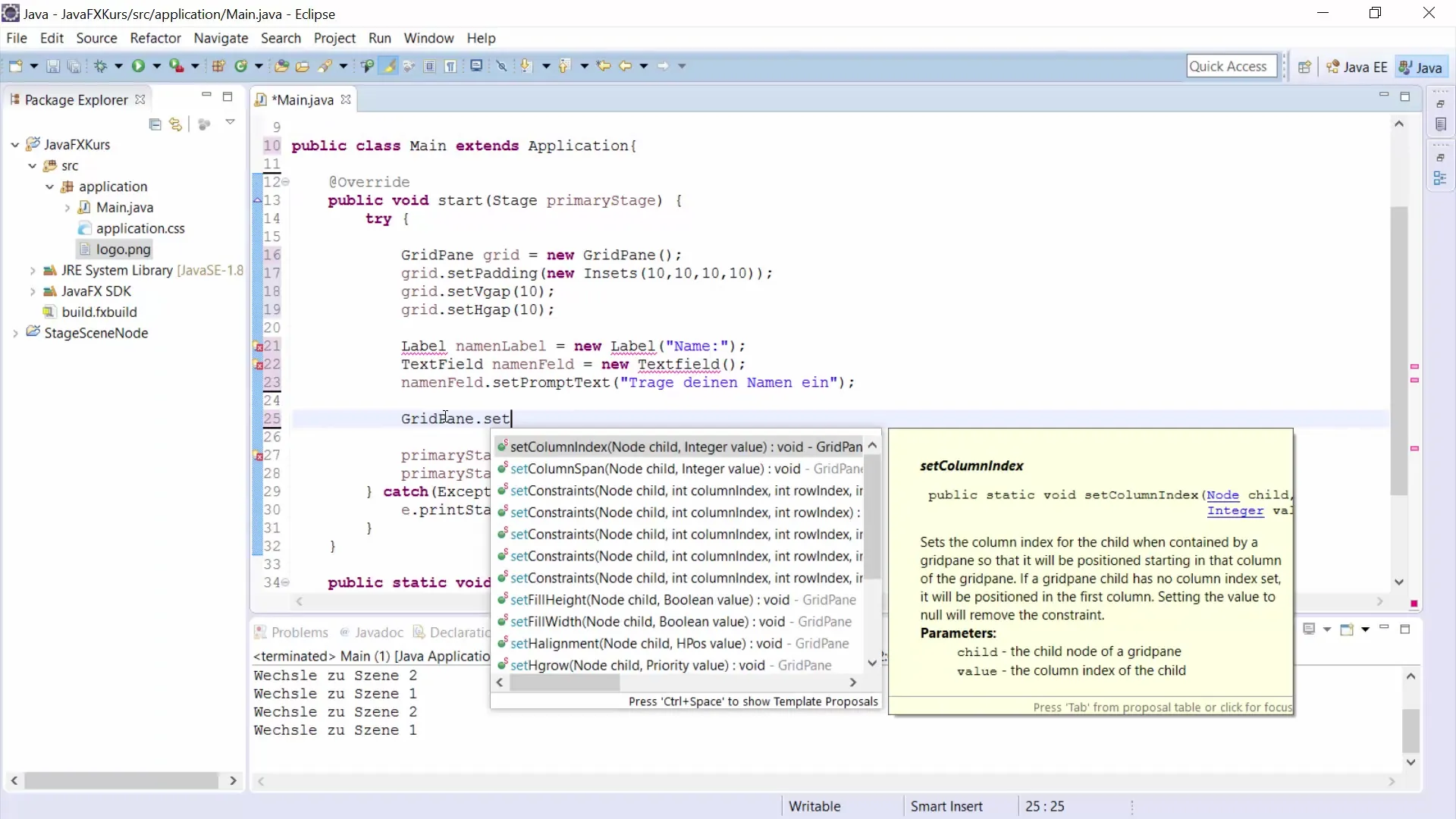
Step 5: Create a Button for Input
This button should trigger an action when clicked.
This code checks whether the fields are filled and outputs the values to the console.
Step 6: Add All Elements to GridPane
Ensure that the button is placed below the text fields to provide logical user guidance.
Step 7: Set Up and Display the Scene
The size of the button and the layout are crucial for making your window user-friendly.
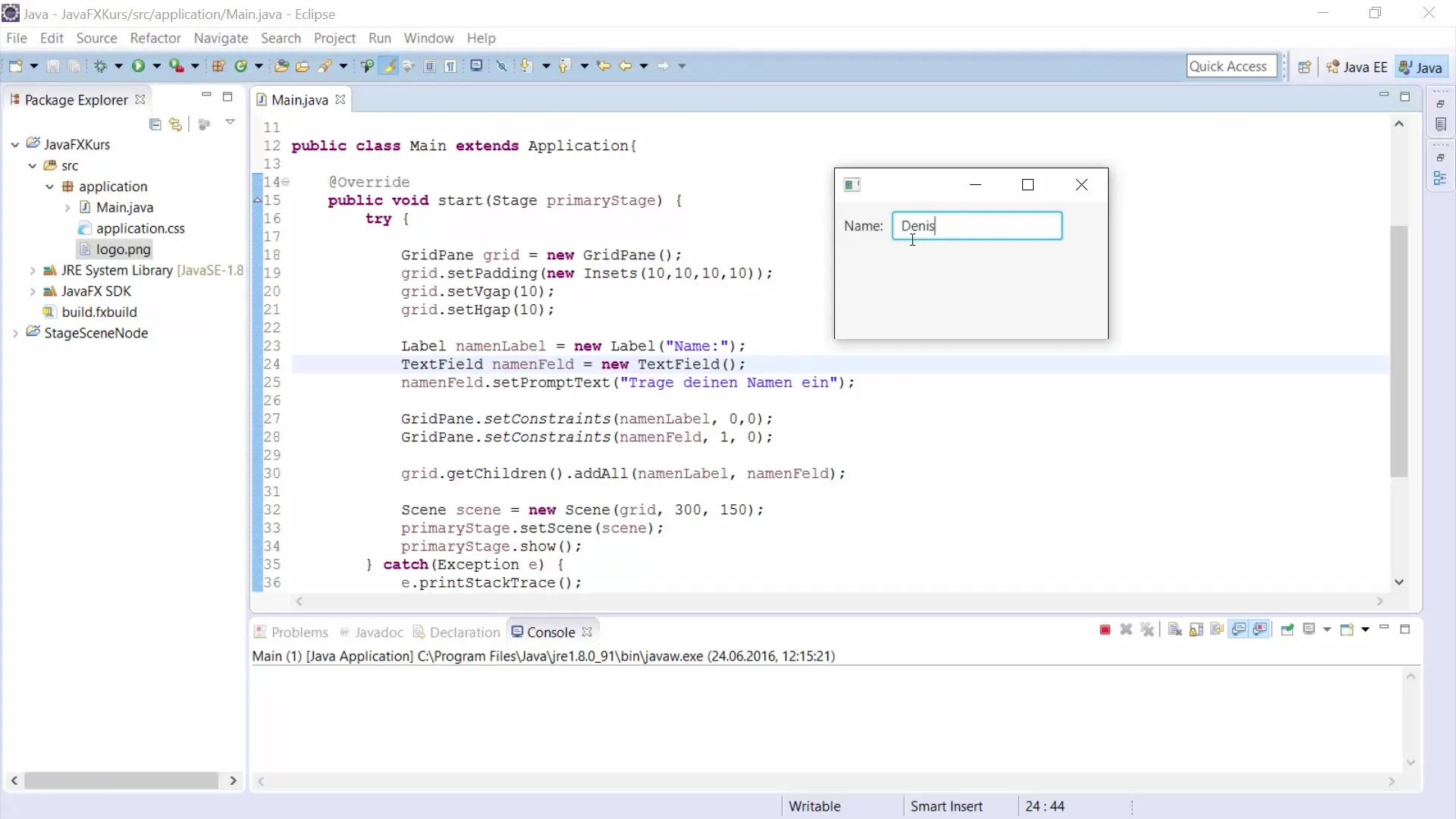
Summary – JavaFX GUI Development: Effectively Using TextField and GridPane
In this tutorial, you learned how to use TextFields and GridPane with JavaFX to create a simple GUI where users can input data. You learned the technique for arranging GUI elements in a GridPane and how important it is to set spacing and padding correctly to enable an appealing user interface. These fundamentals form the basis for more complex applications that you can develop in the future.
Frequently Asked Questions
What is a GridPane?A GridPane is a layout container in JavaFX that allows you to arrange UI elements in a grid.
How do I add buttons to a GridPane?Buttons are created like other elements and then added to the GridPane using the add() method or getChildren().add().
How can I read text from a TextField?Use the getText() method of the TextField object to retrieve the entered text.
What happens if a TextField is empty?If the TextField is empty, you can perform a check and prompt the user to fill in both fields.
How can I adjust the spacing between elements in a GridPane?Use the setVgap() and setHgap() methods for vertical and horizontal spacing and setPadding() for outer spacing.