A Color Picker in JavaFX provides an effective way to allow users to select colors in a graphical user interface. You can select a color from a palette or define a custom color. In this tutorial, you will learn how to integrate a Color Picker into your JavaFX application to dynamically display colored elements. You will be using various color representations such as HSB, RGB, and hexadecimal.
Key Takeaways
- Using the Color Picker to select and display colors
- Implementing HSB and RGB color representations
- Managing transparency through an alpha value
- Customizing UI elements and their interactions
Step-by-Step Guide
To implement a Color Picker, start by creating the foundation of your JavaFX application.
1. Basic Setup of the Color Picker
First, create an instance of the Color Picker. This is done by creating a new Color Picker object.
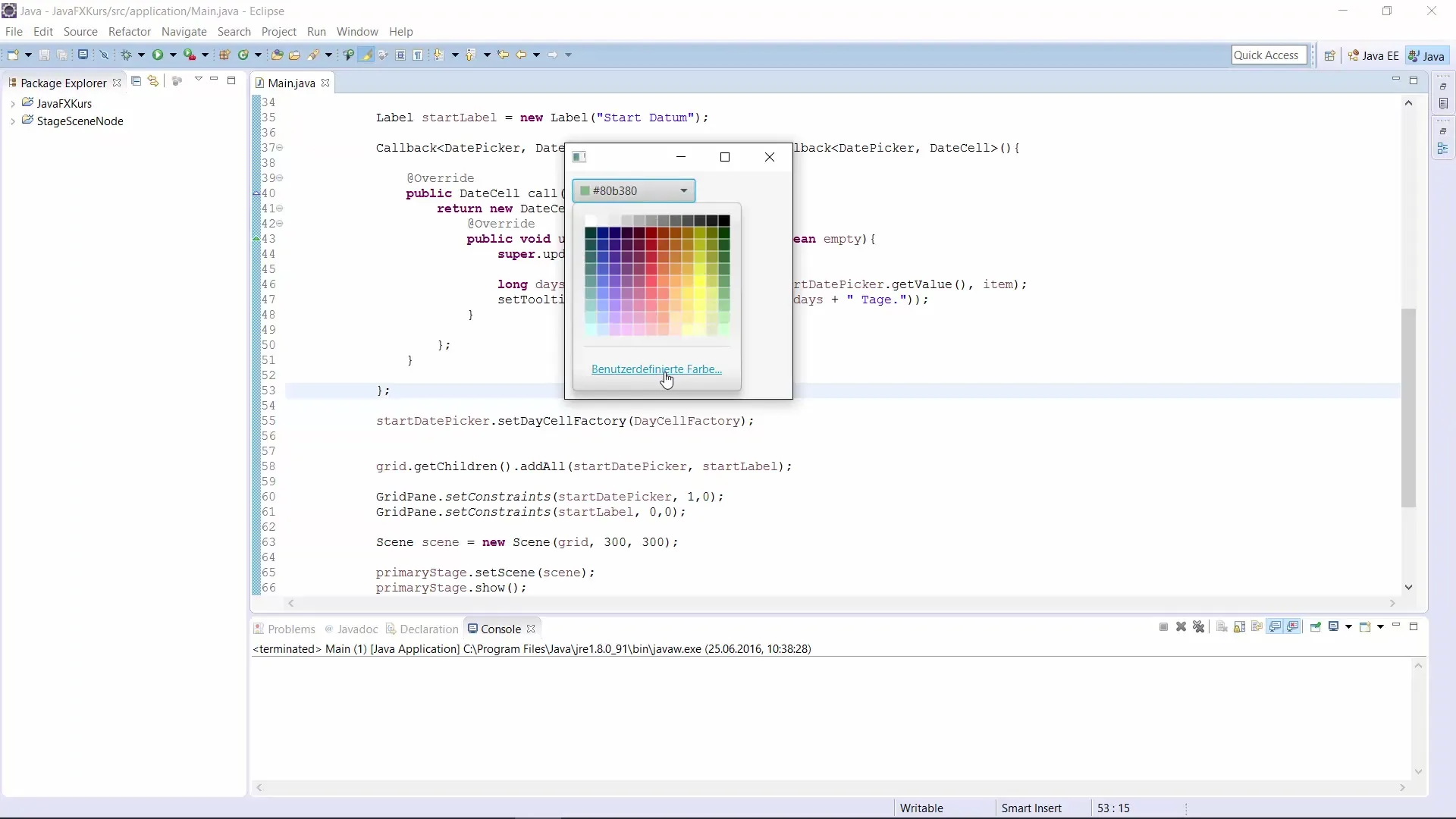
With these lines, you get a simple tool that allows you to interactively select different colors.
2. Adding Additional UI Components
To visually represent the selected color, add a rectangle. The rectangle should display the currently selected color. Create it with a size of 200x200.
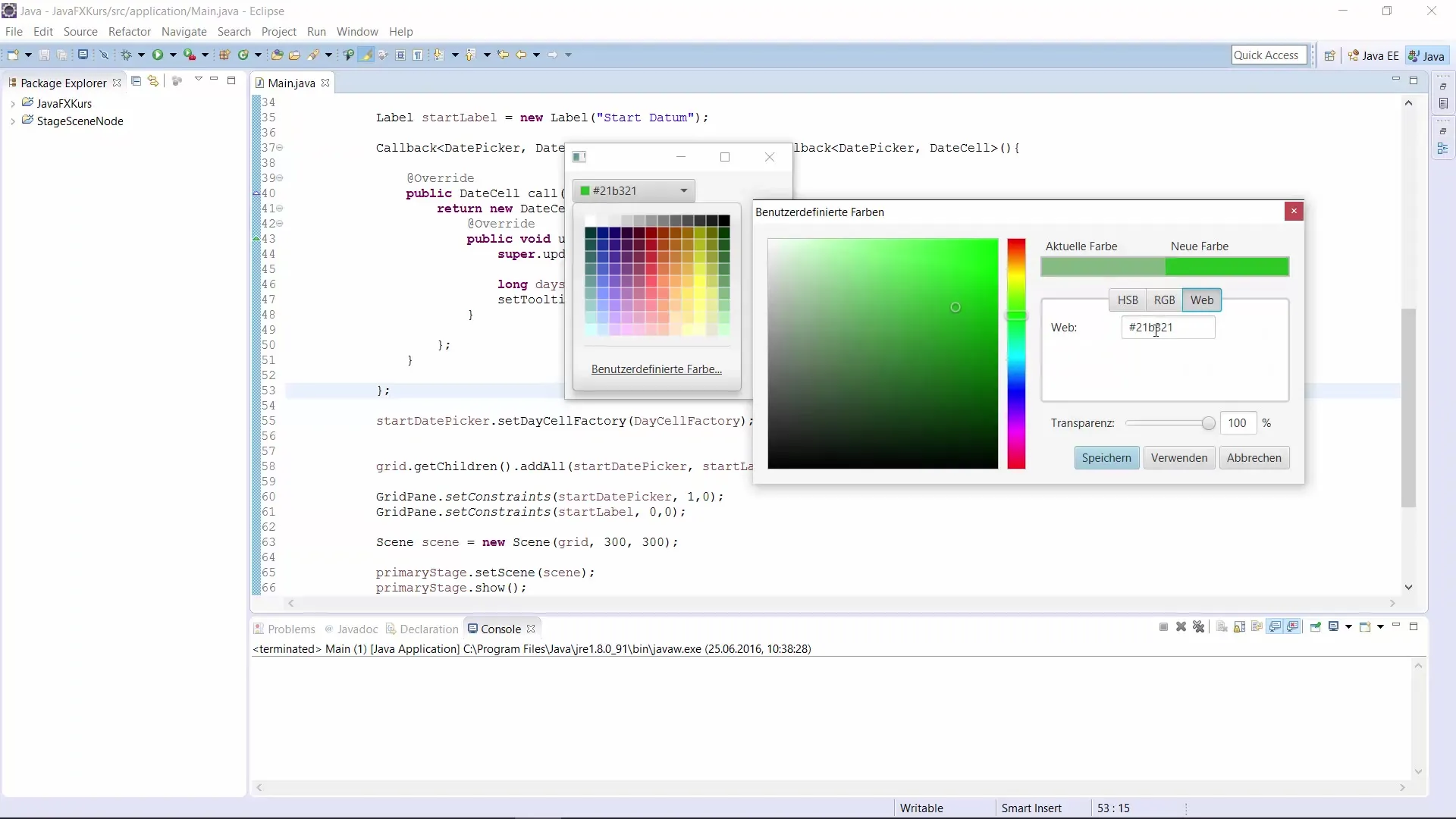
Now you will be able to dynamically display the selection in your layout after you have set the appropriate position of the rectangle.
3. Integrating the Rectangle into the User Interface
Import the necessary classes for the Rectangle and add it to your UI elements. When adding, make sure that it is inserted at the correct location in the scene.
If everything is built correctly, you can start the application, and both the Color Picker and the rectangle should be displayed.
4. Adding Functionality for Color Selection
To enable interactive color selection, you need to set an event that connects the Color Picker with your rectangle. This is done through the setOnAction() method.
An ActionEvent is used to fill the color of the rectangle with that of the Color Picker by using the getValue() function of the Color Picker.
5. Testing the Application
Test the application by selecting different colors in the Color Picker and observe how the color in the rectangle changes accordingly.
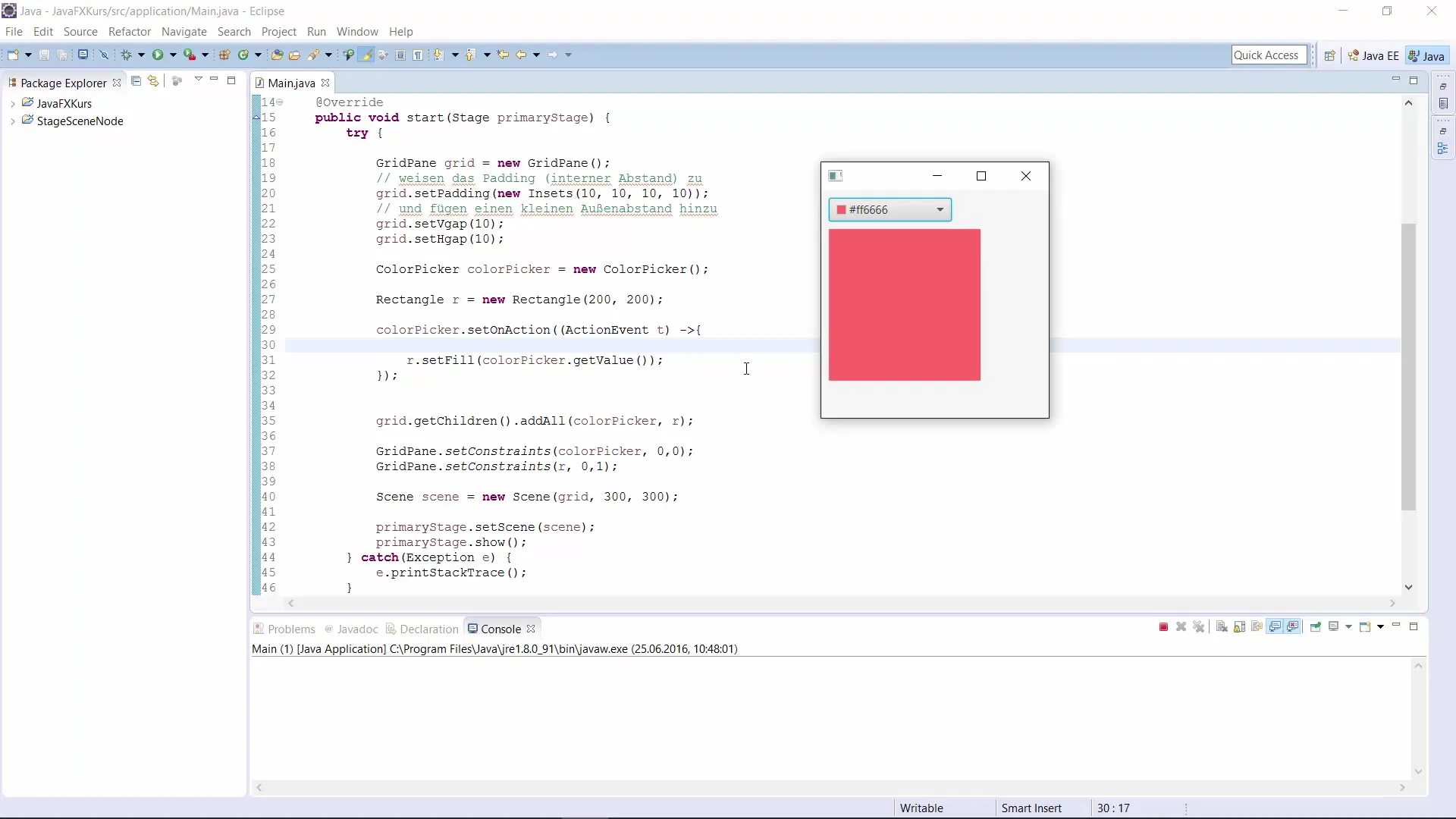
The implementation is now complete. You have successfully enabled color changes in your application and can use this technique for various projects, such as drawing applications.
Summary – Guide: Implementing a Color Picker in JavaFX
Implementing a Color Picker in JavaFX allows you to quickly and efficiently select colors in your application. With the steps you have followed, you can easily incorporate this functionality into your projects and create an appealing graphical user interface. Use the various color models and interactions to create a dynamic user experience.
Frequently Asked Questions
What is a Color Picker?A Color Picker is a UI element that allows users to select colors.
How can I adjust transparency in a Color Picker?You can set the alpha value to control the transparency of the selected color.
What color representations does the Color Picker use?The Color Picker supports HSB, RGB, and hexadecimal color values.
How do I display the selected color?By creating a rectangle in your user interface and setting its fill color to the color selected from the Color Picker.