In this tutorial, you will learn how to develop a simple color-mixing program using JavaFX sliders. The sliders allow users to adjust RGB values for colors using a graphical user interface. Let's get started!
Main Takeaways
- Inserting and configuring sliders in JavaFX
- Dynamic color adjustment based on slider values
- Styling the GUI for an appealing design
Step-by-Step Guide
Step 1: Setting Up the Development Environment
Start your Eclipse and create a new JavaFX project. Make sure you have imported the necessary libraries for JavaFX so you can use the GUI components without any issues.
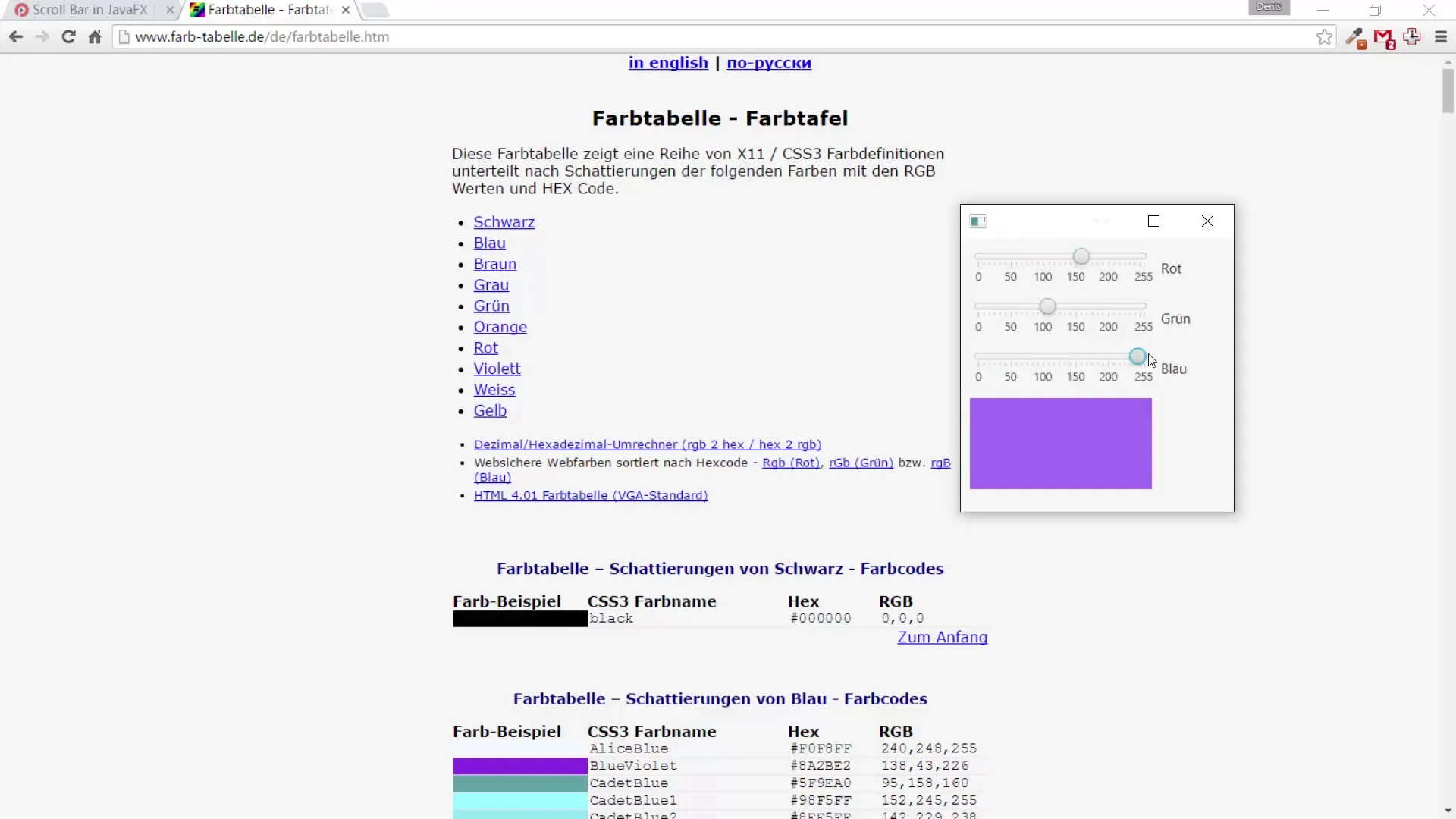
Step 2: Creating the Layout Foundation
Define a GridPane as the layout for your application. This makes it easier to arrange the various GUI elements.
Add the required insets for spacing between the GUI elements.
Step 3: Adding a Rectangle for Color Display
Now add a rectangle that displays the color. You can initially set it to black by setting the RGB values to 0.
Place the rectangle in the GridPane.
Step 4: Creating Sliders for RGB Values
Create three sliders, one for each color value (Red, Green, Blue). Set the minimum value to 0 and the maximum value to 255.
Step 5: Adding Labels
Add labels to indicate which slider corresponds to which color. Three labels should be created for Red, Green, and Blue.
Step 6: Adding Listeners to the Sliders
To change the color dynamically, add a listener to each slider. The listener responds to changes in the slider value and updates the color of the rectangle accordingly.
Step 7: Styling the GUI
To make the user interface more visually appealing, you can add styles to the sliders and the rectangle. For example, it might be useful to enable tick marks and labels for the sliders.
Step 8: Testing the Program
Run the program and test the sliders. You should be able to adjust the RGB values, and the color in the rectangle should change accordingly.
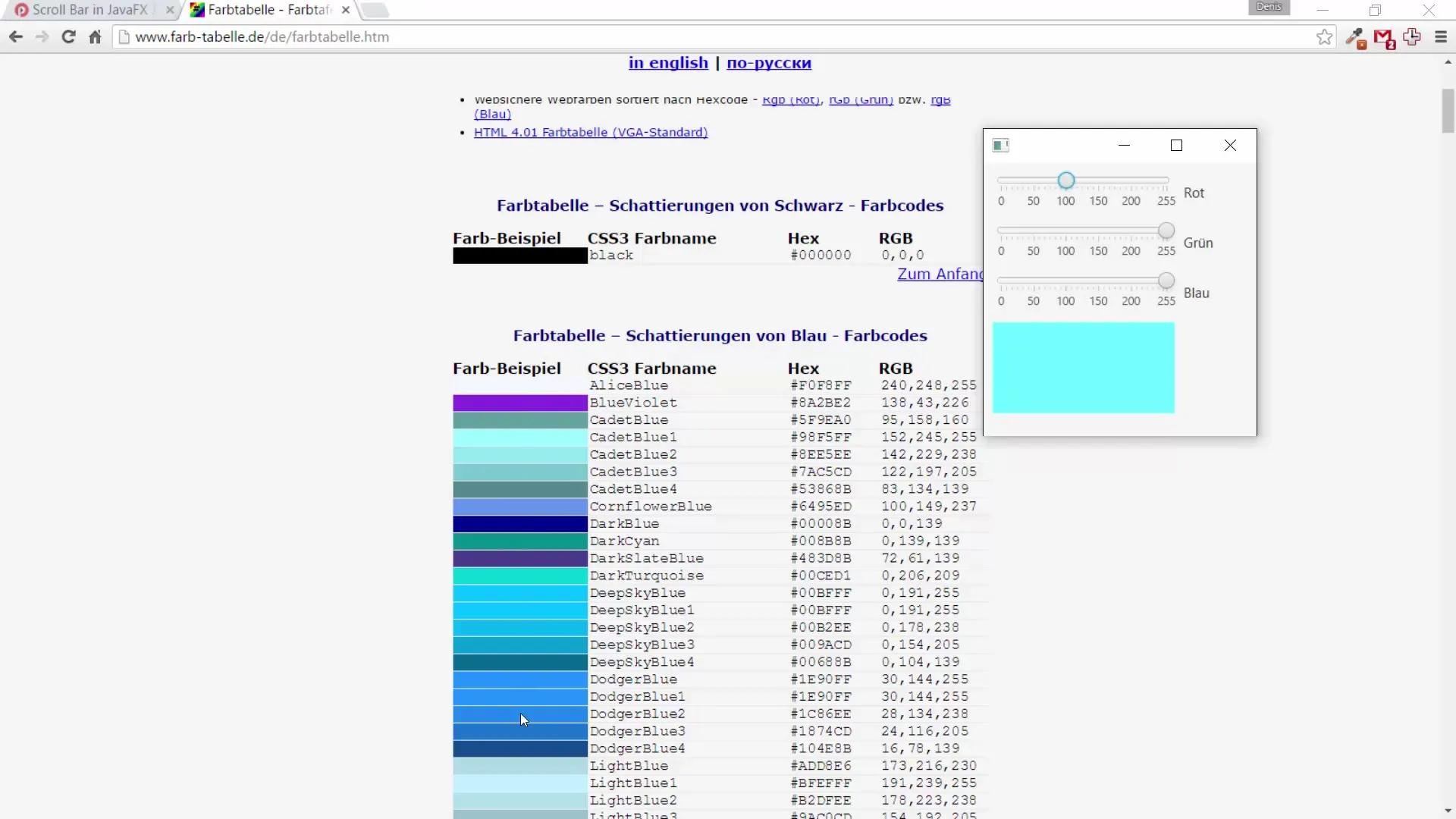
Summary - Color Mixing Program with Sliders in JavaFX
In this tutorial, you have learned how to insert sliders in JavaFX to create a color-mixing program. You have combined various GUI elements to develop an interactive user interface that responds to inputs.
Frequently Asked Questions
What is a slider in JavaFX?A slider is a control in JavaFX that allows users to select a value within a certain range.
How can I read the values of the sliders?You can read the values using the getValue() method of the slider object.
Can I add more colors?Yes, you can add additional sliders for other colors by repeating the process for the RGB value.
How do I change the layout of the GUI?You can change the arrangement of the elements in the GridPane by adjusting the row and column indices in the add() method.
What are tick marks and how do they work?Tick marks are visual markers on a slider that help clarify the position of the value. They can be added by using setShowTickMarks(true).