No matter whether you are working on a small project or a comprehensive application, the user interface always plays a crucial role. In this guide, you will learn how to create a scrollable view in JavaFX using ScrollPane. This allows you to conveniently organize and present content.
Key Insights
- ScrollPane allows you to insert scrollable areas into your application.
- The scroll bar can be configured in different directions (horizontal and vertical).
- With the right listener implementation, you can dynamically update content in ScrollPane.
Step-by-Step Guide
To successfully implement a ScrollPane in your JavaFX application, proceed as follows:
First, create a large image that will later be used in the ScrollPane. You can use the command new Image(getClass().getResourceAsStream("build.jpack")) to load the image. The image could be a sample image for your application.
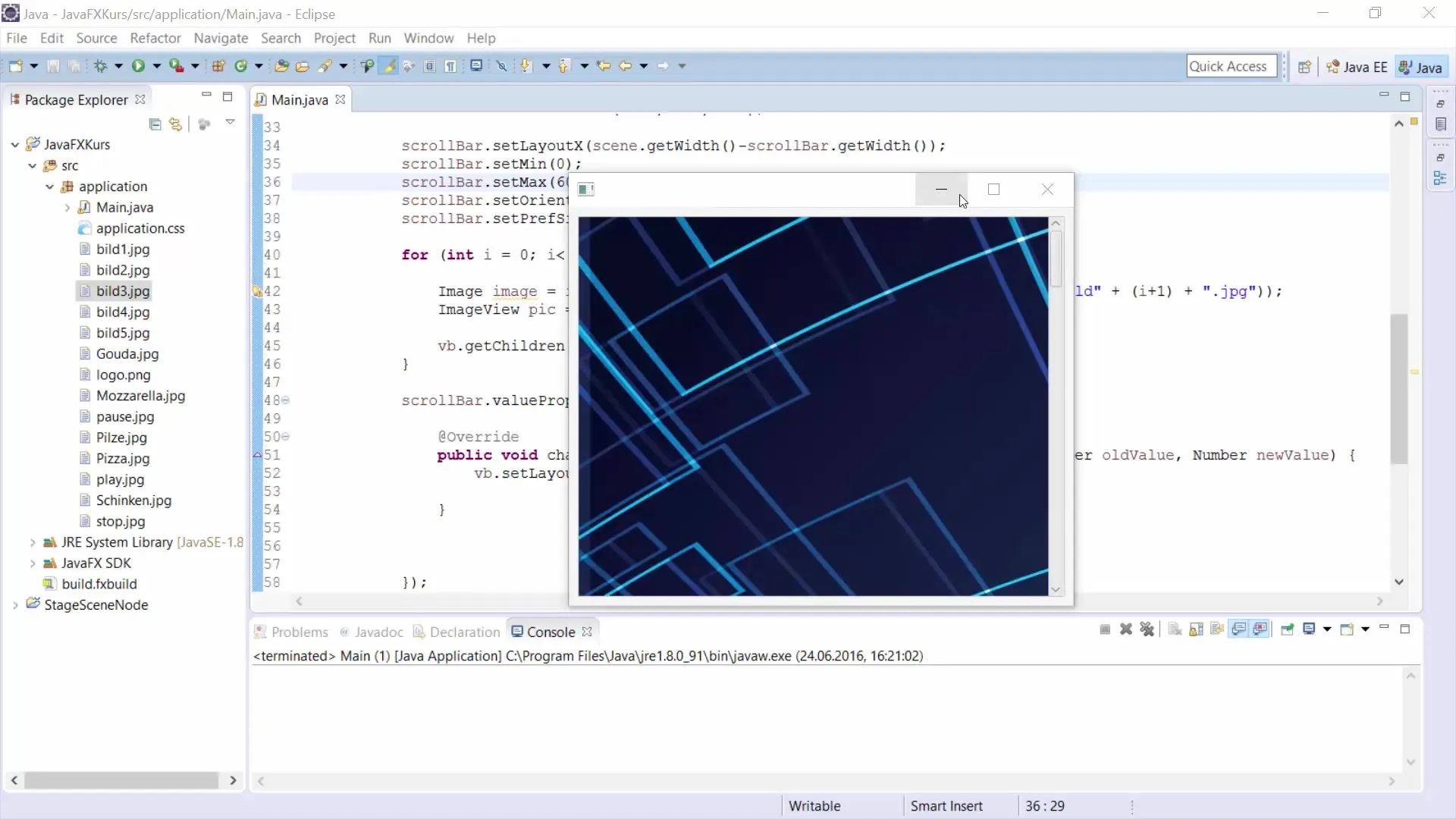
After creating the image, define a ScrollPane where you want to insert the content. Create a new ScrollPane with ScrollPane sp = new ScrollPane() and add the ImageView element that displays your image.
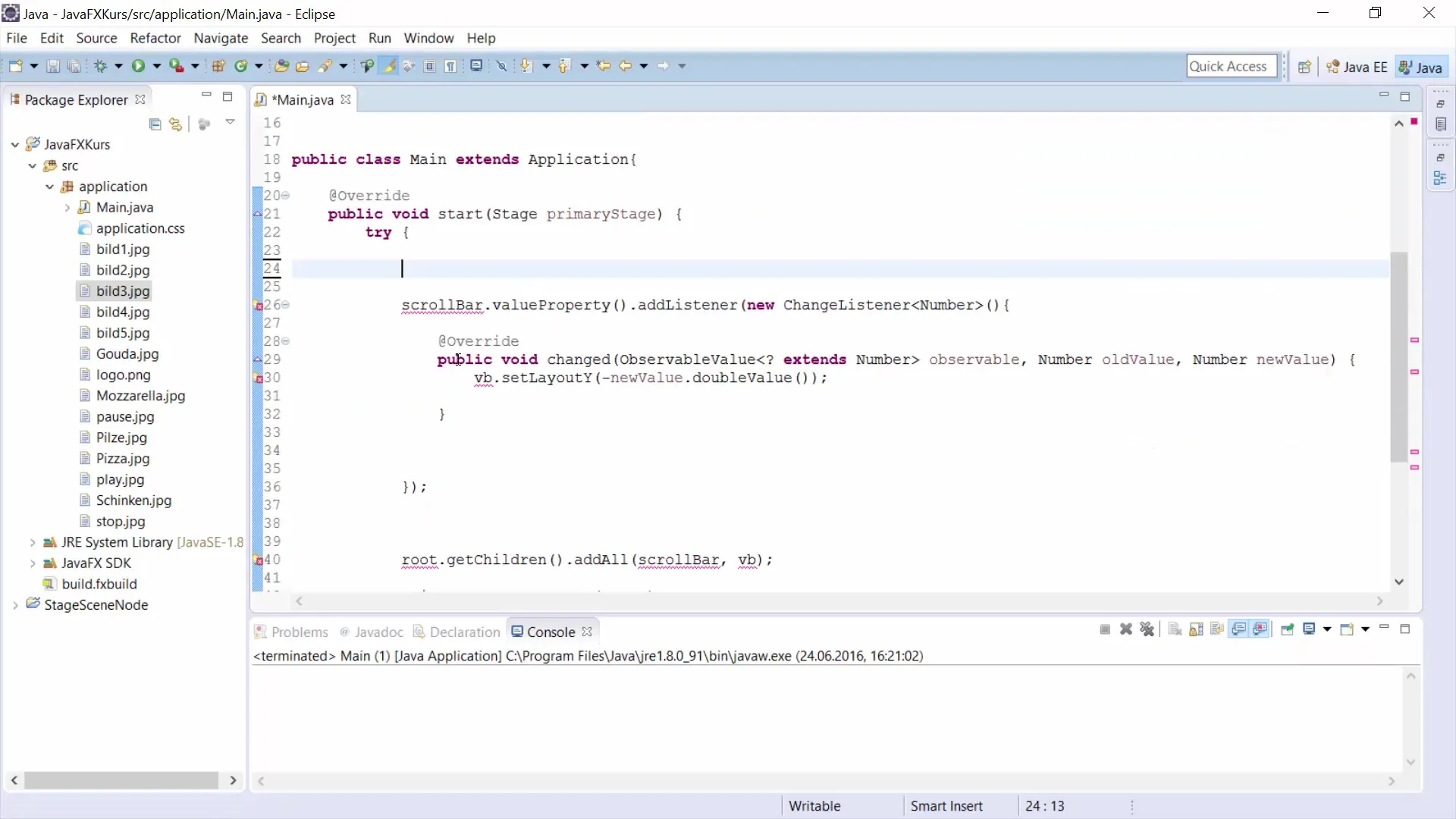
You can define the content of the ScrollPane with the setContent() method, which accepts the ImageView element as a parameter. With new ImageView(image), you display the image in the ImageView.
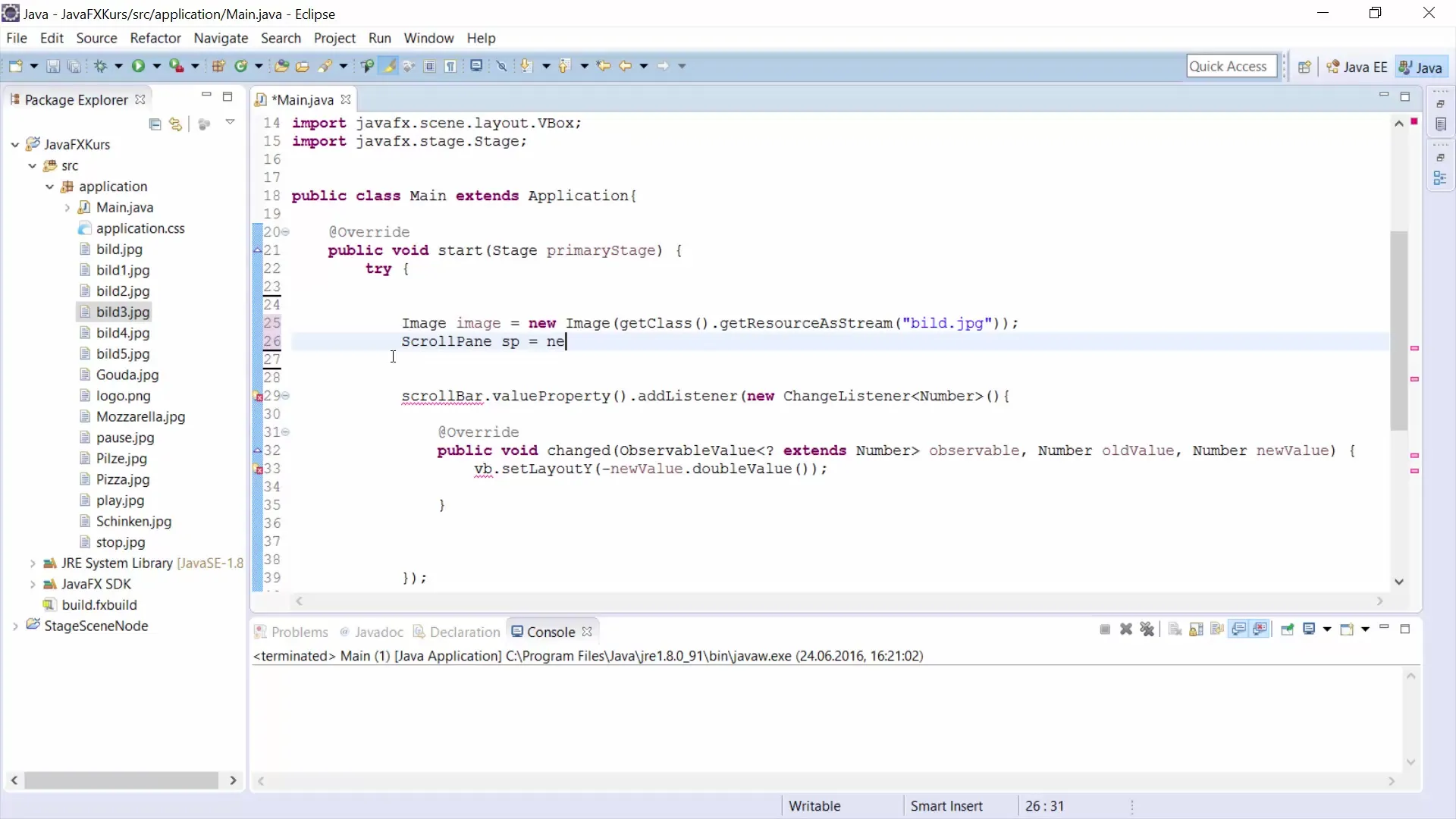
To dynamically output values while scrolling, you can use listeners. Add a listener to the ScrollPane that displays the old and new values during a scroll event. You can achieve this with setOnScroll(e -> {... }) and output the values using System.out.
Now create a new Scene into which your ScrollPane will be integrated. Use Scene scene = new Scene(sp, 300, 400) to define the dimensions. This will display the ScrollPane in the scene.
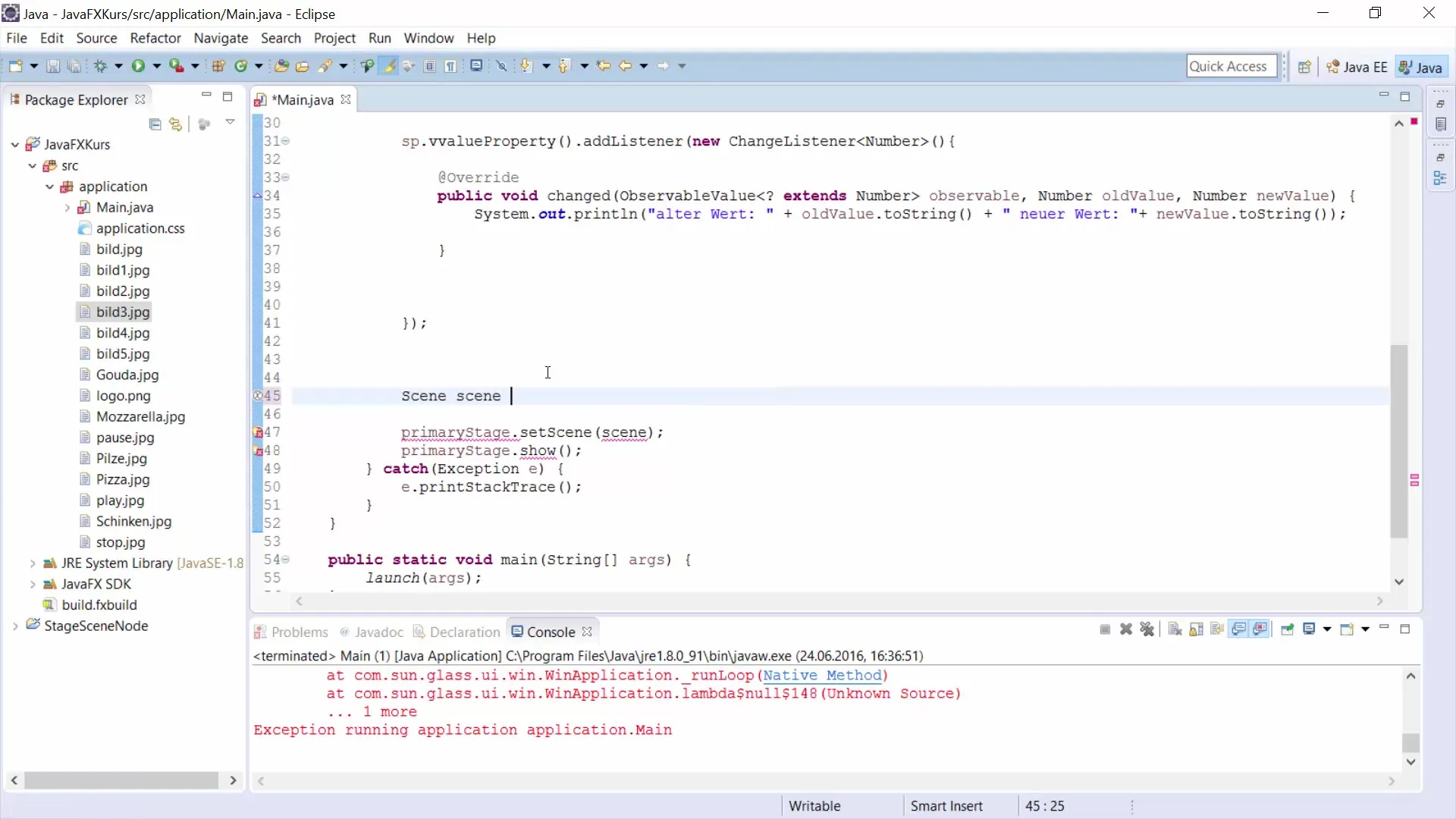
If you have correctly added the image or ScrollPane, you can now display the window. Use primaryStage.setScene(scene) to show the scene on the stage. Then you can make the window visible with primaryStage.show().
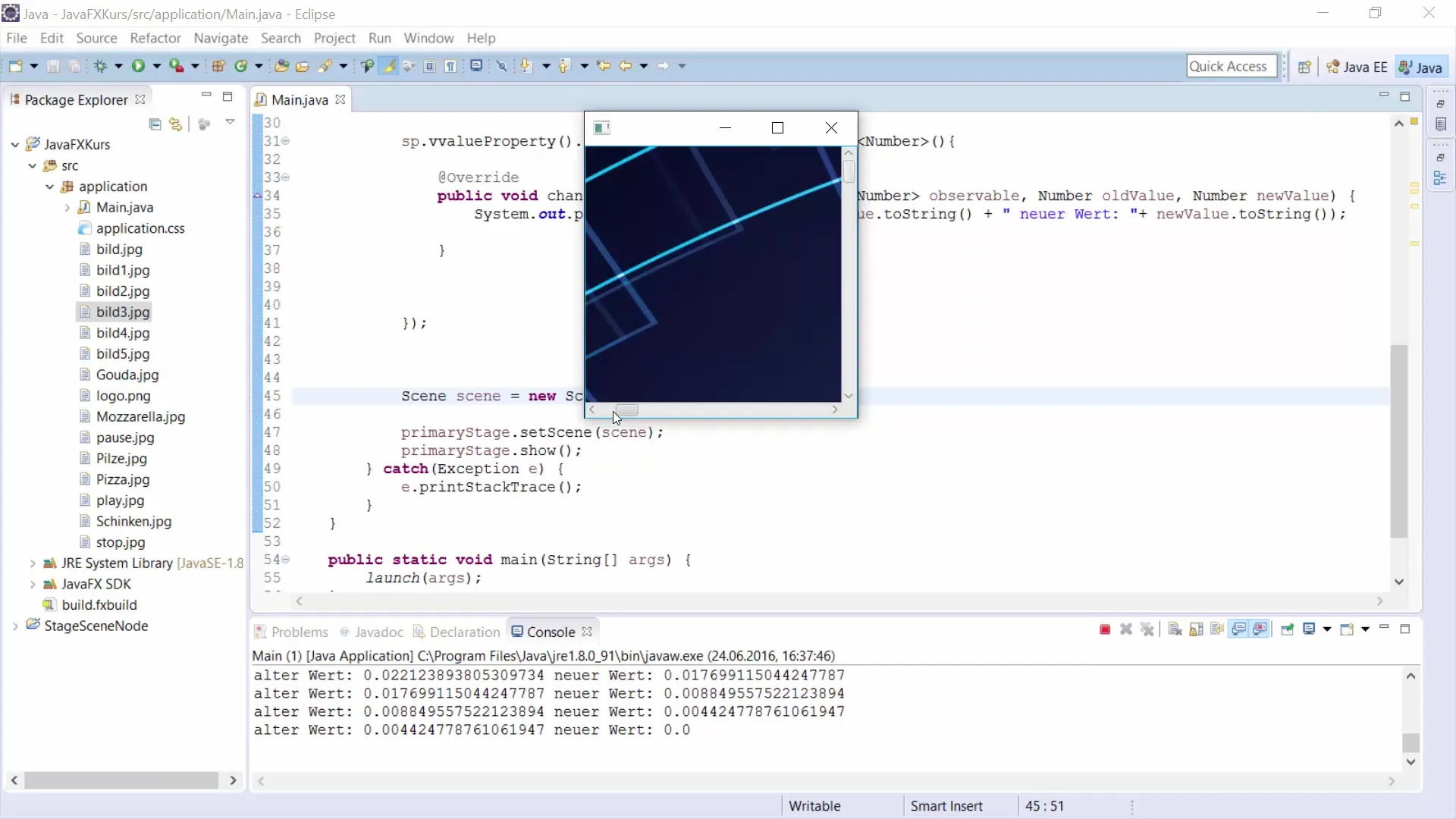
To control the scroll direction, you can set the bar policy of your ScrollPane. For example, if you want to set the horizontal scroll bar to "never show," use sp.setHbarPolicy(ScrollPane.ScrollBarPolicy.NEVER).
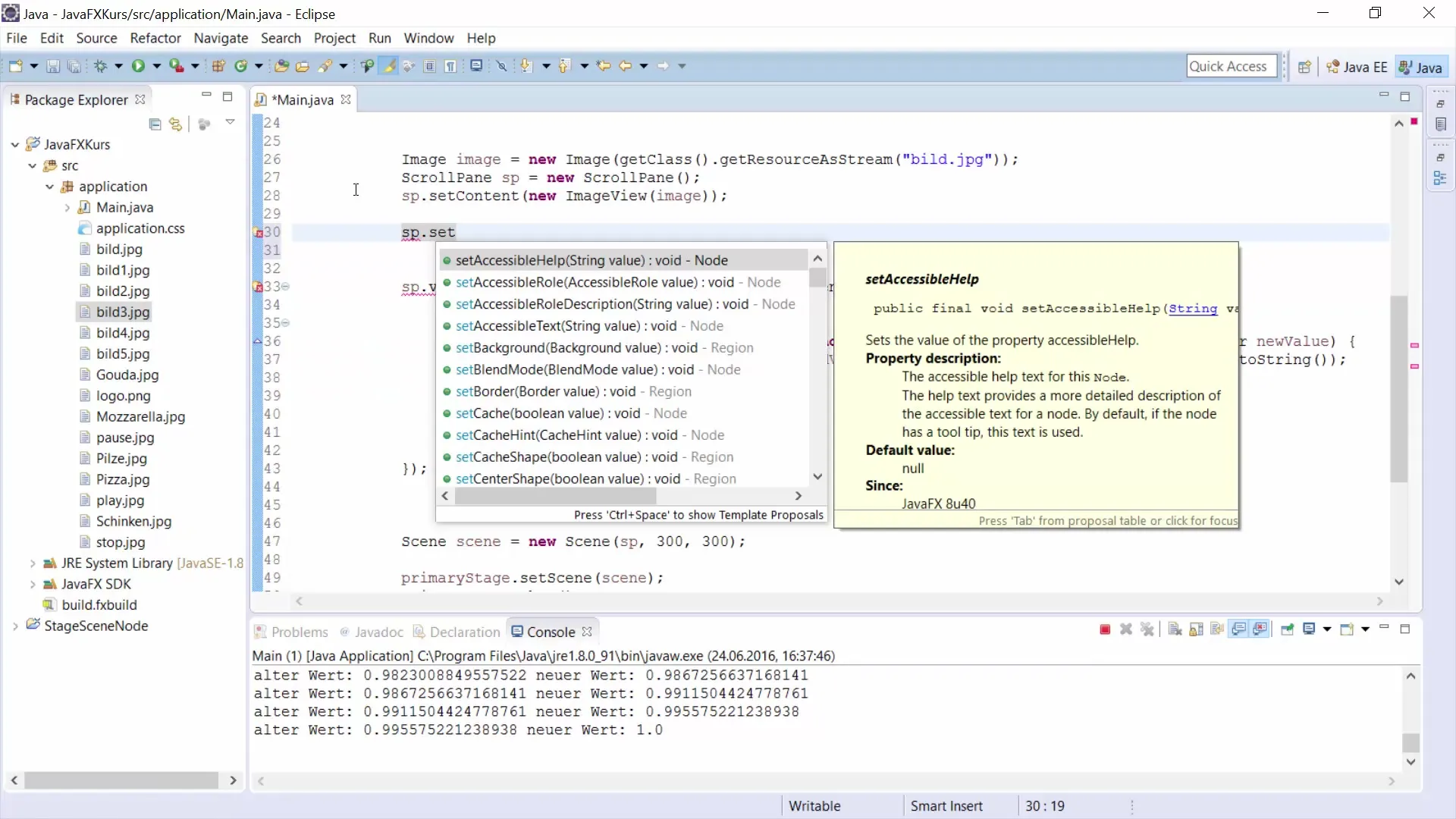
Once you have made these settings, the horizontal scroll bar will no longer be displayed, meaning that only vertical scrolling is possible. By configuring the scroll bars as needed, you have control over the user experience.
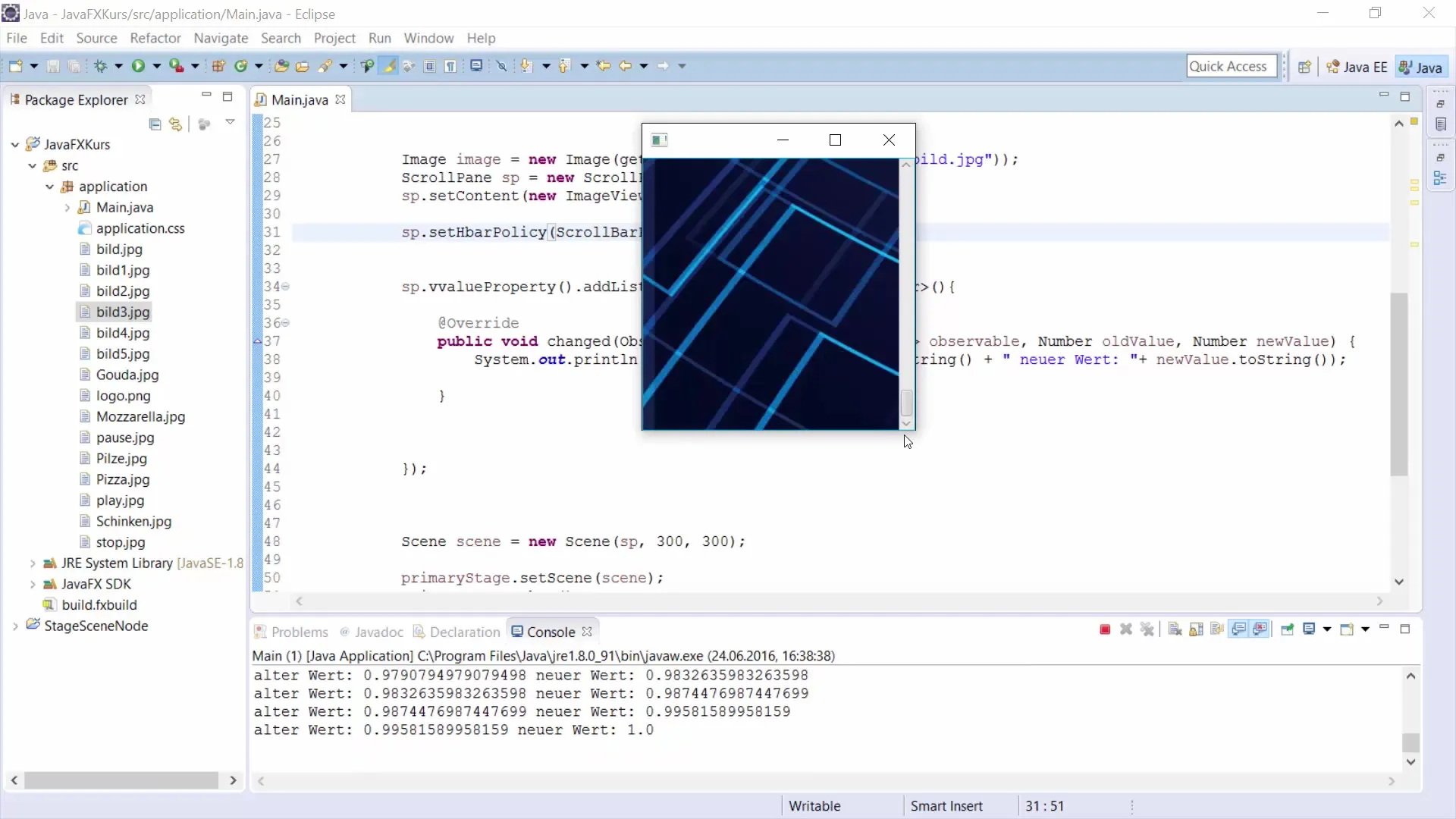
This concludes the introduction to ScrollPane implementation. This is how you can effectively and flexibly use ScrollPane in your JavaFX applications to create a user-friendly and well-organized interface.
Summary – Guide to Using ScrollPane in JavaFX for GUI Development
With this guide, you have now gone through all the steps to implement ScrollPane in your JavaFX application. You have learned how to load images, configure ScrollPane, and use listeners to output dynamic values. With these insights, you can significantly improve the user experience in your application.
Frequently Asked Questions
What is ScrollPane in JavaFX?ScrollPane in JavaFX is a container that allows you to scroll content when it is larger than the display area.
How do I insert an image into ScrollPane?You load the image with new Image(getClass().getResourceAsStream("yourImagePath")) and add it to an ImageView, which is then inserted into the ScrollPane.
How can I control the scroll bars?You can configure the visibility of the scroll bars using the setHbarPolicy() and setVbarPolicy() methods to always show, never show, or show only as needed.
Is it possible to scroll in both directions with ScrollPane?Yes, by default, a ScrollPane can scroll both vertically and horizontally unless you configure one of the directions to "do not show."